Creating Array and Fetching Elements in JavaScript
Summary
TLDRIn this JavaScript tutorial, David Reddy introduces the concept of arrays, explaining their necessity when dealing with multiple values without keys. He demonstrates two methods of creating arrays: using the 'new Array' constructor and direct assignment within square brackets. Reddy then shows how to assign values to an array, check its length, and use the 'push' method to add elements dynamically. Additionally, he clarifies the zero-based indexing system in arrays and the potential issue of accessing an undefined element beyond the array's length, suggesting a precautionary check before fetching values.
Takeaways
- 📚 The video is part of a series on JavaScript, continuing from previous discussions on objects, primitive types, and the concept that non-primitive data types are objects.
- 🗃 Arrays are introduced as a data structure for when you need to store multiple values without assigning keys to each value, unlike objects.
- 🔑 Arrays can be created using the `new Array()` constructor function or by using square brackets `[]` to assign values directly.
- 📦 Two methods for creating an array are shown: one that initializes an empty array and another that pre-populates the array with values.
- 🔍 The `length` property of an array is used to determine the number of elements it contains, which is demonstrated in the script.
- 📈 The `push` method is explained as a way to add elements to an array after its creation, with examples of how it works.
- 🔑 Accessing array elements is done using index numbers, starting with zero as the index for the first element.
- 🚫 Attempting to access an element at an index that does not exist in the array results in `undefined`, which is a potential source of errors.
- 🔄 The script suggests checking the array's `length` before trying to access an element to avoid errors related to out-of-bounds indices.
- 🔍 The video promises to cover how to add elements to an array using index values in a future video.
- 👋 The presenter invites viewers to comment, subscribe, and engage with the content, indicating an interactive and educational approach.
Q & A
What is the main topic of the video?
-The main topic of the video is about arrays in JavaScript.
What are the two ways mentioned in the video to create an array in JavaScript?
-The two ways to create an array in JavaScript mentioned in the video are using the 'new Array' constructor function and directly assigning values within square brackets.
What is the purpose of arrays in JavaScript when objects can also store collections of data?
-Arrays are used for storing collections of values without the need for keys, unlike objects, which require key-value pairs.
How does the video demonstrate creating an empty array in JavaScript?
-The video demonstrates creating an empty array by using 'new Array' and by using an empty square bracket notation, both of which result in an empty array.
What is the property used to check the length of an array in JavaScript?
-The 'length' property is used to check the number of elements in an array in JavaScript.
How can you add values to an array that was initially empty?
-You can add values to an initially empty array using the 'push' method in JavaScript.
What happens if you try to access an index that is beyond the length of the array?
-If you try to access an index beyond the length of the array, JavaScript will return 'undefined'.
How does the video explain the concept of array indexing?
-The video explains array indexing by stating that it starts with zero, and each subsequent element increases the index by one.
What is the method used in the video to add a single value to an array?
-The 'push' method is used in the video to add a single value to an array.
Why does the video suggest checking the length of an array before accessing its elements by index?
-The video suggests checking the length of an array before accessing its elements by index to avoid accessing undefined values or going out of bounds.
How does the video address the issue with 'undefined' when accessing an out-of-bounds index in an array?
-The video mentions that it's not ideal to get 'undefined' and suggests that it would be better to get an error or a message indicating the index is out of bounds, similar to Java's 'ArrayIndexOutOfBoundsException'.
Outlines
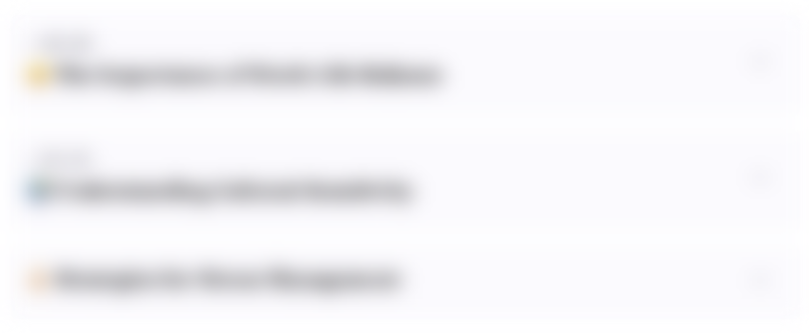
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
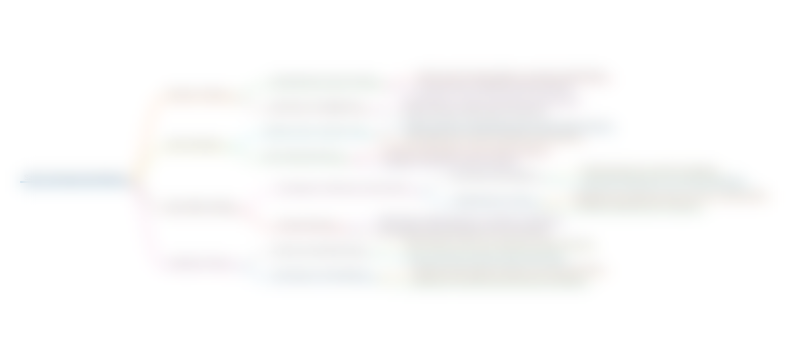
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
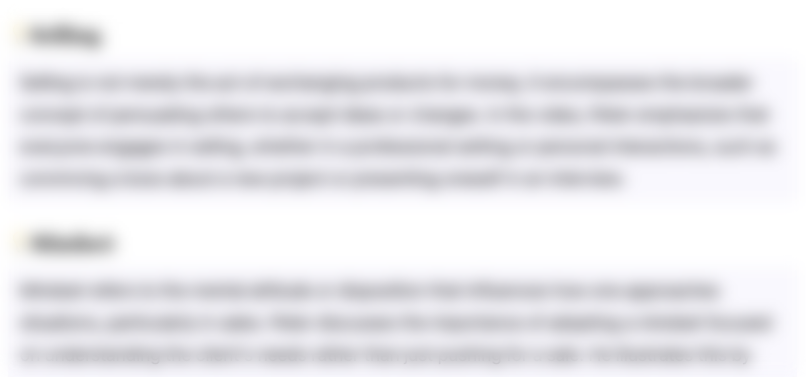
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
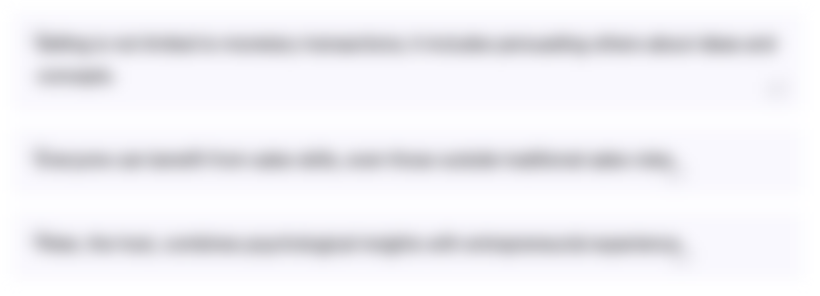
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
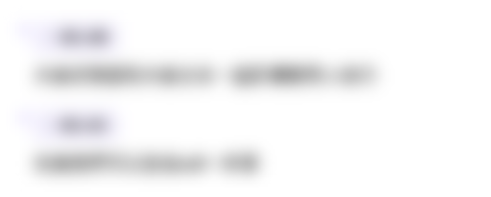
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantVoir Plus de Vidéos Connexes
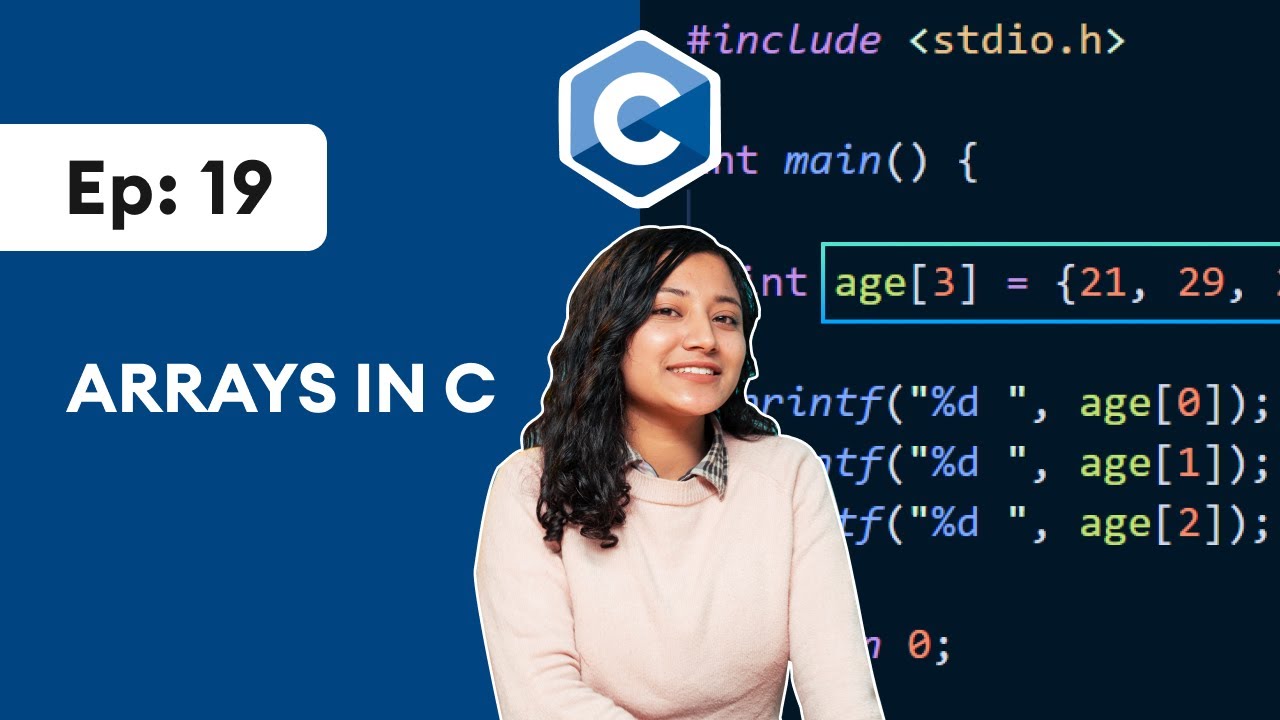
#19 C Arrays | C Programming For Beginners
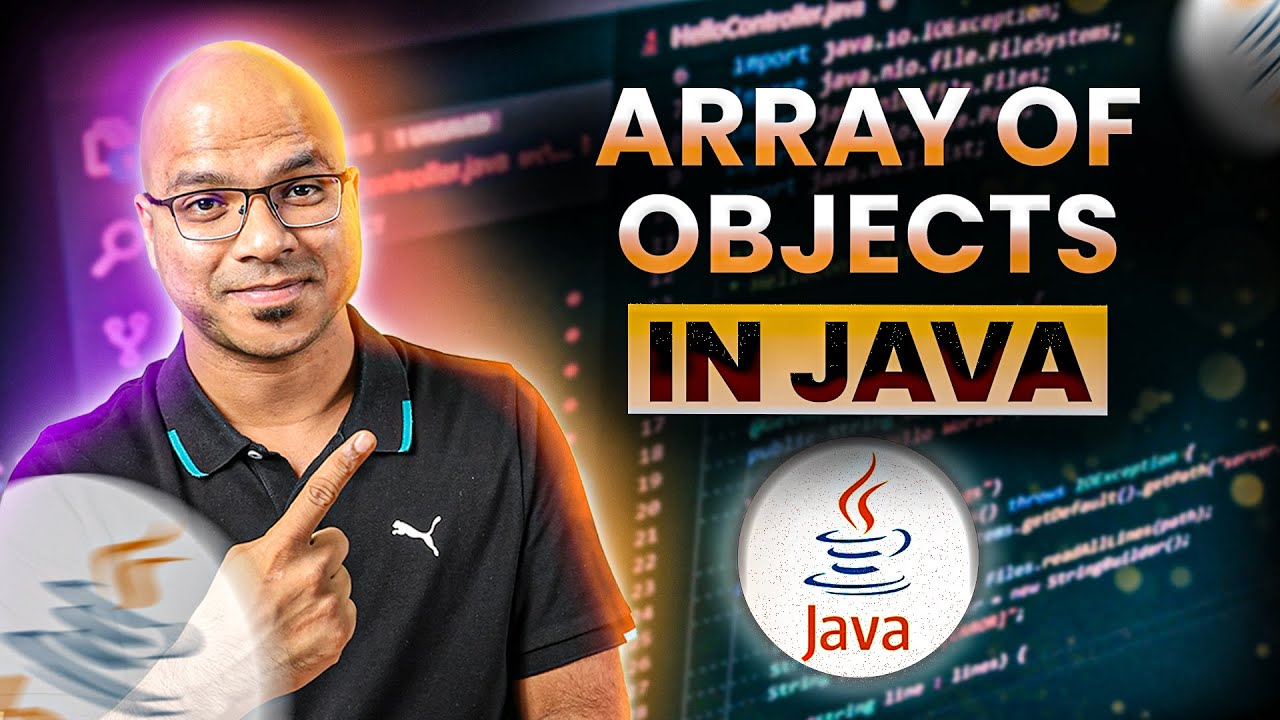
#32 Array of Objects in Java
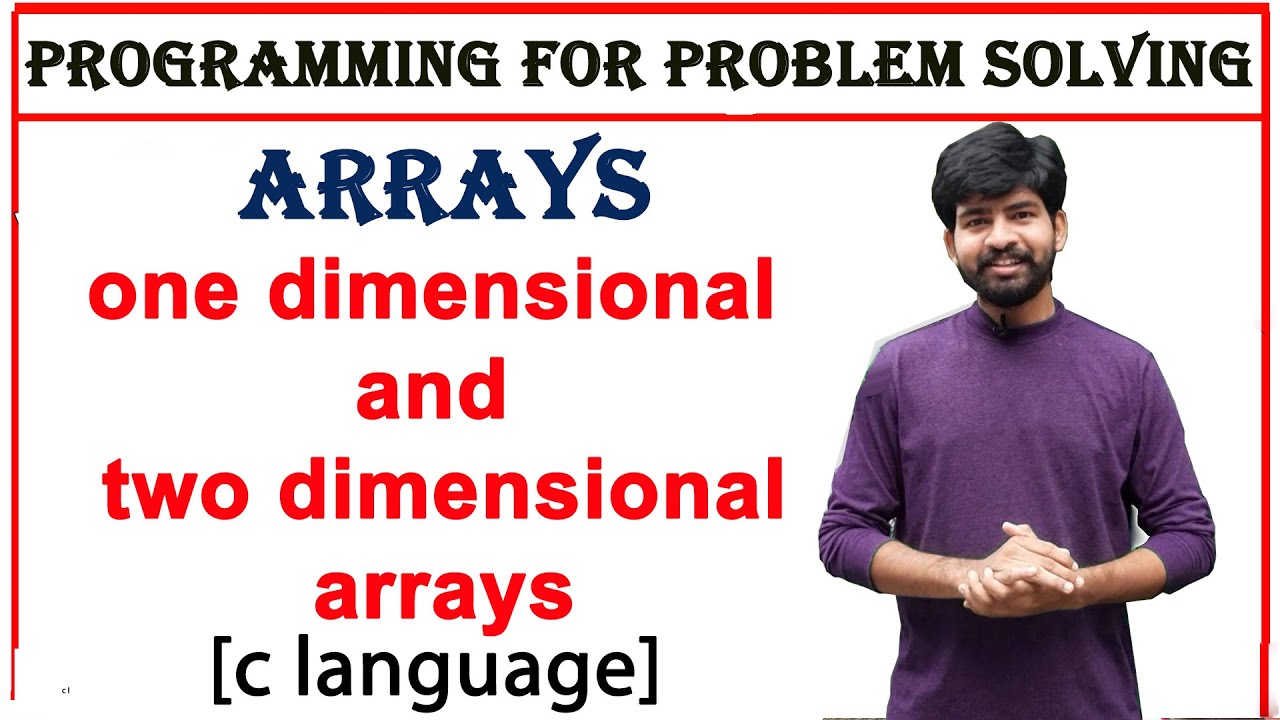
arrays in c, one dimensional array, two dimensional array |accessing and manipulating array elements

Looping Arrays, Breaking and Continuing | JavaScript 🔥 | Lecture 044
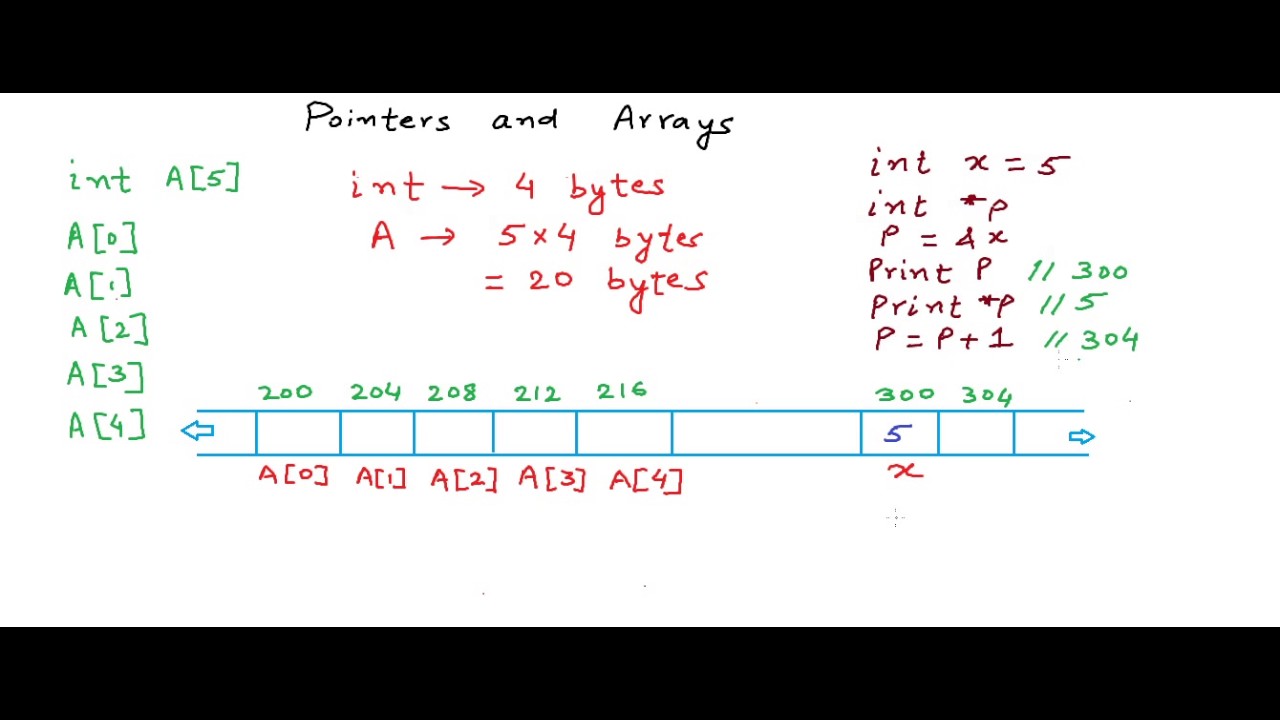
Pointers and arrays
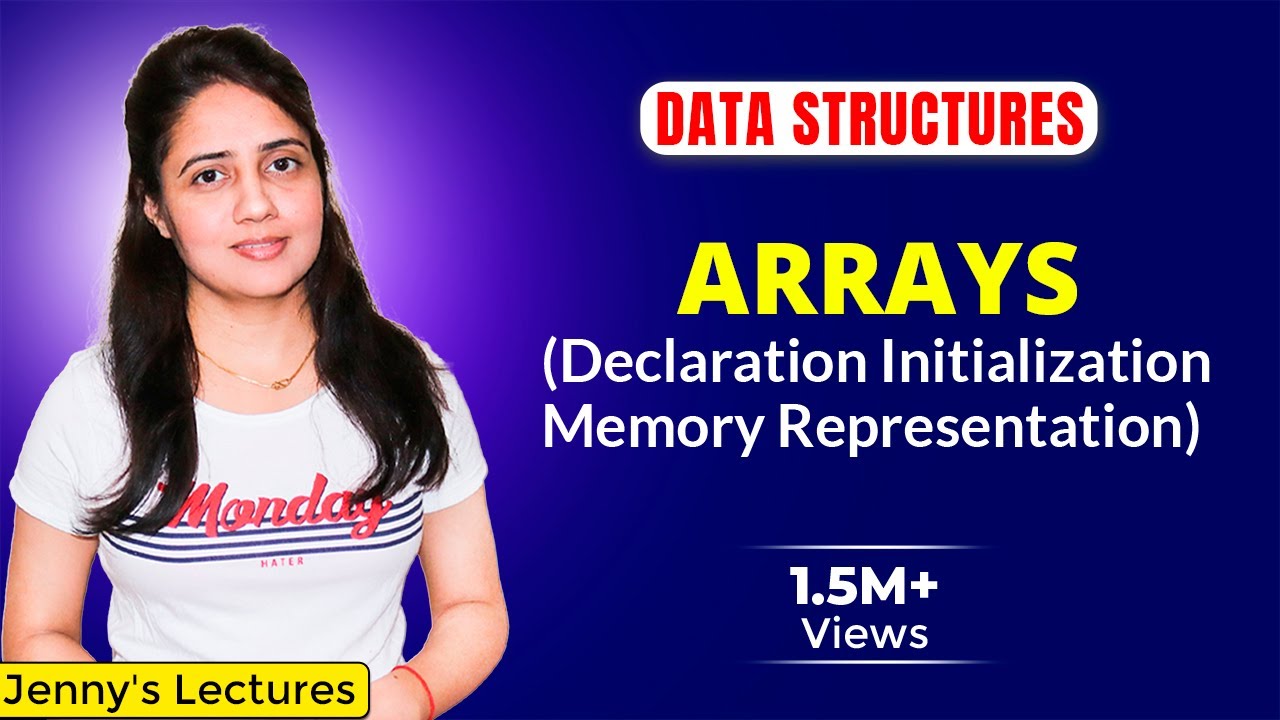
1.1 Arrays in Data Structure | Declaration, Initialization, Memory representation
5.0 / 5 (0 votes)