C# Logging In 100 seconds
Summary
TLDRThis video explains how to use ILogger, a flexible logging interface in C# applications. It walks through injecting ILogger into classes and logging messages with varying severity levels, such as informational or error logs. Configuration is typically done in the appsettings.json file, with options to send logs to different providers like the console, Windows Event Viewer, or Azure services. The video also highlights the benefits of structured logging, which enables better querying by logging not only messages but also specific parameters for more efficient troubleshooting.
Takeaways
- 🔧 ILogger is a simple but powerful interface for logging messages in C# applications.
- 📥 To use ILogger, inject an instance of it into your class's constructor.
- ⚙️ ILogger is a generic interface, requiring a type parameter that becomes the log's category name.
- 📝 You can log messages using methods like 'LogInformation' for info messages and 'LogError' for errors (including exception details).
- 📊 Log methods have different severity levels, helping to quickly identify relevant logs when troubleshooting.
- 📂 Logging is usually configured in the appsettings.json file, allowing you to enable logs based on categories and severity levels.
- 💻 By default, logs are sent to the console, but you can configure other logging providers, such as Windows Event Viewer, Azure App Service, or Azure Application Insights.
- 🔑 The Log APIs support message templates, enabling structured logging with fields instead of plain messages.
- 🔍 Structured logging allows querying logs by specific fields, making filtering and troubleshooting more efficient.
- 🚀 Using ILogger in C# applications ensures robust logging, which is essential for debugging and improving application stability.
Q & A
What is ILogger in C#?
-ILogger is a generic interface in C# used for logging messages in applications. It helps in recording log messages with various severity levels like information, error, and more.
How do you use ILogger in a class?
-To use ILogger in a class, you inject an instance of ILogger into the class’s constructor. You also need to specify a type parameter for the ILogger interface, which becomes the category name for logging messages.
What are the different severity levels in ILogger?
-ILogger provides methods like LogInformation, LogError, etc., to log messages with different severity levels. These severity levels help in categorizing logs based on their importance or relevance.
How is logging usually configured in a C# application?
-Logging is typically configured in the appsettings.json file, where you can set various categories and severity levels to control which logs are enabled for the application.
Where are logs sent by default when using ILogger?
-By default, logs are sent to the console. However, this can be configured to send logs to different providers like Windows Event Viewer, Azure App Service, or Azure Application Insights.
What is a logging provider, and why is it important?
-A logging provider is a destination where log messages are sent. It is important because it allows you to control where your logs are stored, such as in the console, cloud services, or other monitoring tools.
What is structured logging, and how does it work in ILogger?
-Structured logging allows log messages to include parameters that are stored as fields. This makes querying logs easier because you can filter log entries by these fields, such as player IDs or other specific details.
What is the benefit of using message templates in ILogger?
-Message templates in ILogger allow you to create structured log messages. This helps with storing parameters separately, enabling easier querying and analysis of logs based on specific fields.
Can ILogger be used with multiple logging providers?
-Yes, ILogger supports multiple logging providers. This allows you to send logs to various locations such as the console, Windows Event Viewer, Azure services, and more, depending on your configuration.
Why is having good logging important in a C# application?
-Good logging is crucial for troubleshooting and debugging. Well-structured logs with different severity levels help you quickly identify and isolate issues, making it easier to maintain and optimize the application.
Outlines
🔧 Introduction to ILogger in C# Applications
This paragraph introduces ILogger, a simple yet powerful interface for logging messages in C# applications. It explains how to start using ILogger by injecting it into a class constructor and mentions that ILogger is a generic interface requiring a type parameter, which serves as the category name for the logs. The paragraph also describes various log methods, such as `LogInformation` and `LogError`, which handle logs of different severity levels. This feature helps developers quickly isolate important logs during troubleshooting.
⚙️ Configuring Logging in appsettings.json
The paragraph details how logging is usually configured within the `appsettings.json` file in C# applications. Developers can use combinations of categories and severity levels to define which logs should be captured. It emphasizes the importance of setting the correct configurations to ensure relevant logs are generated based on the application's requirements.
📤 Exploring Logging Providers
This section explains the concept of logging providers and how logs are sent to different destinations. By default, logs are sent to the console, but other logging providers like Windows Event Viewer, Azure App Service, and Azure Application Insights can be configured. These providers offer more advanced logging capabilities based on specific project needs.
📝 Leveraging Message Templates for Structured Logging
The paragraph introduces message templates, which allow developers to write log messages in a more structured manner. Instead of simple string messages, structured logging captures both the message and parameters as fields, making it easier to filter and query logs by specific fields, such as player IDs. This feature enhances troubleshooting by providing more granular details in the logs.
🚀 Conclusion: The Importance of ILogger in C#
The final paragraph encourages developers to incorporate ILogger into their C# applications. It highlights the benefits of well-structured logging, especially when troubleshooting. Good logs can be a lifesaver during critical situations, making ILogger a crucial tool for developers. The paragraph ends with a call-to-action for viewers to like the video and stay tuned for more content.
Mindmap
Keywords
💡ILogger
💡Log Methods
💡Severity Levels
💡Structured Logging
💡Log Providers
💡AppSettings.json
💡Message Templates
💡Troubleshooting
💡Exception Details
💡Azure Application Insights
Highlights
ILogger is a simple yet powerful interface for logging messages in C# applications.
To get started, inject an instance of ILogger into your class via the constructor.
ILogger is a generic interface, requiring a type parameter that becomes the category name for logging messages.
Log methods like LogInformation and LogError allow you to log messages with varying severity levels.
LogError can include exception details to provide more context in error logs.
Logging is configured in the appsettings.json file, where you can set combinations of categories and severity levels.
Logs are sent to default providers like the console, but additional providers like Windows Event Viewer, Seq, Azure App Service, or Azure Application Insights can be configured.
ILogger supports structured logging, where message templates are used instead of simple string logs.
Structured logging allows logging providers to store both the log messages and parameters as fields.
Using structured logging makes querying logs more powerful, allowing you to filter by specific fields like Player ID.
Structured logging enhances the ability to craft detailed queries for more effective troubleshooting.
Having well-structured logs saves time and effort when resolving issues within your C# applications.
ILogger allows for flexibility in logging severity, making it easier to isolate relevant logs during troubleshooting.
You can configure logging to suit the needs of your application, choosing what to log and where to store those logs.
Structured logging is particularly useful when working with cloud-based providers like Azure, enabling richer querying capabilities.
Transcripts
meet eye logger the simple but powerful
interface to lock messages in c-sharp
applications to get started inject an
instance of eye logger into your class
va's Constructor notice that ilogger is
actually a genetic interface so you need
to specify a type parameter which
becomes the category name to log
messages you can use one of the many log
methods like log information to lock an
informational message or clock error to
lock an error which can also include
exception details these different
methods red locks with different
severity levels so you can quickly
isolate the most relevant logs when
troubleshooting your app logging is
usually configured in the app
settings.json file where you can use a
combination of categories on severity
levels to tell the app which logs to
neighbor now where do these Lots go to
well now for login providers come into
play by default log will be sent to your
console but that is just the default
login provider you can easily configure
other login providers to send your logs
to places like the windows even viewer
seek Azure app service or even Azure
application insights the log apis also
support message templates so instead of
writing your messages like this
you can write them like this
allows you to take advantage of
structured logging which allows login
providers to store not just the log
messages but also the parameters
themselves as Fields this is incredibly
powerful because now when you query your
logs you can quickly craft a query that
filters by one of those fields like the
player ID here and you can do similar
things with any other login provider so
don't forget to use ilogger in your next
C sharp application having good logs in
plot will save your day if you want to
see more short videos like this make
sure you hit like button thanks for
watching and I'll see you in the next
one
関連動画をさらに表示

The Logging Everyone Should Be Using in .NET

Beyond Console Log in 100 Seconds

#17 Event Binding | Angular Components & Directives | A Complete Angular Course
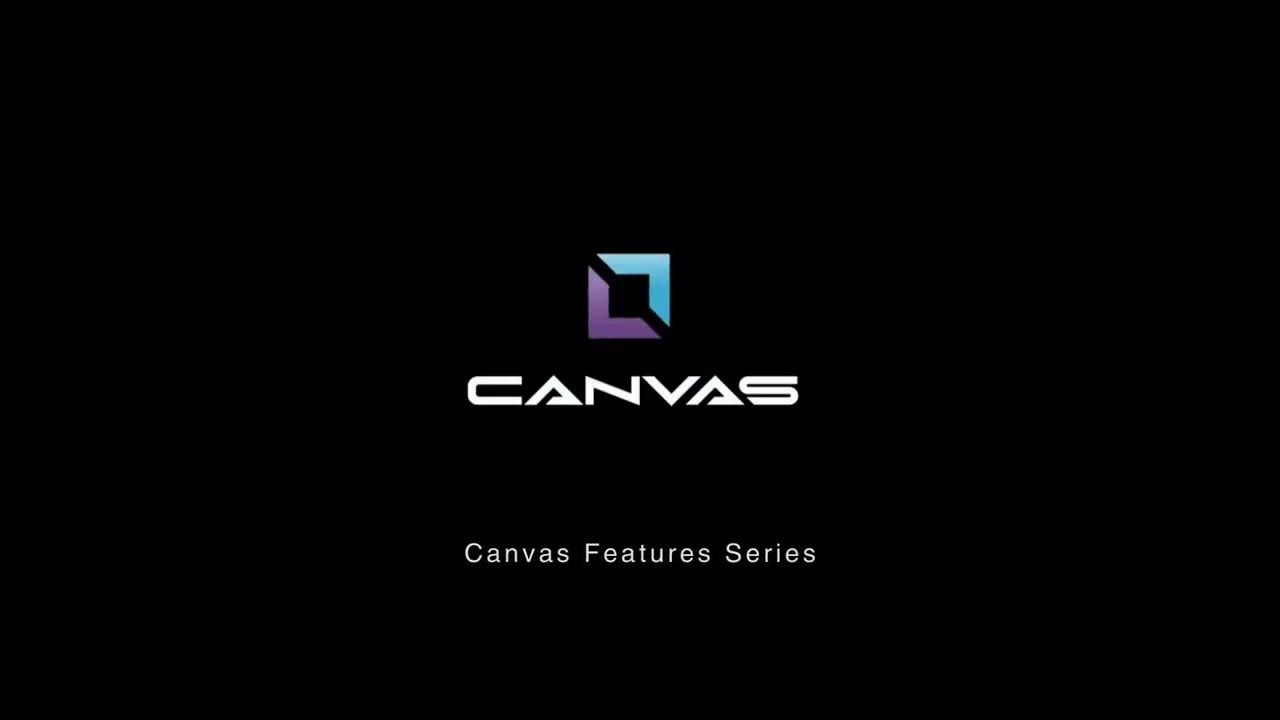
Canvas Feature Series - Data Log (Historian Feature)
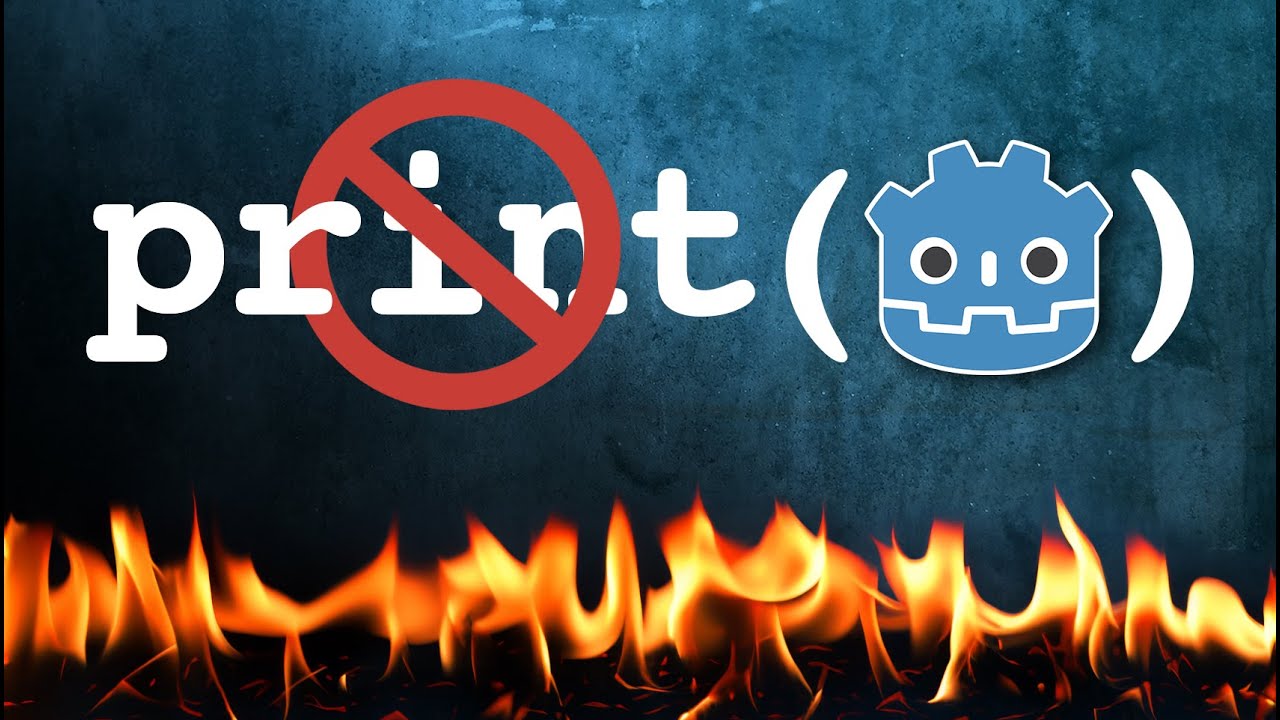
Godot Debugging Techniques EVERY Dev Should Know

M-x Compile: A Deep Dive into Compiling Code with Emacs
5.0 / 5 (0 votes)