L6. Sieve of Eratosthenes | Maths Playlist
Summary
TLDRIn this video, the instructor explains an optimized algorithm for finding prime numbers up to a given number using the Sieve of Eratosthenes. The initial brute force method is discussed, followed by the introduction of the sieve algorithm, which significantly reduces time complexity by precomputing prime numbers. The video covers the step-by-step process, explains the logic behind eliminating non-prime numbers, and introduces optimizations to further enhance efficiency. Finally, the video provides insights into the time and space complexities of the algorithm, offering helpful code implementation tips.
Takeaways
- 🧮 The problem involves finding all prime numbers up to a given number, 'n'.
- ⚙️ The brute force method has a time complexity of O(n * √n), which is inefficient for large values of 'n'.
- ⏱️ The optimized solution uses the Sieve of Eratosthenes algorithm, which improves time complexity by precomputing prime numbers.
- 🔢 The key idea is that for any prime number, all its multiples are not prime and can be marked as non-prime.
- 🟢 The algorithm works by creating an array of size 'n+1', marking 1 for prime candidates and 0 for non-prime numbers.
- ✖️ Once a prime is identified, all its multiples are marked as non-prime starting from the prime squared.
- 🚀 The optimized time complexity of the algorithm is O(n log log n), thanks to precomputation and the efficient marking process.
- 📝 The sieve only needs to loop up to the square root of 'n', reducing unnecessary computations for larger values.
- 🧠 The space complexity is O(n) because of the additional array used to store the prime status of each number.
- 📈 This approach is highly efficient for large values of 'n', and the precomputation step is the key to optimizing prime number checking.
Q & A
What is the problem the speaker is trying to solve?
-The problem is to print all prime numbers up to a given number 'n'. For example, if n = 10, the prime numbers up to 10 are 2, 3, 5, and 7.
What is the brute force solution mentioned for finding prime numbers up to n?
-The brute force solution involves iterating through all numbers from 2 to n and checking each number to see if it's prime by dividing it by all numbers up to its square root. This method has a time complexity of O(n√n).
Why is the brute force solution inefficient for large values of n?
-The brute force solution is inefficient because for large values like n = 10^5 or 10^6, the time complexity grows significantly, leading to an enormous number of operations, making the algorithm slow.
What is the optimized approach introduced in the script?
-The optimized approach introduced is the Sieve of Eratosthenes, an algorithm that marks the multiples of each prime number as non-prime. This allows prime numbers to be determined in constant time after some initial precomputation.
How does the Sieve of Eratosthenes algorithm work?
-The algorithm works by creating an array where all numbers are initially marked as prime. Starting from the first prime (2), it marks all multiples of 2 as non-prime, then moves to the next number (3), marking all its multiples, and so on. This process continues until all numbers are either marked as prime or non-prime.
What is the time complexity of the Sieve of Eratosthenes?
-The time complexity of the Sieve of Eratosthenes is O(n log log n), which is much more efficient than the brute force method.
How does the Sieve of Eratosthenes avoid unnecessary computations?
-The algorithm avoids unnecessary computations by starting from the square of each prime number when marking multiples, as smaller multiples would have already been marked by earlier primes. Additionally, it only processes numbers up to the square root of n, as larger numbers are already covered.
What space complexity does the Sieve of Eratosthenes have?
-The space complexity of the Sieve of Eratosthenes is O(n), as it requires an array of size n to store whether each number is prime or not.
What optimizations are suggested for the Sieve of Eratosthenes?
-The two main optimizations are: 1) starting to mark multiples from the square of each prime number to avoid redundant work, and 2) stopping the outer loop once it reaches the square root of n, as no further marking is necessary beyond that point.
What is the role of precomputation in the Sieve of Eratosthenes?
-Precomputation plays a critical role in the Sieve of Eratosthenes by calculating which numbers are prime in advance. Once this precomputation is done, determining whether any given number is prime can be done in constant time, O(1).
Outlines
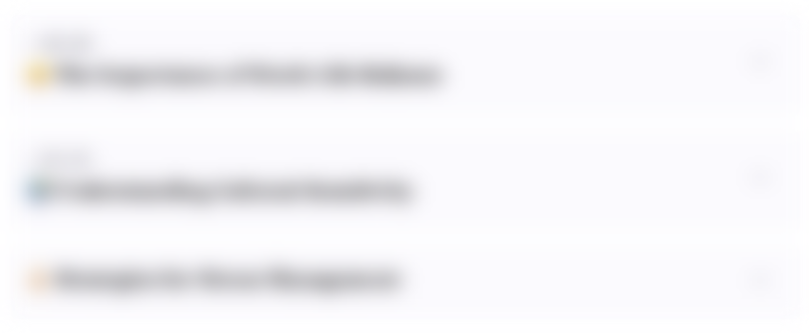
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
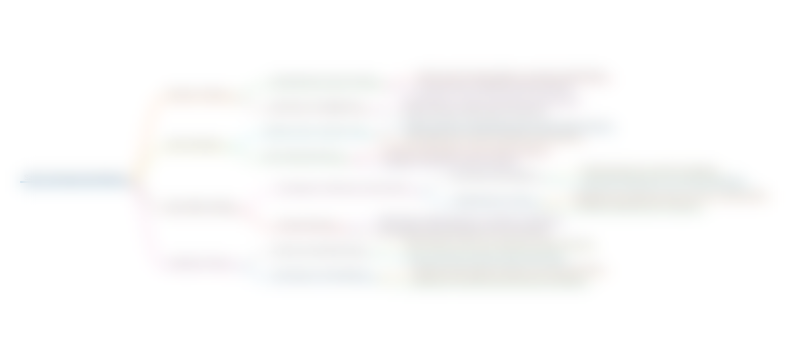
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
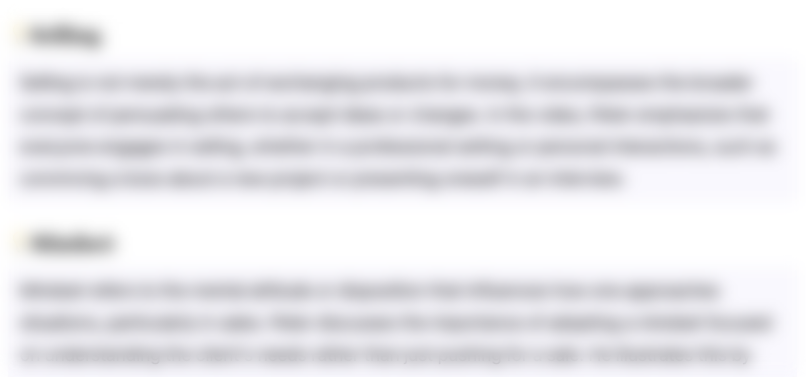
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
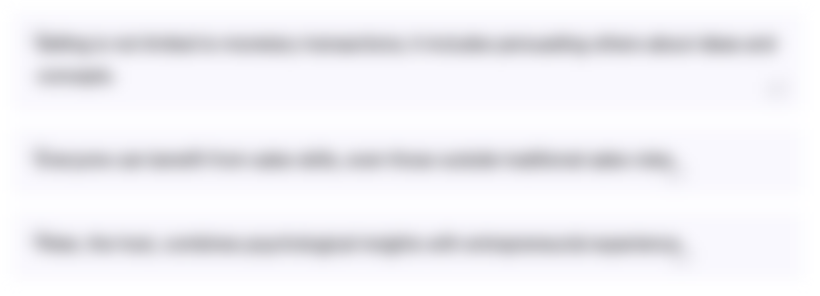
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
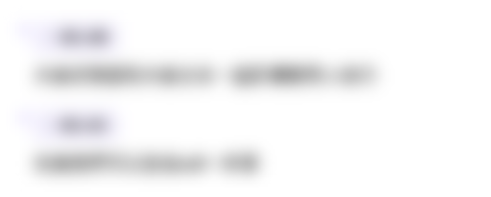
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraVer Más Videos Relacionados

TEORI BILANGAN BULAT-PART.1 (MATEMATIKA DISKRIT-PART.5)
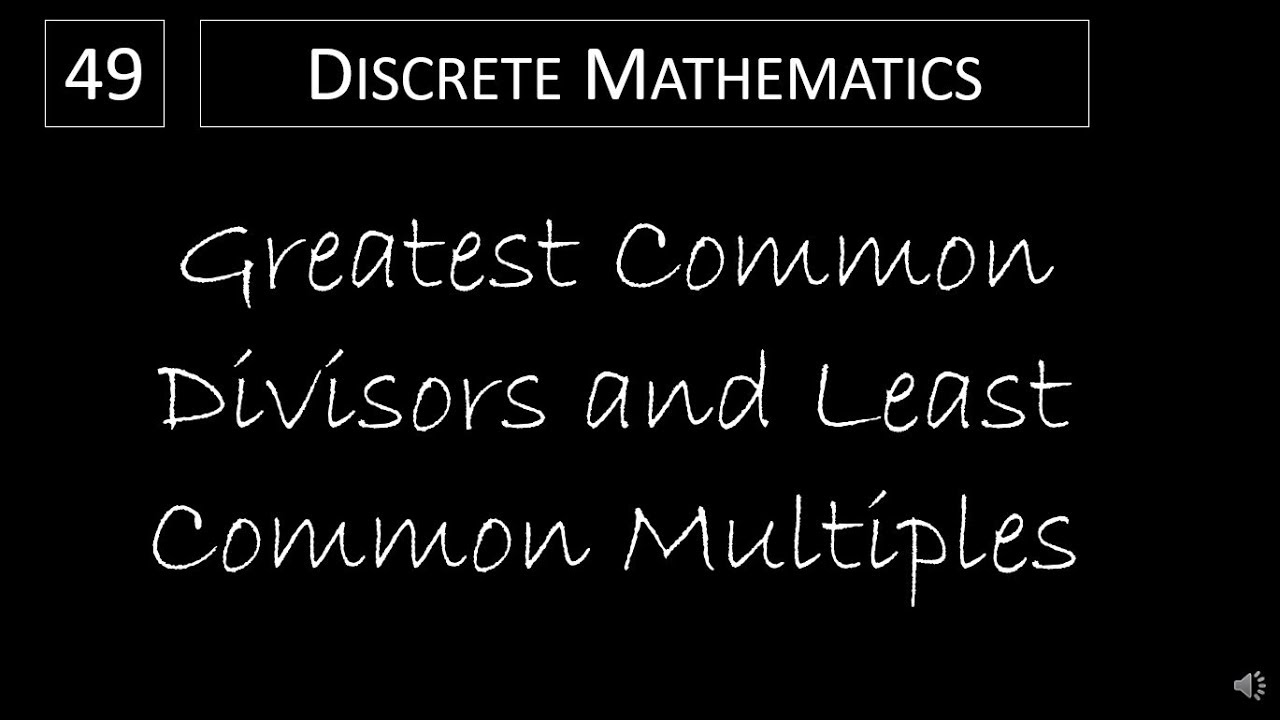
Discrete Math - 4.3.2 Greatest Common Divisors and Least Common Multiples

BAB 3 Menentukan Faktor Positif | Matematika Dasar | Alternatifa

Q 1-Ex 7.1-Cube and Cube Roots-NCERT Maths Class 8th-Chapter7
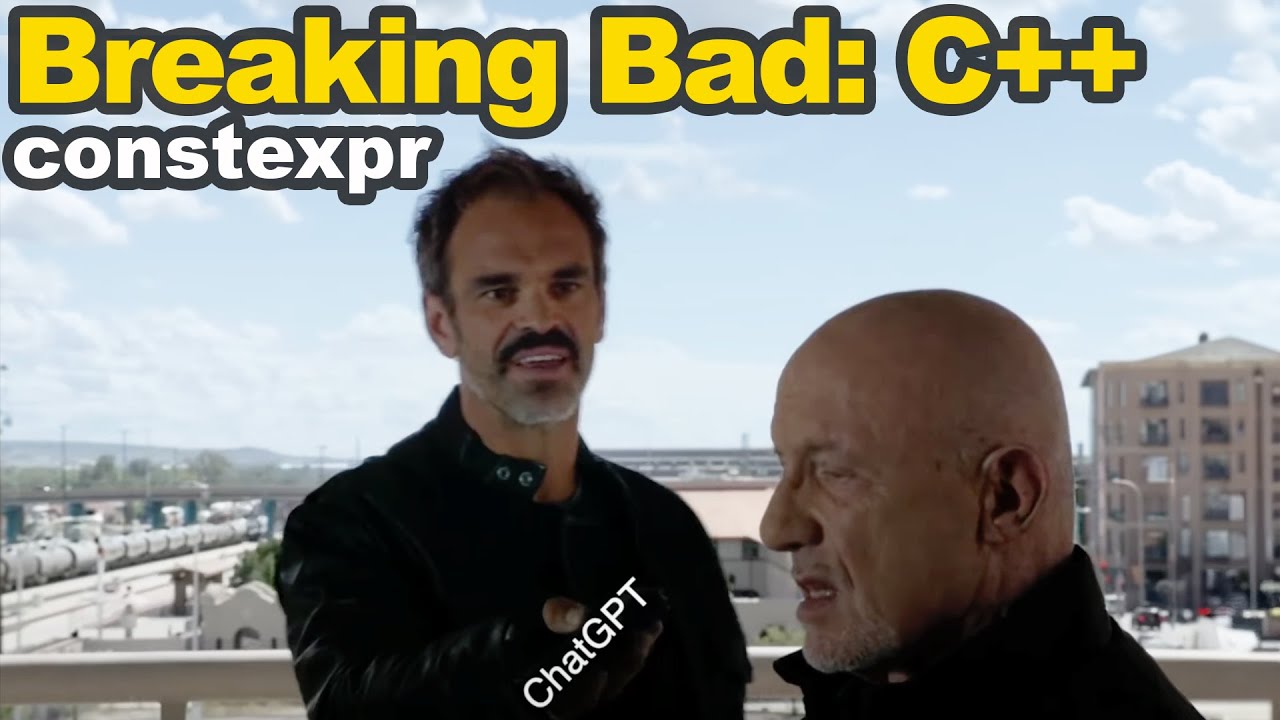
C++ Super Optimization: 1000X Faster
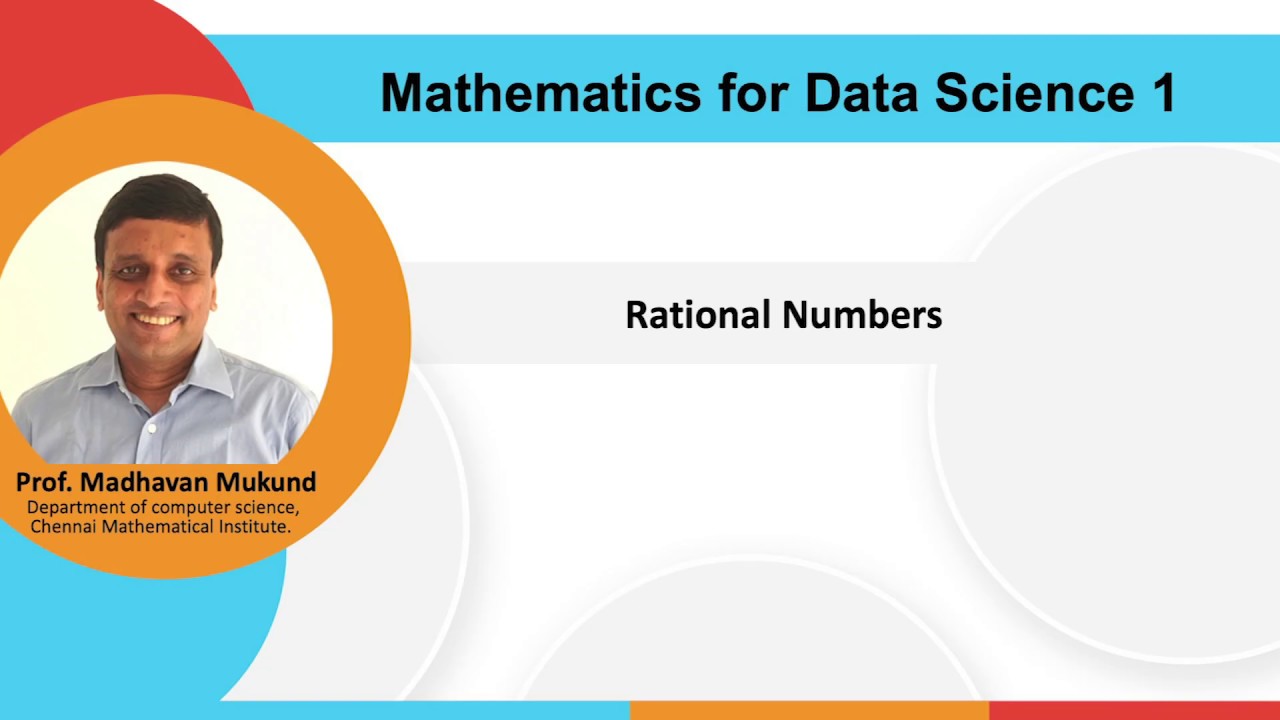
Lec 02 - Rational Numbers
5.0 / 5 (0 votes)