Classes and Objects in Python | OOP in Python | Python for Beginners #lec85
Summary
TLDRThis Python programming tutorial focuses on Object-Oriented Programming (OOP), emphasizing the importance of classes and objects. It explains the drawbacks of procedural programming and how OOP simplifies complex projects. The video uses a YouTube channel analogy to illustrate classes as blueprints for creating objects. It discusses attributes and methods, differentiating them from variables and functions. The instructor clarifies that everything in Python is an object, and classes are necessary for defining custom data types. The video concludes with a practical demonstration of creating a class and objects, and assigns an exercise to create a 'CarDesign' class with two objects.
Takeaways
- 📚 Object-Oriented Programming (OOP) is preferred over the Procedure-Oriented Programming (POP) approach, especially for handling large real-world projects.
- 🏗 A class in Python serves as a blueprint for creating objects, which are instances of the class.
- 👤 Objects have attributes (data) and methods (functions) attached to them, distinguishing them from simple variables and functions.
- 🎥 The instructor uses a virtual YouTube channel example to explain how classes and objects model real-world entities.
- 💻 Everything in Python is an object, including basic data types like integers and strings.
- 📝 The class design is like a prototype, similar to an idli maker, which simplifies object creation just as an idli maker helps make idlis efficiently.
- 🚗 A class can create many objects, like a car blueprint that helps produce multiple cars efficiently.
- 🛠 The class keyword is used to define a class, and PascalCase is recommended for naming class names.
- 🆕 Objects are created by calling the class as a constructor, and the `pass` statement can be used as a placeholder when defining empty classes.
- 📦 Classes can be thought of as user-defined data types, and objects as instances of those types, helping to manage real-world complexity in programs.
Q & A
What is the main focus of the video script?
-The main focus of the video is to discuss object-oriented programming (OOP) in Python, particularly about classes and objects, how to create them, and why they are important.
What are the drawbacks of the procedure-oriented programming (POP) approach?
-Procedure-oriented programming (POP) struggles to model real-world problems and cannot efficiently handle large, complex projects. This is why OOP is used in industries for large-scale software development.
What analogy does the speaker use to explain the concept of a class?
-The speaker uses an 'idli maker' analogy, where the idli maker is the class (blueprint) and the idlis produced are the objects (instances). This analogy helps explain how a class is like a design for creating objects.
What is the purpose of a class in OOP?
-A class in OOP serves as a blueprint or design for creating objects. It allows developers to define attributes and methods, making it easier to create and manage multiple objects with similar properties and behaviors.
How does the speaker differentiate between attributes and methods in OOP?
-Attributes represent the information or data associated with an object (e.g., name, address), while methods represent the actions or functions an object can perform (e.g., teaching, preparing a quiz). Attributes are attached to objects, and methods are the actions the object can perform.
Why is everything in Python considered an object?
-In Python, everything is considered an object because all data types, such as integers, strings, and even functions, are instances of inbuilt classes (e.g., int, str). This object-oriented structure allows for consistent handling of data and operations.
Why do we need classes if objects are important in Python?
-Classes are needed because they provide a structured way to define the properties and behaviors of objects. Without classes, managing multiple objects and their attributes or behaviors would be chaotic and inefficient.
What is the syntax for creating a class in Python?
-To create a class in Python, you use the `class` keyword followed by the class name in Pascal case (e.g., `ClassName`) and a colon. Inside the class, you can define attributes and methods.
What is the difference between free-floating variables/functions and attributes/methods?
-Free-floating variables and functions are not associated with any object, while attributes and methods are tied to a specific object. In OOP, attributes hold data specific to an object, and methods define the object's behavior.
What assignment is given at the end of the video?
-The assignment is to create a class called `CarDesign` and then create two objects of that class. The task is to practice defining and using classes and objects in Python.
Outlines
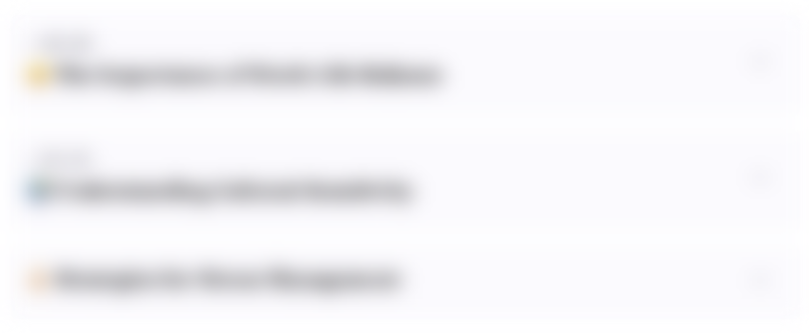
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
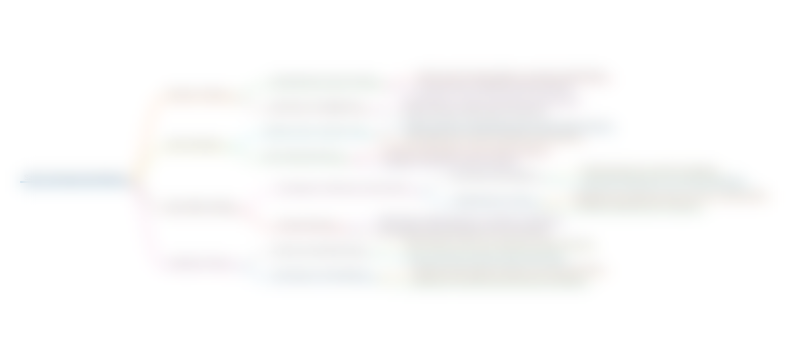
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
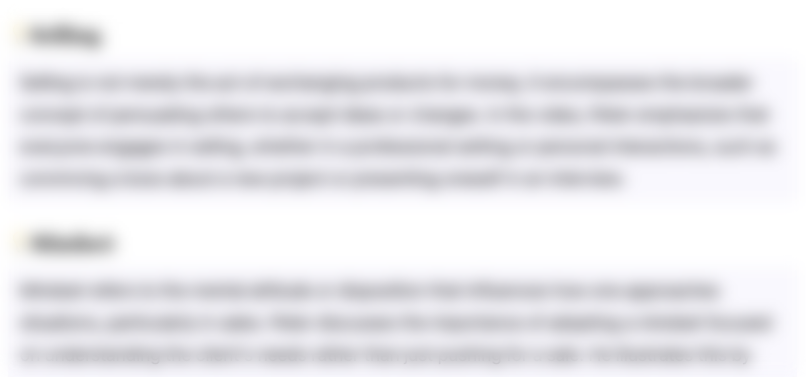
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
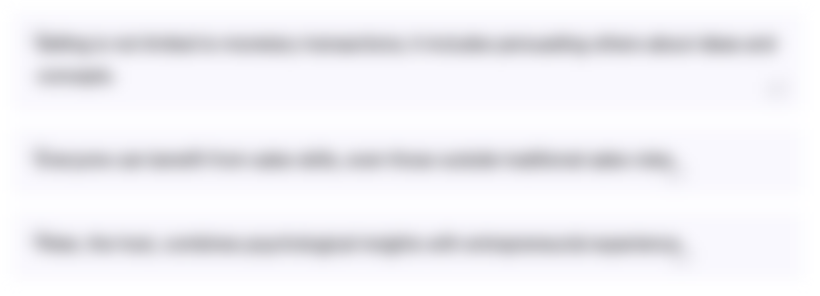
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
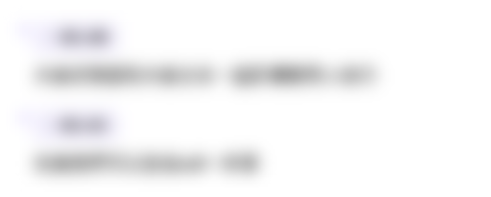
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraVer Más Videos Relacionados
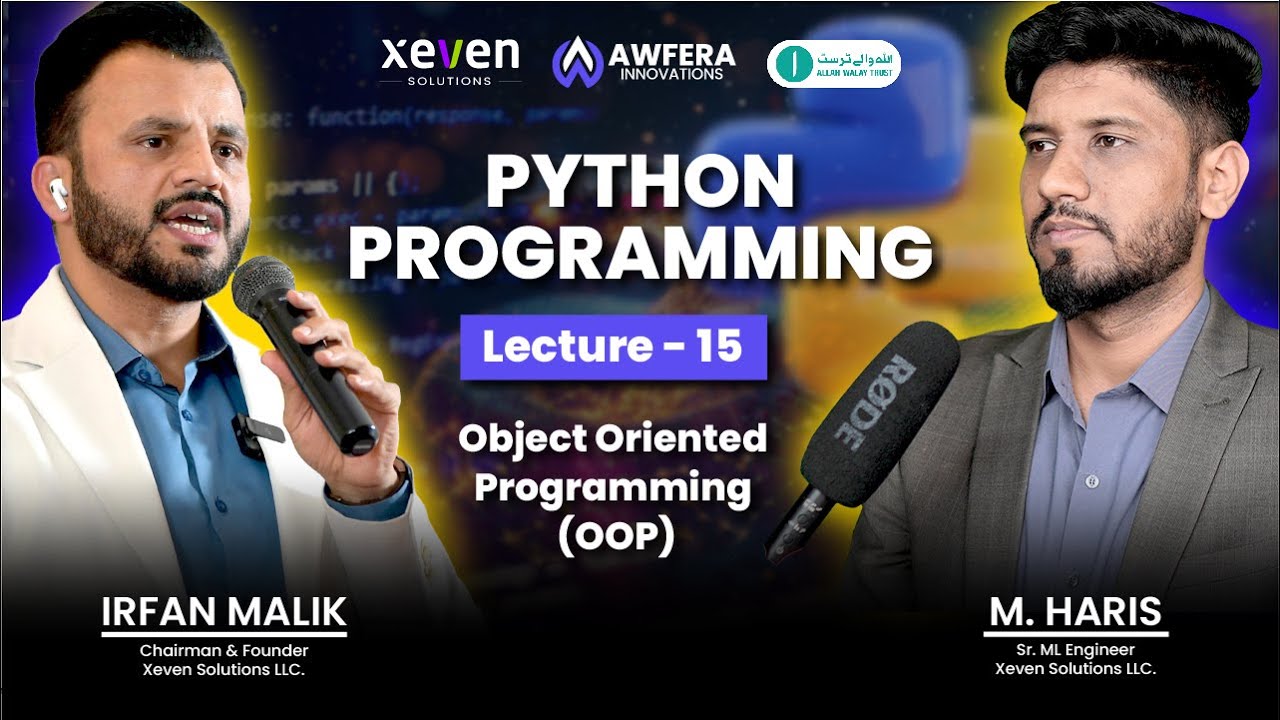
Lecture15: Object Oriented Programming (OOP)
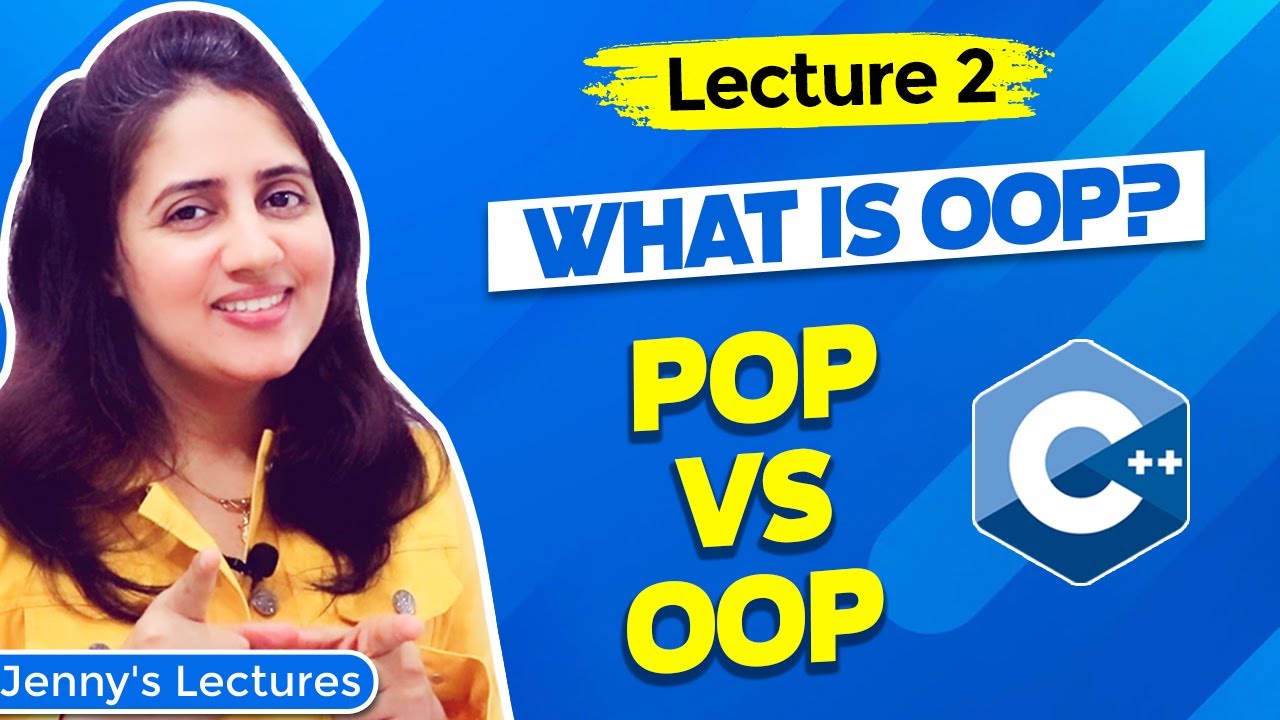
Lec 2: What is Object Oriented Programming (OOP) | POP vs OOP | C++ Tutorials for Beginners
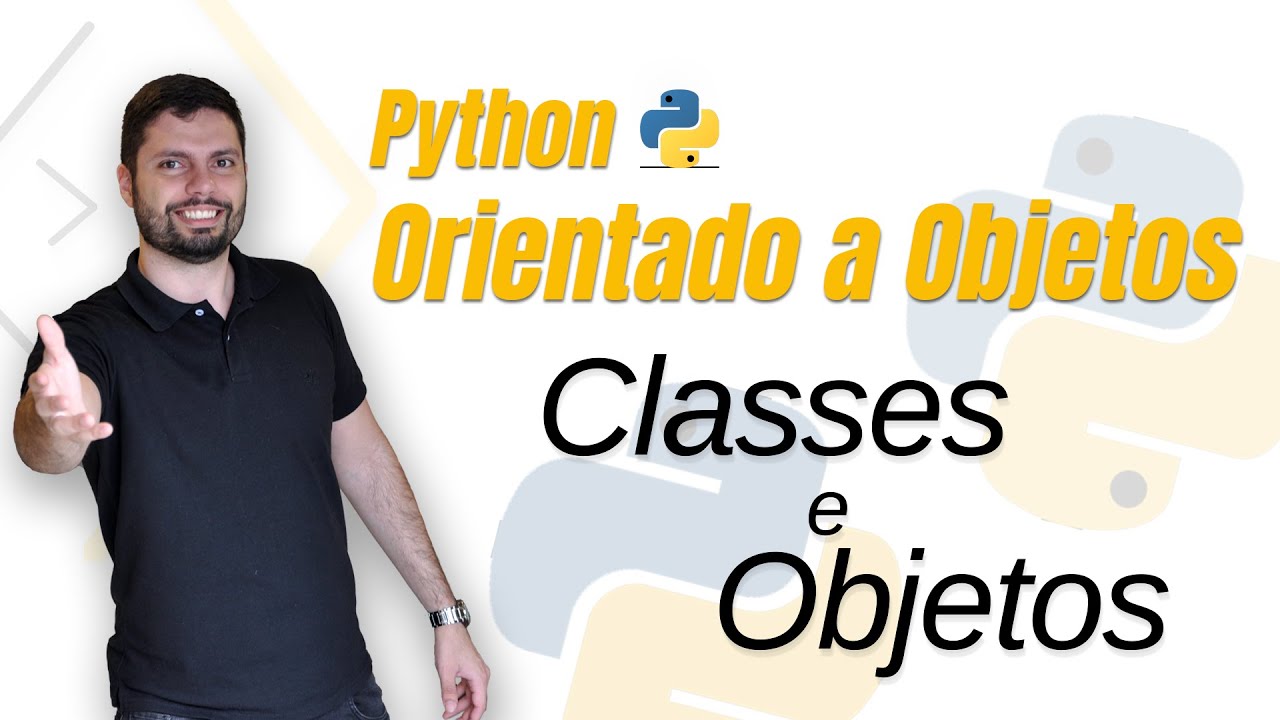
Como criar Classes e Objetos - Curso Python Orientado a Objetos [Passo a Passo]

AP CS A - 2.1 Instances of Classes
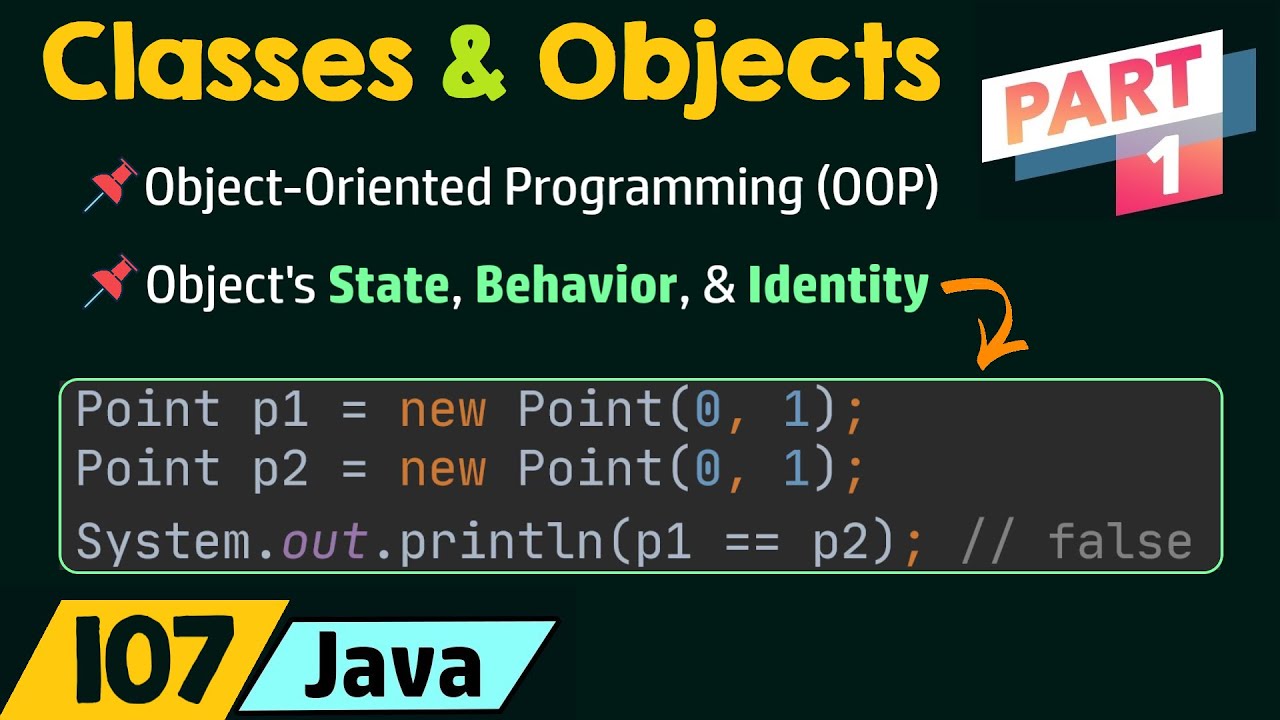
Introduction to Classes and Objects (Part 1)
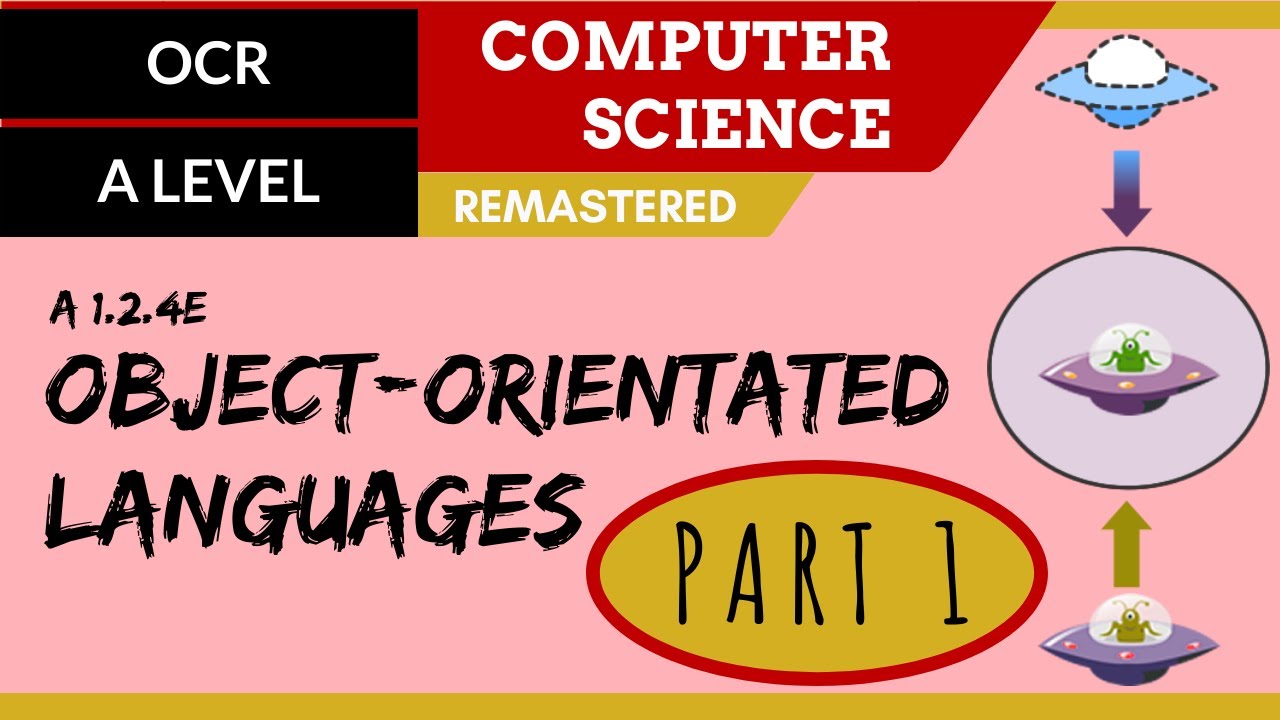
36. OCR A Level (H446) SLR7 - 1.2 Object-oriented languages part 1
5.0 / 5 (0 votes)