Express JS #5 - Post Requests
Summary
TLDRThis tutorial explains how to create a resource on a backend server using a POST request in Express. It walks through setting up an API to accept user data from the frontend, such as username, password, and email, and saving it to a database or file. The video also demonstrates how to use Thunder Client in VS Code for testing POST requests. It introduces Express middleware to parse JSON request bodies and how to handle response statuses, including returning the created user data and status codes like 201. The tutorial ends by simulating user ID generation in the absence of a database.
Takeaways
- 📝 You can create data on the backend using POST requests, which typically saves data to a database or a file.
- 🌐 When a user fills out a form on the frontend (like a signup form), a POST request is made to the backend when the form is submitted.
- 📦 The data sent from the frontend to the backend during a POST request is known as a 'request body' or 'payload'.
- 🔄 The backend processes the request, validates the data, and saves it before responding with a status code (usually 201 for created resources).
- 🚀 To make POST requests, you need an HTTP client tool such as Postman, Hopscotch, or the VS Code extension Thunder Client.
- ⚙️ Thunder Client is an integrated REST API tool in VS Code that allows developers to make API requests directly from the IDE.
- 🔧 Express, by default, doesn't parse JSON in the request body, so you need to use middleware like `express.json()` to handle JSON data.
- 👨💻 Once the request body is properly parsed, you can perform operations like adding new users by pushing the data to an array.
- 🔄 To simulate auto-incrementing IDs in the absence of a database, the example uses the last array element's ID and increments it by one.
- 📡 After processing a POST request, the server should return a status code 201 to indicate successful resource creation.
Q & A
What is a POST request in the context of an API?
-A POST request is used to send data to the server to create a new resource, such as submitting a form to register a user, and it includes a request body or payload.
What is the purpose of a request body in a POST request?
-The request body, also known as payload, contains the data being sent from the client to the server. In a POST request, the request body includes information like user details (username, password, etc.) that the server will process.
Why is it important to use middleware when handling POST requests in Express?
-Middleware is important for parsing incoming request bodies in Express. For example, the `express.json()` middleware is needed to parse JSON data sent in the POST request, as Express does not handle this automatically.
What is the significance of the 201 status code in a POST request?
-A 201 status code indicates that a new resource has been successfully created. It is typically returned after a successful POST request.
What tool is introduced to test API requests within VS Code?
-The tool introduced for testing API requests within VS Code is Thunder Client. It is a lightweight REST API client that allows you to make HTTP requests directly from VS Code.
Why does the console log ‘undefined’ when sending data in a POST request initially?
-The console logs ‘undefined’ because Express is not automatically parsing the JSON data from the request body. This issue is resolved by using the `express.json()` middleware.
How can the same URL path be used for both GET and POST requests in Express?
-The same URL path can be used for both GET and POST requests because the HTTP method (GET or POST) determines how the server will handle the request, even if the path is identical.
What happens if you send a POST request without a database to store data?
-Without a database, you can simulate storing data by using an array in memory. In the example, new users are added to a mock array, and the array is updated with each POST request.
How does the example in the script generate a unique ID for each new user without a database?
-The example generates a unique ID by taking the ID of the last user in the array, incrementing it by 1, and assigning it to the new user.
What is the role of the spread operator in the new user creation process?
-The spread operator is used to unpack all the fields from the request body and include them in the new user object being created, making it easier to handle multiple fields.
Outlines
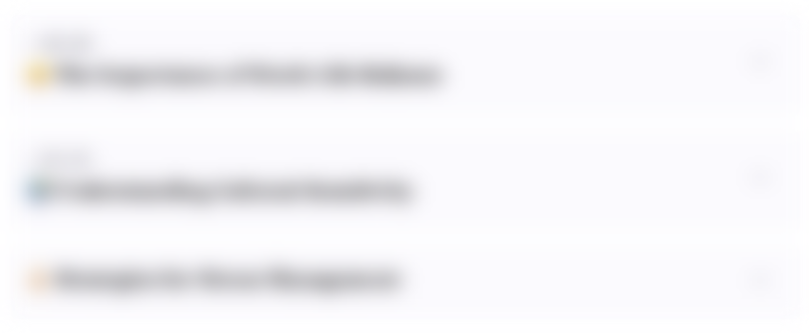
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
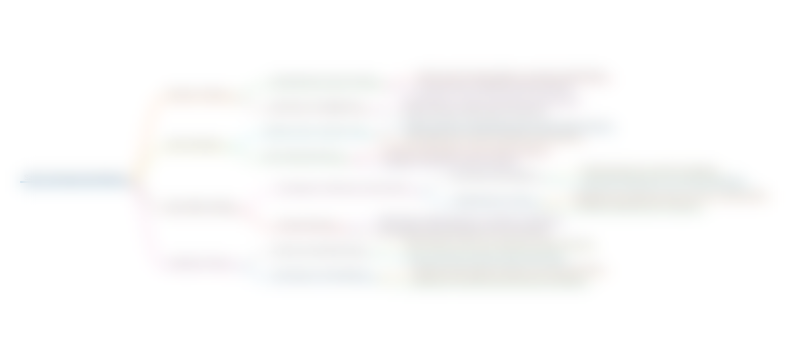
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
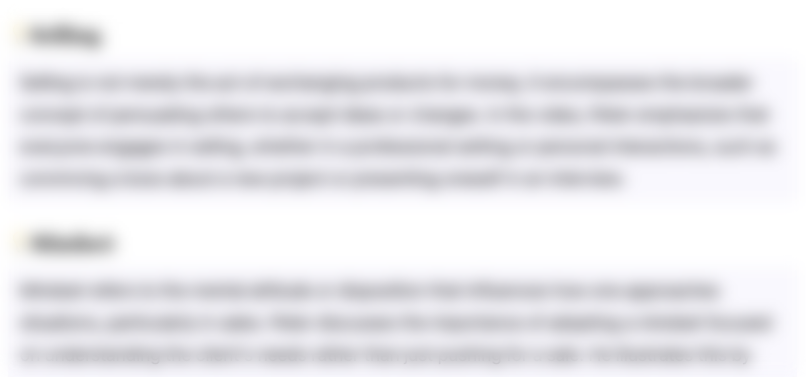
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
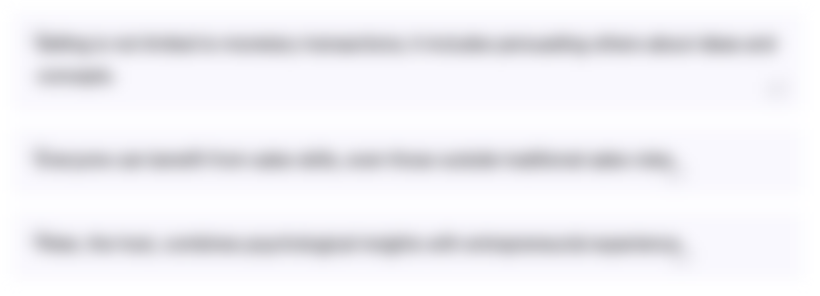
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
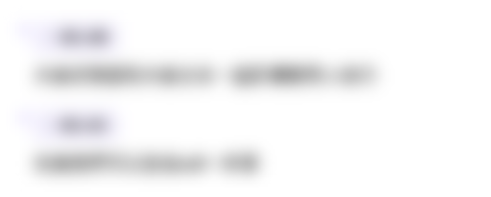
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示
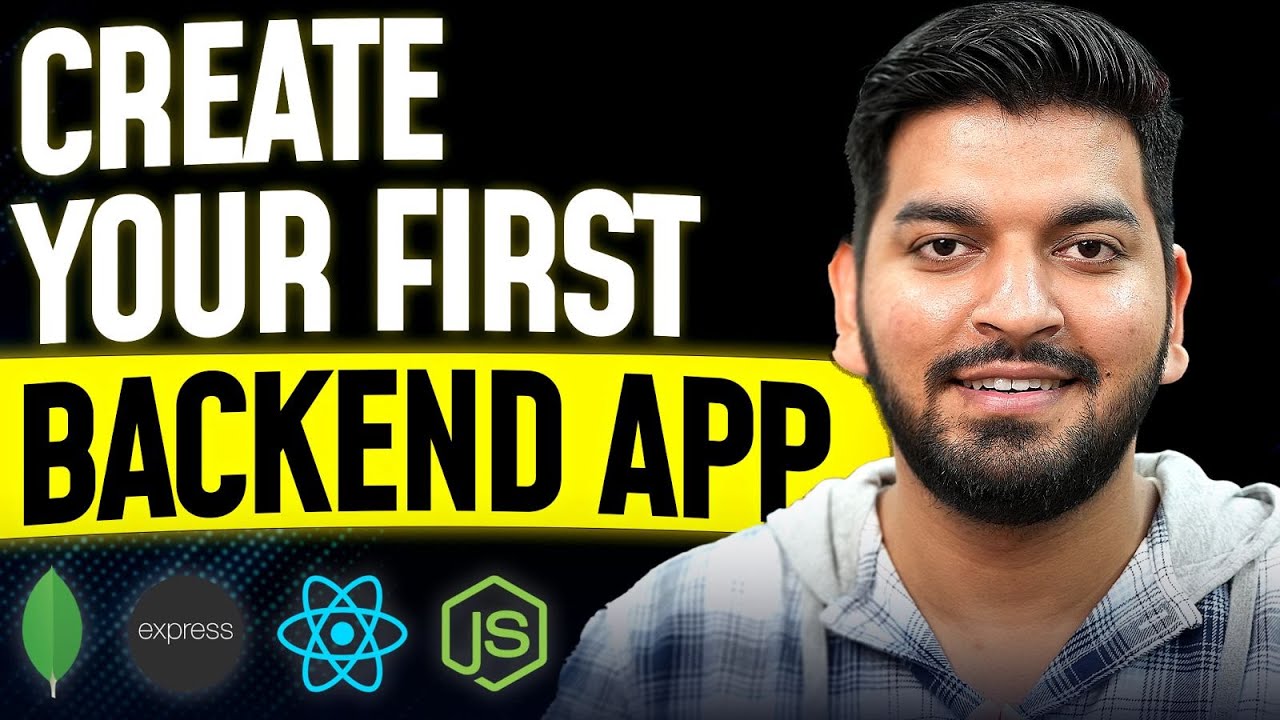
Code your FIRST BACKEND App 2024

MERN Stack Tutorial #2 - Express App Setup

Configure NGINX as a Reverse Proxy
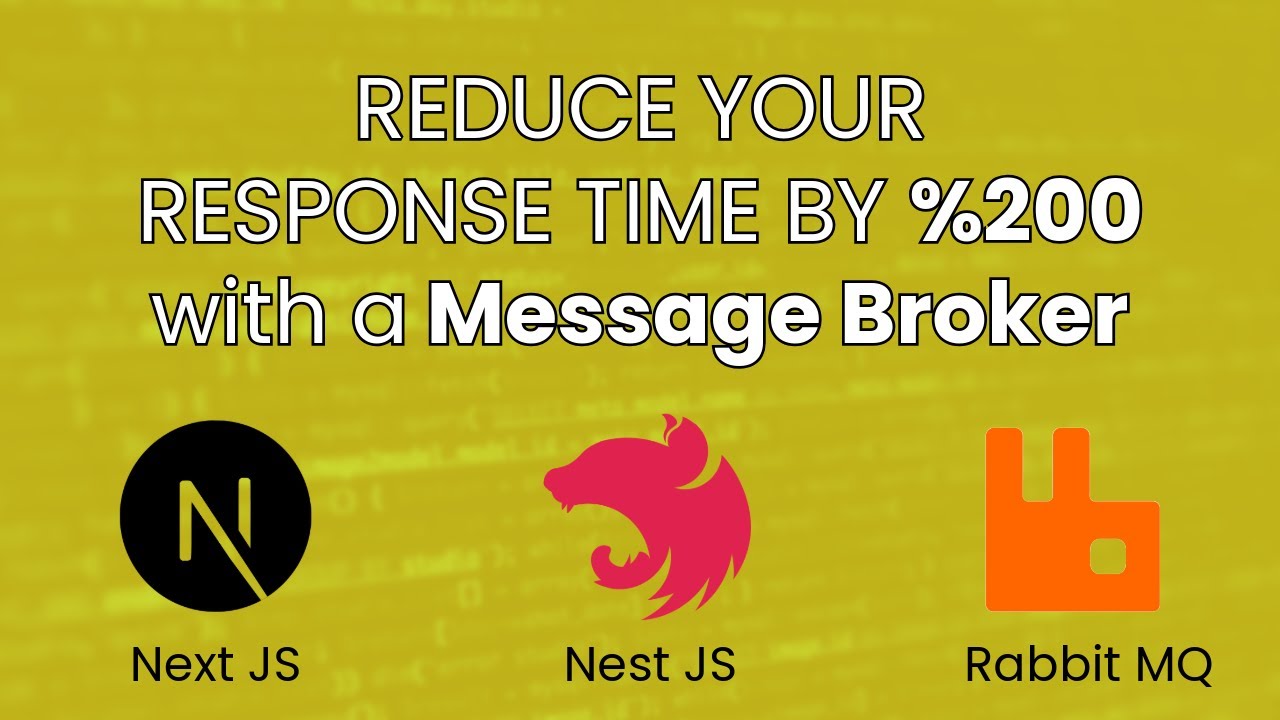
Learn how you can take advantage of a Message Broker - Fullstack Web Development - Next JS - Nest JS

#09 An overview of how web works | Fundamentals of NODE JS | A Complete NODE JS Course
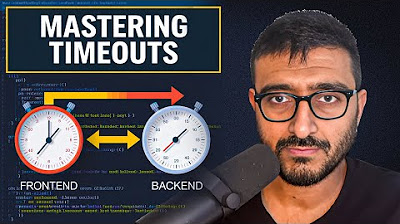
Frontend and Backends Timeouts
5.0 / 5 (0 votes)