C Programming Tutorial - 10 - Creating a Header File
Summary
TLDRThis video tutorial introduces the concept of preprocessor directives in programming, focusing on 'include' and 'define'. The presenter explains how 'include' integrates external files like 'stdio.h' and 'stdlib.h' into the code, while 'define' creates constants with unchangeable values. The tutorial demonstrates creating a custom header file and using constants to calculate the age of girls one is allowed to date based on a humorous formula. The script concludes with a practical example, enhancing understanding of these fundamental programming concepts.
Takeaways
- ๐ The video discusses the concept of preprocessor directives in programming, which are lines at the top of a program that perform specific tasks before the program compiles.
- ๐ The '#include' directive is used to include the contents of another file, such as 'stdio.h' or 'stdlib.h', into the program, facilitating the use of standard input/output functions like 'printf'.
- ๐ ๏ธ The process of converting code into a format that a computer can understand is called 'compiling'.
- ๐ The '#include' directive can be used with angle brackets for searching in default locations or double quotes for searching in the same directory as the source file.
- ๐ The video introduces how to create a custom header file, which is a new file with a '.h' extension that can be included in the main program for reusability of code.
- ๐ The '#define' directive is used to define constants, which are like variables but with values that cannot be changed once set.
- ๐จ Constants are typically written in all uppercase letters to distinguish them from variables in the code.
- ๐ Preprocessor directives do not require a semicolon at the end, unlike regular C statements.
- ๐จโ๐ป The video demonstrates how to use a custom header file and constants in a program to calculate the age of girls the programmer is allowed to date, based on a simple formula.
- ๐ The presenter uses humor to engage the audience, suggesting they go find someone to date after learning the concept of constants and custom header files.
- ๐ The video emphasizes the importance of understanding preprocessor directives as a fundamental part of programming in C.
Q & A
What are preprocessor directives used for in a program?
-Preprocessor directives are used to give instructions to the preprocessor before the actual compilation of the program. They include files, define constants, and can perform other tasks before the code is translated into machine code.
What is the purpose of the '#include' directive?
-The '#include' directive is used to include the contents of one file into another. It allows for the use of standard libraries like stdio.h and stdlib.h, and can also include custom header files created by the programmer.
What does the '<stdio.h>' header file provide?
-'<stdio.h>' is a standard input/output header file that provides functions for input and output operations, such as printf for printing to the screen.
What is the difference between a variable and a constant in programming?
-A variable is a named storage location that can hold a value which can be changed throughout the program. A constant, on the other hand, is a value that cannot be changed once it is set.
Why should constants be written in all uppercase letters?
-Constants are written in all uppercase letters to distinguish them from variables. This is a convention that helps programmers quickly identify constants in the code.
What is the purpose of the '#define' directive?
-'#define' is a preprocessor directive used to define constants. It replaces occurrences of the constant's name with its value throughout the program before compilation.
Why is it important to understand the process of compiling a program?
-Understanding the compiling process is important because it clarifies how code is transformed from human-readable form into machine code. This knowledge helps in debugging and optimizing code performance.
What is the significance of creating a custom header file in a program?
-Creating a custom header file allows programmers to organize and reuse code effectively. It promotes modularity and can include constants, macro definitions, and function prototypes.
How does the script demonstrate the use of a custom header file?
-The script demonstrates by creating a new file named 'bies_info.h', defining constants within it, and then including this file in the main program using the '#include' directive.
What is the formula used in the script to calculate the age of girls someone is allowed to date?
-The formula used in the script is to take the person's age, divide it by two, and then add seven to find the minimum age of girls they are allowed to date.
What is the practical example given in the script to utilize the custom header file and constants?
-The practical example is a program that calculates the age of girls the programmer, named Bucky, is allowed to date based on his age using the formula provided and constants defined in the custom header file.
Outlines
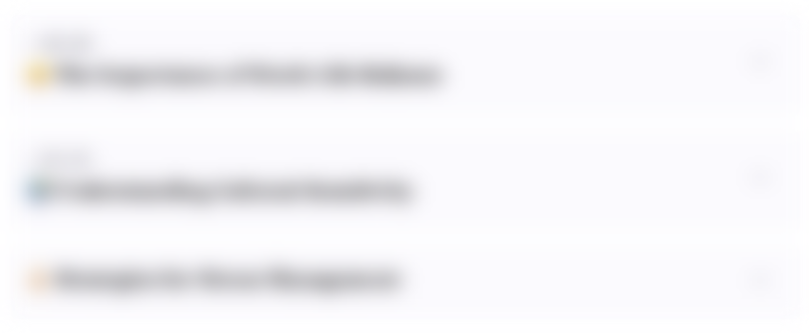
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
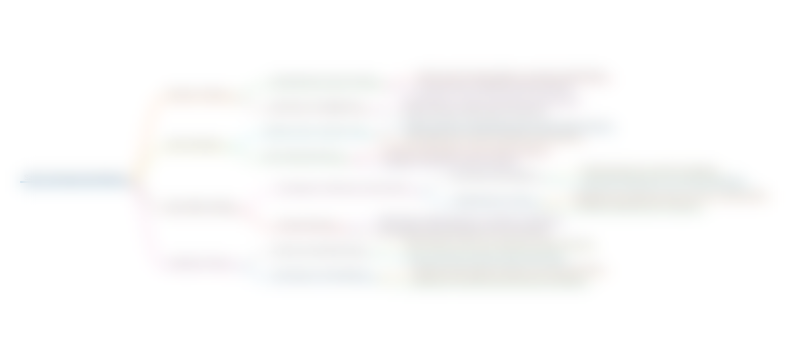
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
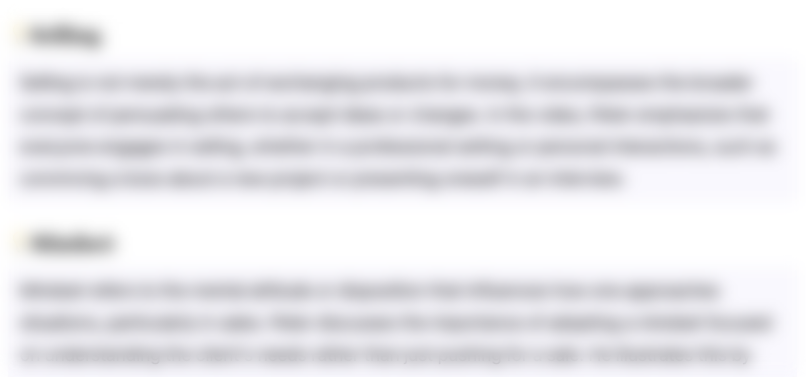
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
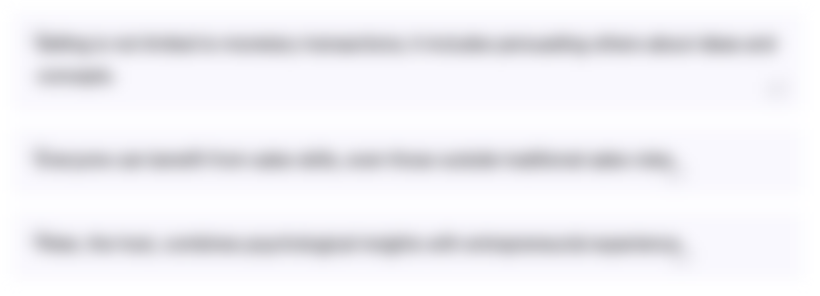
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
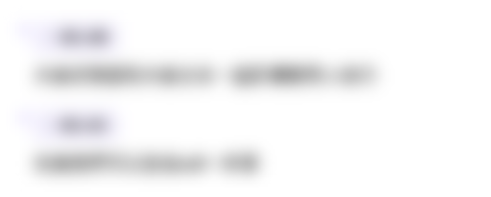
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)