Your Second Day in C (Understand .h header and .c source files) - Crash Course in C Programming
Summary
TLDRThis video tutorial introduces key C programming concepts, focusing on how to structure code using header files (.h) and source files (.c). It covers creating custom data types, defining and declaring functions, and organizing code to enhance readability and maintainability. The video demonstrates how to compile C programs with GCC, use header guards to prevent duplicate inclusions, and pass data through pointers. By the end, viewers will understand how to modularize their code, work with custom data types, and organize complex programs effectively, laying a strong foundation for more advanced C programming.
Takeaways
- 😀 Header files (.h) are used to declare function prototypes and data types for easy reuse in multiple source files.
- 😀 Header guards prevent multiple inclusions of the same header file, ensuring efficiency and avoiding errors during compilation.
- 😀 Functions can be declared in header files but must be defined in a corresponding .c file, allowing for modular code.
- 😀 When defining custom data types (like vectors), use structs in C and declare them in header files to be used across different parts of the program.
- 😀 C function names are case-sensitive, meaning you can define functions with the same name as long as they use different cases.
- 😀 Be careful with the scope of local variables in C—returning them from functions can lead to undefined behavior because they go out of scope after the function ends.
- 😀 Use pointers as output parameters when you need to modify values in a function and ensure changes persist outside the function.
- 😀 A modular approach to C programming (splitting code into .h and .c files) helps maintain and scale large codebases, particularly in team environments.
- 😀 When compiling in C, you typically compile the .c files, which are linked together to create the executable, with header files serving as references.
- 😀 The gcc compilation process involves linking object files and libraries, resulting in an executable that can be run from the command line.
- 😀 Proper use of the `#include` directive and header files allows you to keep your code organized, reusable, and easier to manage in large projects.
Q & A
What is the main purpose of header files in C programming?
-Header files in C programming serve as an interface to other parts of the program, providing function declarations and type definitions. They allow functions and types to be shared across multiple source files without duplicating the actual implementation.
Why is it important to use header guards in `.h` files?
-Header guards prevent multiple inclusions of the same header file, which could lead to redefinition errors during the compilation process. They ensure that the contents of a header file are included only once.
What is the role of the `#include` directive in C programming?
-The `#include` directive is used to include the contents of a header file into a source file. This allows the source file to access the declarations (functions, structures, etc.) defined in the header file, enabling code reuse and modularity.
How does C handle the compilation process when using multiple `.c` and `.h` files?
-When using multiple `.c` and `.h` files, the `gcc` compiler compiles each `.c` file separately, linking them together to form an executable. The `.h` files are included in the `.c` files to provide the necessary function declarations and type definitions.
Why is it necessary to split code into multiple files, such as `.c` and `.h` files?
-Splitting code into multiple files helps organize a program, making it easier to maintain, scale, and debug. It also supports modularity, allowing different parts of the program to be worked on independently by different developers.
What is the difference between a `.c` file and a `.h` file?
-A `.c` file contains the implementation of functions and types, while a `.h` file contains declarations (prototypes) of functions and types that are used in the `.c` files. The `.h` files provide an interface to the `.c` files.
How do you ensure that a function defined in a `.c` file is available in other files?
-To make a function available in other files, you declare the function prototype in a `.h` file and include that `.h` file in the other `.c` files that need to use the function.
What is the significance of returning values using pointers in C?
-Returning values using pointers allows the function to modify variables in the caller’s scope directly. This is especially useful when returning large data structures or when multiple values need to be returned from a function.
What does the process of linking do during the compilation of a C program?
-Linking is the process where the compiler combines object files (compiled from `.c` files) into a single executable. It also links external libraries, such as the standard library, to provide additional functionality like `printf` or `malloc`.
What is the benefit of defining custom data types like `vector` in C?
-Defining custom data types, such as `vector`, allows for more organized, readable, and maintainable code. It also enables complex data structures to be manipulated using custom functions, improving abstraction and modularity.
Outlines
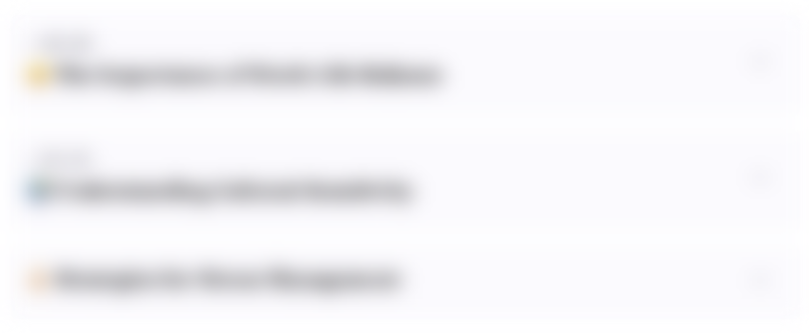
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
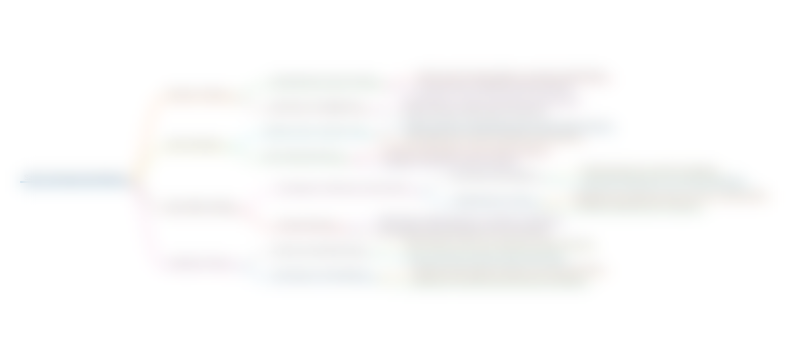
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
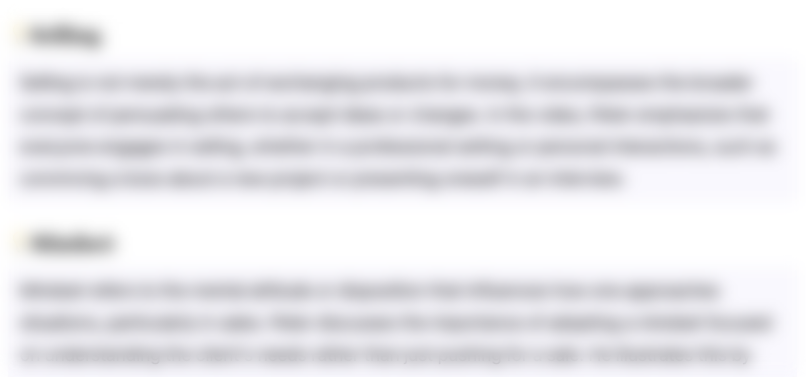
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
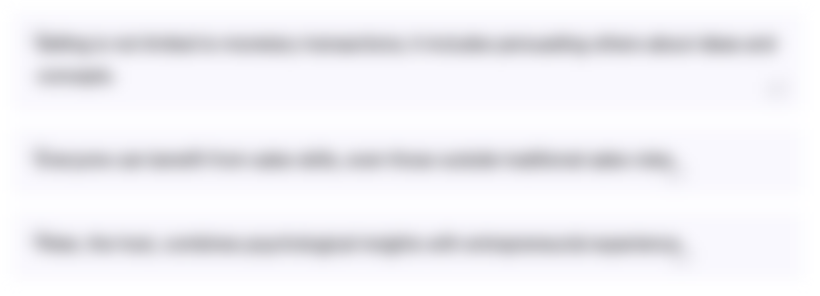
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
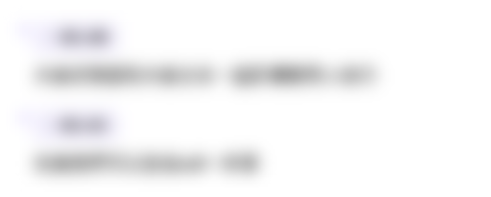
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraVer Más Videos Relacionados
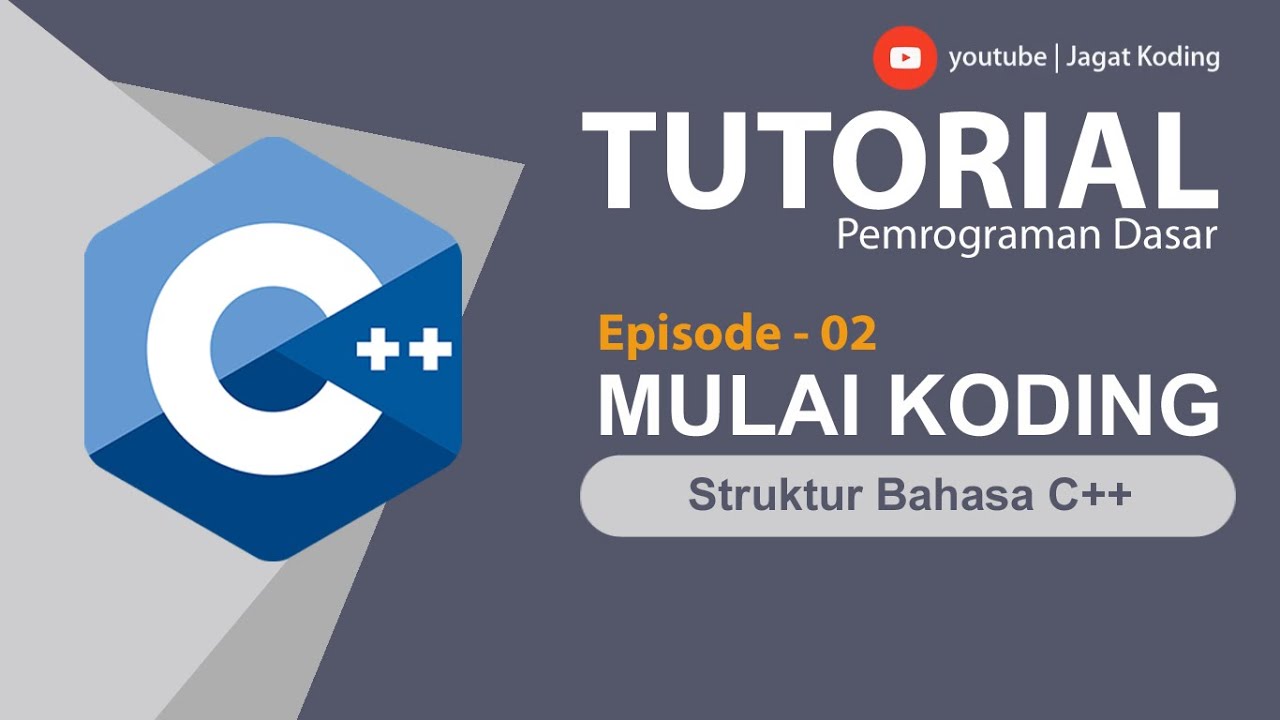
C++ 02 | Belajar Struktur Bahasa Pemrograman C++ | Tutorial Dev C++ Indonesia
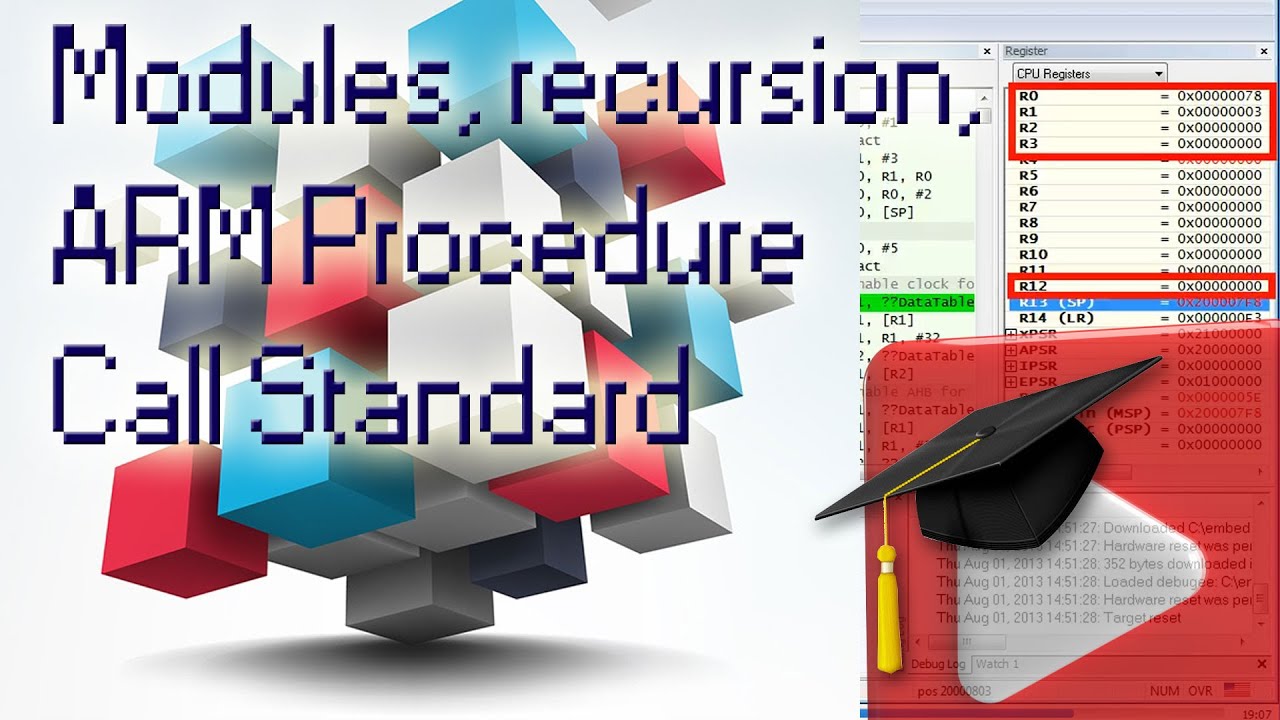
#9 Modules, Recursion, ARM Application Procedure Call Standard (AAPCS)
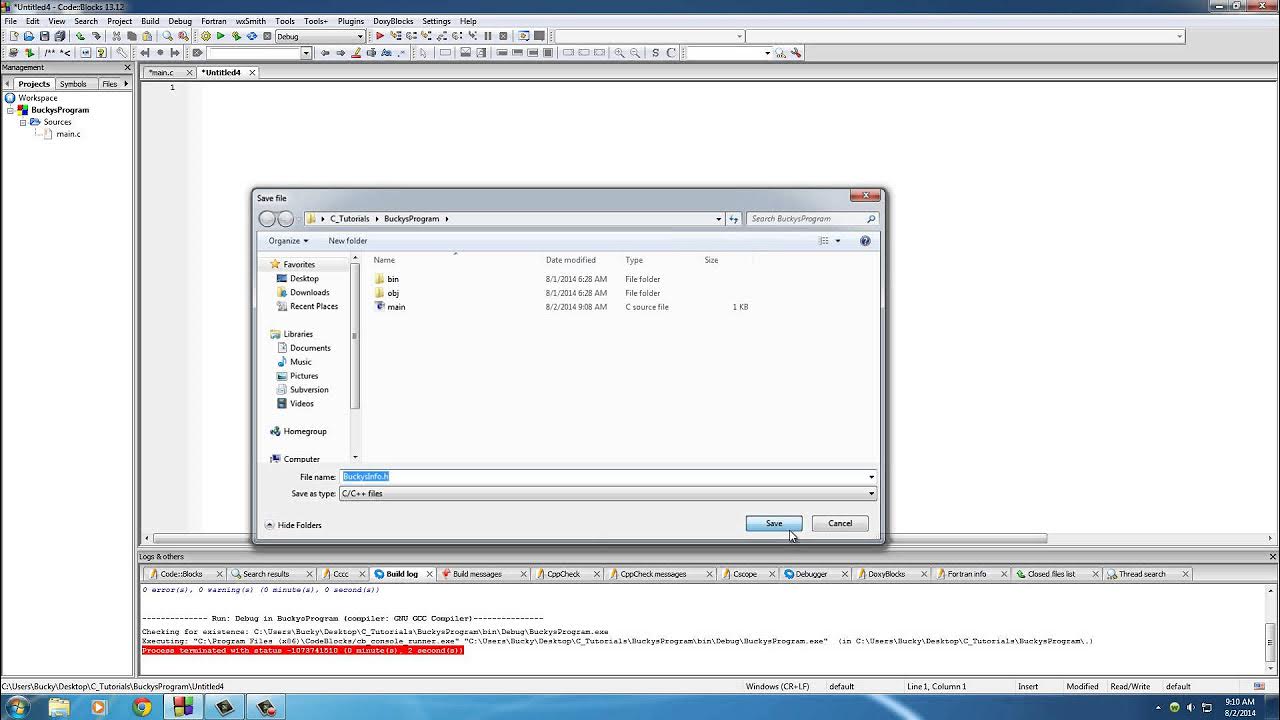
C Programming Tutorial - 10 - Creating a Header File

C Programming – Features & The First C Program
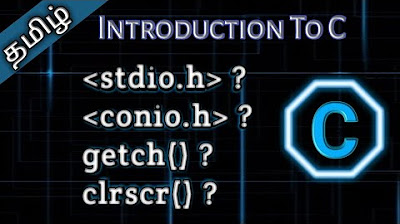
1. Introduction to C Language in Tamil || Tamil Pro Techniques ||
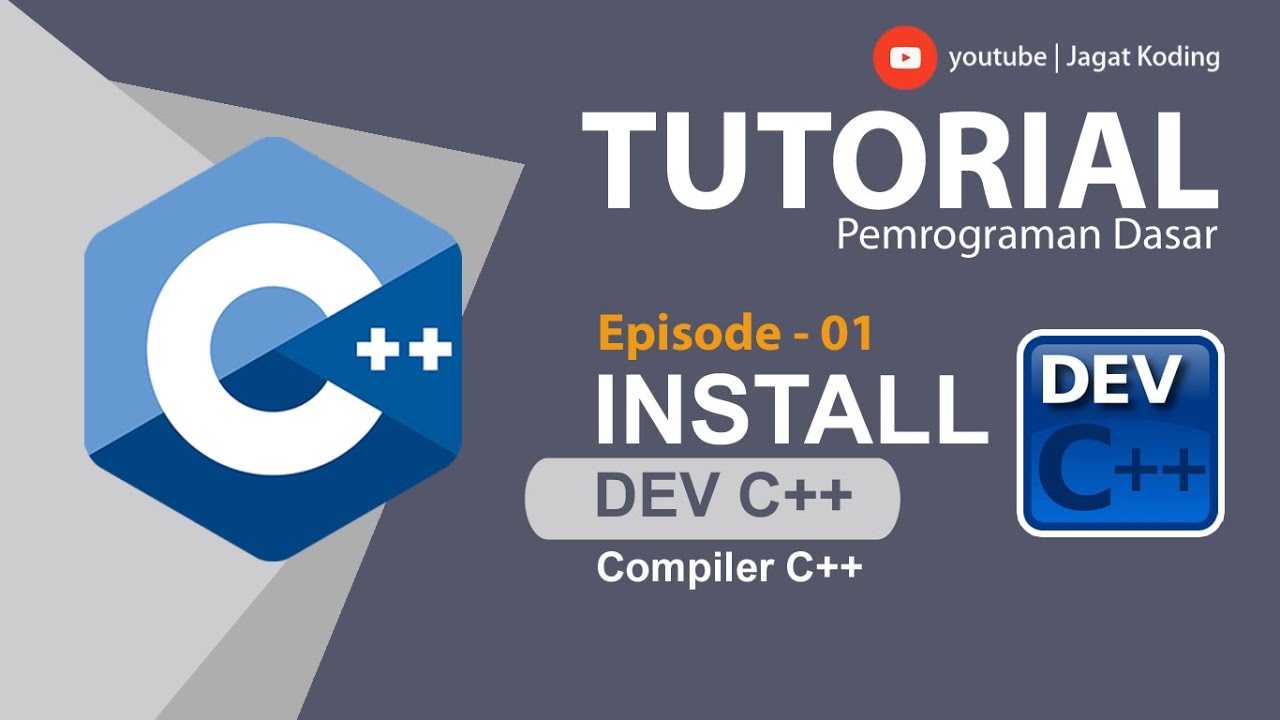
C++ 01 | Cara Instalasi Dev C++ | Tutorial Dev C++ Indonesia
5.0 / 5 (0 votes)