#16 Creating a Custom Middleware | Understanding Middleware | ASP.NET Core MVC Course
Summary
TLDRIn this lecture, the instructor demonstrates how to create and use custom middleware in an ASP.NET Core application. Starting with setting up a new project, the video walks through the process of configuring middleware to handle HTTP requests. It explains the concept of middleware functions, how they can be chained, and the use of terminal middleware to handle requests. The tutorial highlights how middleware functions are executed in sequence, the importance of calling the `next()` method to chain them, and the concept of short-circuiting middleware using the `Run()` method. A great introduction for those wanting to understand ASP.NET Core's middleware pipeline.
Takeaways
- 😀 Creating an ASP.NET Core project begins with selecting the 'Empty' template, which allows for a custom setup and configuration of the application.
- 😀 The 'Program.cs' file is the starting point of the application, where we configure the web application using 'WebApplicationBuilder' and build the application instance.
- 😀 Middleware in ASP.NET Core is a function that handles HTTP requests and responses, allowing us to define how requests are processed.
- 😀 The 'Run()' method is used to define a callback function for handling requests in a simple middleware, which can modify the response based on the HTTP context.
- 😀 To handle asynchronous tasks in middleware, we make the callback function 'async' and use the 'await' keyword to wait for operations to complete.
- 😀 The callback function inside the 'Run()' method serves as middleware and is executed for each request, but it must be configured correctly to handle requests.
- 😀 Multiple middleware functions can be created, and their execution order is important; they are processed in the order they are defined.
- 😀 When using the 'Run()' method, if the 'next()' method is not called, the pipeline terminates, and the next middleware in the sequence will not execute.
- 😀 Middleware created with 'Run()' is considered terminal or short-circuiting, meaning it doesn't pass the request to the next middleware in the pipeline.
- 😀 To chain multiple middleware functions, you need to explicitly call the 'next()' method in each middleware to allow subsequent middleware to execute.
- 😀 Understanding terminal middleware and short-circuiting helps in deciding when middleware should terminate the request pipeline and when it should allow further processing.
Q & A
What is the first step in creating a custom middleware in ASP.NET Core?
-The first step is to create a new ASP.NET Core project using the 'ASP.NET Core Empty' template. You should select C# as the language and provide a project name, such as 'middleware', and specify the location for saving the project.
What does the 'WebApplicationBuilder' instance do in the 'Program.cs' file?
-The 'WebApplicationBuilder' instance allows for configuring the ASP.NET Core application. It is used to set up the application and services before the 'WebApplication' instance is created.
How is the ASP.NET Core application created and hosted on the server?
-An instance of the 'WebApplication' class is created by calling the 'Build' method on the 'WebApplicationBuilder' instance. The 'Run' method is then called to start the server and host the application.
What is the purpose of the 'Run' method in the 'Program.cs' file?
-The 'Run' method starts the server and hosts the ASP.NET Core application. It takes a callback function that processes incoming requests and sends a response back to the client.
What happens when a callback function is passed to the 'Run' method?
-When a callback function is passed to the 'Run' method, it receives an 'HTTPContext' object. This function acts as middleware, handling requests and providing responses for each incoming request.
What is middleware in ASP.NET Core?
-Middleware in ASP.NET Core is a function that handles HTTP requests and responses. It processes requests, performs tasks such as logging or authentication, and then passes the request to the next middleware in the pipeline or sends a response back to the client.
Why does the middleware function not get executed for certain URLs?
-The middleware function is not executed if the correct conditions are not met, such as the request not matching the defined route. In the example, the server was not aware of how to handle the requests until the callback function was passed to the 'Run' method.
What is the effect of not calling the 'next' method in middleware?
-If the 'next' method is not called in middleware, the following middleware in the pipeline will not be executed. This prevents the request from continuing down the pipeline and may result in the response not being processed as expected.
What is the difference between 'Run' middleware and other types of middleware?
-The 'Run' middleware creates a terminal or short-circuiting middleware. This means it does not call the 'next' method and prevents subsequent middleware from being executed. In contrast, other middleware types can call 'next()' to pass the request to the next middleware in the pipeline.
How can multiple middlewares be chained together in ASP.NET Core?
-To chain multiple middlewares, you need to use middleware methods that support calling the 'next()' method, which allows the request to pass through the chain of middlewares. The 'Run' method does not support this, so a different approach is needed for chaining middlewares.
Outlines
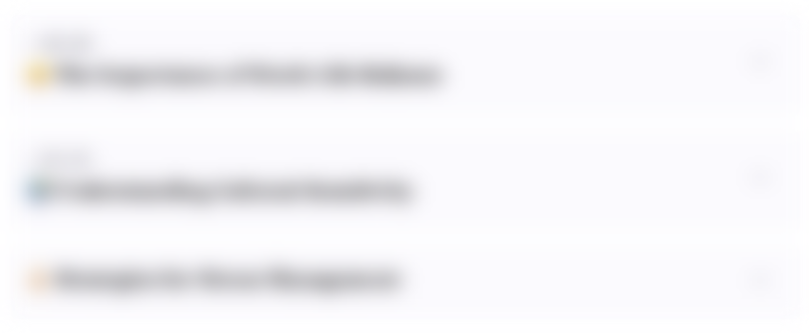
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
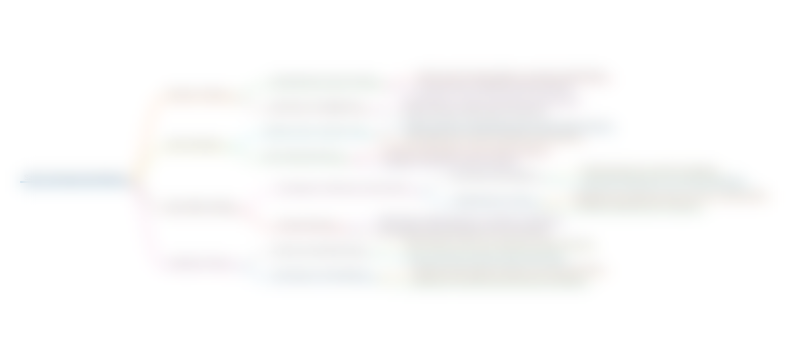
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
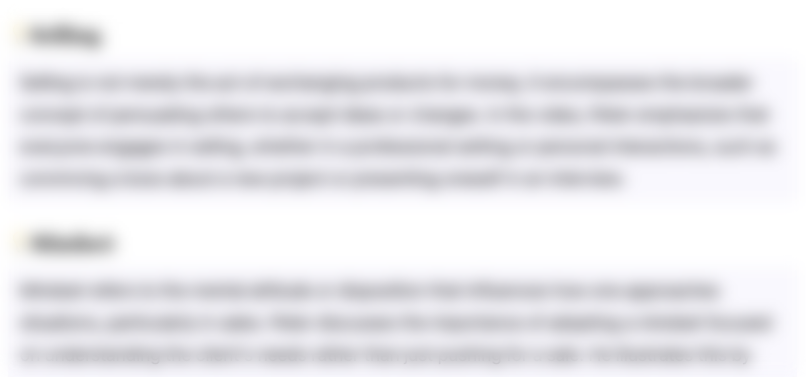
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
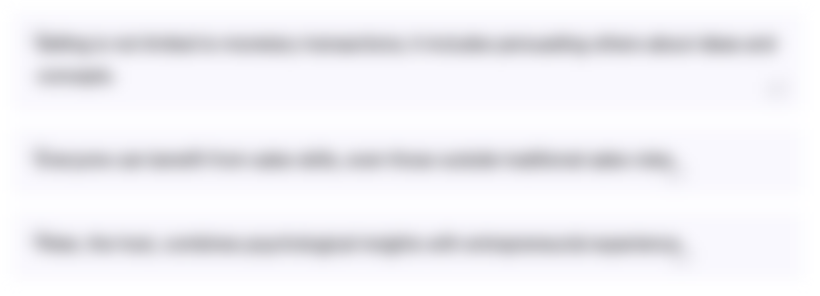
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
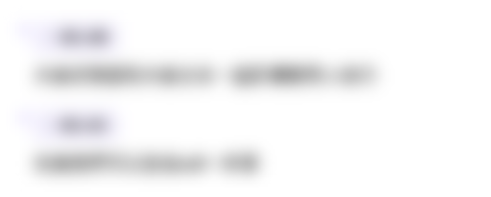
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraVer Más Videos Relacionados

#32 Custom Route Constraint | Understanding Routing | ASP.NET Core MVC Course
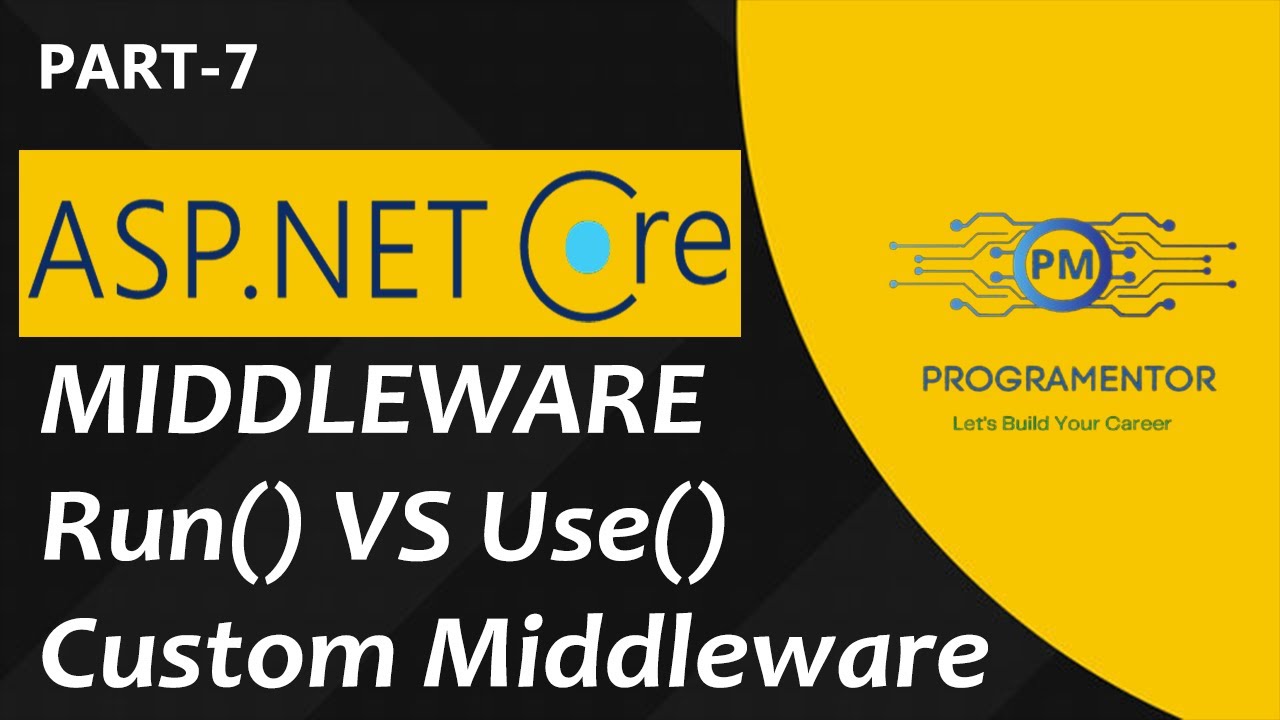
07 - Middleware In ASP.NET Core 6 | Run VS Use In ASP.NET Core | Custom Middleware (Hindi/Urdu)

useContext Hook | Mastering React: An In-Depth Zero to Hero Video Series
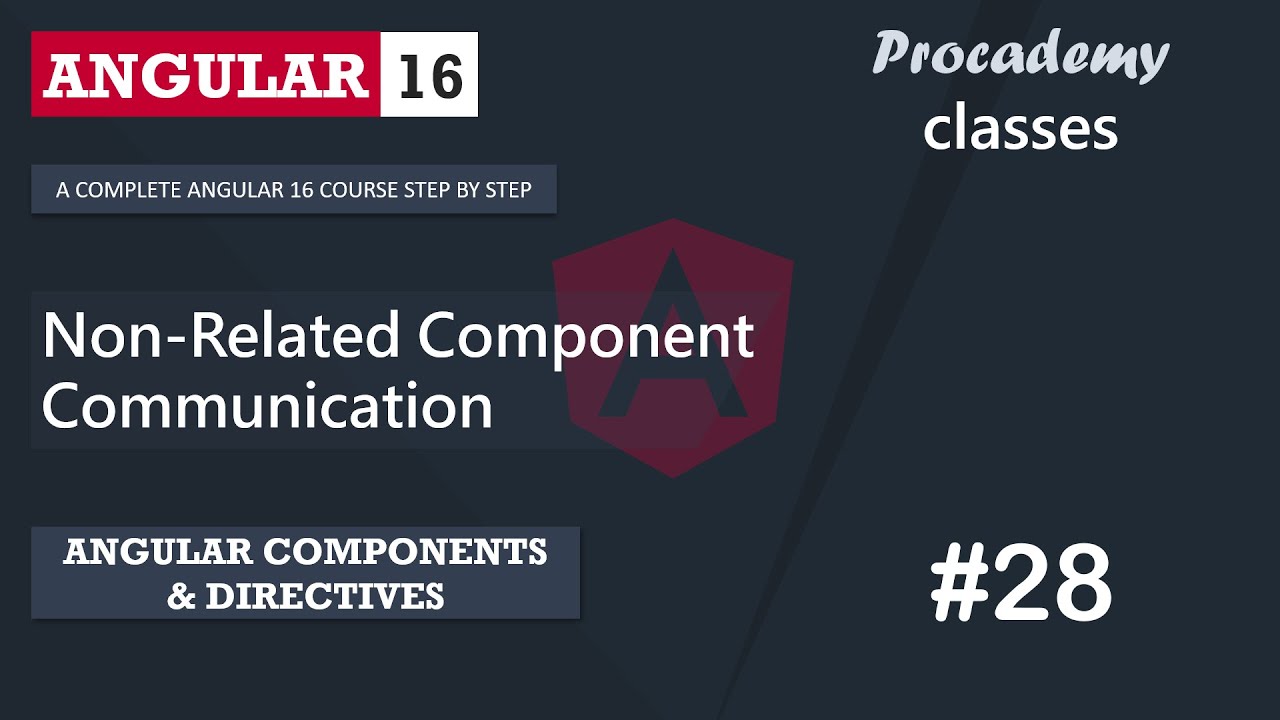
#28 Non-Related Component Communication | Angular Component & Directives | A Complete Angular Course
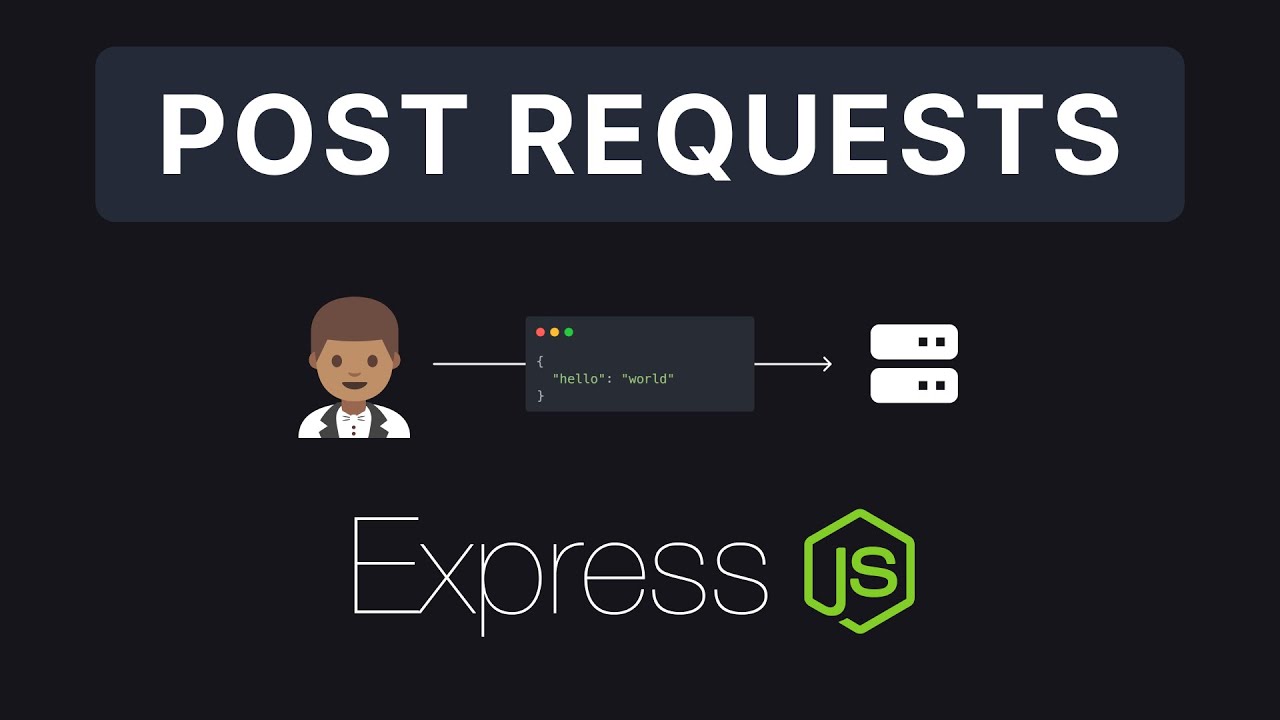
Express JS #5 - Post Requests
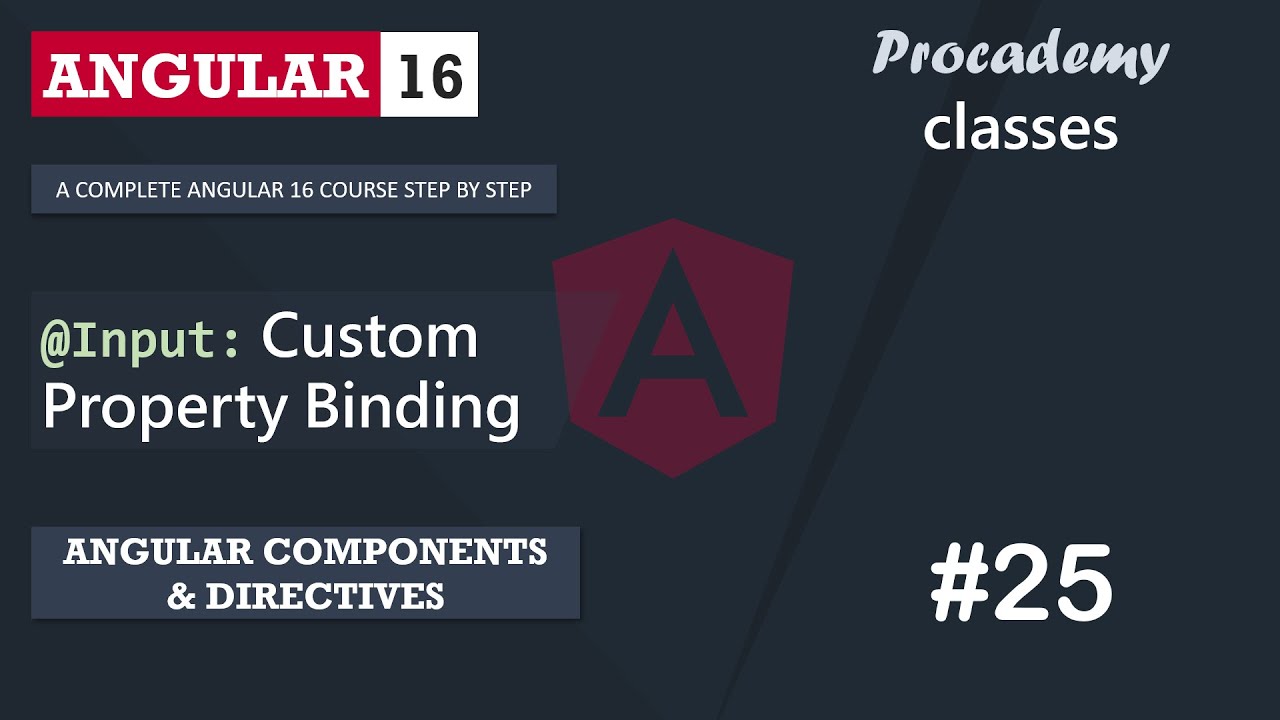
#25 @Input: Custom Property Binding | Angular Components & Directives | A Complete Angular Course
5.0 / 5 (0 votes)