ESP32 Arduino SPIFFS: Write file
Summary
TLDRIn this tutorial, the process of creating and writing to a file using the SPI Flash File System (SPIFFS) on an ESP32 is explained. The video covers the necessary steps to mount the SPIFFS, open a file for writing, and write content to it, using specific methods from the ESP32 SPIFFS library. It also demonstrates how to check for errors, ensure the file is correctly opened for writing, and close the file after writing. The tutorial ends with the successful creation of a file with written content, preparing viewers for future lessons on reading file content.
Takeaways
- 😀 SPIFFS (SPI Flash File System) is designed to work with flash memory in embedded systems, and is one of the file systems supported on the ESP32.
- 😀 The tutorial demonstrates how to write content to a file on the SPIFFS file system using the ESP32.
- 😀 The required library for interacting with the SPIFFS file system is `SPIFFS.h`, which provides methods for file system management.
- 😀 Before interacting with the file system, the `SPIFFS.begin()` method is used to mount the SPIFFS file system, which is necessary for accessing files.
- 😀 If the file system is not formatted, the `SPIFFS.begin()` method can format it automatically using a flag.
- 😀 File operations in the tutorial include opening a file, writing content to it, checking if content was written, and closing the file.
- 😀 To open a file, the `SPIFFS.open()` method is used, where the file path and mode (e.g., `FILE_WRITE`) are provided.
- 😀 The tutorial shows how to check for errors when opening a file by using the file object's boolean operator, which helps to verify if the file opened successfully.
- 😀 Content is written to the file using the `print()` method of the file object, which writes a string to the file in the specified mode.
- 😀 After writing to the file, the number of bytes written is checked to ensure content was successfully saved. If the value is 0 or less, an error message is displayed.
- 😀 Once file operations are complete, the `close()` method is called to finalize and close the file, ensuring all changes are saved.
Q & A
What is the SPIFFS file system and what is it used for?
-SPIFFS stands for SPI Flash File System. It is a file system designed to operate on flash storage devices, commonly used in embedded systems like the ESP32. It allows for file management tasks such as reading, writing, renaming, and deleting files on flash memory.
What is the role of the 'SPIFFS.begin()' method in the script?
-The 'SPIFFS.begin()' method is used to mount the SPIFFS file system, making it ready for file operations. It also takes an optional parameter that formats the file system if an error occurs during mounting, especially useful when using the file system for the first time.
Why is it important to call 'SPIFFS.begin()' before interacting with the file system?
-Calling 'SPIFFS.begin()' ensures that the file system is mounted properly before performing any file operations. Without this, trying to open or write to a file would result in errors because the file system would not be initialized.
What happens if the file system cannot be mounted?
-If the file system cannot be mounted, the script will return immediately, preventing any further file operations. This prevents attempts to read or write files when the file system is not properly initialized.
What does the 'file.open()' method do?
-The 'file.open()' method is used to open a file in a specified mode, such as read or write. In the script, the method is used to open a file in write mode, allowing content to be written to it.
How do you check if a file was successfully opened for writing?
-After calling 'file.open()', the script checks if the file object evaluates to true in an if condition. If the file cannot be opened, the object will return false, and the script will exit early to prevent further operations.
What does the 'file.print()' method do in the script?
-The 'file.print()' method writes content to the opened file. In this script, it is used to write a test string to the file. The method returns the number of bytes written, which is used to verify if the content was successfully saved.
What happens if the file fails to write the content?
-If the file fails to write content (i.e., the number of bytes written is zero), the script will print an error message indicating that the file write operation failed.
What is the purpose of the 'file.close()' method?
-The 'file.close()' method is used to close the file after performing operations like writing. This ensures that all changes are saved and the file is properly closed, releasing any resources associated with it.
What can you do with SPIFFS apart from writing and reading files?
-Apart from writing and reading files, SPIFFS also supports file management operations like renaming, deleting, and checking for the existence of files, which can be performed using appropriate methods provided by the file system's API.
Outlines
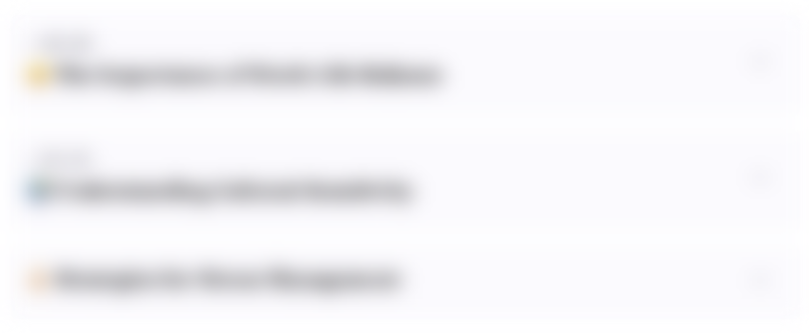
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
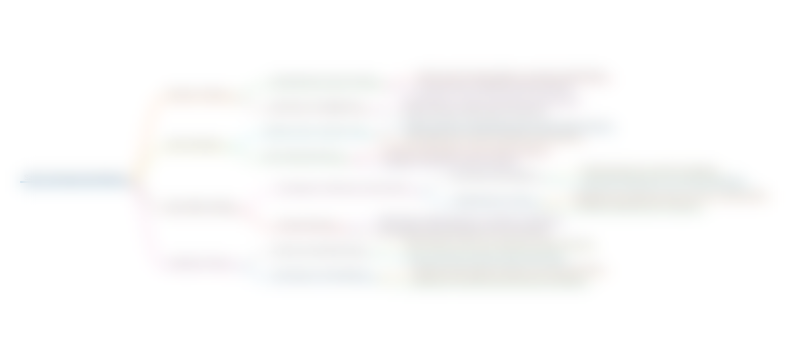
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
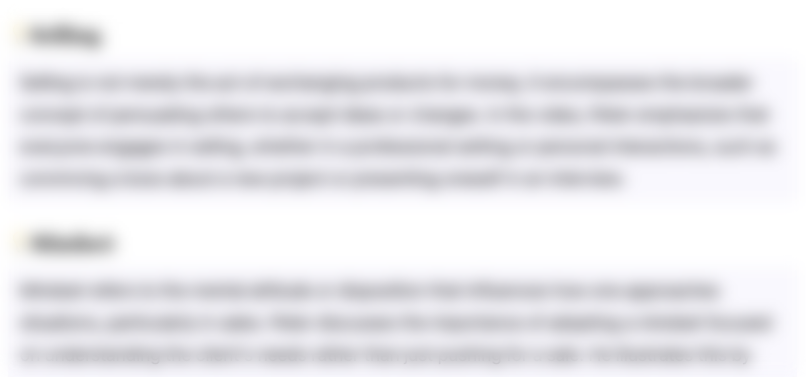
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
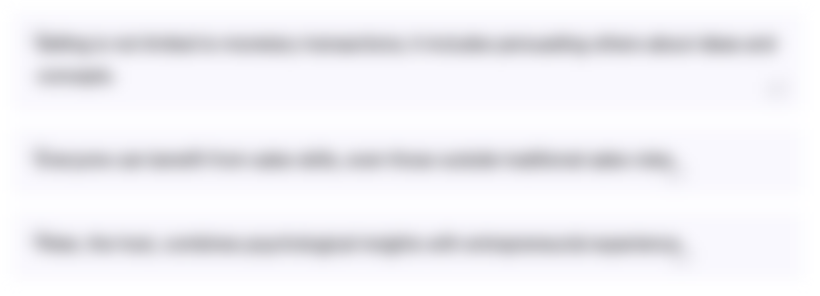
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
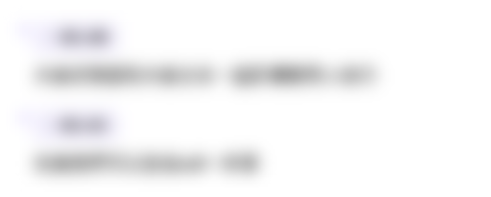
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantVoir Plus de Vidéos Connexes
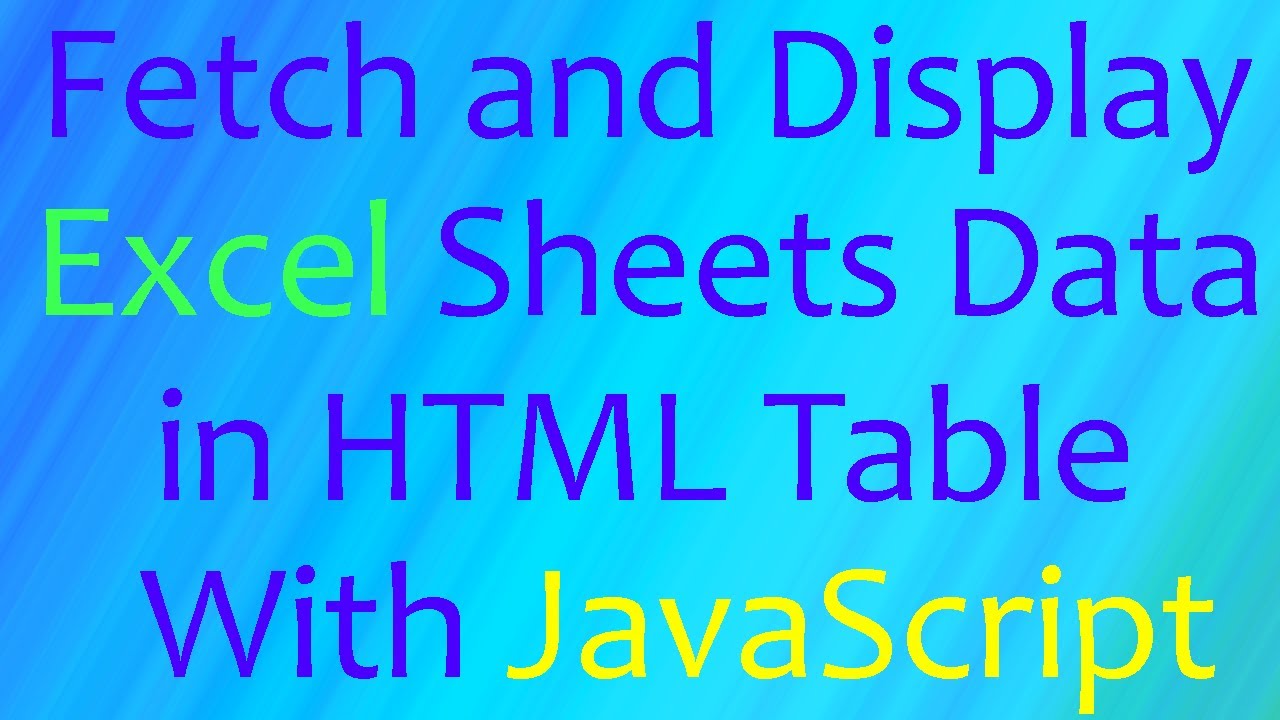
Fetch and Read Excel Sheets Data in HTML Table with JavaScript | Excel to HTML | JS | (Hindi)
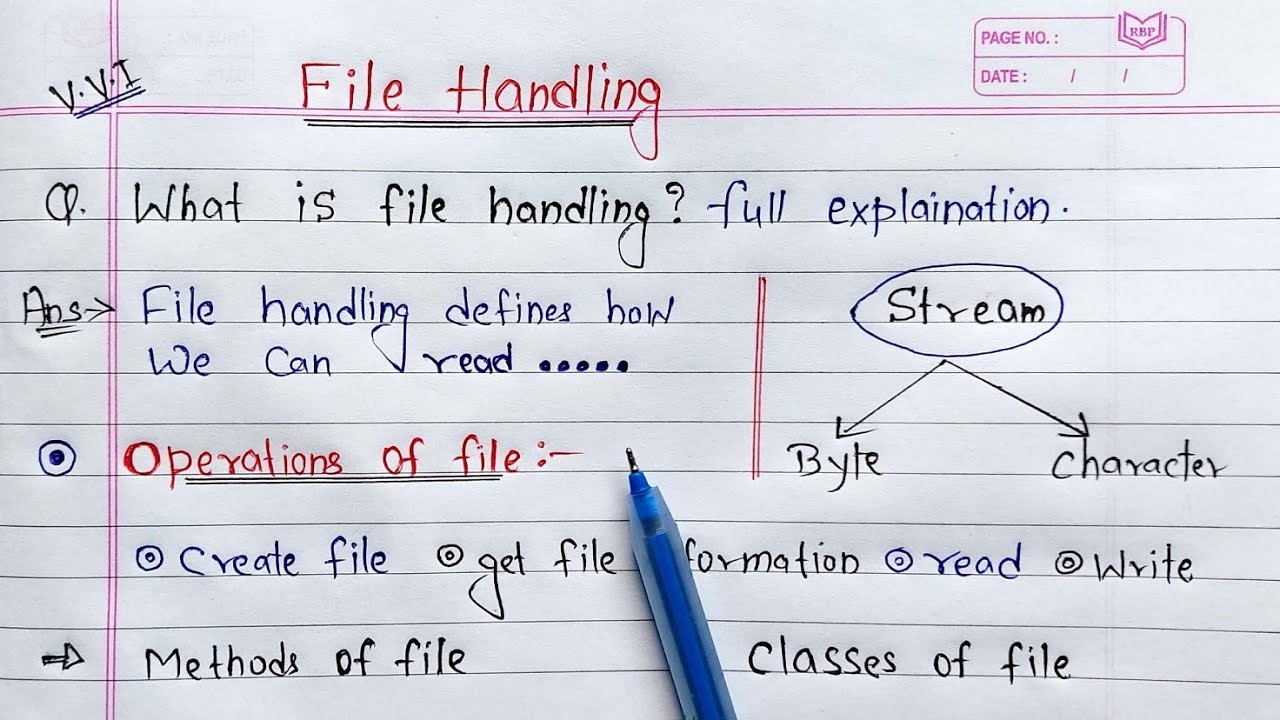
File Handling in Java | Java program to create a File
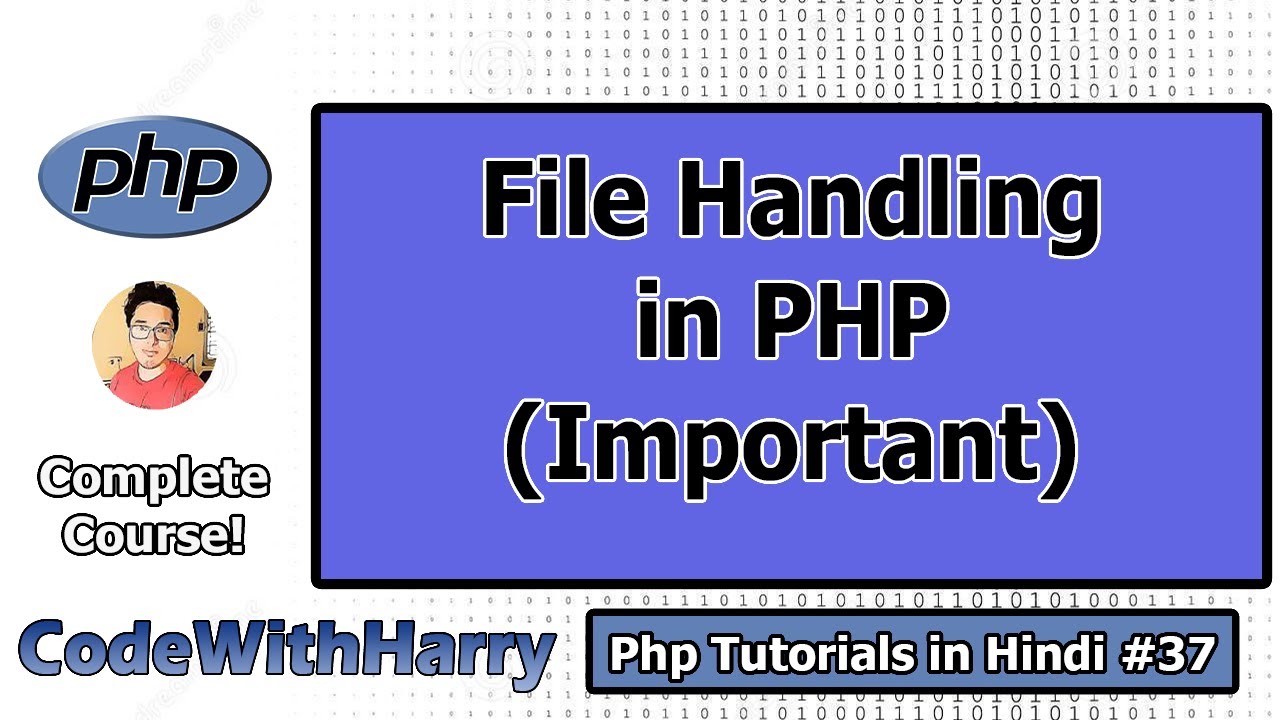
Writing and Appending to Files in PHP in Hindi | PHP Tutorial #37

OS5 - File Descriptors, File Descriptor Table
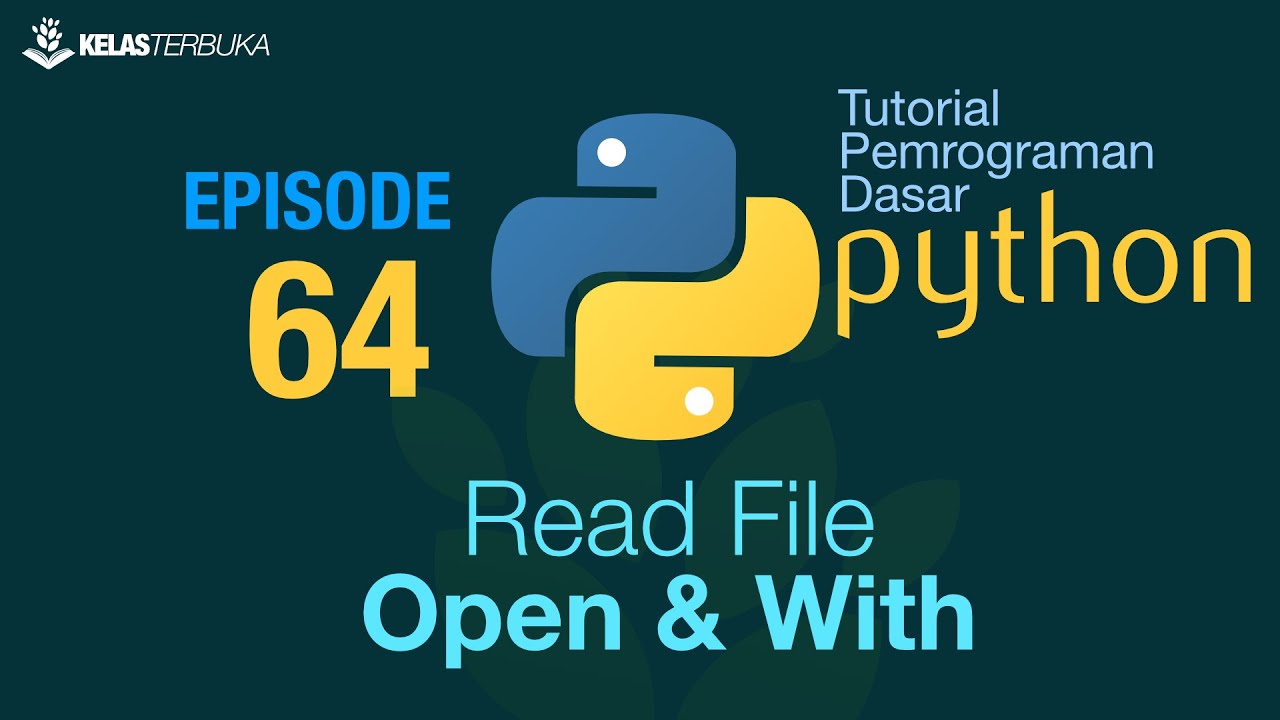
Belajar Python [Dasar] - 64 - Read external file - Open dan With
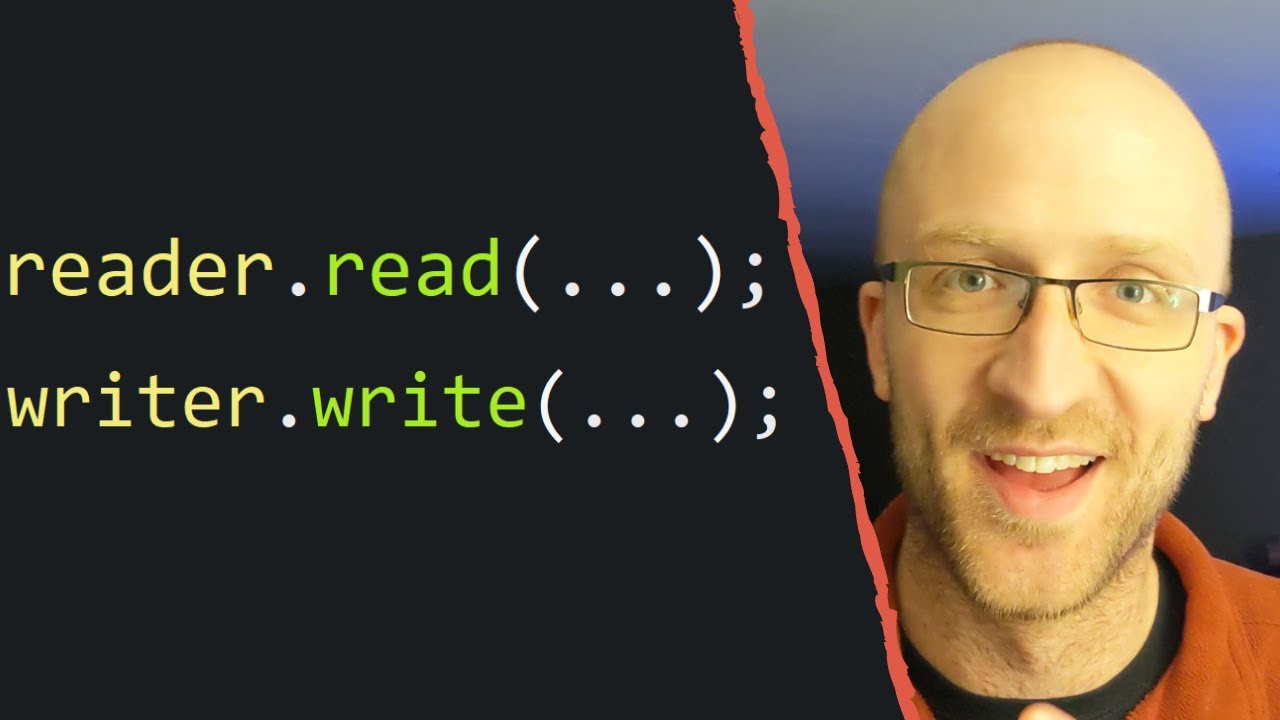
Java File Input/Output - It's Way Easier Than You Think
5.0 / 5 (0 votes)