Lecture 25 : Binary Search Tree (BST) Sort
Summary
TLDRThe video script introduces the concept of binary search tree (BST) sorting, explaining how BSTs can be used to create a sorting algorithm. It defines a BST and its properties, contrasting balanced and unbalanced trees. The script then details the BST sort algorithm, which involves inserting elements into a BST and performing an inorder traversal to obtain a sorted sequence. It also discusses the time complexity of building a BST, which can range from O(n log n) in the best case to O(n^2) in the worst case. The script concludes by drawing parallels between BST sort and quicksort, noting their similar time complexities and the number of comparisons involved. The next class will explore the randomized version of BST sort and the expected balanced height of a randomly built BST.
Takeaways
- ๐ A binary search tree (BST) is a tree data structure where each node has a key value, and all nodes in the left subtree have keys less than the node's key, while all nodes in the right subtree have keys greater than the node's key.
- ๐ BST sort is a sorting algorithm that utilizes the properties of a binary search tree to order elements. It involves inserting elements into a BST and then performing an inorder traversal to retrieve them in sorted order.
- ๐ The efficiency of a BST depends on its balance. A balanced BST has a height of log n for n nodes, allowing for search and query operations to be performed in logarithmic time.
- ๐ The process of BST sort involves initializing an empty BST, inserting each element of the array into the BST, and then conducting an inorder traversal of the BST to obtain the sorted elements.
- ๐ณ The insertion process in a BST involves starting at the root and moving left or right depending on whether the element to be inserted is less than or greater than the current node's key, continuing until a null position is found where the new element is inserted.
- ๐ The time complexity for an inorder tree walk, which is used to extract the sorted elements from the BST, is O(n), as each node is visited exactly once.
- โณ The time complexity to build the BST depends on the nature of the input data. In the best case, with a balanced tree, it is O(n log n), while in the worst case, with an unbalanced tree, it can be O(n^2).
- ๐ The lower bound for building a BST is O(n log n) because even in the best-case scenario with a balanced tree, each insertion requires a number of comparisons proportional to the height of the tree.
- ๐ข The worst-case scenario for building a BST occurs when the input data is already sorted or reverse sorted, leading to a degenerate tree structure where each insertion requires a comparison with all previously inserted elements.
- ๐ There is a relationship between the performance of BST sort and quicksort. Both algorithms have the same time complexity in their best and worst cases, and they perform the same number of comparisons when using a stable partitioning method.
- ๐ฒ The next topic to be discussed is the randomized version of BST sort, which is expected to have a balanced tree height of log n on average, making it more efficient for sorting.
Q & A
What is a binary search tree (BST)?
-A binary search tree (BST) is a tree data structure with the property that for any given node with a key value X, all nodes in the left subtree have key values less than X, and all nodes in the right subtree have key values greater than X.
What are the characteristics of a balanced BST?
-A balanced BST is one where the height of the tree is log(n), meaning that it maintains a relatively equal distribution of nodes across its levels, ensuring efficient search and insertion operations.
What is the difference between a good and a bad binary search tree?
-A good binary search tree is balanced, where the height is log(n). A bad binary search tree is unbalanced, where the height can be as large as n, leading to inefficient operations.
Why is a balanced BST preferred?
-A balanced BST is preferred because it allows for efficient search, insertion, and deletion operations, all of which can be performed in O(log n) time, as opposed to O(n) in an unbalanced tree.
What is BST sort?
-BST sort is a sorting algorithm that involves inserting elements into a BST and then performing an inorder traversal to retrieve the elements in sorted order.
How does the BST insert operation work?
-The BST insert operation starts with the root and compares the value to be inserted with the current node's value. If the value is less, it moves to the left subtree; if greater, to the right subtree, continuing this process until it finds an appropriate position to insert the new node.
What is an inorder traversal in a BST?
-An inorder traversal in a BST involves visiting the left subtree, then the root node, and finally the right subtree. This traversal method retrieves the elements in sorted order.
What is the time complexity of inorder traversal in a BST?
-The time complexity of inorder traversal in a BST is O(n), where n is the number of nodes, since each node is visited exactly once.
What is the time complexity of building a BST?
-The time complexity of building a BST depends on the order of insertion: it is O(n log n) for a balanced tree and O(n^2) for a completely unbalanced tree.
How does the time complexity of BST sort compare to quicksort?
-Both BST sort and quicksort have a worst-case time complexity of O(n^2) and a best-case time complexity of O(n log n). The similarity in time complexity arises because both algorithms perform a similar number of comparisons.
Outlines
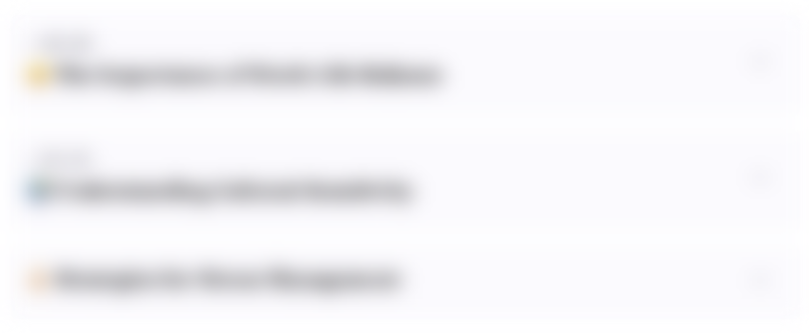
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
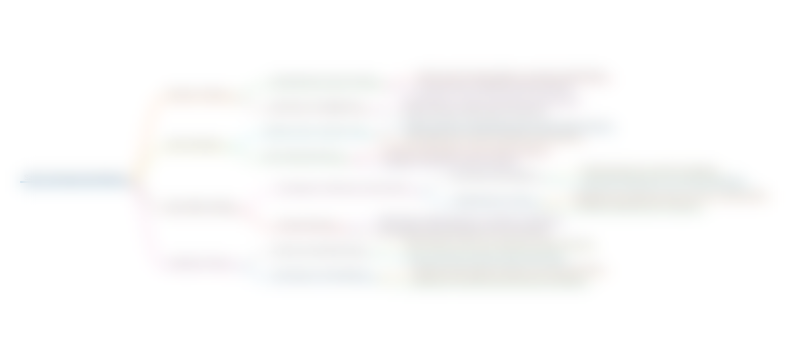
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
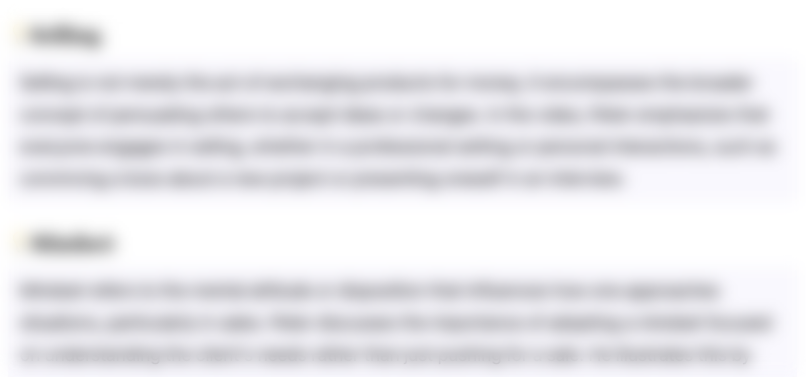
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
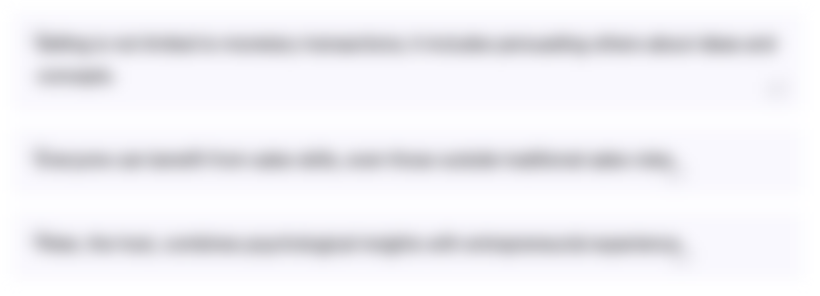
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
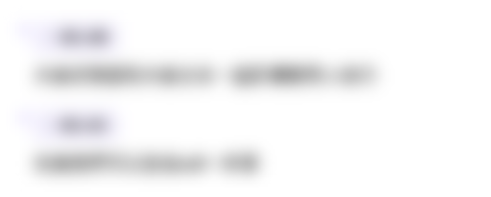
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
5.0 / 5 (0 votes)