C Programming (Important Questions Set 1)
Summary
TLDRThis video script discusses various C programming concepts, including the behavior of printf functions, character data types, and integer arithmetic. It explains how printf can print strings and return the number of characters printed, the impact of using %10s for formatting strings, and the arithmetic modulo operation with character overflow. Additionally, it covers the declaration of integers with modifiers like signed and unsigned, and how the internal representation of integers in computers affects the output of printf statements, emphasizing the importance of careful code analysis for exams like GATE.
Takeaways
- 💻 The script discusses a C program fragment involving nested printf functions and explains their output.
- 🔢 The outer printf function prints the number of characters printed by the inner printf function, which prints 'Hello World!'.
- 📏 The script explains the use of format specifiers like %d for integers and %s for strings in printf functions.
- 📑 It highlights the importance of including the null character when counting the total number of characters in a string.
- 📐 The script demonstrates how printf can be used with padding (%10s) to control the width of the output.
- 🔄 The script explores the concept of data types and arithmetic operations, particularly with character variables.
- 🔒 It explains how character variables can only hold values up to 255 and uses modulo arithmetic to show how values exceeding this are handled.
- 📝 The script discusses the declaration of integer variables in C, including signed and unsigned integers.
- 🔄 It clarifies that omitting the data type for a variable defaults to int, which is implicitly assumed by the compiler.
- 🔢 The script explains the internal representation of negative numbers in computers using 2's complement form.
- 💡 It emphasizes the importance of carefully reading each line of code, especially for understanding questions in competitive exams like GATE.
Q & A
Question 1: What does the first printf function in the program do?
-The first printf function prints 'Hello World!' on the screen, followed by the number of characters in the string, which is 12. Hence, the output is 'Hello World!12'.
Question 2: Why does the second printf function use '%10s' instead of '%s'?
-The '%10s' format specifier ensures that the string is printed within 10 characters wide. Since 'Hello' has 5 characters, 5 white spaces are printed first, followed by 'Hello'. This is used to align the text in a wider field.
Question 3: How does the character variable behave when assigned a value exceeding its range?
-When a character variable is assigned a value exceeding its range (which is 255 for 8 bits), it follows arithmetic modulo 256. For example, assigning 265 results in 265 mod 256, which equals 9. Thus, the value 9 is printed.
Question 4: How does a signed integer differ from an unsigned integer in the context of printf?
-A signed integer allows negative values, while an unsigned integer only handles positive values. When using '%u', the printf function interprets the value as unsigned, potentially printing a large number instead of a negative value, as it interprets the binary form differently.
Question 5: Why does the program print '4294967293' when using '%u'?
-The value -3 is represented in two's complement form, and when printed as an unsigned integer using '%u', the value is interpreted as 4294967293 due to how unsigned integers handle binary representation on a 4-byte machine.
Question 6: What is the significance of arithmetic modulo 2 raised to the power n in the context of character data types?
-For a character variable, which is 8 bits long, the maximum value it can hold is 255 (2^8 - 1). If a value exceeds this range, it wraps around according to modulo arithmetic (value mod 256).
Question 7: Why is 'signed i;' considered a correct definition?
-Even though 'int' is not explicitly mentioned, the compiler implicitly assumes the 'int' data type. Therefore, 'signed i;' is equivalent to 'signed int i;', making it a valid declaration.
Question 8: What is the internal representation of negative integers like -3 in computers?
-Negative integers are represented using two's complement. To get the two's complement of a number, first find the one's complement (invert the bits) and then add 1 to the result. This allows negative numbers to be handled in binary form.
Question 9: What happens if you mix signed and unsigned integers in arithmetic operations?
-When you mix signed and unsigned integers, the result may be unexpected because the unsigned integer takes precedence in most cases, leading to a potential misinterpretation of the result as a large positive number instead of a negative one.
Question 10: Why is it important to carefully read format specifiers like '%d' and '%u' in C programming?
-The format specifier controls how values are printed. '%d' prints signed integers, while '%u' prints unsigned integers. Misusing these specifiers can lead to incorrect output, such as interpreting a negative value as a large positive number, which can lead to confusion.
Outlines
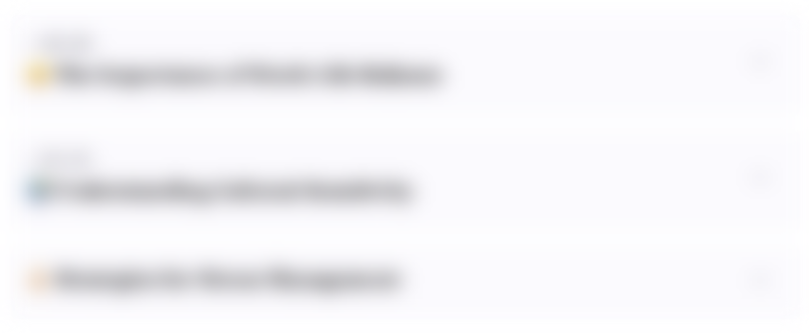
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
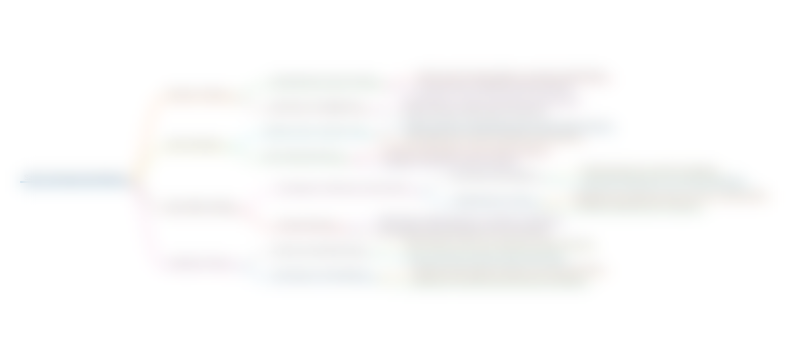
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
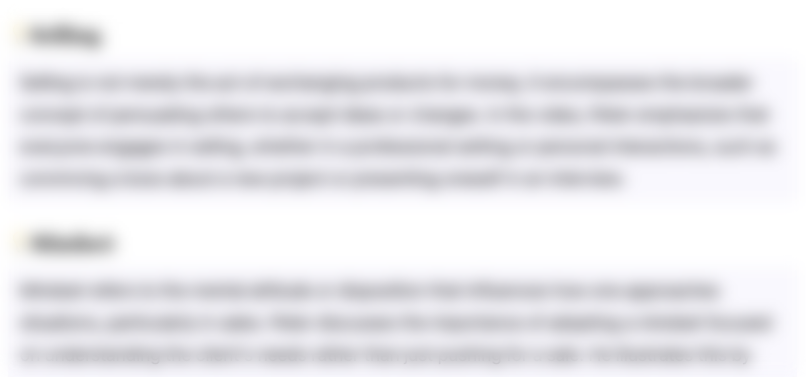
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
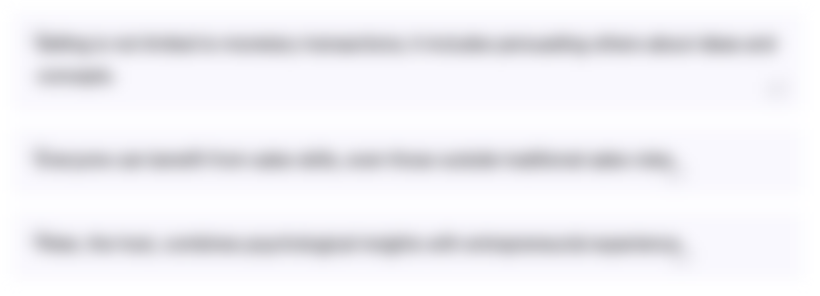
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
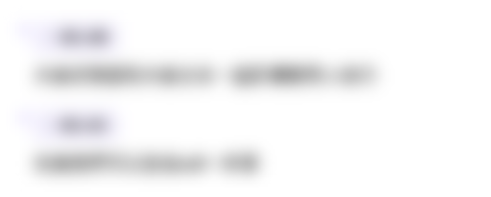
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示

#2 Tipe Data, Variabel, dan Operator

C_75 Pointers in C-part 5 | Pointer Arithmetic (Addition) with program
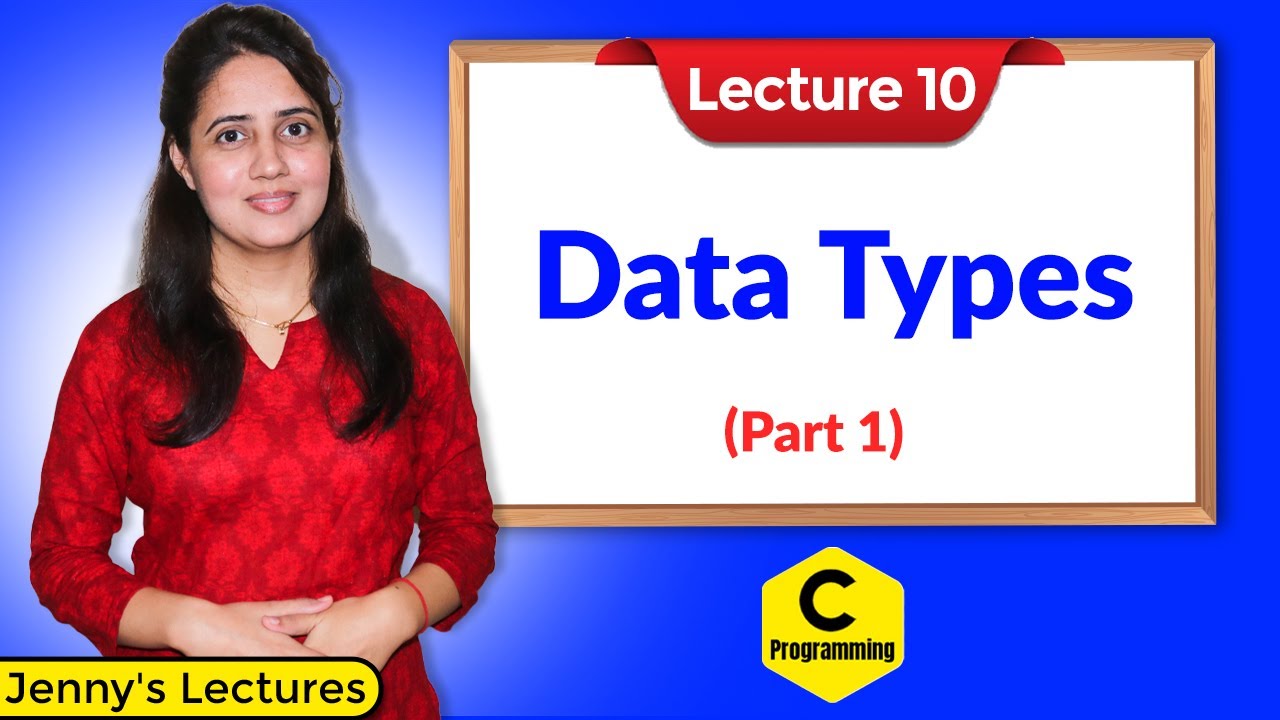
C_10 Data Types in C - Part 1 | C Programming Tutorials for Beginners
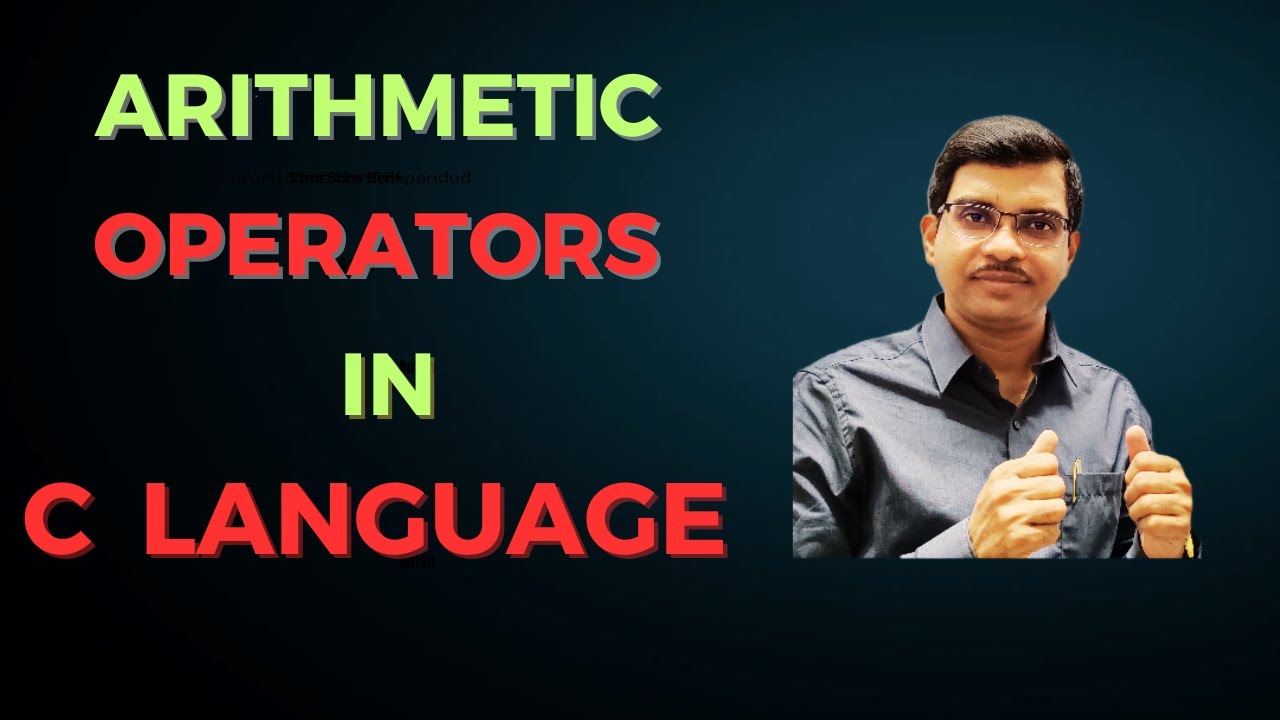
C_12 Arithmetic Operators in C Language | C Programming Tutorials
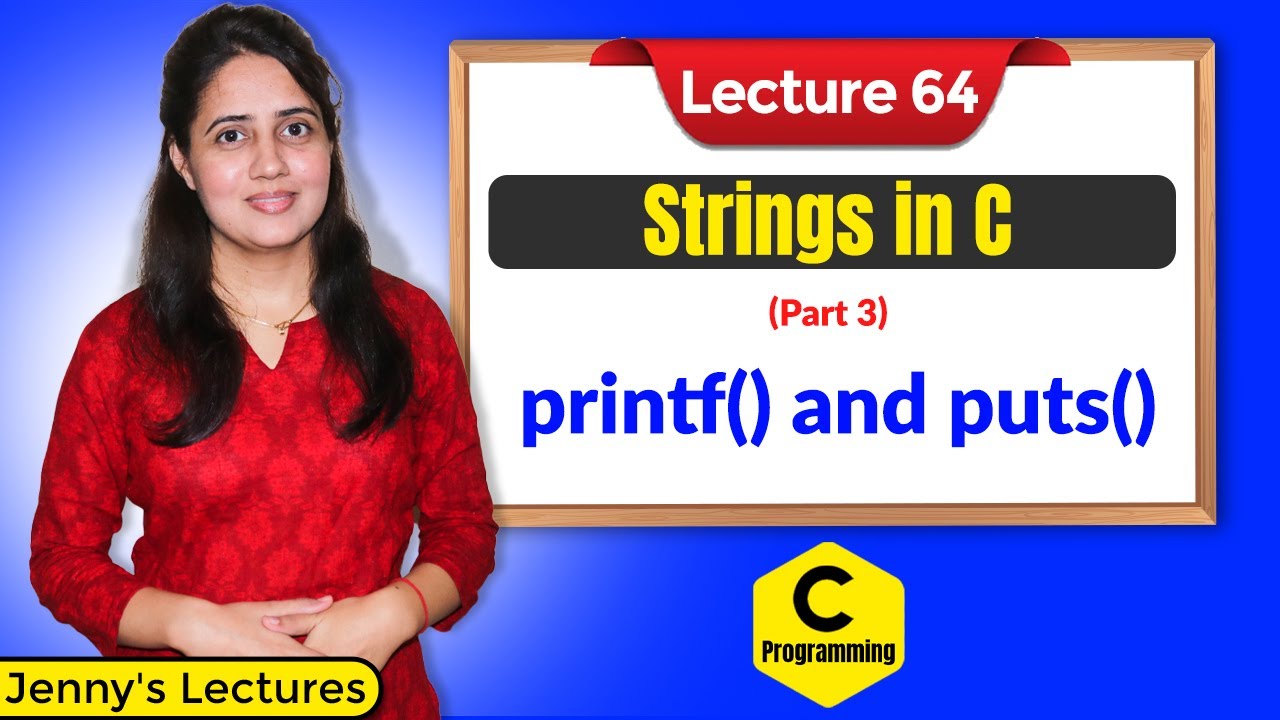
C_64 Strings in C- part 3 | printf and puts function in C
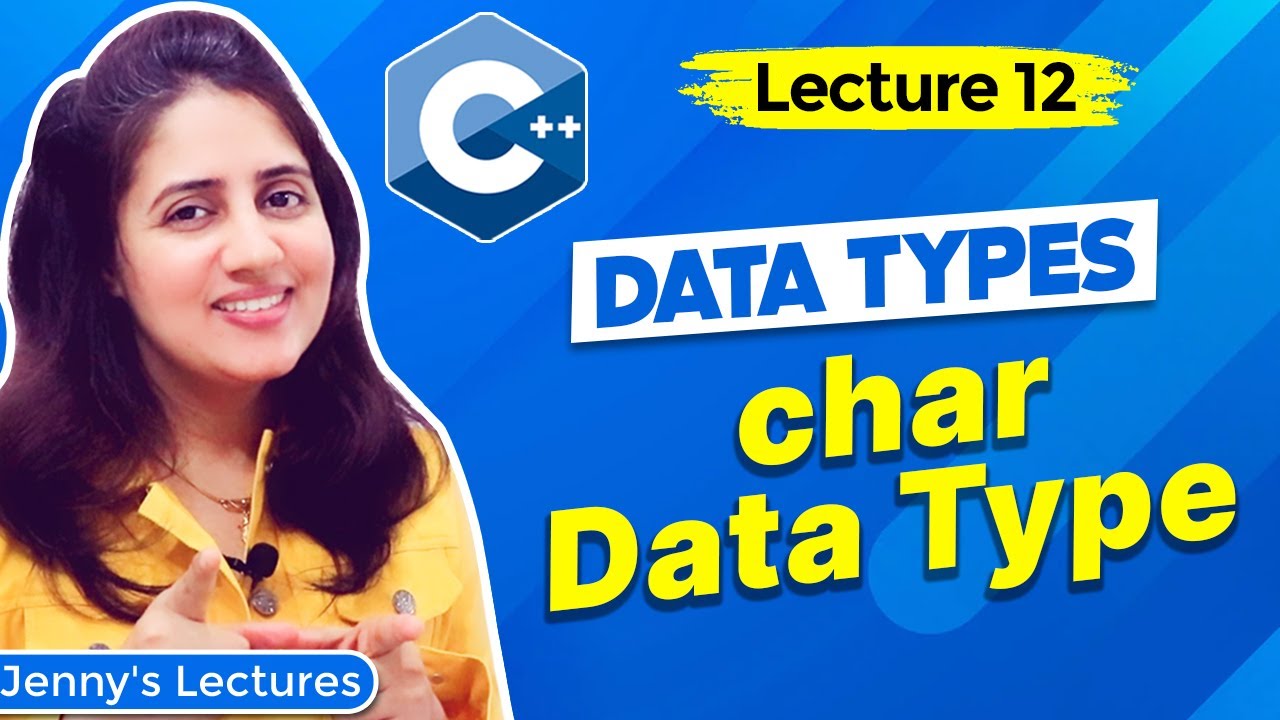
Lec 12: Data Types in C++ - part 3 | char Data Type | C++ Tutorials for Beginners
5.0 / 5 (0 votes)