G-19. Detect cycle in a directed graph using DFS | Java | C++
Summary
TLDRIn this video, we explore how to detect a cycle in a directed graph using the Depth-First Search (DFS) algorithm. The concept of a directed graph is explained, followed by a detailed walkthrough of how DFS is applied to identify cycles. We introduce the use of two visited arrays: one to track all visited nodes and another to track nodes in the current DFS path. Key points include backtracking, cycle detection, and handling directed edges. The video also covers the time and space complexity of the algorithm, with practical examples and a code walkthrough in both C++ and Java.
Takeaways
- 😀 Depth-First Search (DFS) is used to detect cycles in a directed graph.
- 😀 In a directed graph, a cycle exists when you can traverse the edges and return to the starting node.
- 😀 Undirected graphs allow cycle detection by revisiting a node, but directed graphs require careful path tracking.
- 😀 DFS in directed graphs requires two 'visited' arrays: one for marking all visited nodes and another for tracking nodes on the current DFS path.
- 😀 The 'visited' array tracks nodes that have been explored, while the 'path_visited' array ensures nodes are only revisited in the same path.
- 😀 A cycle is detected if a node that is already in the 'path_visited' array is revisited during DFS traversal.
- 😀 The algorithm is designed to check each node and its neighbors, backtracking once a path is fully explored.
- 😀 Backtracking in DFS resets the 'path_visited' status for nodes but leaves them marked in the 'visited' array.
- 😀 Once a cycle is found, further exploration of the graph is unnecessary, improving efficiency.
- 😀 The time complexity of DFS for cycle detection in directed graphs is O(V + E), where V is vertices and E is edges.
- 😀 Space complexity for this DFS approach is O(2V) due to the use of two arrays for visited and path-visited tracking.
Q & A
What is the difference between a directed and an undirected graph?
-In an undirected graph, edges have no direction, meaning the connection between nodes is bidirectional. In a directed graph, edges have a direction, meaning that the connection from one node to another is one-way.
Why can't we use the same cycle detection algorithm for undirected graphs in directed graphs?
-In undirected graphs, revisiting a node in the DFS traversal means a cycle has been found. However, in directed graphs, the direction of the edges must be considered. Re-entering a node doesn't necessarily mean a cycle unless it's part of the same DFS path.
What is the key modification in the DFS algorithm for detecting cycles in directed graphs?
-The key modification is the use of two 'visited' arrays: one to mark nodes that have been visited, and another ('path visited') to track nodes that are currently in the DFS path. A cycle is detected if a node is revisited while still in the current DFS path.
How do the two 'visited' arrays work in detecting cycles in a directed graph?
-The first 'visited' array tracks whether a node has been visited during any DFS traversal. The second, 'path visited' array tracks whether a node is part of the current DFS path. If a node is visited again while it's still marked as 'path visited', a cycle is detected.
What happens when a node has no adjacent nodes during the DFS traversal?
-If a node has no adjacent nodes, the DFS traversal for that node is considered complete. The algorithm then backtracks, marking the node as no longer part of the current DFS path.
Why do we backtrack when a node has no further adjacent nodes in DFS?
-Backtracking is necessary because it indicates that there are no more paths to explore from that node. Once backtracked, the node is removed from the 'path visited' array, allowing other paths to be explored.
How does the algorithm handle revisiting nodes in the DFS path?
-If a node is revisited while it is still in the DFS path (i.e., it is marked as 'path visited'), the algorithm detects a cycle and returns true. This means the node has been part of a cycle in the current traversal.
What is the time complexity of the cycle detection algorithm in a directed graph?
-The time complexity of the cycle detection algorithm using DFS is O(V + E), where V is the number of vertices (nodes) and E is the number of edges in the graph.
What is the space complexity of the cycle detection algorithm in a directed graph?
-The space complexity is O(2n), where n is the number of nodes in the graph, due to the two 'visited' arrays used to track the status of nodes.
What is the challenge presented in the video regarding the visited arrays?
-The challenge is to optimize the algorithm by reducing the two 'visited' arrays to just one. The hint is to use different values within a single array to represent both the 'visited' and 'path visited' states.
Outlines
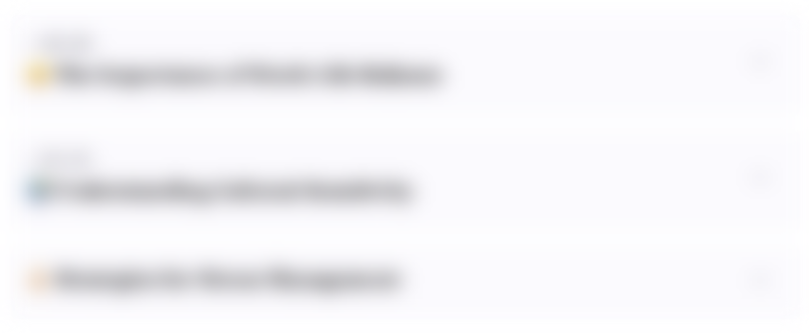
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
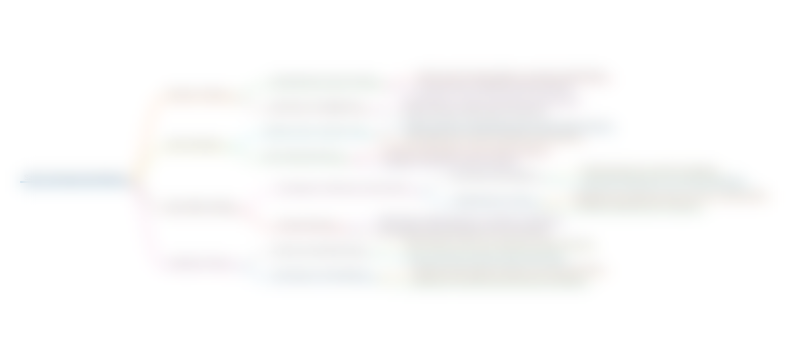
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
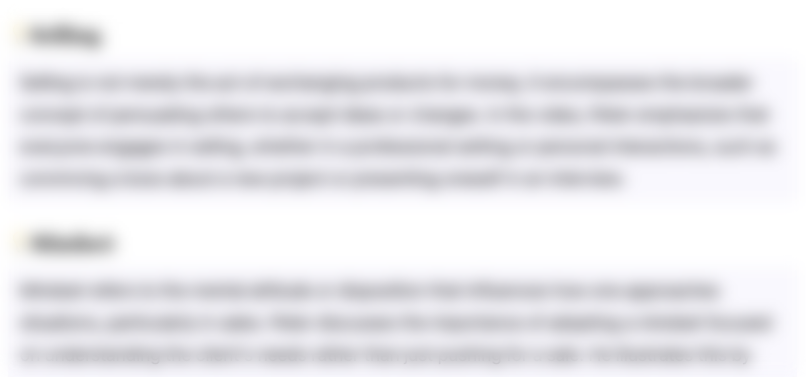
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
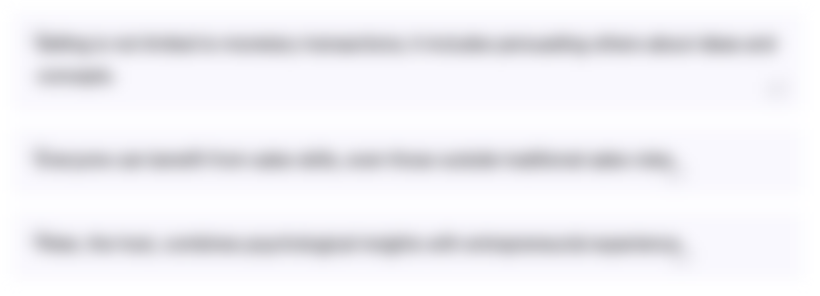
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
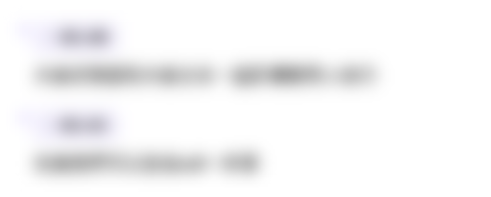
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraVer Más Videos Relacionados

Breadth First Search BFS
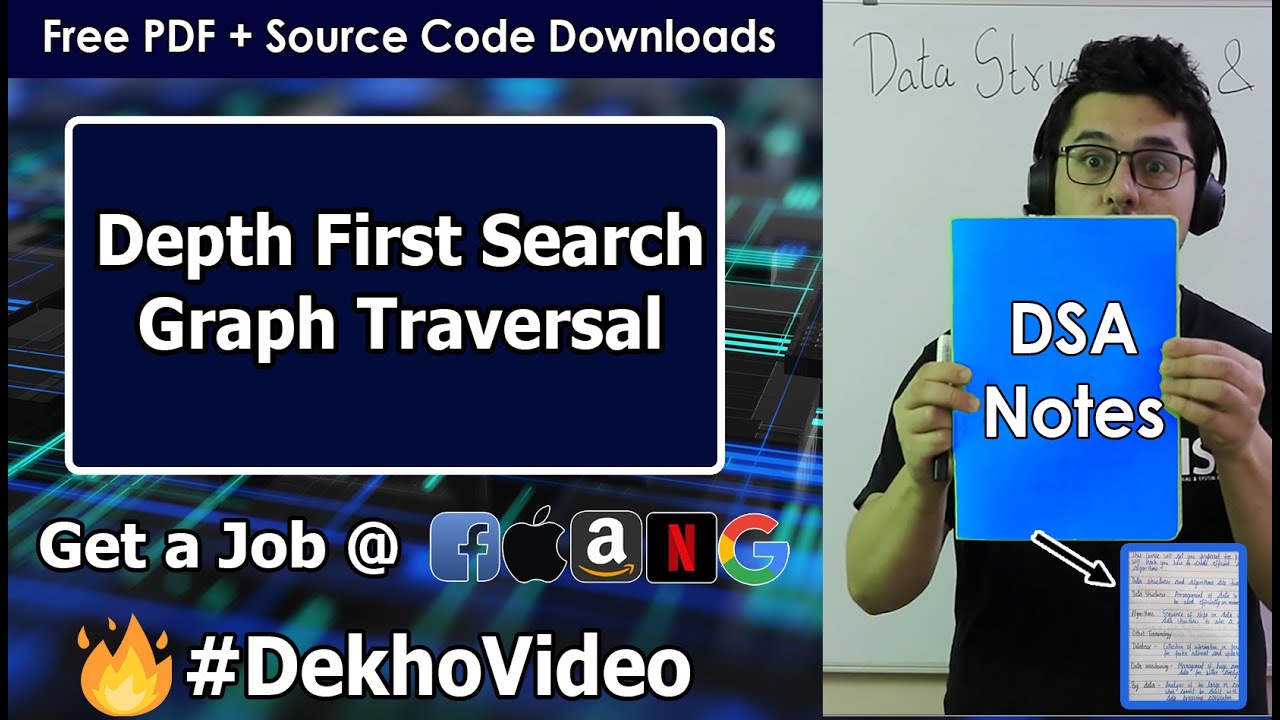
Depth First Search (DFS) Graph Traversal in Data Structures
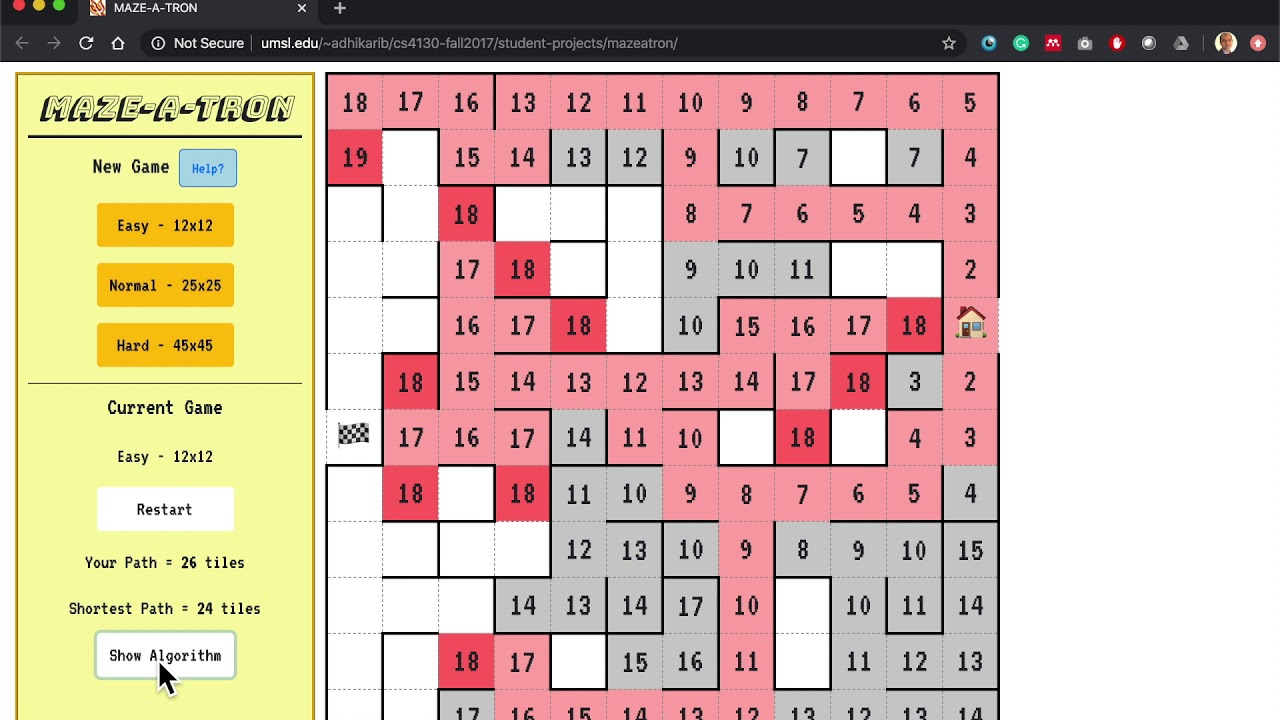
AI - Ch03 - Uninformed search algorithms
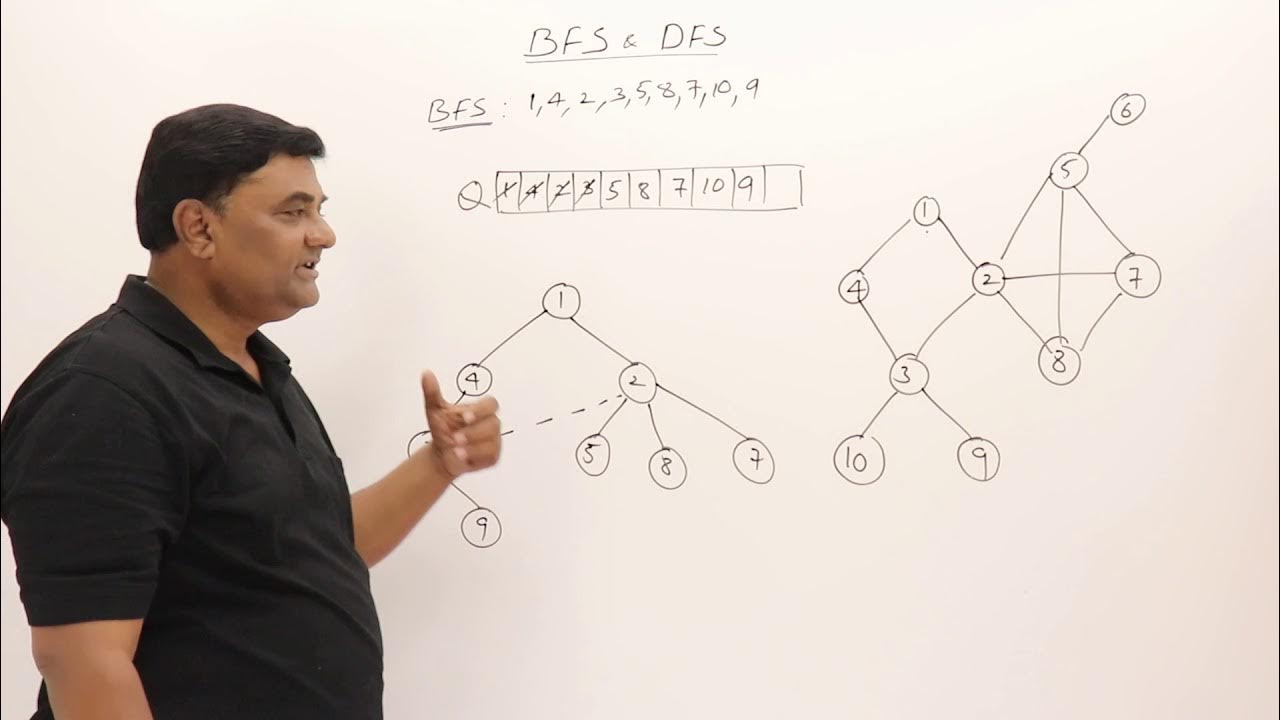
5.1 Graph Traversals - BFS & DFS -Breadth First Search and Depth First Search
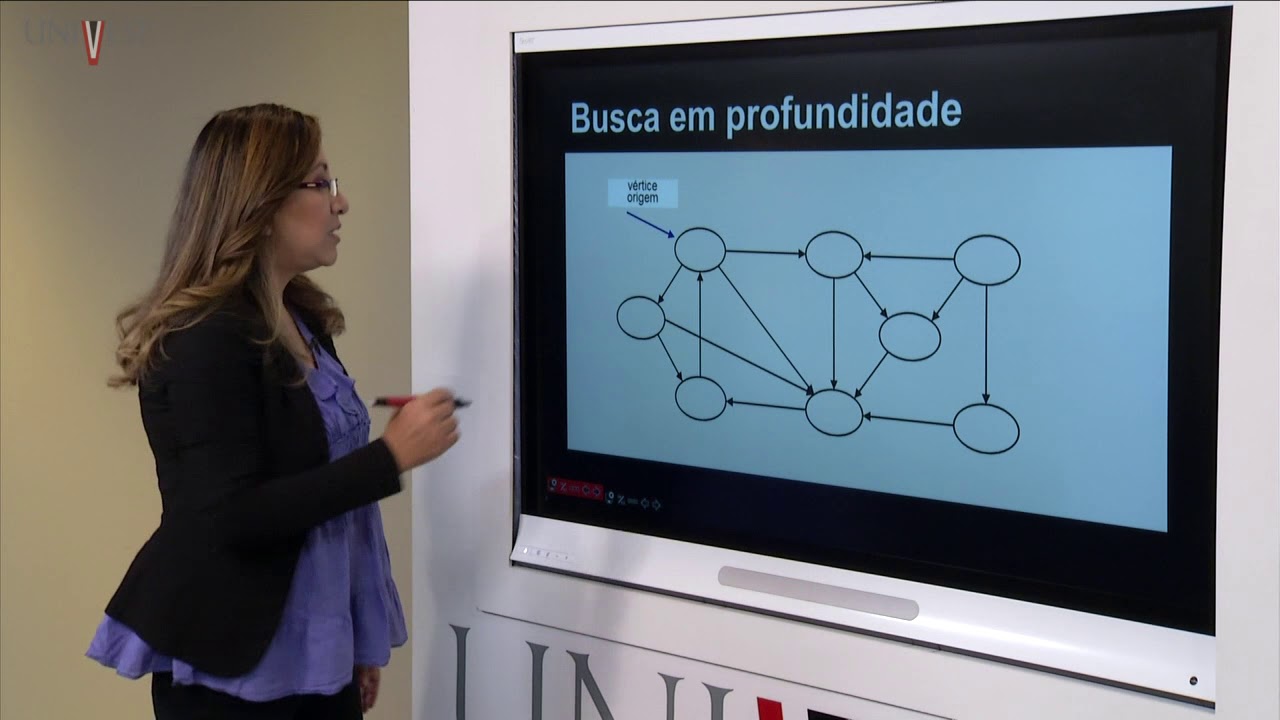
Projeto e Análise de Algoritmos - Aula 12 - Algoritmos de busca em largura e profundidade em grafos

DFS -Depth First Seach
5.0 / 5 (0 votes)