For Loops in Python
Summary
TLDRIn this tutorial, the concept of Python's `range()` function and `for` loops is introduced. The `range()` function is shown to generate sequences of integers, and how different parameters (start, stop, and step) can customize these sequences. The tutorial then explains how to use a `for` loop to iterate over such sequences. An example demonstrates summing the numbers from 0 to 9 using a `for` loop. The video emphasizes how these concepts work together to solve problems iteratively, with a focus on understanding the flow of values through variables and loops.
Takeaways
- 😀 The `range()` function generates a sequence of integers, often used in loops for iteration.
- 😀 The syntax of `range(start, stop, step)` allows for flexibility in generating sequences of numbers.
- 😀 When using `range(6)`, it generates numbers from 0 to 5, as the stop value is exclusive.
- 😀 To include a higher number in the sequence, you must set the stop value one higher than the desired number.
- 😀 You can start a range at a number other than 0, such as `range(1, 7)`, which generates numbers from 1 to 6.
- 😀 The `range()` function can also include a step value to increment by a number other than 1, e.g., `range(0, 8, 2)` generates [0, 2, 4, 6].
- 😀 A `for` loop iterates over each element in a sequence generated by functions like `range()`.
- 😀 In a `for` loop, a variable (commonly `i`) holds the current value from the sequence during each iteration.
- 😀 The `for` loop allows repeated execution of a block of code, making it useful for tasks like summing numbers or processing lists.
- 😀 The running total of a sum can be updated within the `for` loop by modifying a variable on each iteration, as shown with `sum = sum + i`.
- 😀 Python's IDEs, like PyScripter, provide helpful tips for built-in functions like `range()`, making coding easier by showing syntax and usage details.
Q & A
What is the purpose of the `range()` function in Python?
-The `range()` function in Python is used to generate a sequence of numbers. It is commonly used for creating ranges of numbers in loops, such as `for` loops, and can specify a start, stop, and step value.
How does the `range()` function handle the stop value?
-The stop value in the `range()` function is exclusive, meaning the generated sequence will include numbers up to, but not including, the stop value. For example, `range(6)` will generate the numbers 0, 1, 2, 3, 4, 5.
What happens if you want to include the stop value in a range?
-If you want to include the stop value in a range, you must specify a value that is one greater than the intended stop value. For example, `range(7)` includes 6, but not 7.
What does the `step` parameter do in the `range()` function?
-The `step` parameter in the `range()` function determines the increment between numbers in the generated sequence. For example, `range(0, 8, 2)` generates numbers 0, 2, 4, 6, incrementing by 2 each time.
How does the `for` loop work with the `range()` function?
-In a `for` loop, the `range()` function generates a sequence of numbers, and the loop iterates over each value in that sequence. The variable defined in the loop (often `i`) takes each value from the sequence during each iteration.
What is the purpose of the `i` variable in a `for` loop?
-The variable `i` in a `for` loop is used to refer to the current value in the sequence generated by the `range()` function. It holds the value for each iteration, which can be used in the loop's body.
How can a `for` loop be used to sum a range of numbers?
-A `for` loop can sum a range of numbers by initializing a variable (e.g., `sum = 0`) before the loop, and then adding the value of `i` (from the range) to `sum` in each iteration. For example, `sum += i` inside the loop will accumulate the sum of numbers in the range.
In the example of summing numbers from 0 to 9, why does the sum start at 0?
-The sum starts at 0 because we need an initial value to begin adding to. Starting with 0 ensures that the sum correctly starts from 0 and adds each number in the range during each iteration.
What is meant by 'iterative' in the context of loops?
-In the context of loops, 'iterative' refers to repeating a set of actions multiple times. Each repetition of the loop is called an iteration, and it typically involves updating variables or performing calculations with each iteration.
What are some best practices for naming variables in a `for` loop?
-A common convention for variable names in `for` loops is to use short, descriptive names like `i`, `j`, or `k`, especially when the loop is used for iterating through sequences. However, the name should always reflect the purpose of the variable in the loop for better readability.
Outlines
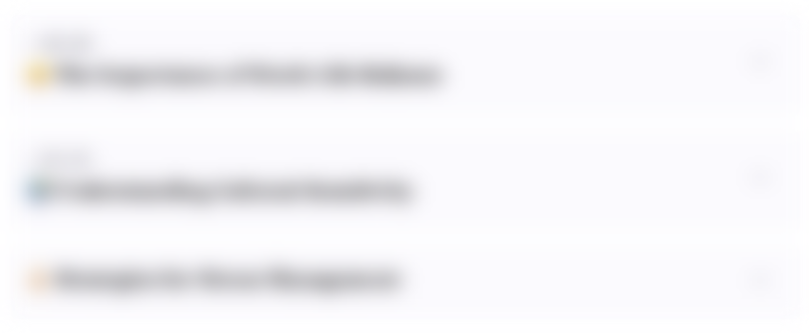
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
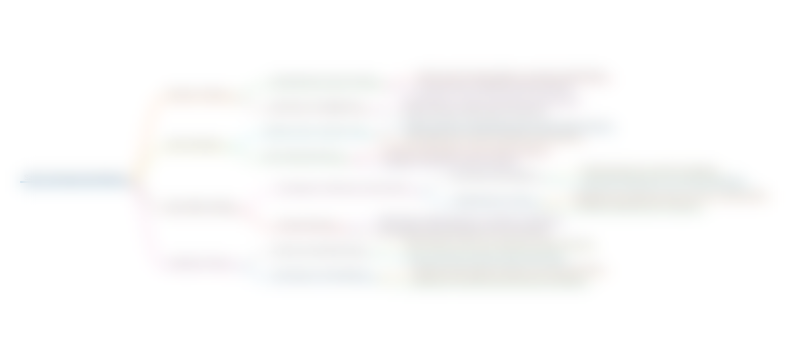
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
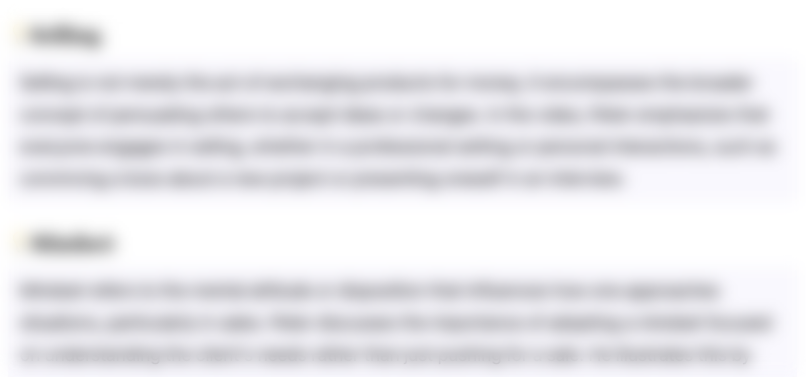
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
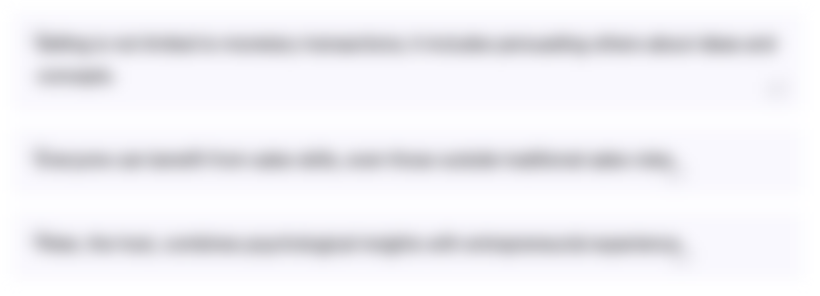
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
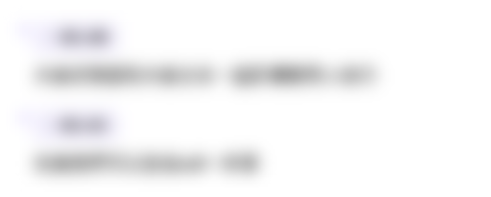
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenant5.0 / 5 (0 votes)