Java Tutorial: Associativity of Operators in Java
Summary
TLDRThis video covers essential topics related to operator precedence and associativity in Java, explaining how Java evaluates expressions. The speaker clarifies that, unlike mathematical BODMAS rules, Java relies on precedence (which operator is applied first) and associativity (the direction of execution). Through examples, they demonstrate how operators like multiplication, division, addition, and subtraction are evaluated in different contexts, helping viewers understand complex expressions. The video is highly relevant for students, interviewees, and anyone preparing for exams, offering a deep dive into an often misunderstood topic.
Takeaways
- 💻 The video focuses on explaining operator precedence and associativity in Java.
- 🔢 Java does not use the BODMAS rule, but instead follows precedence and associativity rules for operator evaluation.
- 🛠️ Operators like multiplication (*) and division (/) have higher precedence than addition (+) and subtraction (-).
- ⚖️ Associativity determines the order in which operators with the same precedence are evaluated (left to right or right to left).
- 📊 In Java, multiplication and division operators are left-associative, meaning they are evaluated from left to right.
- 🧮 Example: The expression '60 / 5 - 34 * 2' evaluates division first (60/5=12), then multiplication, and finally subtraction, resulting in -56.
- 📃 The instructor emphasizes using parentheses to avoid errors in complex expressions where multiple operators are involved.
- 🎓 The video includes a quadratic expression example, showing how operator precedence affects evaluation and how to correct it using parentheses.
- 📈 Operator precedence is critical in programming, especially during interviews or exams, as it affects how expressions are executed.
- ✅ The video encourages viewers to understand these concepts early on to avoid common mistakes in programming and interviews.
Q & A
What is operator precedence in Java?
-Operator precedence in Java determines the order in which operators are evaluated in expressions. Operators with higher precedence are evaluated before those with lower precedence.
How does associativity affect the evaluation of expressions?
-Associativity defines the direction in which operators with the same precedence are evaluated. Most operators in Java are left-to-right associative, meaning they are evaluated from left to right.
What is the difference between precedence and associativity?
-Precedence determines which operator is evaluated first based on priority, while associativity resolves the order of evaluation for operators with the same precedence, dictating whether they are evaluated from left to right or right to left.
Why is the rule of BODMAS not used in Java?
-Java uses precedence and associativity rules instead of the BODMAS rule for evaluating mathematical expressions. The precedence of operators in Java is defined by the language, not mathematical conventions like BODMAS.
How does Java evaluate the expression `6 * 5 - 34 / 2`?
-In Java, `6 * 5` is evaluated first because multiplication has a higher precedence than subtraction. Then, `34 / 2` is evaluated because division also has higher precedence than subtraction. Finally, `30 - 17` is calculated, resulting in 13.
What happens when operators have the same precedence?
-When operators have the same precedence, associativity determines the order of evaluation. For example, both division and multiplication have the same precedence, so Java evaluates them left to right.
How can parentheses change the order of evaluation?
-Parentheses override the default precedence and associativity rules, forcing specific parts of an expression to be evaluated first. For example, `(6 - 5) * 2` will evaluate the subtraction before the multiplication, even though multiplication has higher precedence.
What happens if the denominator of an expression becomes zero?
-If the denominator of an expression becomes zero, Java will throw an `ArithmeticException`, indicating a division by zero error. Division by zero is undefined in mathematics and causes an error in programming.
What is the significance of knowing precedence and associativity for interviews or exams?
-Understanding operator precedence and associativity is crucial for writing and debugging Java code. It also helps in solving coding questions in interviews and exams where correct expression evaluation is necessary.
Why is it important to use parentheses in complex expressions?
-Parentheses help clarify the intended order of evaluation in complex expressions, preventing unexpected results due to precedence and associativity rules. This makes code more readable and less prone to errors.
Outlines
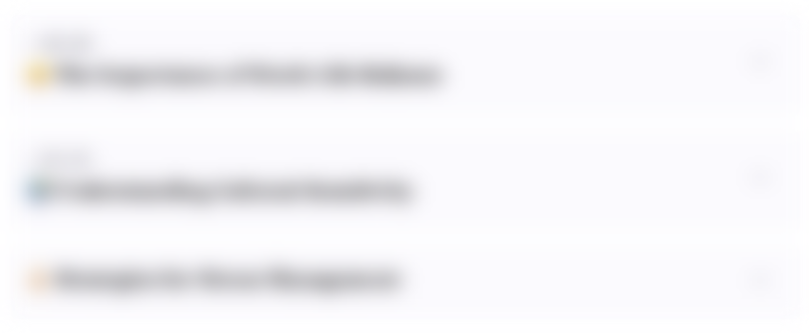
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
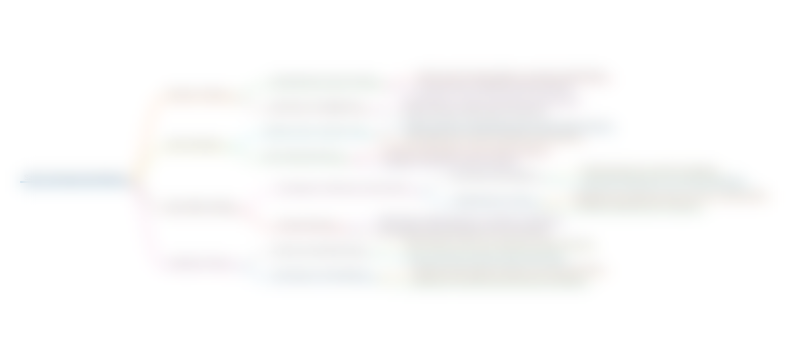
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
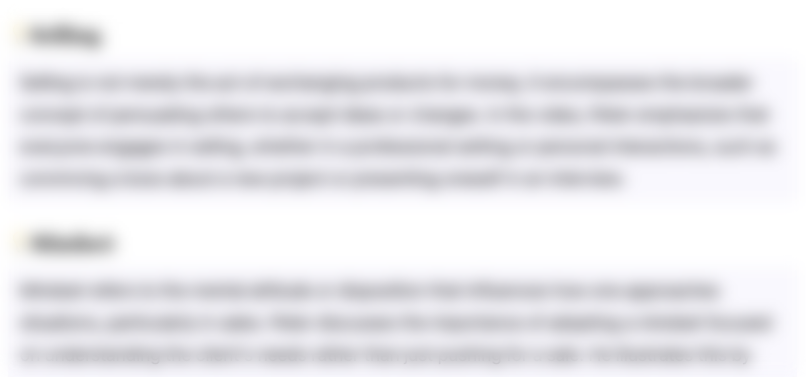
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
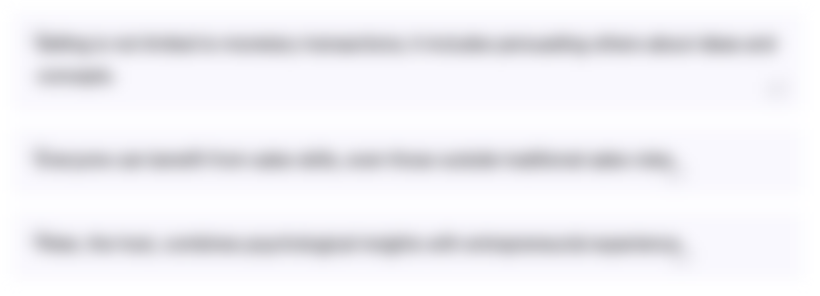
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
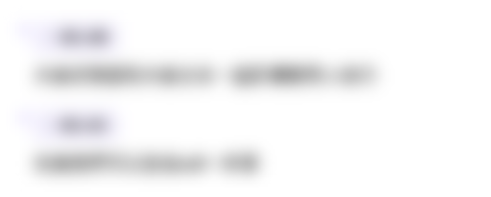
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示
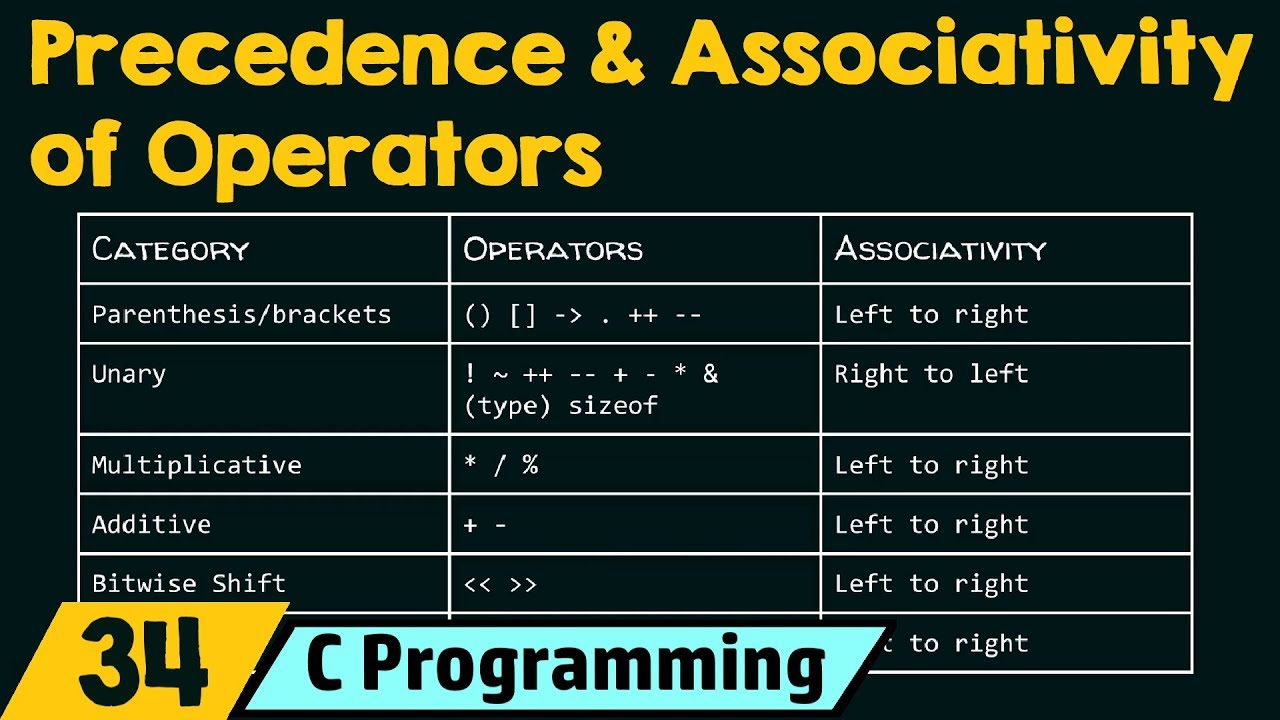
Precedence and Associativity of Operators
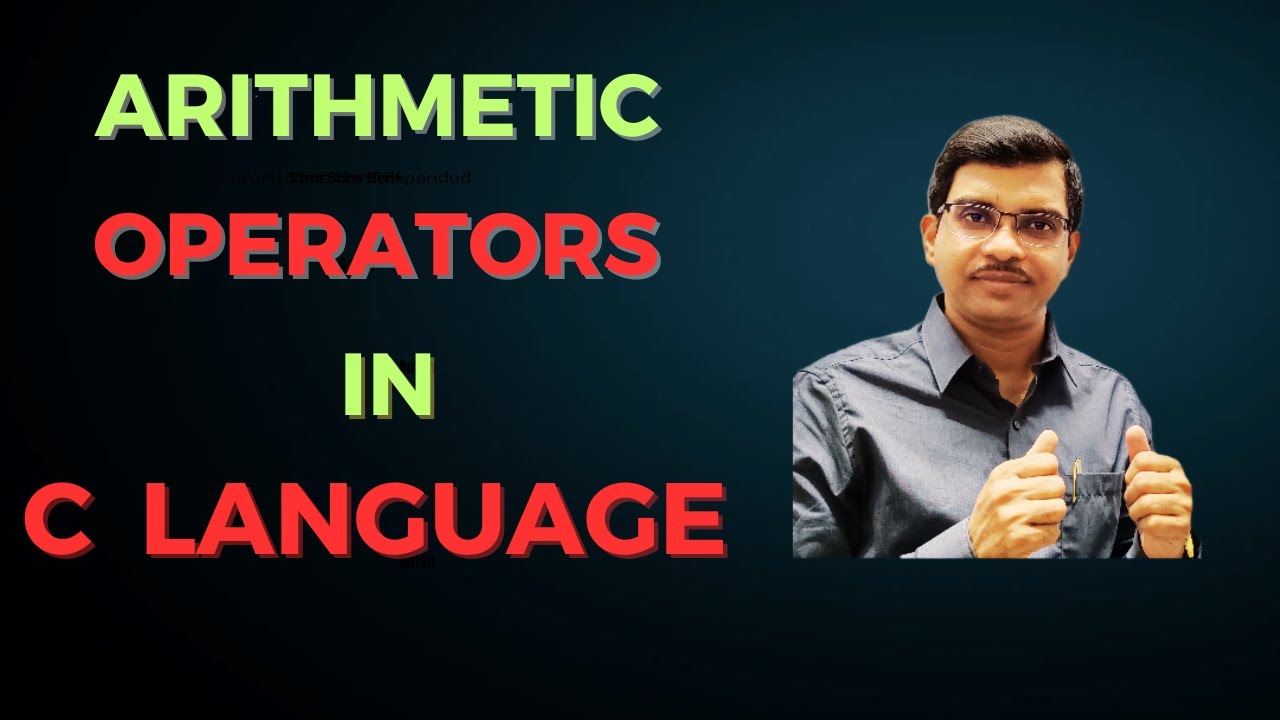
C_12 Arithmetic Operators in C Language | C Programming Tutorials
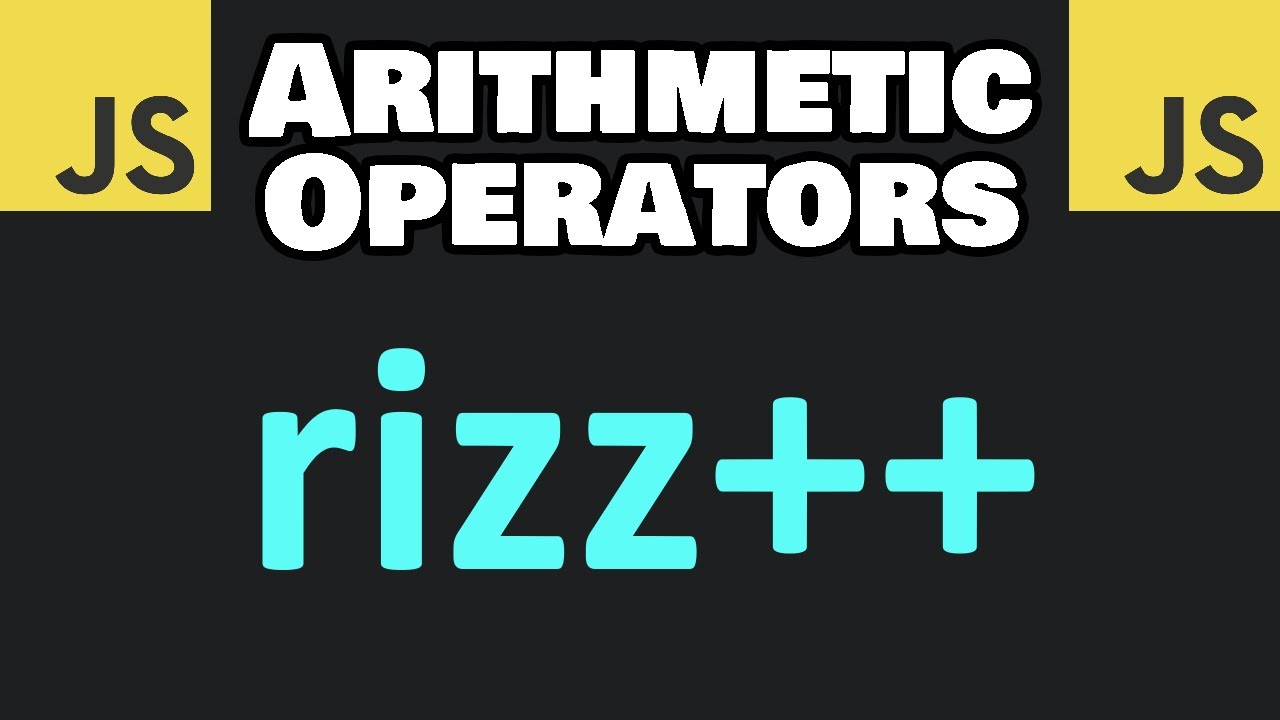
JavaScript ARITHMETIC OPERATORS in 8 minutes! ➕
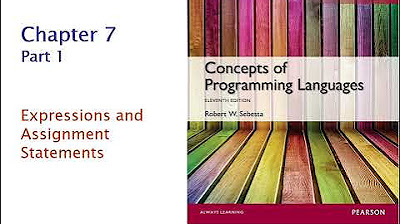
COS 333: Chapter 7, Part 1
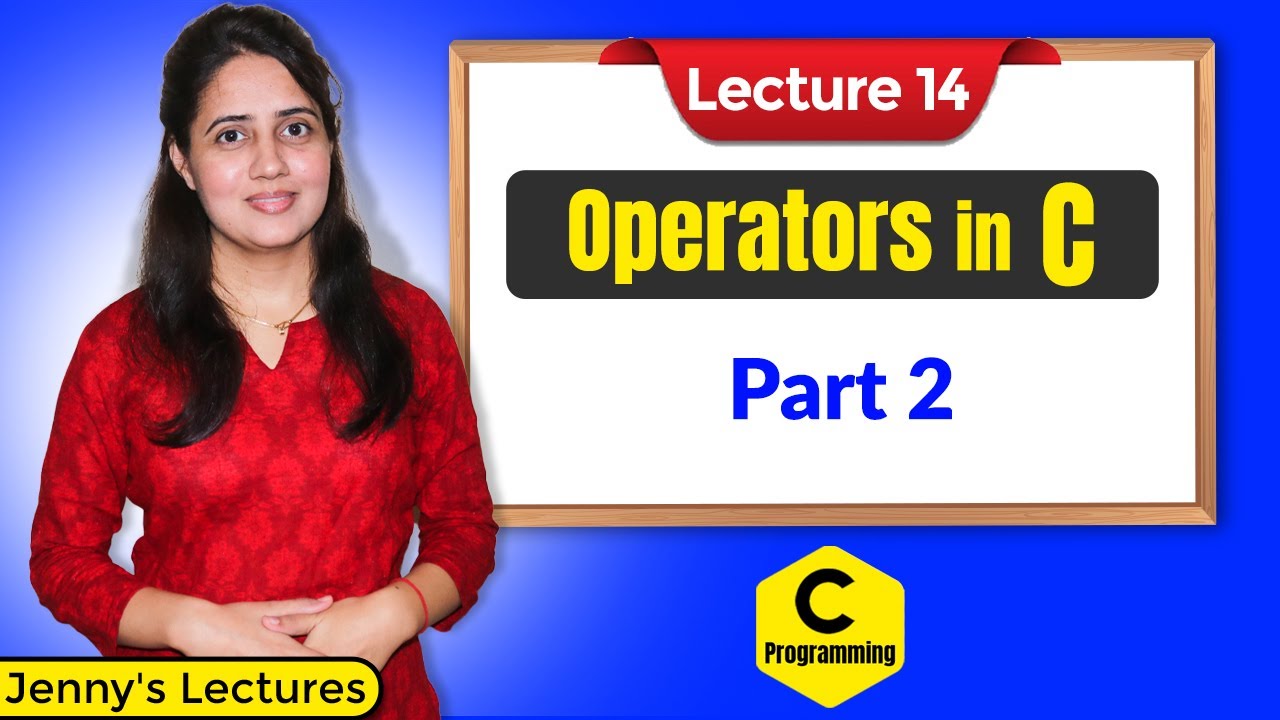
C_14 Operators in C - Part 2 | Arithmetic & Assignment Operators | C Programming Tutorials
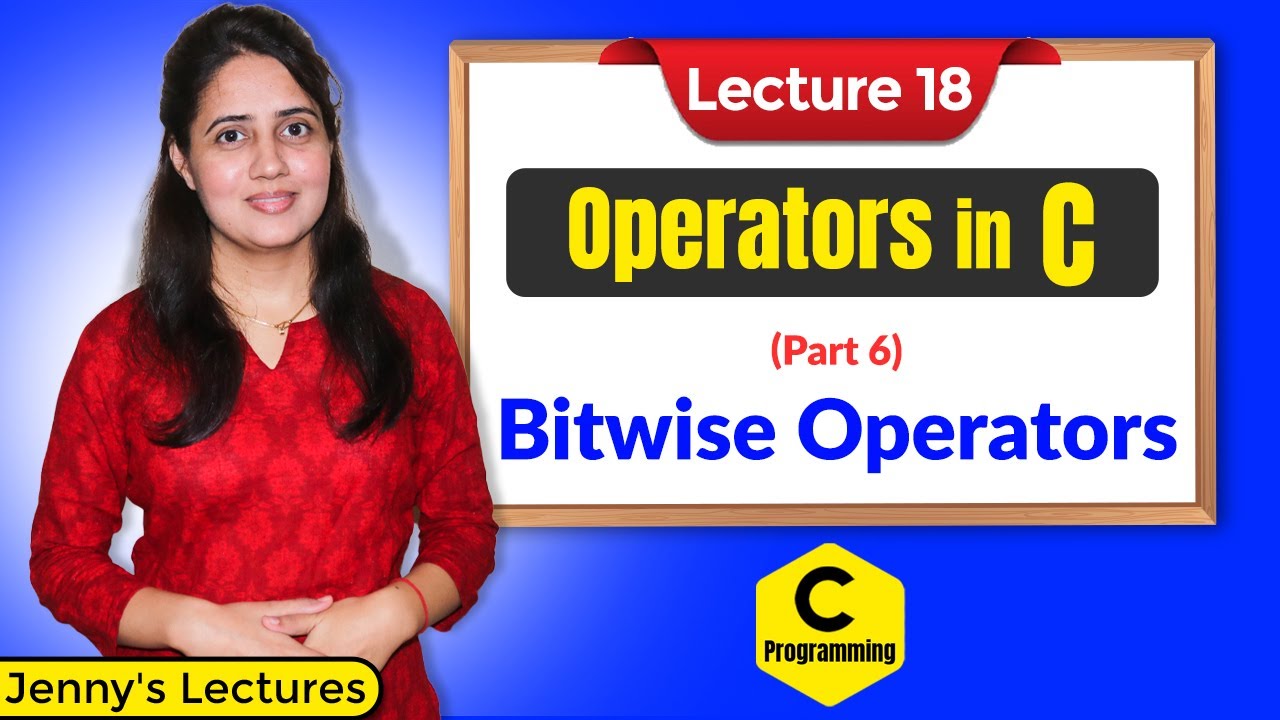
C_18 Operators in C - Part 6 | Bitwise Operators | C Programming Tutorials
5.0 / 5 (0 votes)