C_11 Data Types in C - Part 2 | Programming in C
Summary
TLDRIn this video, the instructor explains the intricacies of integer data types in C programming, focusing on signed and unsigned integers. By demonstrating how memory is allocated and how format specifiers (like %d for signed and %u for unsigned integers) affect the output, the video clarifies why certain values may appear unexpectedly. Examples show how overflow works, with values outside the defined range wrapping around, and encourage learners to practice coding to deepen their understanding of these concepts. The instructor also introduces C's integer memory sizes, using practical coding examples to illustrate key points.
Takeaways
- π **Data types in C**: The video discusses various primitive data types like `int`, `float`, `double`, `void`, and `char`, highlighting their uses and characteristics.
- π **Memory size of data types**: The size of data types (e.g., 2 bytes for `int` in some systems) is crucial in determining their range and storage capacity.
- π **Signed vs Unsigned integers**: Signed integers can hold both positive and negative values, while unsigned integers can only hold non-negative values, affecting their value range.
- π **Overflow and underflow in signed integers**: If the value assigned exceeds the range of a signed integer, it will wrap around and result in unexpected negative values when printed with the `%d` specifier.
- π **Range differences**: A signed integer with a value beyond its maximum range (e.g., `32768` for a 2-byte `int`) will lead to incorrect output due to overflow, while the corresponding unsigned type will produce a different result.
- π **Using format specifiers**: The `%d` format specifier is used for signed integers and `%u` for unsigned integers. Using `%u` with a signed integer may result in a seemingly incorrect positive value.
- π **Memory and initialization**: When initializing variables in C, the memory space is allocated based on the data type, but if the value is out of the defined range, the stored value might not be what you expect.
- π **Example of incorrect output**: Initializing an integer beyond its range (e.g., `32768` for a signed `int`) will give a stored value of `-32768` but will print this value incorrectly when `%d` is used.
- π **Practical demonstration**: Multiple examples with different values like `32768`, `-10`, and `0` are used to show how variables behave when assigned values outside the valid range for their data type.
- π **Importance of testing**: It's important to practice with actual code and verify the expected output by running the program on your own system to understand how overflow, underflow, and type conversion work in practice.
Q & A
What is the difference between signed and unsigned integers in C?
-A signed integer can store both positive and negative values, while an unsigned integer can only store positive values (including zero). The range of a signed integer is typically from -32768 to 32767 for a 2-byte integer, while an unsigned integer range is from 0 to 65535.
Why does an initialized signed integer sometimes produce an unexpected output?
-An unexpected output occurs when the value assigned to a signed integer exceeds its range. For example, initializing a signed integer with 32768, which is beyond the positive range of a 2-byte signed integer, will cause it to wrap around to -32768 due to overflow.
What is the role of the format specifier '%d' in C?
-The '%d' format specifier is used to print signed integers in C. It tells the compiler to treat the variable as a signed integer when printing the value, which can be either positive or negative.
What happens when a signed integer exceeds its range and is printed with '%u'?
-When a signed integer exceeds its range and is printed with '%u', which is the format specifier for unsigned integers, the negative value is interpreted as a large positive number due to the way memory is represented for unsigned integers.
How do signed and unsigned integers differ in terms of memory representation?
-Signed integers can store both negative and positive numbers by using one bit for the sign. Unsigned integers, however, only store positive numbers (including zero) and use all bits to represent the magnitude of the value, allowing them to store higher positive values.
What would happen if you initialize an integer with a value greater than its maximum range?
-If you initialize an integer with a value greater than its maximum range, it will cause an overflow. For signed integers, the value will wrap around to the negative side of the range. For unsigned integers, the value will be treated as a very large positive number.
Why is it important to practice using different integer values in C?
-Practicing with different integer values is important because it helps you understand how data types behave when their values exceed or fall below their defined ranges. This can help you avoid errors and unexpected results in your programs, especially when working with memory allocation and format specifiers.
What happens if you print a signed integer with a format specifier '%u'?
-When you print a signed integer using the '%u' format specifier (which is for unsigned integers), the signed value is treated as an unsigned number. If the signed integer is negative, its memory representation is interpreted as a large positive number.
What is the correct range for an unsigned 2-byte integer in C?
-The range for an unsigned 2-byte integer is from 0 to 65535, as all bits are used to store positive values, and there is no sign bit.
How do memory addresses relate to the variables declared in C?
-In C, each variable is assigned a specific memory location. The variable name acts as a reference to this memory address. When you assign a value to a variable, it is stored in that memory location, and the program accesses this memory location when retrieving or modifying the variable's value.
Outlines
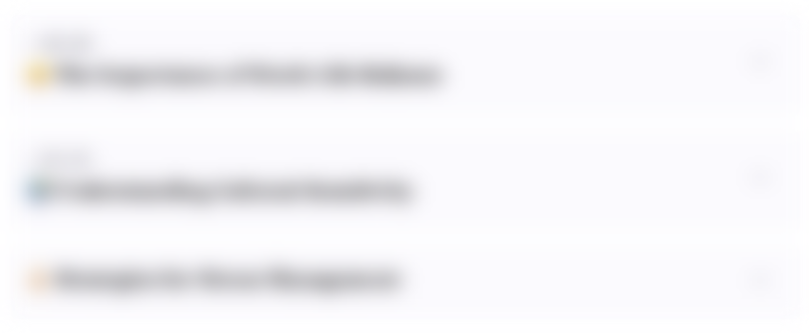
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
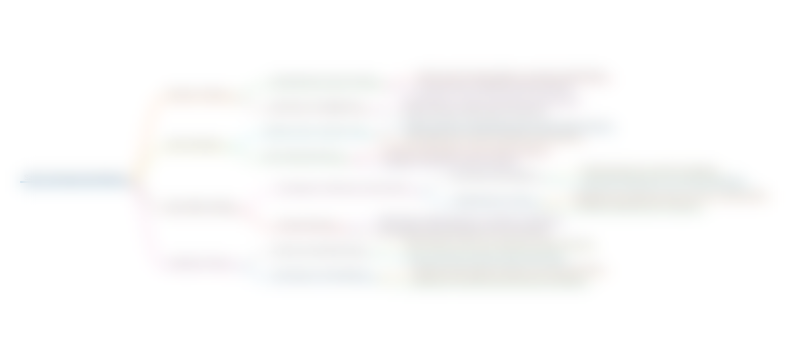
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
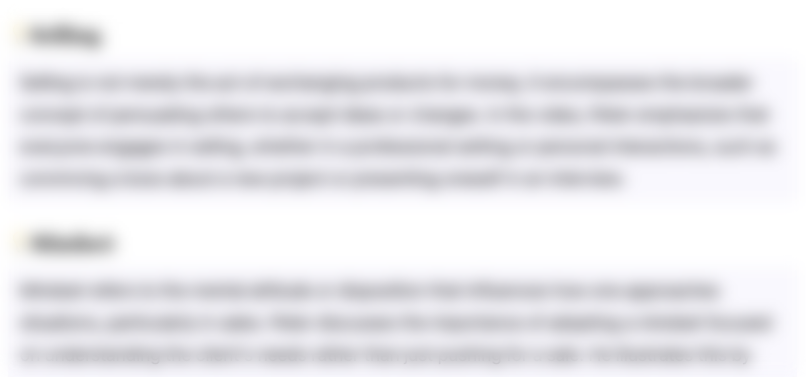
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
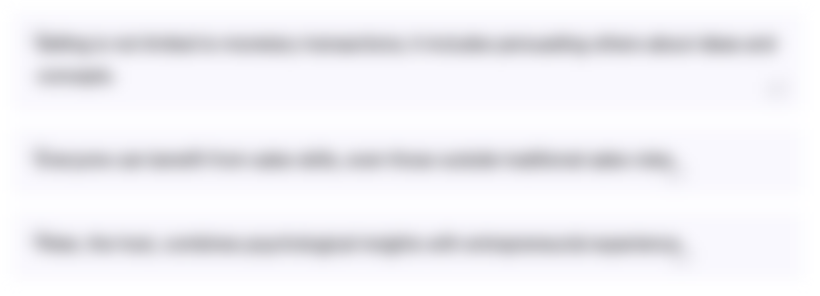
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
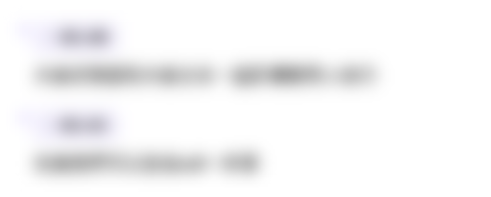
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
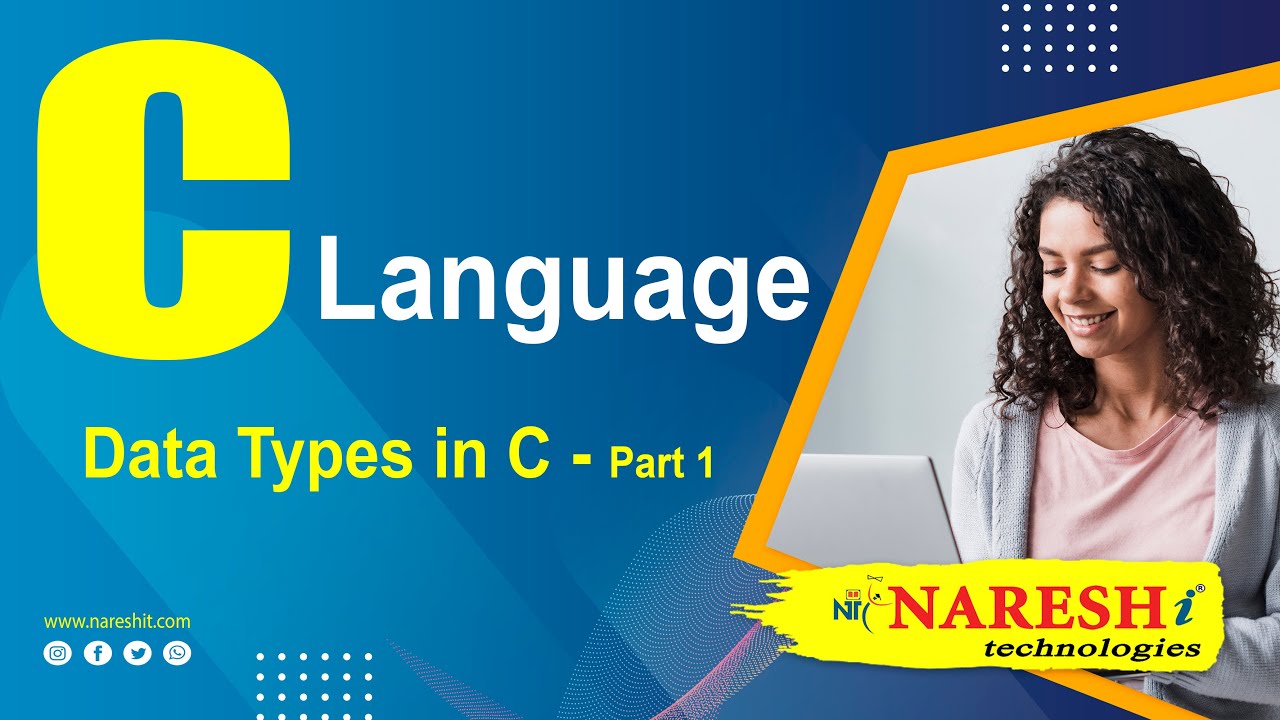
Data Types in C - Part 1 | C Language Tutorial

C Programming (Important Questions Set 1)

Input and Output Addressing in Siemens PLC - Tia Portal Tutorial
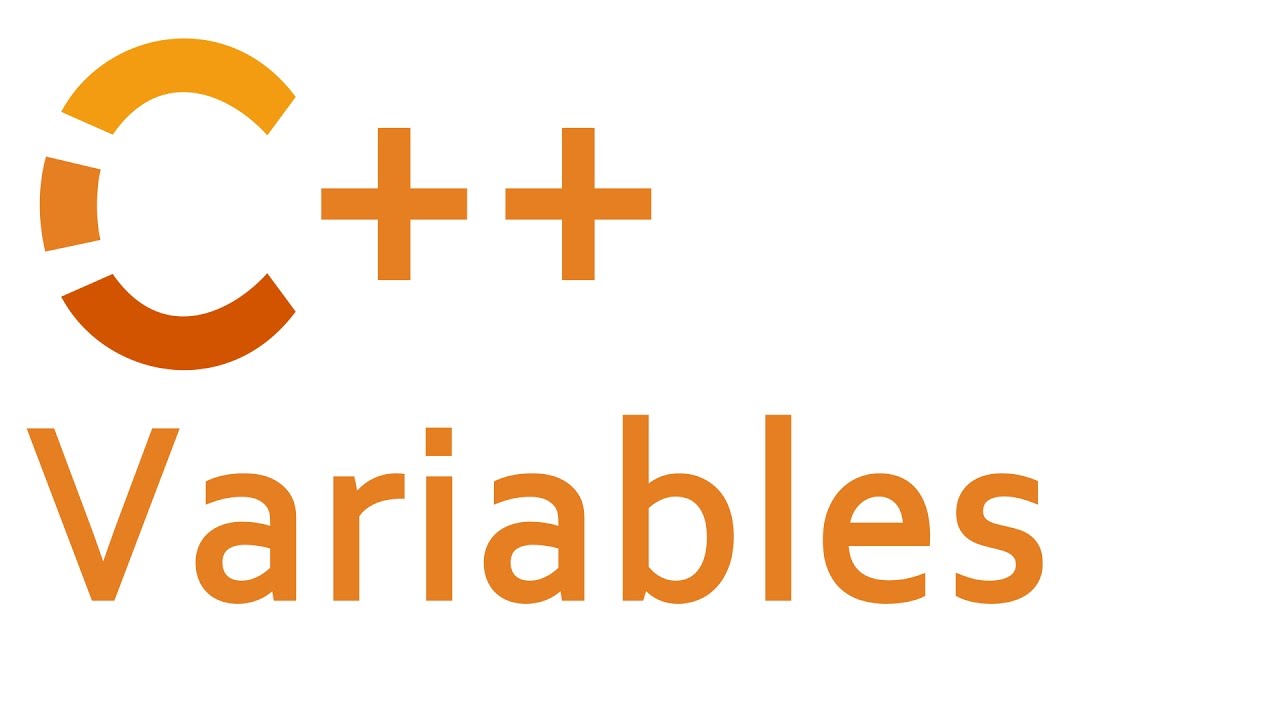
Variables in C++
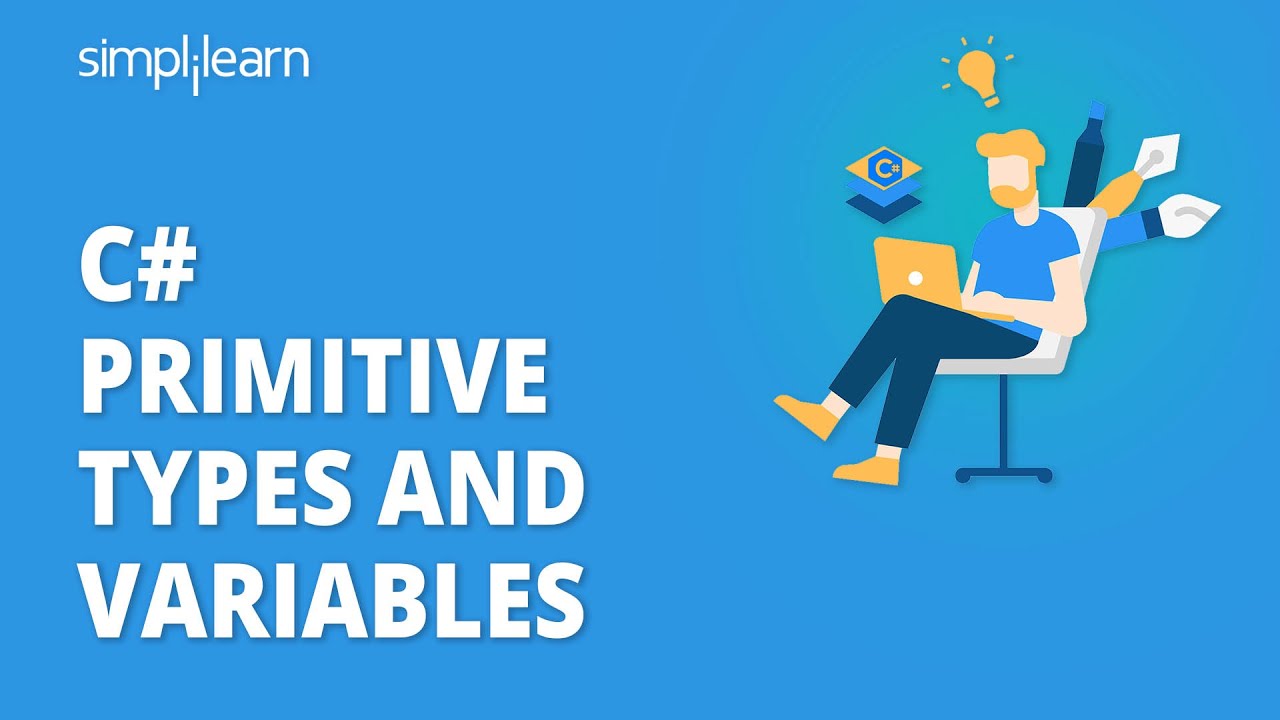
C# Primitive Types and Variables | Datatype Literals and Variables | C# Tutorial | Simplilearn

C_09 Data Types in C Language | C Programming Tutorials
5.0 / 5 (0 votes)