How to Filter Array of Objects in Javascript - ReactJS Edition
Summary
TLDRIn this video, the speaker explains how to efficiently filter arrays in JavaScript using the `filter` function, a method that streamlines the process compared to traditional looping techniques. The speaker demonstrates how to filter an array of people by department, showcasing two approaches: one with stateful items for reusable filtered data, and another without, where the filtered results are directly used. The video emphasizes the benefits of using `filter` to save time, reduce code complexity, and improve code readability, especially when dealing with large datasets or multiple filtering conditions.
Takeaways
- ๐ The filter function in JavaScript simplifies filtering arrays based on conditions.
- ๐ Previously, filtering required using loops (forEach or for) to check conditions manually.
- ๐ The filter function can be used to directly filter arrays based on a given condition (e.g., department).
- ๐ You can use the filter function to create a new array that contains only the items that meet the condition.
- ๐ Instead of modifying the original array, the filter function returns a new filtered array.
- ๐ It's a cleaner and more efficient approach compared to using forEach or for loops for filtering.
- ๐ In the example, an array of people is filtered by the 'Department' field to find people who work in the 'Dev' department.
- ๐ The filter function is often used in combination with useState in React to store filtered results in stateful items.
- ๐ You can filter the array directly and use it without creating additional state if you don't need to store the filtered results.
- ๐ The filter function is versatile and can be applied to any array, making it easier to manage complex filtering conditions.
- ๐ Using filter reduces the amount of code and complexity in your project, making your codebase more efficient and maintainable.
Q & A
What is the main topic of the video?
-The main topic of the video is filtering arrays in JavaScript using the `filter` method, and comparing it with traditional methods like `forEach` and `map`.
Why did the speaker mention that they didn't know about the `filter` function initially?
-The speaker mentions that they didn't know about the `filter` function for a long time and initially relied on using loops like `forEach` or `map` to filter arrays, which can be more complicated and error-prone.
How does the `filter` method work in JavaScript?
-The `filter` method in JavaScript creates a new array that contains all elements from the original array that satisfy the provided condition, represented by a function passed as an argument.
What was the problem with using `forEach` or `map` for filtering data?
-Using `forEach` or `map` to filter data can lead to messy code, with conditions inside loops that are harder to maintain and can introduce performance issues or bugs.
What is the benefit of using the `filter` method instead of `forEach` or `map`?
-The `filter` method is cleaner, more efficient, and directly returns a new array of elements that meet the specified condition, eliminating the need for messy loops or additional logic.
How can you use the `filter` method to get people from a specific department?
-You can use the `filter` method by passing a function that checks if the person's department matches the desired one, for example, `people.filter(p => p.department === 'Dev')`.
What does the `filter` method return when applied to an array?
-The `filter` method returns a new array that contains only the elements that satisfy the condition provided in the callback function.
Can you filter an array without storing the result in a state variable? How?
-Yes, you can filter an array without using a state variable by directly calling the `filter` method and using the result immediately, such as for displaying filtered data in a component with `map`.
What does the speaker mean by saying the `filter` method can reduce the amount of code you write?
-The speaker means that by using the `filter` method, you can avoid writing extra code like loops or additional logic for conditions, making the code more concise and easier to manage.
Why is it useful to store filtered data in a state variable, according to the speaker?
-Storing filtered data in a state variable is useful when you want to reuse or dynamically display the filtered data in different parts of your application, making it easier to manage changes and updates.
Outlines
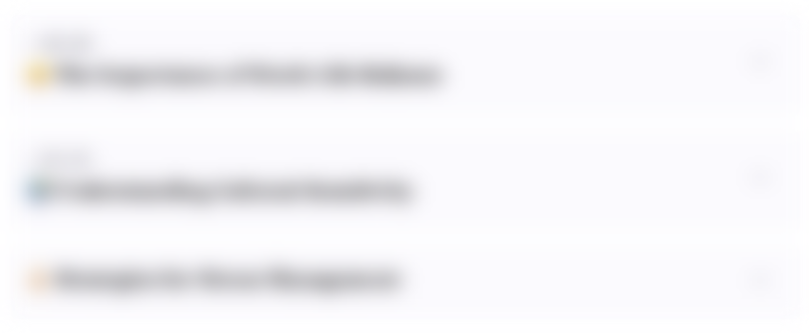
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
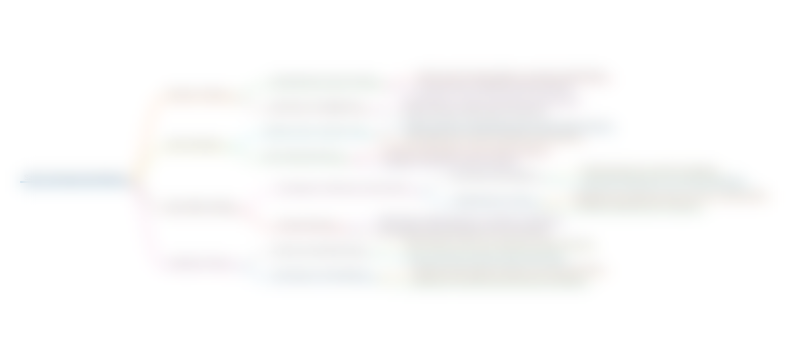
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
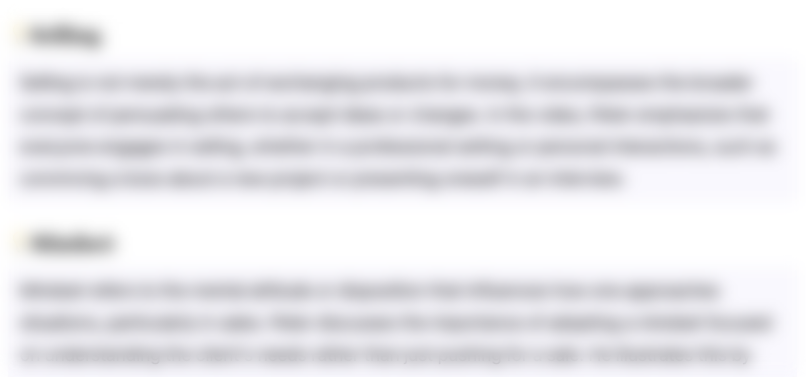
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
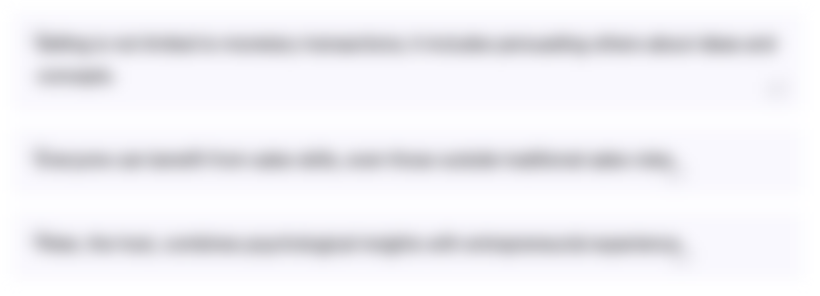
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
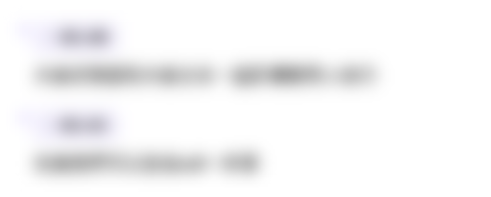
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
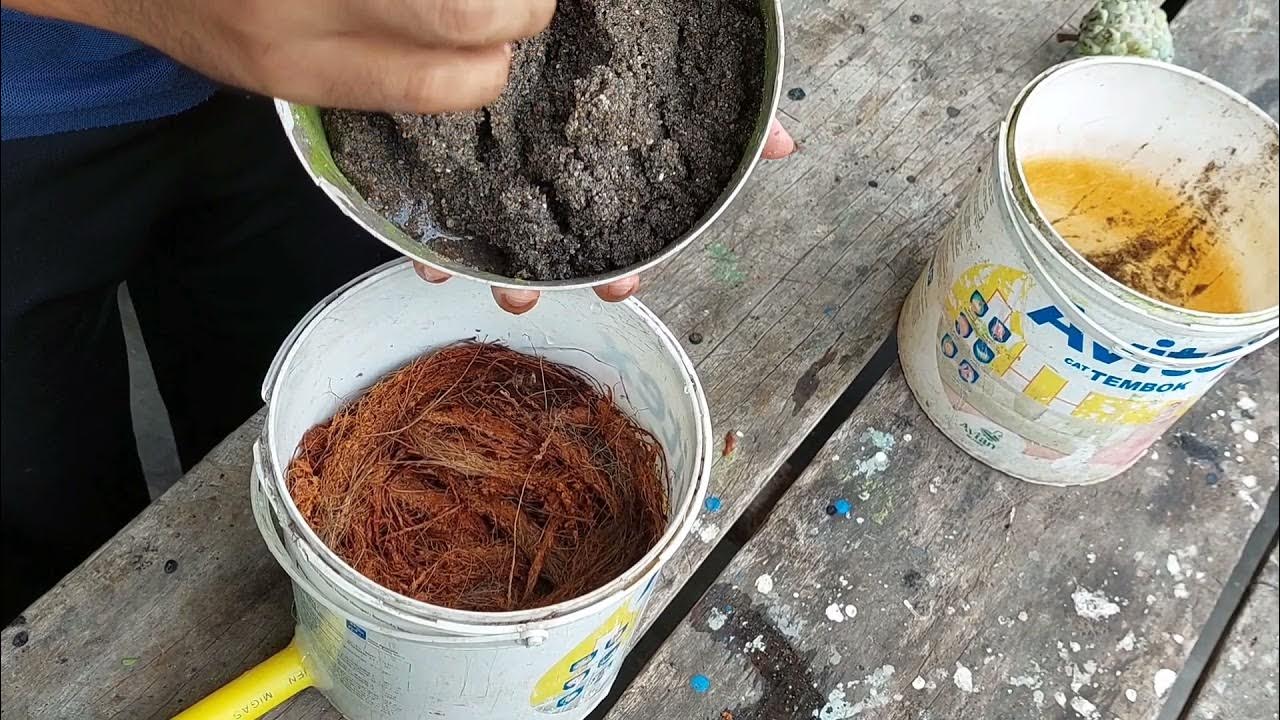
Penyaring Sederhana Air sumur mengandung Zat Besi
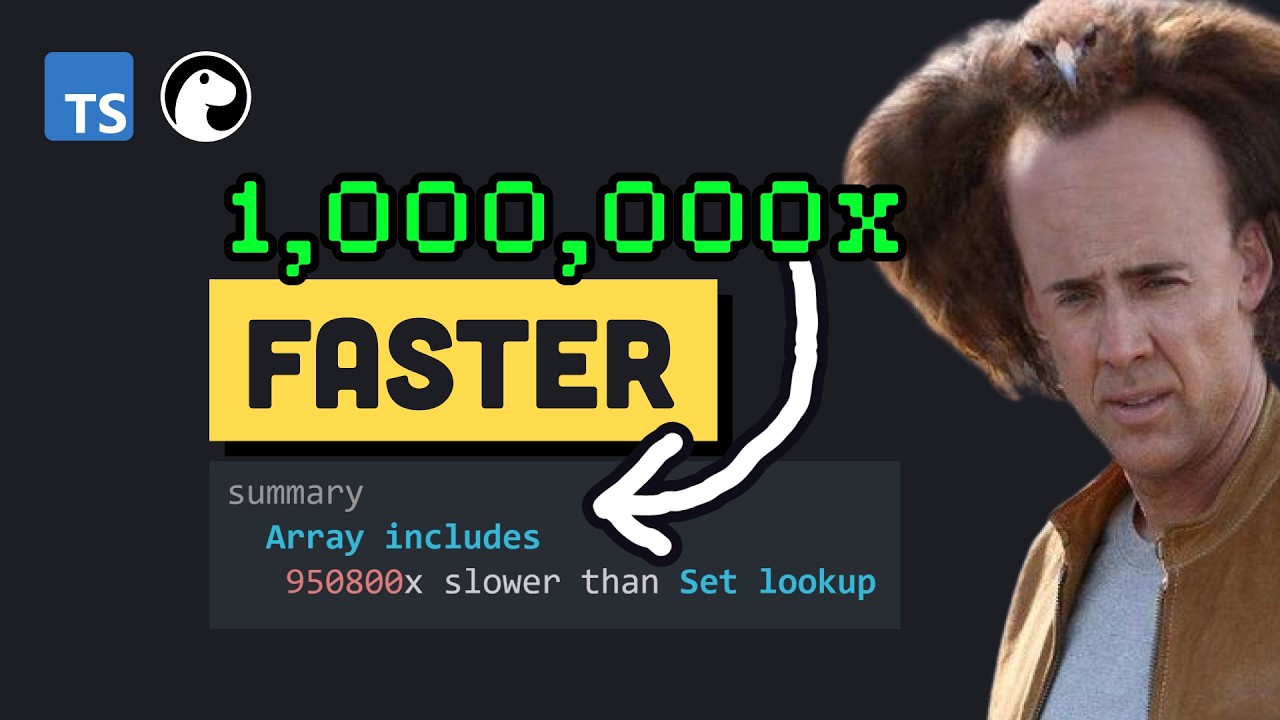
JavaScript performance is weird... Write scientifically faster code with benchmarking
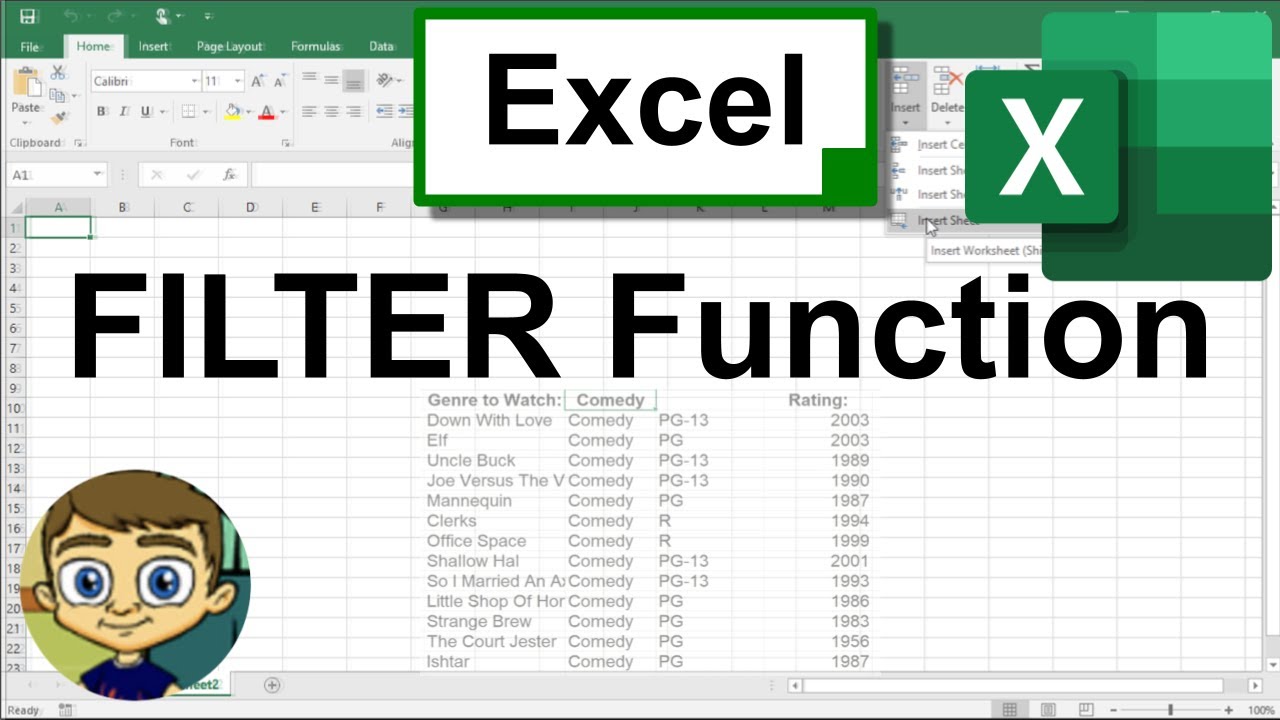
Using the Excel FILTER Function to Create Dynamic Filters
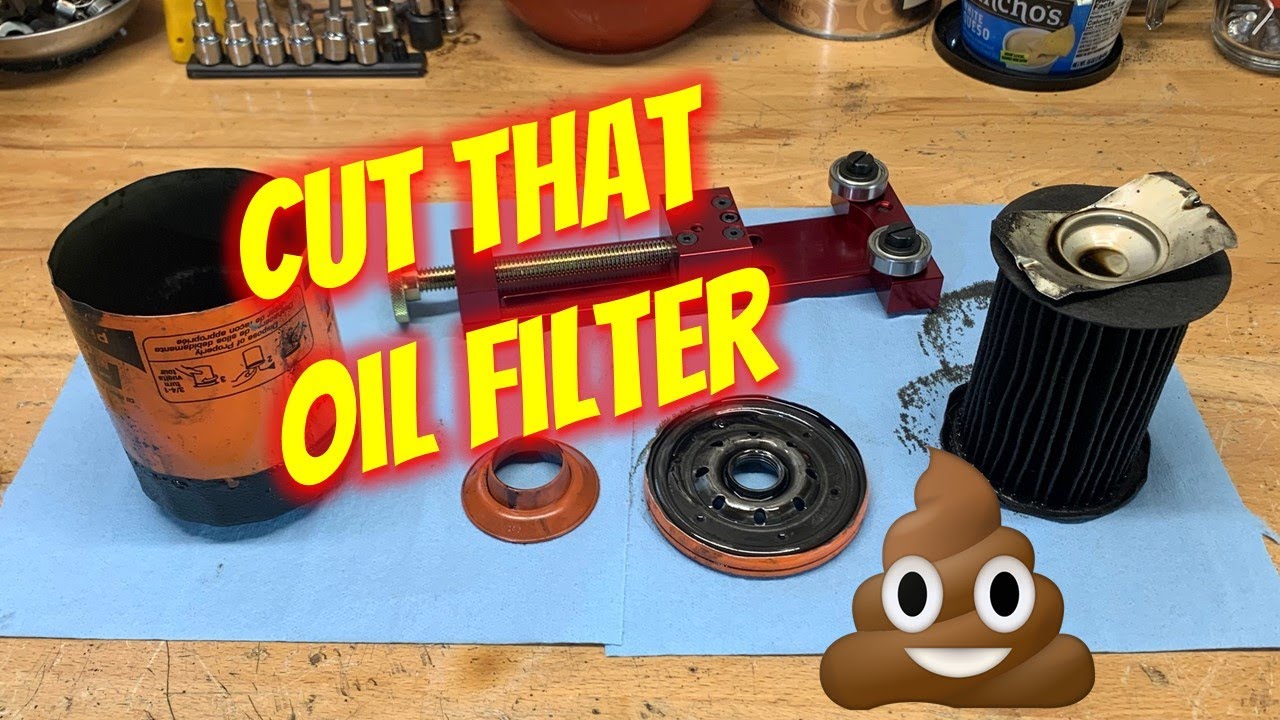
You Should Be Inspecting Your Oil Filters
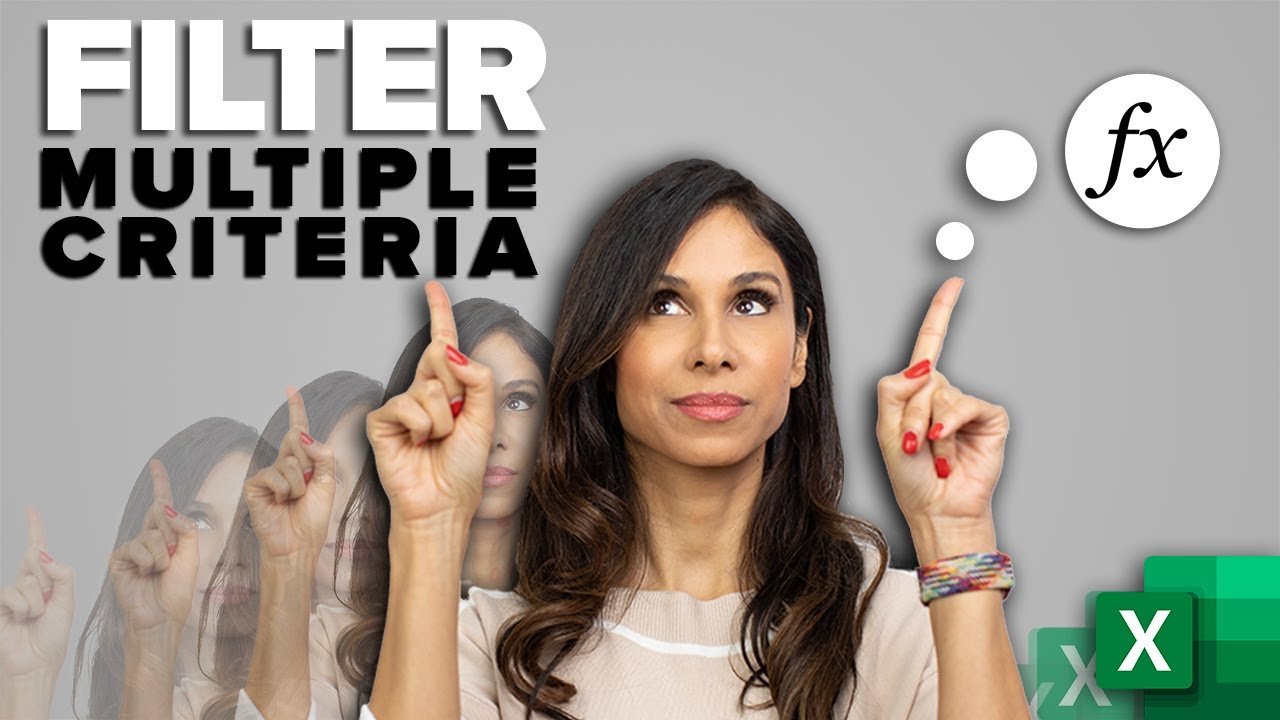
How To Use Excel FILTER Function With Multiple Criteria & Return Only the Columns You Need
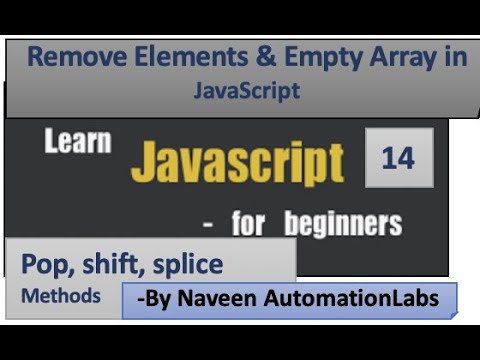
Remove Elements and Empty an Array in JavaScript - Part - 14
5.0 / 5 (0 votes)