Python Bytecode: An Introductory Tutorial
Summary
TLDRThis educational video delves into the intricacies of Python bytecode, aiming to deepen the viewer's understanding of Python's inner workings. It starts with a simple function definition and explores how Python's dynamic typing allows for versatile function usage. The video then uncovers the attributes of a function object, highlighting the 'co_code' attribute which stores compiled bytecode. Utilizing the 'dis' module, the tutorial demonstrates how to disassemble Python code, revealing the bytecode instructions and their order. It explains opcodes like 'load_fast', 'binary_add', and 'return_value', and discusses stack operations and jump targets. The tutorial also touches on dead code and Python's implicit 'return None' behavior, providing insights into Python's code generation mechanism.
Takeaways
- ๐ Python is a dynamically typed language, allowing functions to work with any type of objects that support the '+' operation.
- ๐ Functions in Python are objects with attributes such as `__code__`, which contains the compiled bytecode.
- ๐พ The bytecode is stored as a sequence of instructions that can be inspected to understand how Python executes code.
- ๐ The `dis` module can be used to disassemble Python functions and view the underlying bytecode instructions.
- ๐ข Bytecode instructions are represented by numbers, which can be converted to human-readable form using the `ord` and `chr` functions.
- ๐ Opcodes like `LOAD_FAST`, `BINARY_ADD`, and `RETURN_VALUE` are fundamental operations that Python's virtual machine executes.
- ๐ The stack-based nature of Python's bytecode execution is crucial for understanding how values are manipulated during function calls.
- ๐ Jump targets in bytecode allow for control flow operations like conditionals and loops.
- ๐ Python caches bytecode in `__pycache__` folders to speed up subsequent executions of modules.
- ๐ Dead code, such as unreachable return statements, can still be present in the bytecode due to Python's code generation rules.
Q & A
What is the main focus of the video?
-The main focus of the video is to explore the manipulation of functions in Python, particularly by examining the bytecode instructions that Python uses to execute functions.
Why is understanding Python bytecode important?
-Understanding Python bytecode is important because it provides a deeper level of insight into how Python functions are executed, which can help in optimizing code and debugging.
What is the purpose of the 'dis' module mentioned in the video?
-The 'dis' module is used to disassemble Python code into bytecode instructions, allowing developers to see the underlying operations that Python performs when executing a function.
How does Python handle dynamically typed functions?
-Python handles dynamically typed functions by allowing any two objects that support the '+' operation to be passed to a function, regardless of the types originally intended by the function's definition.
What is the significance of the 'dunder code' attribute of a function object?
-The 'dunder code' attribute of a function object holds the code object, which contains the compiled bytecode instructions for the function.
What does the 'load fast' instruction in Python bytecode do?
-The 'load fast' instruction in Python bytecode is used to load a local variable onto the stack, with the specific variable indicated by a following byte.
How does the 'binary add' instruction work in the context of bytecode?
-The 'binary add' instruction pops the top two values from the stack, adds them together, and then pushes the result back onto the stack.
What is the role of the stack in Python bytecode execution?
-The stack in Python bytecode execution is used to temporarily store values that can be manipulated by instructions, following a last-in, first-out principle.
Why does the video mention 'dead code' in the context of bytecode?
-The video mentions 'dead code' to explain that certain instructions in the bytecode may never be reached due to the flow of the code, such as unreachable return statements within conditional blocks.
What is the purpose of the implicit 'load constant of none' and 'return value' in Python functions?
-The implicit 'load constant of none' and 'return value' in Python functions ensure that all functions return a value, which is 'None' by default if no explicit return statement is present at the top level of the function.
How does the video demonstrate the difference between Python implementations?
-The video demonstrates the difference between Python implementations by noting that the discussed bytecode and virtual machine concepts apply specifically to CPython and may not apply to other implementations like PyPy or Jython.
Outlines
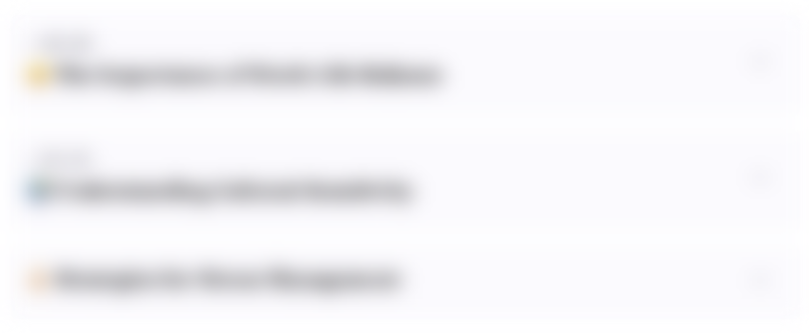
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
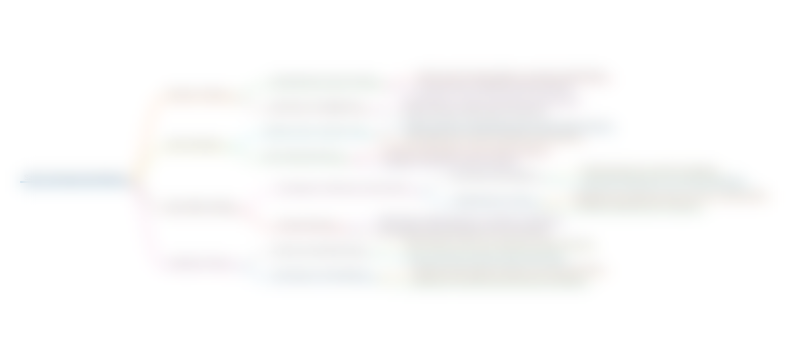
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
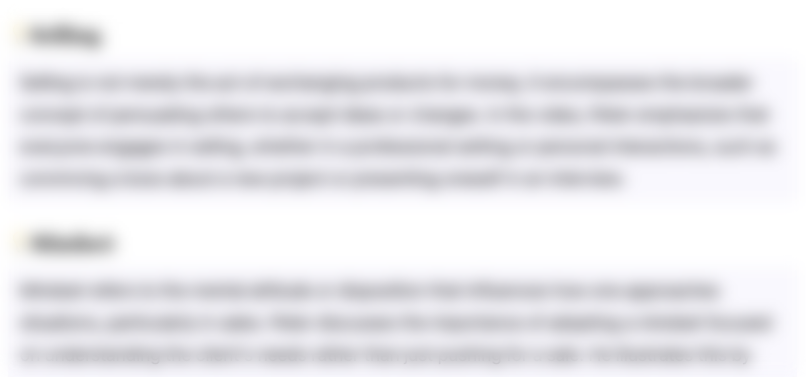
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
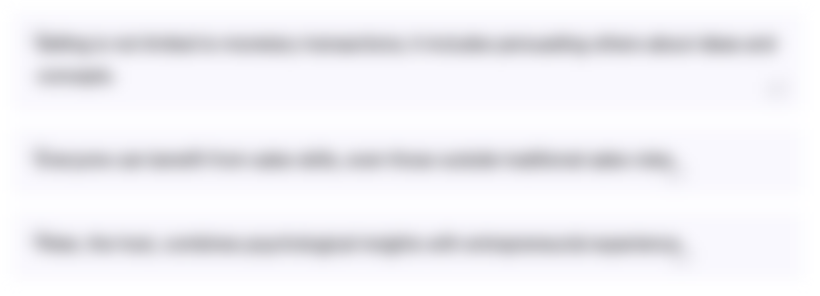
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
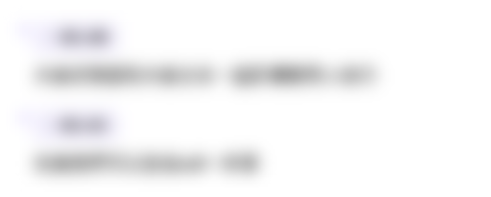
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
5.0 / 5 (0 votes)