Data structures: Binary Search Tree
Summary
TLDRThis lesson introduces binary search trees (BSTs), a specialized binary tree structure that enables efficient data organization for quick search, insertion, and deletion operations. The session compares arrays and linked lists, highlighting their performance drawbacks, especially with large datasets. It emphasizes the advantages of BSTs, where average-case complexities for operations can reach O(log n) if the tree remains balanced, while also addressing the consequences of unbalanced trees. The importance of maintaining balance and the fundamentals of tree operations are outlined, setting the stage for deeper exploration in future lessons.
Takeaways
- đ A binary search tree (BST) is a special type of binary tree optimized for efficient data organization, allowing quick searches and updates.
- đ For modifiable collections, arrays and linked lists are common data structures, but their search and modification times vary significantly.
- đ Searching in an unsorted array or linked list takes O(n) time, while insertion can be O(1) for arrays and O(1) or O(n) for linked lists, depending on the position.
- đ Sorted arrays allow for binary search with O(log n) complexity, but insertion and removal can still require O(n) time due to shifting elements.
- đ A balanced binary search tree can perform search, insertion, and deletion operations in O(log n) time on average, avoiding the O(n) worst-case scenario.
- đ The property of a binary search tree dictates that the left subtree contains only nodes with values less than or equal to the node, and the right subtree contains only nodes with values greater.
- đ An unbalanced binary search tree behaves similarly to a linked list, leading to inefficient operations with O(n) time complexity.
- đ To maintain efficiency, it is crucial to keep the binary search tree balanced during insertions and deletions.
- đ Insertion into a binary search tree requires finding the correct position, which can be done in O(log n) time, followed by a constant time link adjustment.
- đ Deletion from a binary search tree also involves searching for the node first, followed by adjusting links, maintaining an average time complexity of O(log n).
Q & A
What is a binary search tree (BST)?
-A binary search tree is a type of binary tree where for each node, the values of all nodes in its left subtree are less than or equal to the node's value, and the values of all nodes in its right subtree are greater.
How does the search operation in a binary search tree work?
-In a BST, to search for a value, start at the root and compare the target value to the node's value. If they match, the search is complete. If the target value is smaller, move to the left child; if larger, move to the right child, continuing this process until the value is found or a leaf node is reached.
What are the average and worst-case time complexities for search, insertion, and deletion in a binary search tree?
-In average cases, search, insertion, and deletion operations in a balanced BST have a time complexity of O(log n). In the worst case, if the tree is unbalanced, these operations can degrade to O(n).
Why is it important to keep a binary search tree balanced?
-Keeping a BST balanced ensures that the height of the tree remains logarithmic relative to the number of nodes, which optimizes the time complexity for search, insertion, and deletion operations.
What happens during an insertion operation in a binary search tree?
-To insert a value in a BST, first locate the appropriate position by comparing the value with nodes starting from the root, moving left or right as necessary. Once the correct spot is found, create a new node and link it as a child of the corresponding parent node.
How does removal work in a binary search tree?
-Removal in a BST involves first locating the node to be deleted. If the node has no children, it can be removed directly. If it has one child, the child replaces the node. If it has two children, find the node's in-order predecessor or successor, replace the node's value with that node, and then delete the predecessor or successor.
What is the difference in complexity between arrays and linked lists when it comes to insertion and removal?
-In arrays, insertion can be O(1) if space is available but O(n) if shifting is required. Removal is typically O(n) due to the need to shift elements. In linked lists, insertion at the head is O(1) but can be O(n) at the tail, while removal is O(n) for searching the element.
What role does sorting play in search efficiency in data structures?
-In sorted arrays, binary search can be used to find elements efficiently in O(log n) time. However, maintaining sorted order during insertion and removal can lead to O(n) complexity for these operations.
Can a binary search tree be unbalanced, and what is the impact of this?
-Yes, a BST can become unbalanced, which can lead to a structure similar to a linked list, resulting in O(n) time complexity for operations. This is why balancing strategies are important.
What are some methods for balancing a binary search tree?
-Balancing methods include AVL trees and Red-Black trees, which automatically adjust the structure during insertions and deletions to maintain logarithmic height.
Outlines
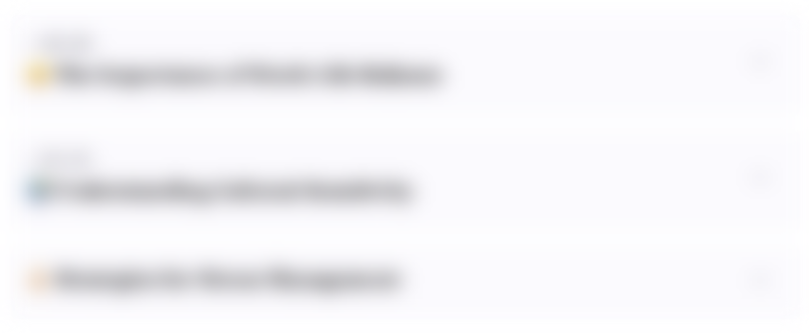
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
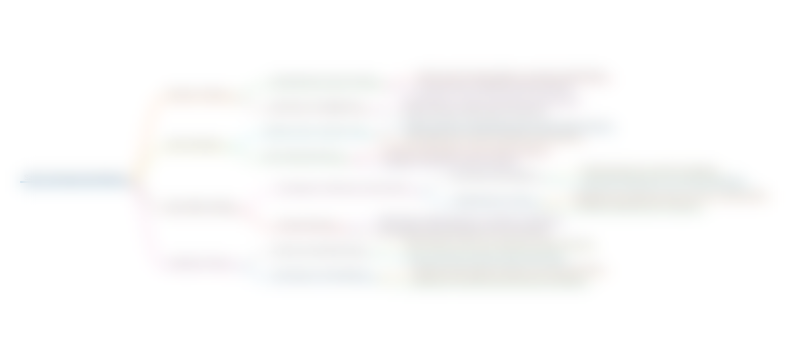
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
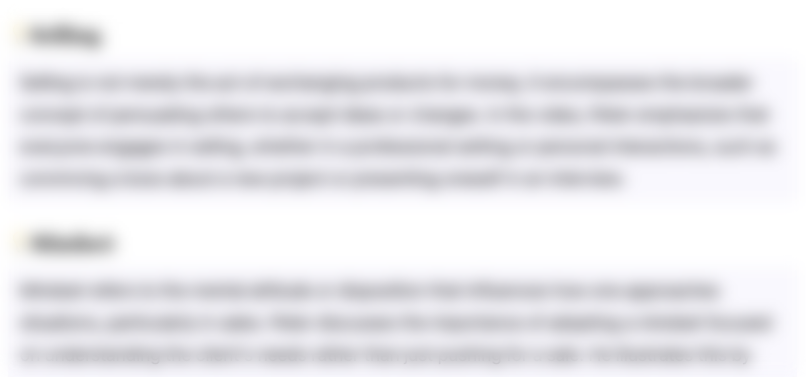
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
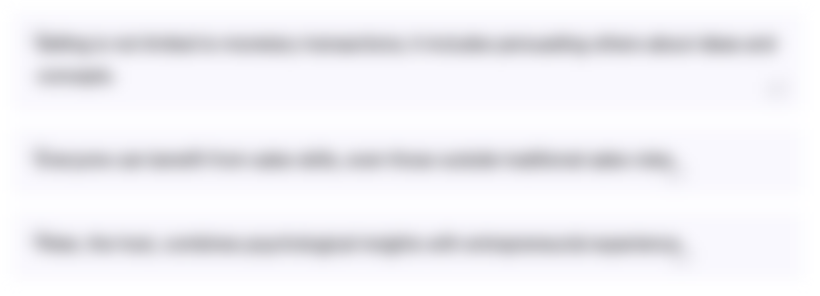
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
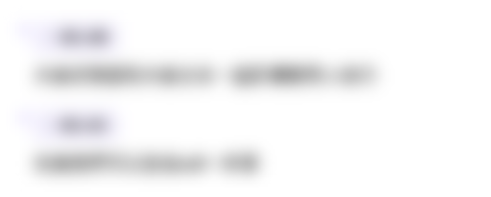
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantVoir Plus de Vidéos Connexes
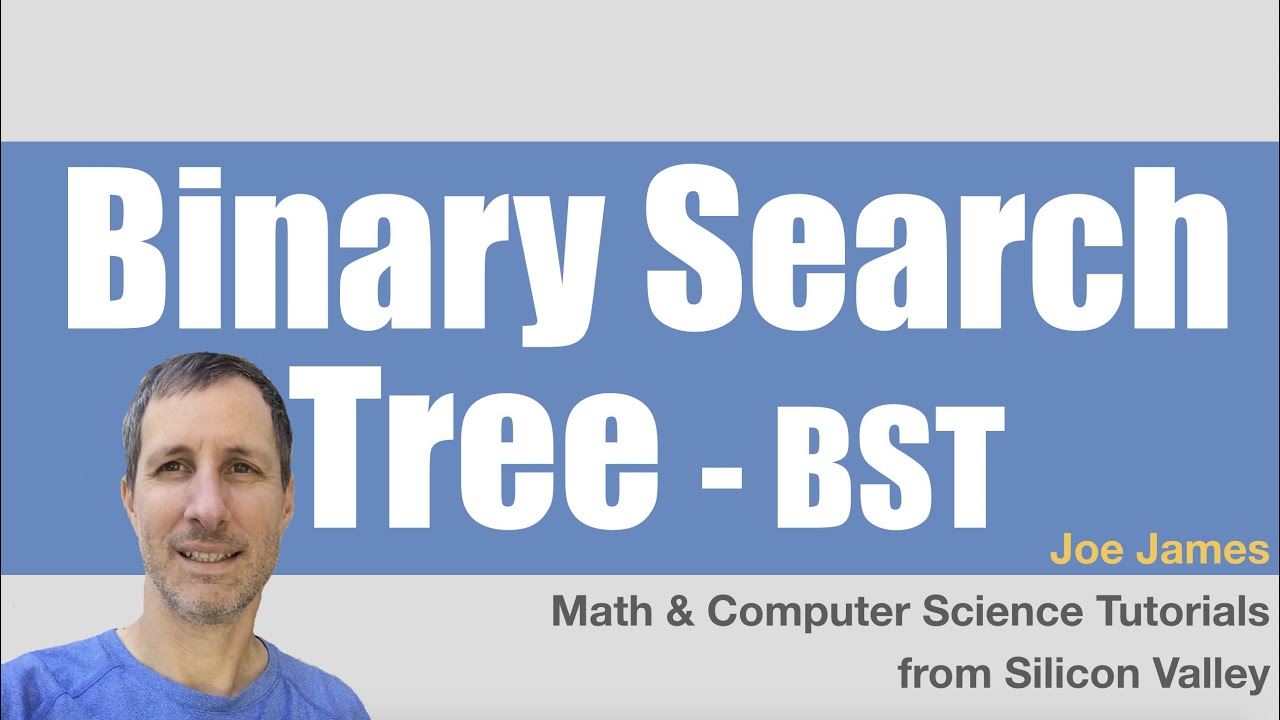
Binary Search Trees (BST) Explained in Animated Demo

Lecture 25 : Binary Search Tree (BST) Sort
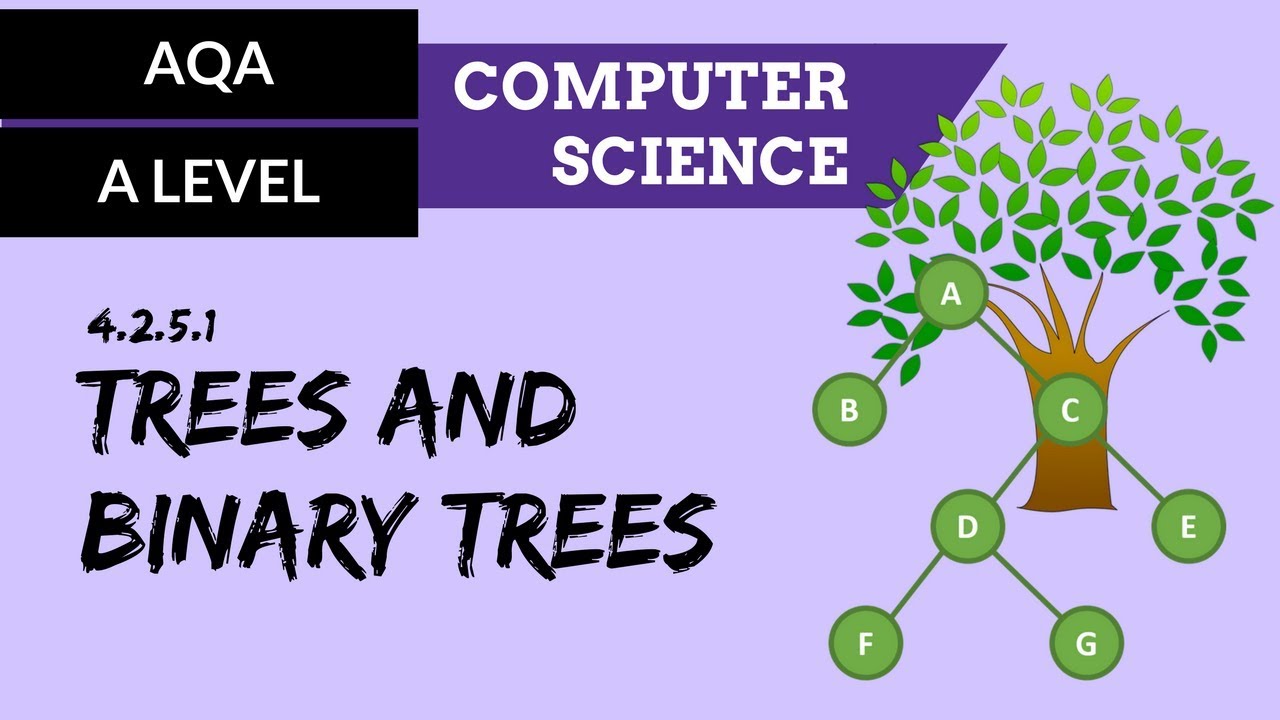
AQA AâLevel Trees & Binary trees
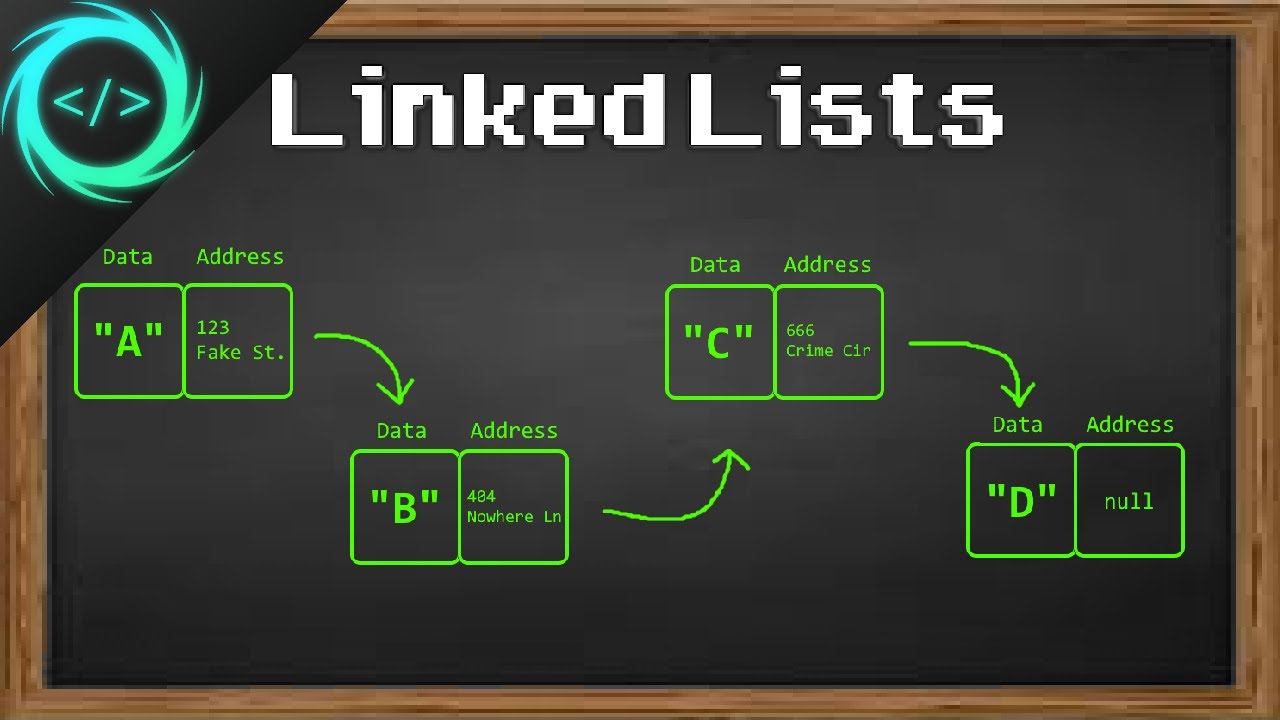
Learn Linked Lists in 13 minutes đ

L-3.7: Introduction to Trees (Binary Tree, Almost Complete Binary Tree, Full BT, Complete BT, BST)
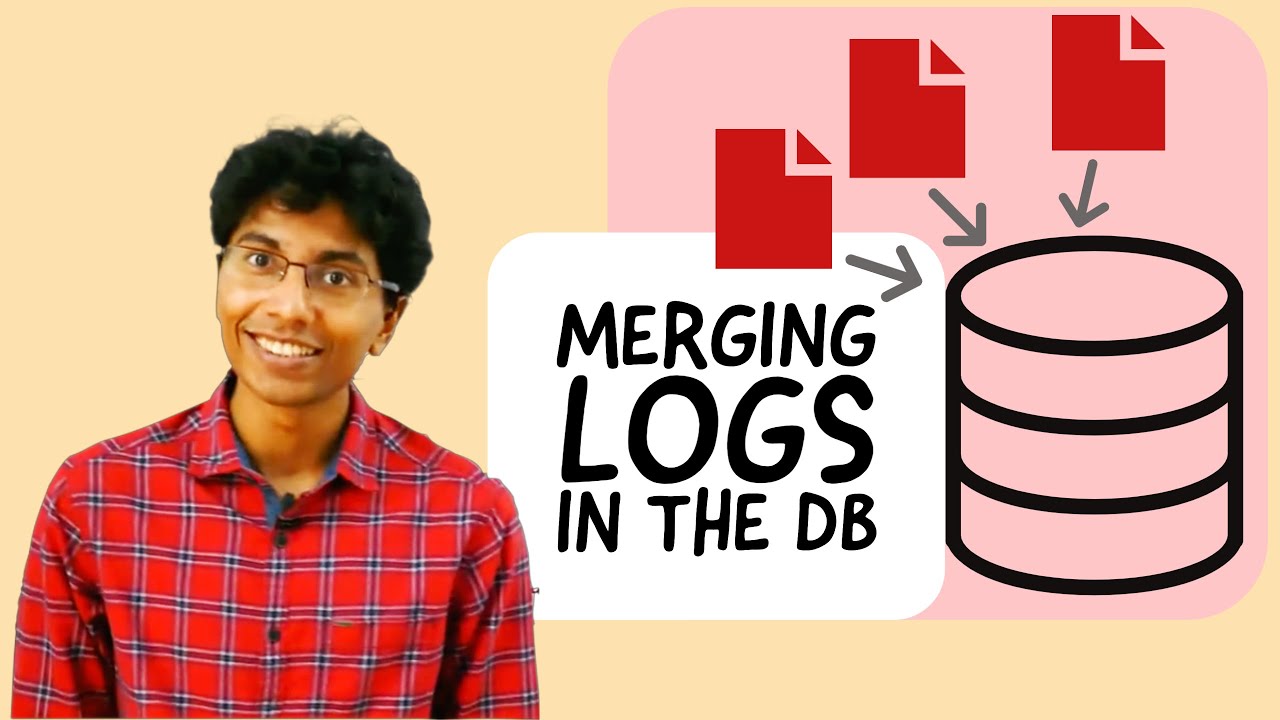
How databases scale writes: The power of the log âïžđïž
5.0 / 5 (0 votes)