8 Design Patterns EVERY Developer Should Know
Summary
TLDRThis video delves into eight essential design patterns every developer should know, as outlined in the seminal book 'Design Patterns' by the Gang of Four. It covers creational patterns like Factory and Singleton, structural patterns such as Adapter and Facade, and behavioral patterns including Observer and Strategy. The script uses relatable examples, like ordering a burger or YouTube notifications, to explain these patterns, aiming to simplify complex programming concepts for better software design.
Takeaways
- 📚 The video discusses eight essential design patterns from the book 'Design Patterns: Elements of Reusable Object-Oriented Software' by the 'Gang of Four'.
- 🍔 The Factory pattern is likened to ordering a burger at a restaurant, where a factory class instantiates objects without exposing the instantiation logic to the client.
- 🏗️ The Builder pattern provides a step-by-step approach to object creation, allowing for more control over the construction process.
- 🔒 The Singleton pattern ensures a class has only one instance and provides a global point of access to it, useful for maintaining a single copy of application state.
- 📢 The Observer pattern, also known as the pub-sub pattern, allows multiple objects to listen for and respond to events, as exemplified by YouTube notifying subscribers of new uploads.
- 🔄 The Iterator pattern simplifies the way we traverse through elements of a collection without exposing its underlying representation.
- 🛠️ The Strategy pattern enables the dynamic selection of an algorithm from a family of algorithms, allowing behavior to be selected at runtime without modifying the class.
- 🔌 The Adapter pattern allows objects with incompatible interfaces to collaborate by converting the interface of one class into an interface expected by the clients.
- 🎭 The Facade pattern provides a simplified interface to a complex subsystem, hiding the complexities behind a single, unified interface.
- 📈 The video emphasizes the importance of design patterns in software development, highlighting their relevance even in the rapidly changing landscape of JavaScript frameworks.
- 📘 The presenter mentions a new course on object-oriented design patterns, including video lessons, written articles, and code examples in four programming languages.
Q & A
What are the three categories of design patterns introduced by the 'Gang of Four'?
-The three categories of design patterns are creational patterns, structural patterns, and behavioral patterns.
What is the purpose of the Factory design pattern?
-The Factory design pattern is used to create objects without exposing the instantiation logic to the client and refers to the newly created object using a common interface.
Can you explain the concept of the Builder pattern in the context of making a burger?
-The Builder pattern allows for the construction of complex objects step by step. It provides a way to create a complex object by adding one part at a time, which can be useful when the construction process is complex and requires setting a lot of parameters.
What is a Singleton pattern and how is it used in an application?
-The Singleton pattern ensures that a class has only one instance and provides a global point of access to it. It is used for maintaining a single copy of an application state, such as tracking whether a user is logged in.
How does the Observer pattern, also known as the pub-sub pattern, work in the context of YouTube?
-The Observer pattern allows multiple observers to listen for and respond to events. In the context of YouTube, the channel acts as the subject (publisher), and subscribers are the observers. When a new video is uploaded (event), all subscribers receive a notification.
What is the role of the Iterator pattern in programming?
-The Iterator pattern provides a way to access the elements of an object sequentially without exposing its underlying representation. It is used to traverse complex data structures in a simple and consistent manner.
Can you describe the Strategy pattern and its benefits?
-The Strategy pattern defines a family of algorithms, encapsulates each one, and makes them interchangeable. It allows the behavior of an object to be changed at runtime, following the open-closed principle, which states that software entities should be open for extension but closed for modification.
What is the Adapter pattern and how does it solve compatibility issues?
-The Adapter pattern is a structural pattern that allows objects with incompatible interfaces to work together by wrapping their own interface around that of an existing class. It can be used to make a micro USB cable compatible with a standard USB port by using an adapter.
What does the Facade pattern conceal and why is it used?
-The Facade pattern conceals the complex reality of a system's internal workings. It provides a simplified interface to a more complex subsystem, making the system easier to use and understand.
What is the significance of the book 'Design Patterns: Elements of Reusable Object-Oriented Software'?
-The book, authored by the 'Gang of Four,' is significant as it introduced 23 fundamental object-oriented design patterns, which are still widely discussed and used in software development, demonstrating their enduring value.
How does the script relate the design patterns to everyday life examples, such as ordering a burger?
-The script uses the example of ordering a burger to illustrate the Factory pattern, where you can order different types of burgers without worrying about the ingredients and assembly process, just like you would at a restaurant.
Outlines
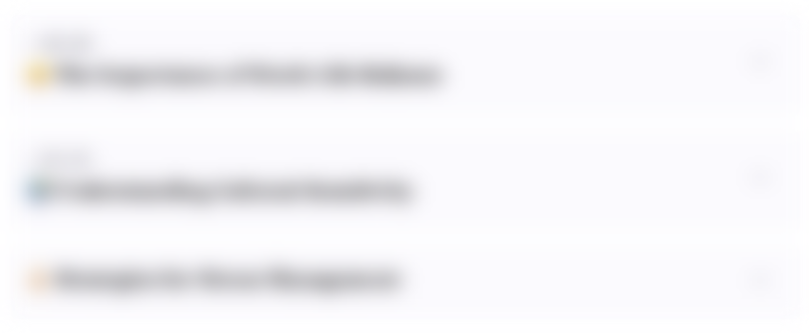
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
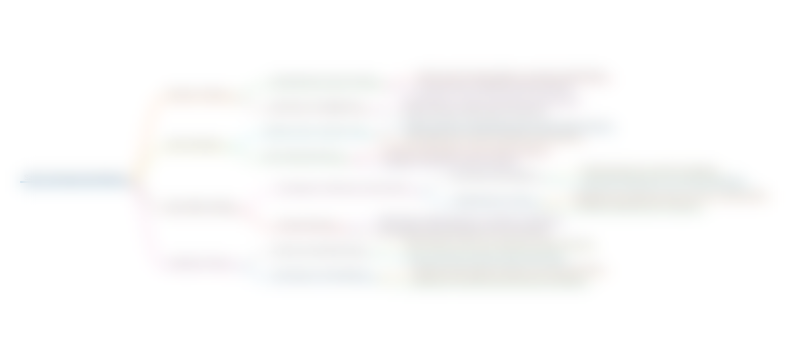
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
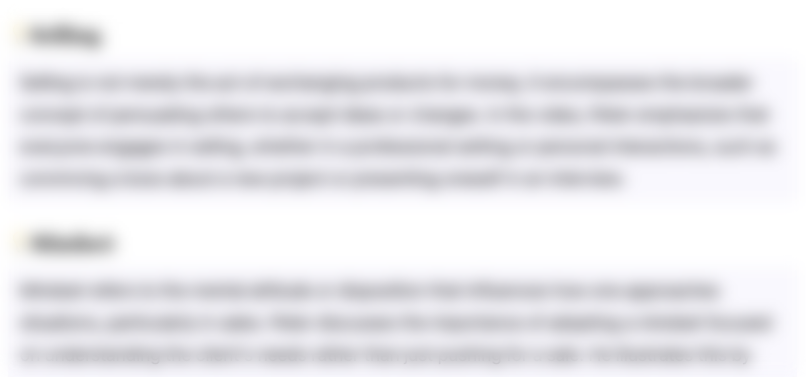
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
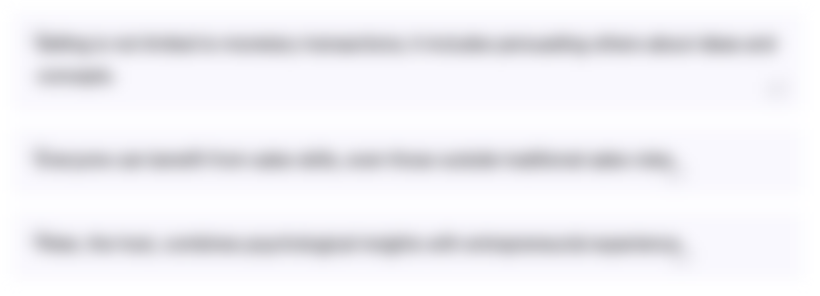
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
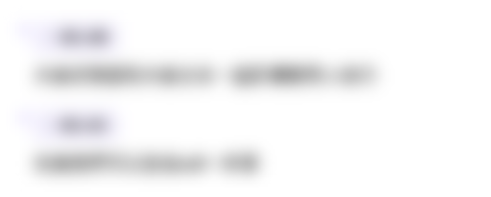
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)