#11 Python Tutorial for Beginners | Operators in Python
Summary
TLDRIn this Python tutorial, Ivan Vetti explores various operators including arithmetic, assignment, relational, and logical. He demonstrates arithmetic operations with variables and shows how to use assignment shortcuts like '+=' for incrementing values. Relational operators are explained with examples comparing variables, using symbols like '<', '>', '==', and '!='. Logical operators 'and', 'or', and 'not' are also covered, illustrating how to combine conditions using truth tables. The video serves as a foundational guide for more complex coding in future tutorials.
Takeaways
- đ The video series on Python continues with a focus on various types of operators.
- đą Arithmetic operators such as addition, subtraction, multiplication, and division are covered, demonstrating how to perform basic mathematical operations using variables.
- â Assignment operators are explained, showing how to assign values to variables and how to use shorthand operators like `+=` for incrementing values.
- đ The concept of unary operators is introduced, with an example of negation using the minus sign to reverse the sign of a number.
- đ Relational operators like `less than`, `greater than`, `equal to`, and `not equal to` are discussed, illustrating how to compare values.
- đ Logical operators `and`, `or`, and `not` are explained, showing how to combine multiple conditions in decision-making.
- đ A truth table is used to demonstrate the behavior of logical operators, helping to understand how true and false values interact.
- đ» The video emphasizes that understanding these operators is foundational for writing more complex Python code in the future.
- đ„ The presenter, Ivan, engages viewers by encouraging them to follow along with the series for more advanced topics.
- đ The video serves as an educational resource, aiming to build a strong base for viewers to tackle more complex programming concepts.
Q & A
What are the different types of operators discussed in the video?
-The video discusses arithmetic operators, assignment operators, relational operators, logical operators, and unary operators.
What is the purpose of arithmetic operators in Python?
-Arithmetic operators in Python are used to perform mathematical operations such as addition, subtraction, multiplication, division, and modulus.
How does the assignment operator work in Python?
-The assignment operator in Python is represented by the '=' symbol, which is used to assign a value to a variable.
What is the shortcut for adding a value to a variable in Python?
-In Python, the shortcut for adding a value to a variable is using the '+=' operator, which increments the variable by the specified value.
Can you assign values to multiple variables in a single line in Python?
-Yes, in Python, you can assign values to multiple variables in a single line using a comma-separated list of variables and values.
What is a unary operator and how is it used in Python?
-A unary operator is an operator that takes only one operand. In Python, the unary operator '-' is used to negate a value, making it negative.
What are relational operators and how are they used to compare values?
-Relational operators are used to compare two values and determine their relationship. They include operators like '<' for less than, '>' for greater than, '==' for equality, and '!=' for inequality.
What is the logical operator 'and' used for in Python?
-The logical operator 'and' is used in Python to combine two conditions, and the result is true only if both conditions are true.
How does the logical operator 'or' differ from 'and' in Python?
-The logical operator 'or' in Python returns true if at least one of the conditions is true, unlike 'and' which requires both conditions to be true.
What is the purpose of the logical operator 'not' in Python?
-The logical operator 'not' in Python is used to reverse the boolean value of an expression, turning true to false and vice versa.
Outlines
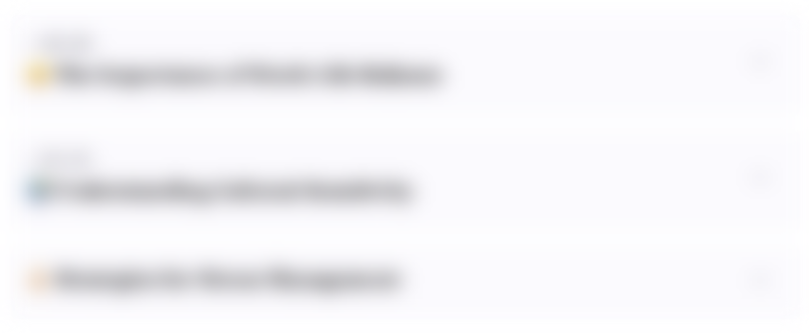
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
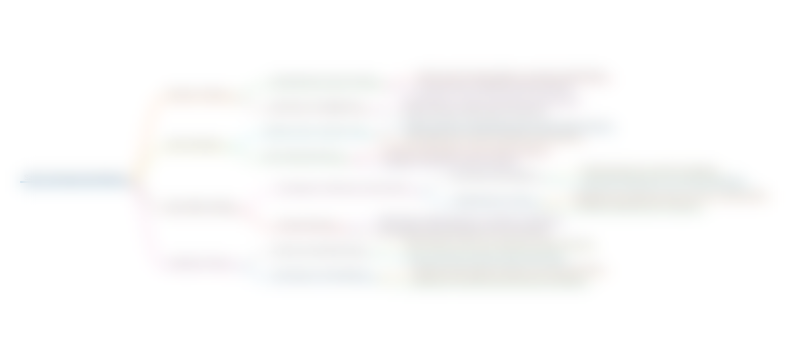
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
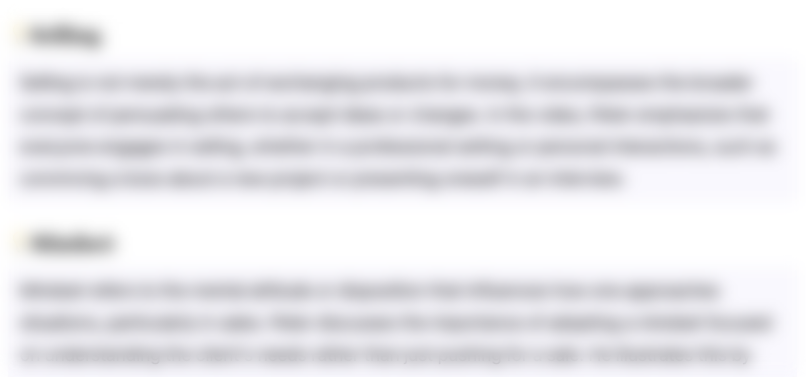
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
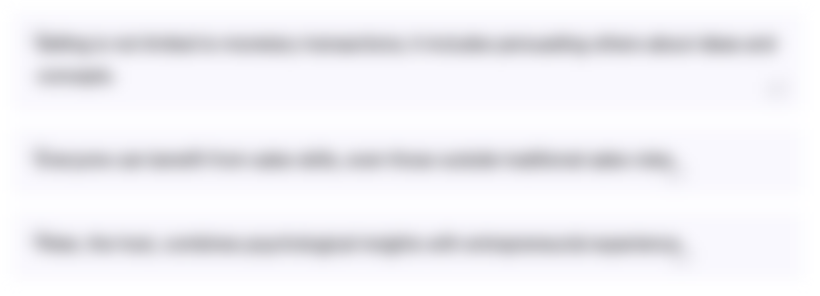
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
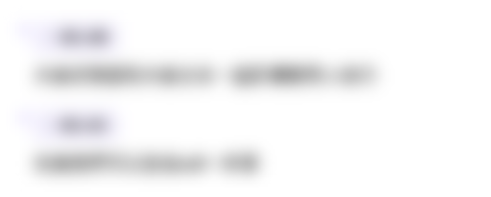
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantVoir Plus de Vidéos Connexes
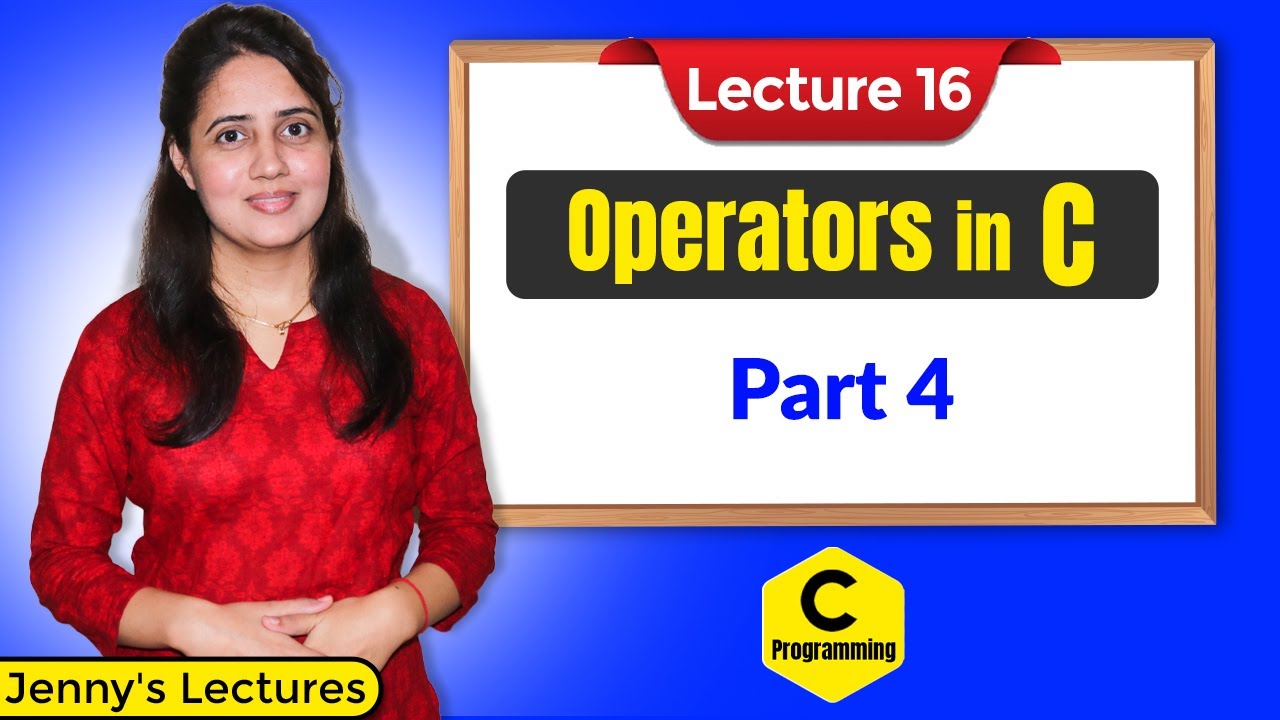
C_16 Operators in C - Part 4 | C Programming Tutorials
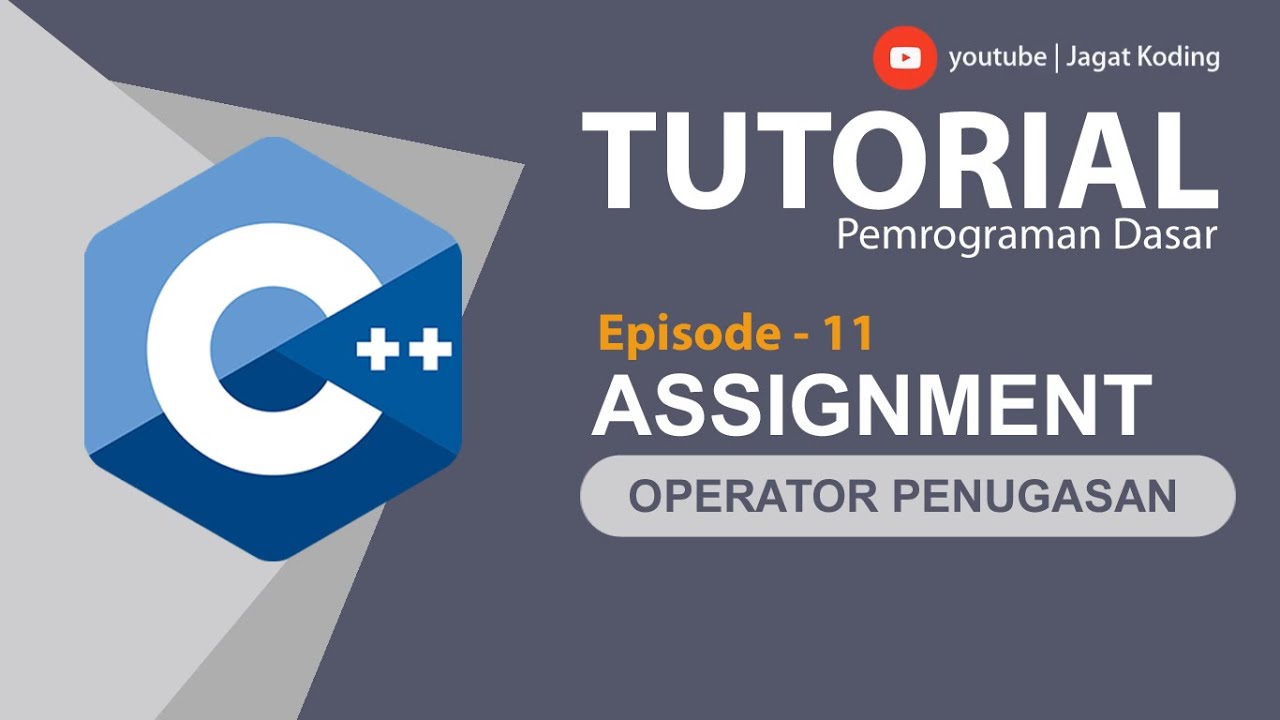
C++ 11 | Operator Assignment | Tutorial Pemrograman C++
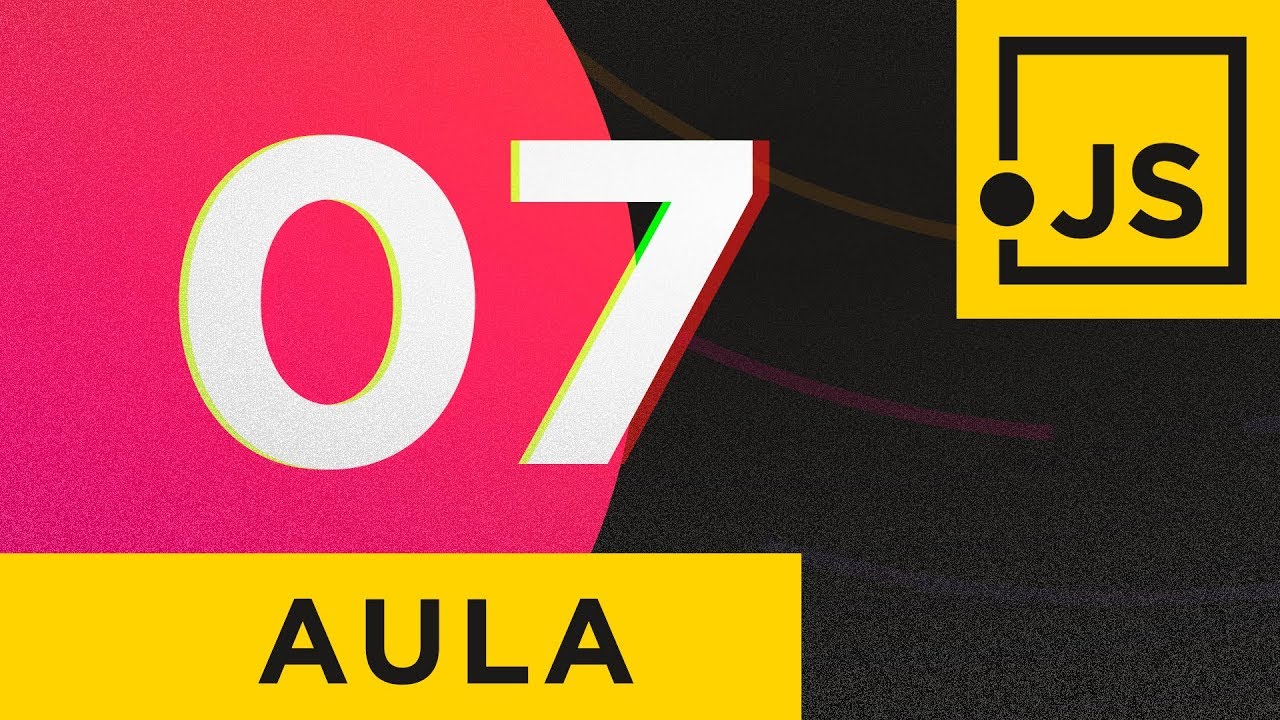
Operators (Part1) - JavaScript Course #07
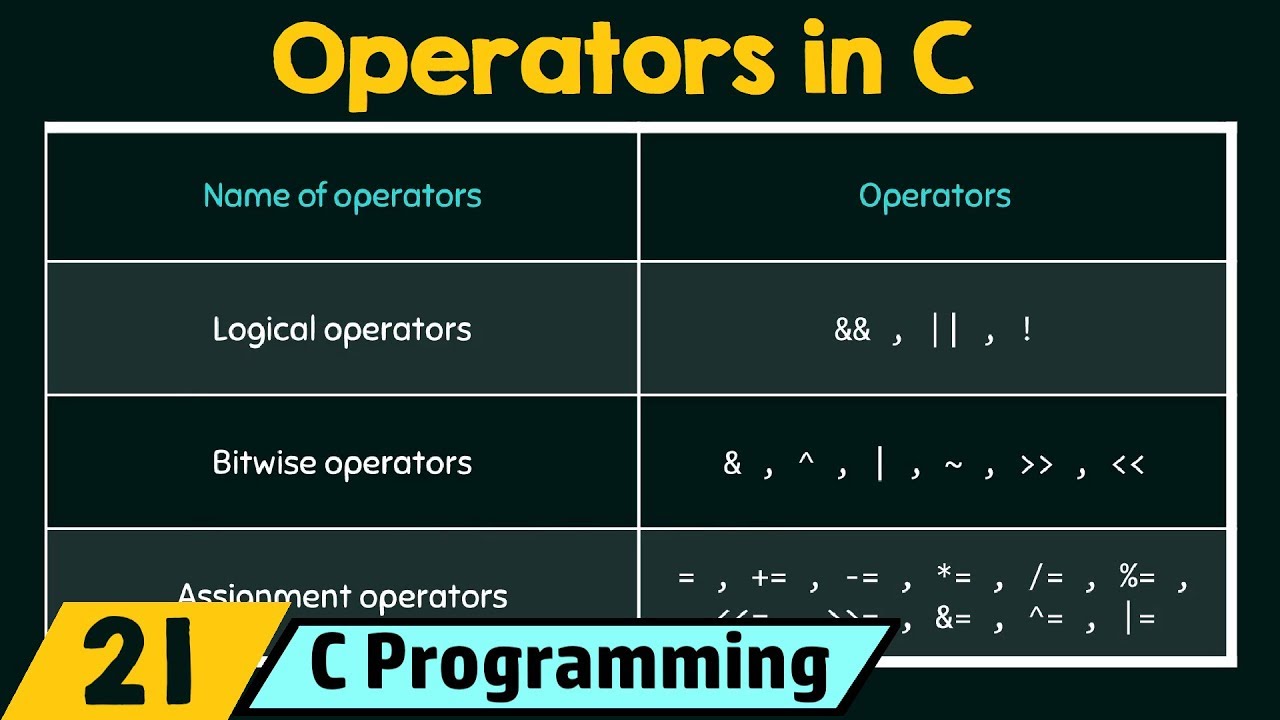
Introduction to Operators in C
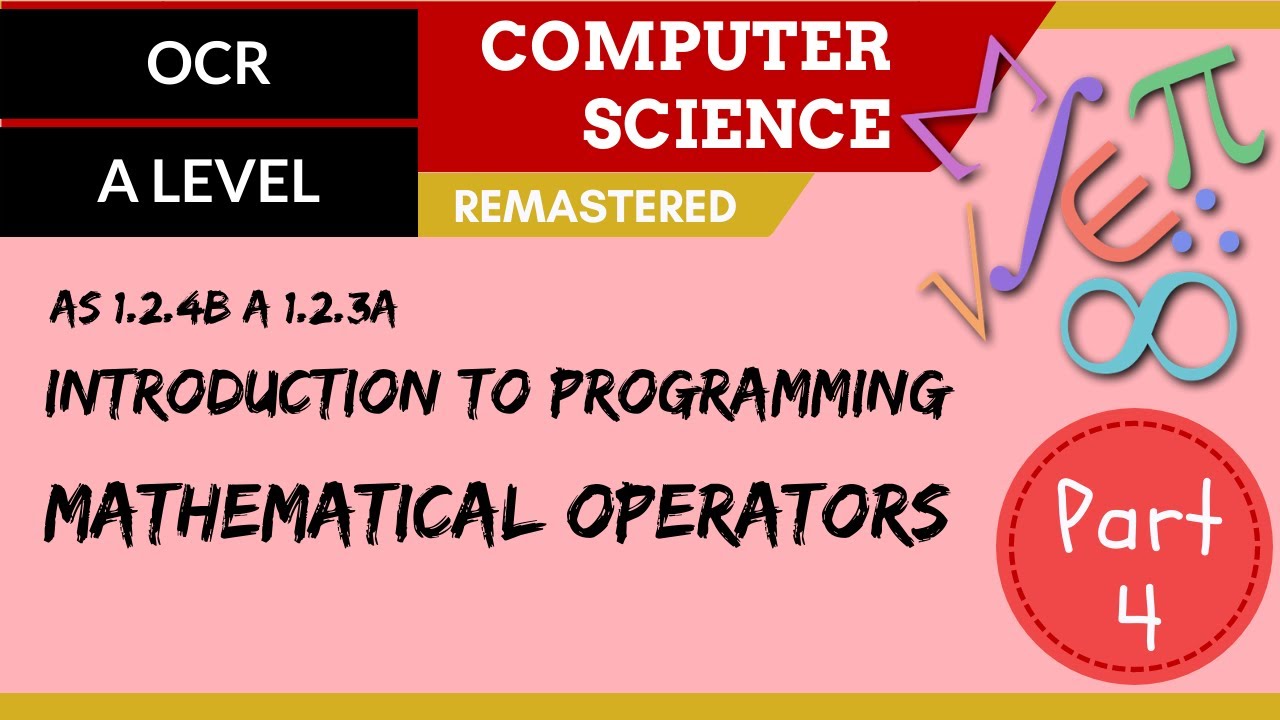
43. OCR A Level (H046-H446) SLR8 - 1.2 Introduction to programming part 4 mathematical operators
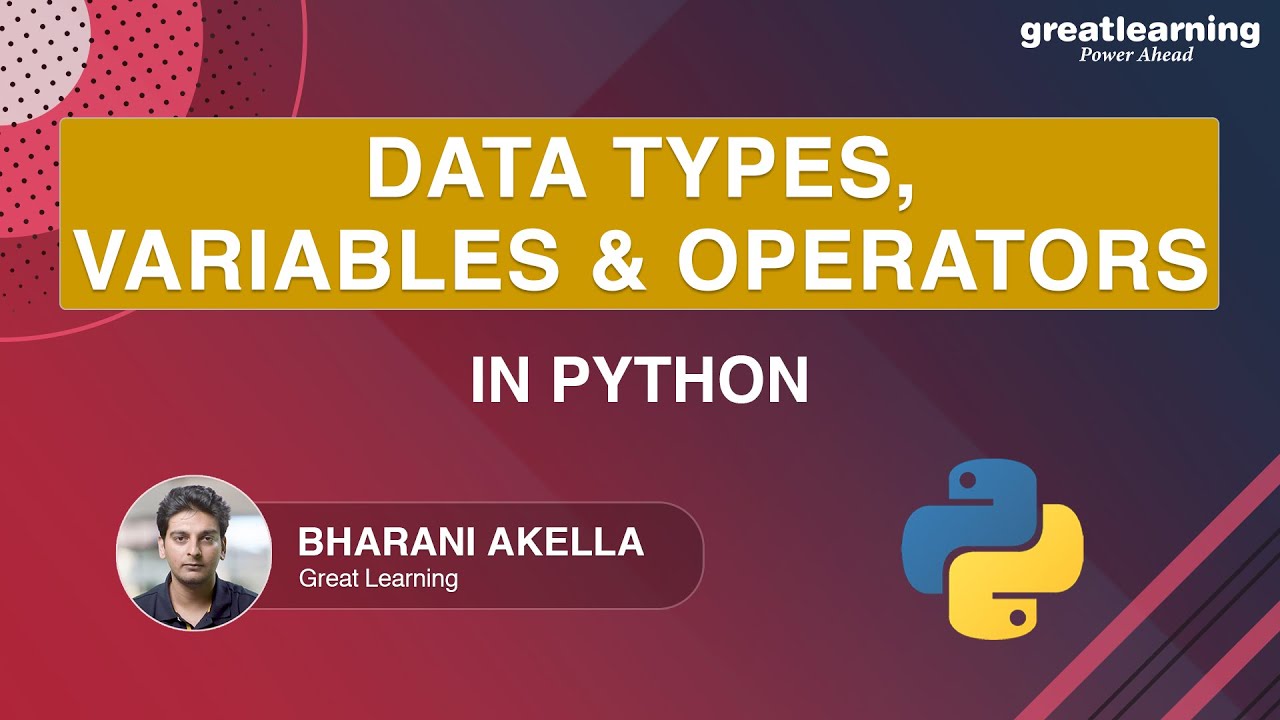
Data Types Variables And Operators In Python | Python Fundamentals | Great Learning
5.0 / 5 (0 votes)