Javascript Design Patterns #1 - Factory Pattern
Summary
TLDRIn this video, the speaker introduces the Factory Design Pattern in JavaScript, explaining how it falls under creational design patterns. The Factory Pattern provides a flexible way to create various types of objects, centralizing object creation to improve code reusability and maintainability. Using a practical example, the speaker demonstrates how to implement a factory that creates different types of employees (developers, testers) based on user input. This pattern can be applied across different programming languages to streamline object creation and keep the codebase clean and organized.
Takeaways
- 💡 The Factory Design Pattern is a creational design pattern focused on object creation.
- 🛠️ Design patterns provide general solutions or templates for solving common software design problems.
- 🌍 The Factory pattern can be applied across different programming languages, not just JavaScript.
- 🏭 A factory in programming terms is an object that creates or manufactures other objects, simplifying object creation.
- 🎯 The Factory pattern centralizes object creation, promoting clean, reusable, and maintainable code.
- 🔄 Using the 'new' keyword multiple times can clutter the code, but the factory pattern helps streamline this process.
- 👨💻 In the example, developers and testers are two types of employees, and the factory pattern simplifies their creation.
- 📦 The 'employee factory' class is responsible for creating objects based on type, using a switch statement to differentiate employee roles.
- 🚀 The method 'employeeFactory.create()' allows for creating objects by passing employee names and types dynamically.
- 📝 This pattern allows for easy expansion, like adding new employee types (e.g., customer support) without modifying the core logic.
Q & A
What is a design pattern in programming?
-A design pattern is a general solution to common software design problems, similar to a blueprint or template that can be modified to solve specific problems. They are not specific to any programming language and can be applied across various languages.
What are the three main types of design patterns?
-The three main types of design patterns are creational patterns, structural patterns, and behavioral patterns.
What is the focus of creational design patterns?
-Creational design patterns focus on providing object creation mechanisms that promote flexibility and reusability of code, especially in situations where many different types of objects need to be created.
How does the factory design pattern work?
-The factory design pattern centralizes object creation, allowing for cleaner and more maintainable code. It provides a way to handle object creation in one place rather than having multiple 'new' object instances scattered across the code.
Why is centralizing object creation beneficial in the factory pattern?
-Centralizing object creation keeps code cleaner, more organized, and makes it easier to maintain, since all object creation is handled in one location rather than being scattered throughout the codebase.
What is the use case described for the factory pattern in the script?
-The script describes a scenario where a software business has different types of employees (developers and testers). The factory pattern is used to centralize the creation of these employee objects, ensuring a clean and efficient way to manage the process.
How is the factory pattern implemented in the example?
-In the example, an employee factory is created with a 'create' method that takes in a name and a type (1 for developers, 2 for testers). Based on the type, it returns a new developer or tester object.
How can additional types of employees be added using the factory pattern?
-To add more types of employees, you can simply extend the factory with additional cases in the switch statement, for example, by adding 'case 3' for customer support employees.
What advantages does the factory pattern offer when scaling to more employee types?
-The factory pattern makes it easy to scale, as adding new types of employees only requires adding a new case to the centralized factory method. This keeps the object creation logic clean and manageable.
Can the factory pattern be used in languages other than JavaScript?
-Yes, the factory pattern is not specific to JavaScript and can be applied to any programming language that supports object-oriented design.
Outlines
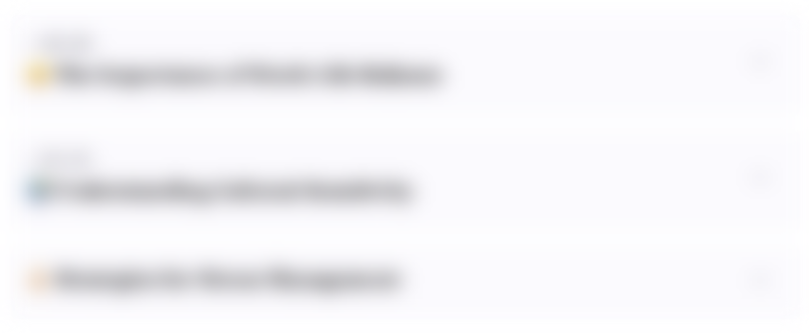
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
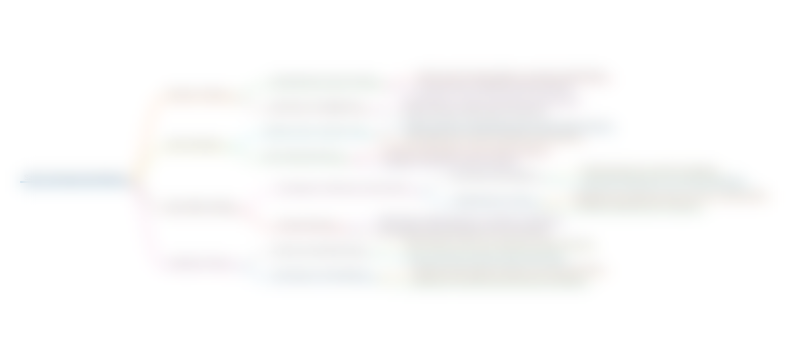
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
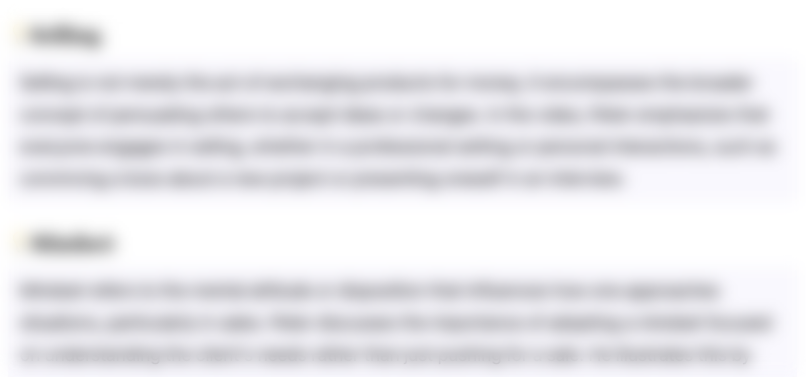
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
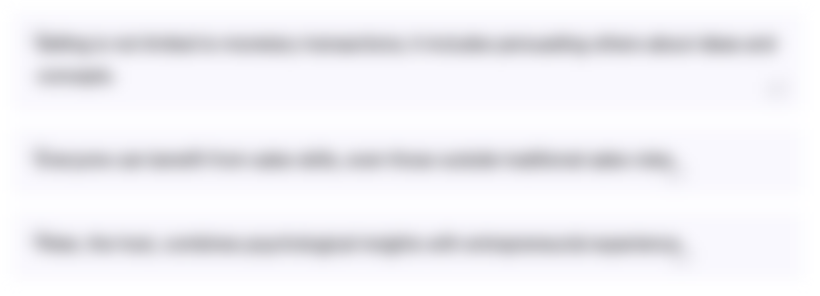
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
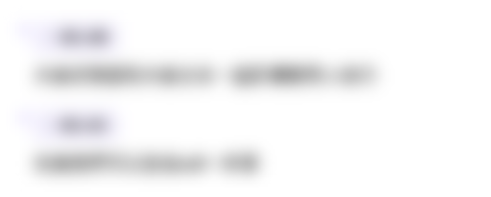
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraVer Más Videos Relacionados

Factory Pattern
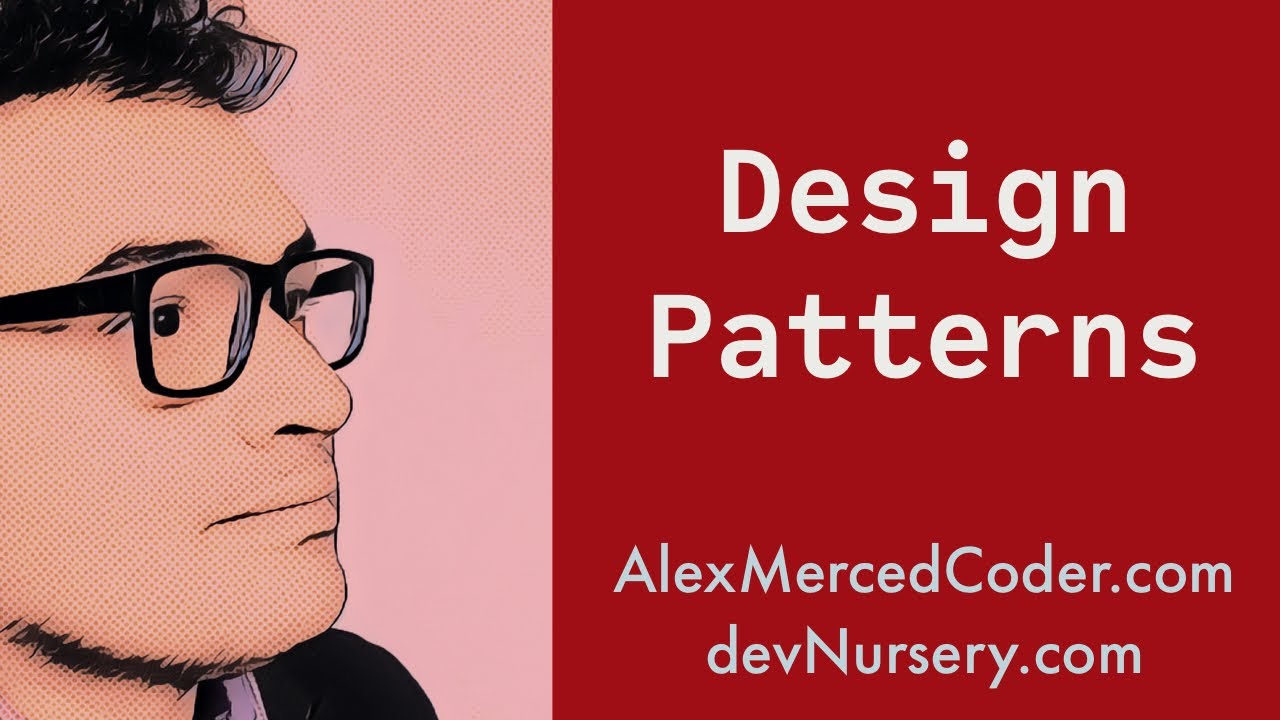
Programming Concepts - Design Patterns (Creational, Structural and Behavioral)
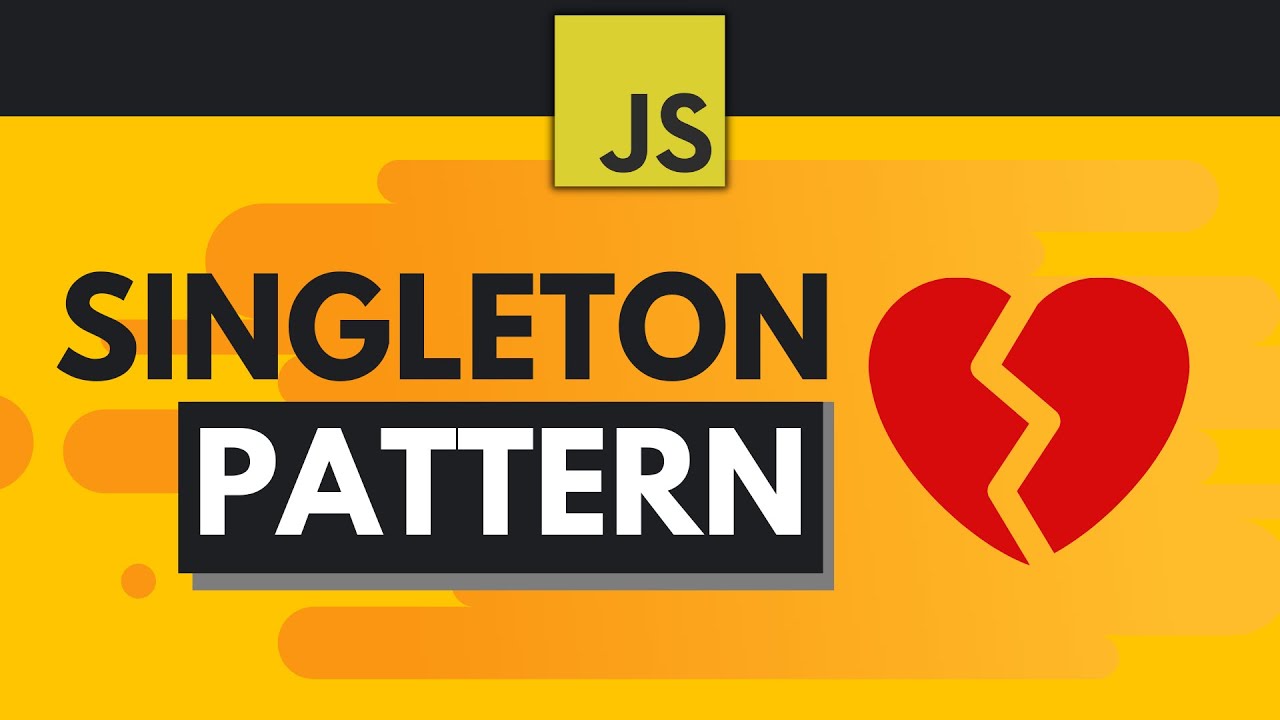
Javascript Design Patterns #2 - Singleton Pattern

Builder Design Pattern Explained in 10 Minutes
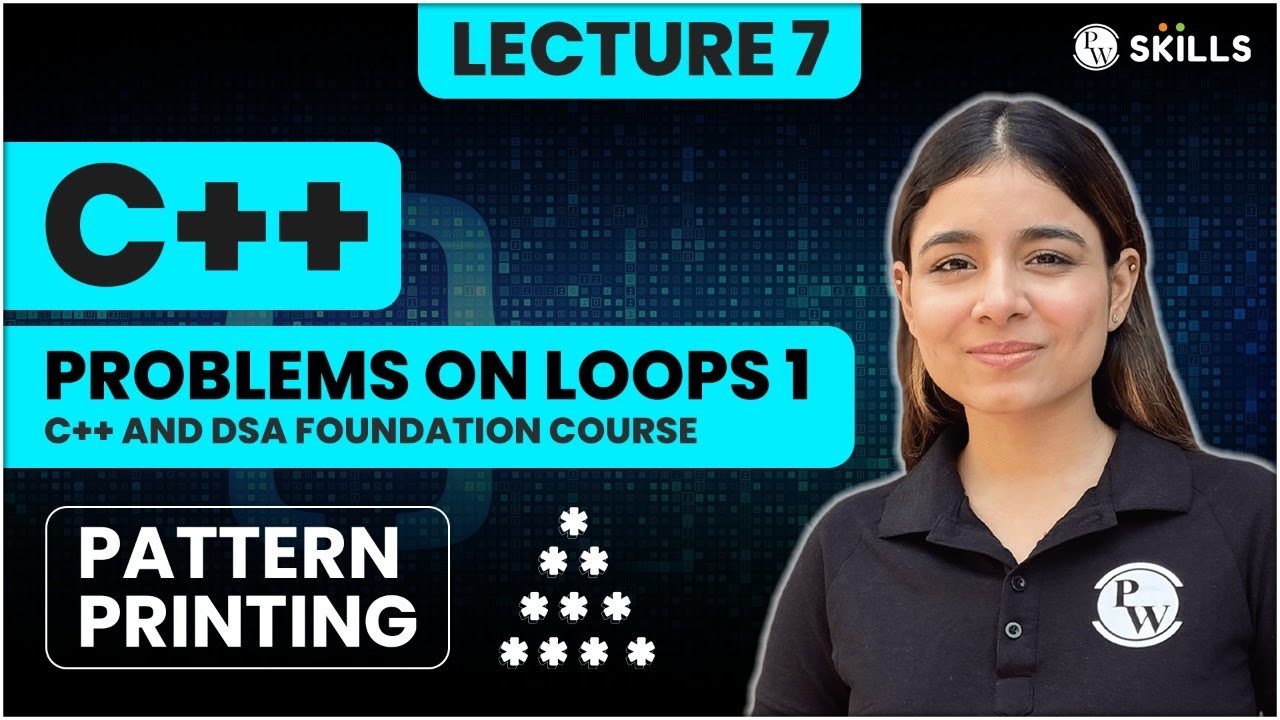
Pattern Printing | Problems on Loops - Part 1 | Lecture 7 | C++ and DSA Foundation Course

Start using this Go design pattern.. Consumer Interfaces!
5.0 / 5 (0 votes)