Como criar Classes e Objetos - Curso Python Orientado a Objetos [Passo a Passo]
Summary
TLDRThis tutorial introduces Object-Oriented Programming (OOP) in Python, focusing on the creation of classes and objects. Using the example of countries, the video demonstrates how to define a class, instantiate objects, and assign attributes such as name, capital, and population. The instructor explains the importance of OOP for organizing code, ensuring data consistency, and reusing code efficiently. Viewers are encouraged to practice by creating their own class for automobiles. The video emphasizes the power of constructors, encapsulation, and control over object data, making it a valuable learning resource for aspiring programmers.
Takeaways
- 😀 OOP is a programming paradigm that helps model real-world objects and behaviors using code, making it easier to manage complex software systems.
- 😀 Classes serve as blueprints for creating objects, defining the attributes (data) and methods (functions) that objects will have.
- 😀 Objects are instances of classes, where each object holds its own data according to the class's structure.
- 😀 Understanding and applying OOP principles is crucial for solving real-world problems with higher quality and efficiency in programming.
- 😀 The class constructor (`__init__` in Python) is used to initialize an object's attributes when it is created, ensuring consistency across instances.
- 😀 You can define mandatory parameters in a constructor to enforce a specific structure for every object created from a class.
- 😀 The importance of encapsulation in OOP is highlighted, allowing better control over data and how it is accessed or modified.
- 😀 OOP promotes code reusability, helping developers avoid redundant code by allowing classes to be reused and extended.
- 😀 Even though attributes in classes are typically defined in the constructor, you can also add additional attributes to objects later as needed.
- 😀 Classes can represent both concrete (e.g., cars) and abstract concepts (e.g., climate), making OOP versatile for various types of applications.
- 😀 Python allows you to create your own classes with custom attributes and methods, empowering you to model real-world entities or abstract concepts as needed.
Q & A
What is Object-Oriented Programming (OOP)?
-Object-Oriented Programming (OOP) is a programming paradigm that represents real-world objects through code. It uses classes as blueprints to define attributes and behaviors, and objects are instances of these classes.
What are the two main concepts in Object-Oriented Programming?
-The two main concepts in OOP are classes and objects. A class is a blueprint that defines attributes and behaviors, while an object is an instance of a class.
How does a class differ from an object in OOP?
-A class is a template or blueprint for creating objects, defining their attributes and behaviors. An object, on the other hand, is an instance of a class, containing specific values for those attributes.
What is the role of the 'self' parameter in the constructor method?
-'self' refers to the instance of the class itself. It is used in the constructor method to refer to the specific object being created, allowing you to assign values to its attributes.
What is a constructor in Python classes?
-In Python, a constructor is defined by the `__init__` method. It initializes the attributes of an object when it is created and can take parameters to assign values to those attributes.
Can you give an example of creating a class and object in Python?
-Sure! Here's an example: ```python class Country: def __init__(self, name, capital, population): self.name = name self.capital = capital self.population = population brazil = Country('Brazil', 'BrasÃlia', 212600000) print(brazil.name) # Output: Brazil```
Why is it beneficial to use OOP for programming?
-OOP helps organize code, making it more modular and reusable. It allows for better data management, encapsulation, and control over how attributes and methods are accessed, resulting in more maintainable and scalable code.
What is encapsulation in OOP?
-Encapsulation is the concept of restricting access to the internal details of an object and only exposing necessary parts through methods or functions. This helps ensure that the object's data is protected from unintended modifications.
What is the difference between class attributes and instance attributes in Python?
-Instance attributes are unique to each object created from the class, whereas class attributes are shared by all instances of the class. Class attributes are typically used for data that is common to all objects of the class.
How can a constructor be used to validate input data in OOP?
-A constructor can validate input data by checking the values passed to it and ensuring they meet certain conditions before assigning them to the object’s attributes. For example, you could check that a population value is not negative before assigning it to the object.
Outlines
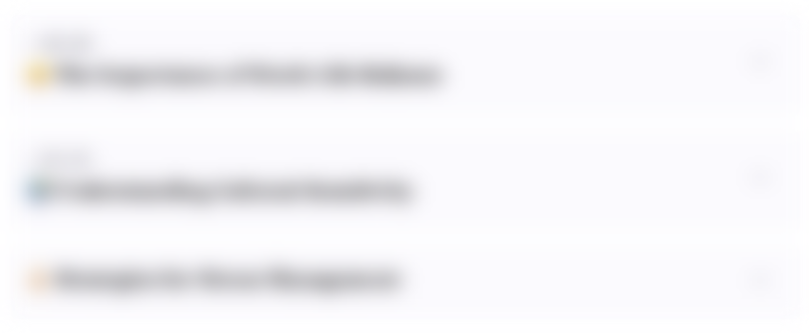
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
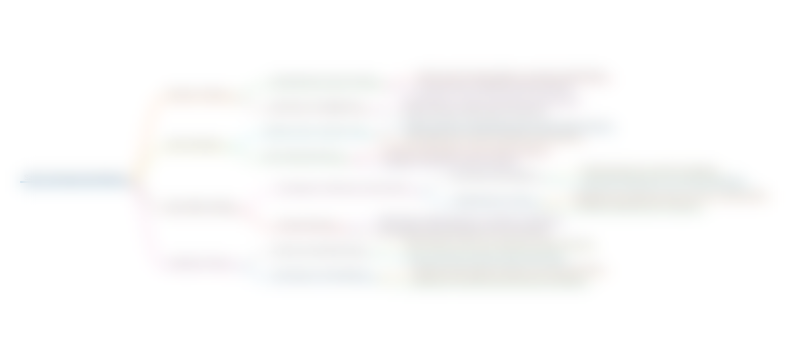
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
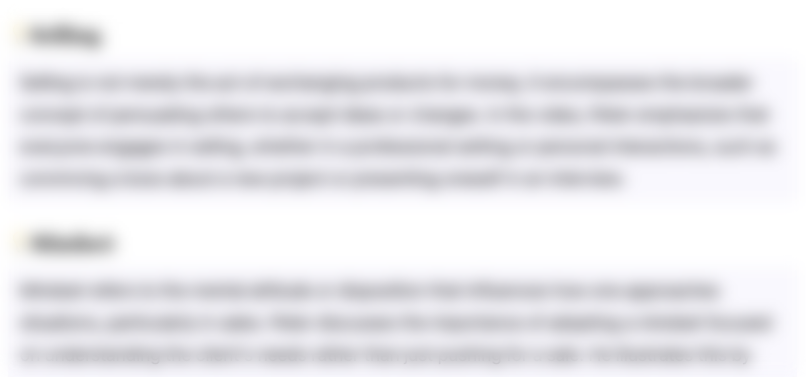
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
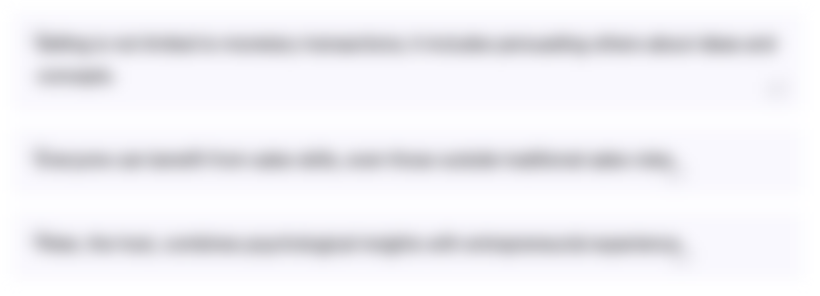
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
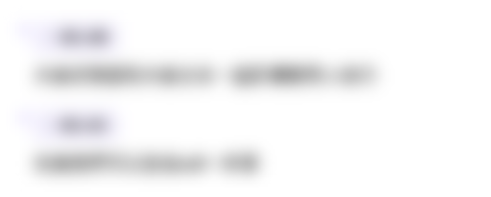
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

Classes and Objects in Python | OOP in Python | Python for Beginners #lec85
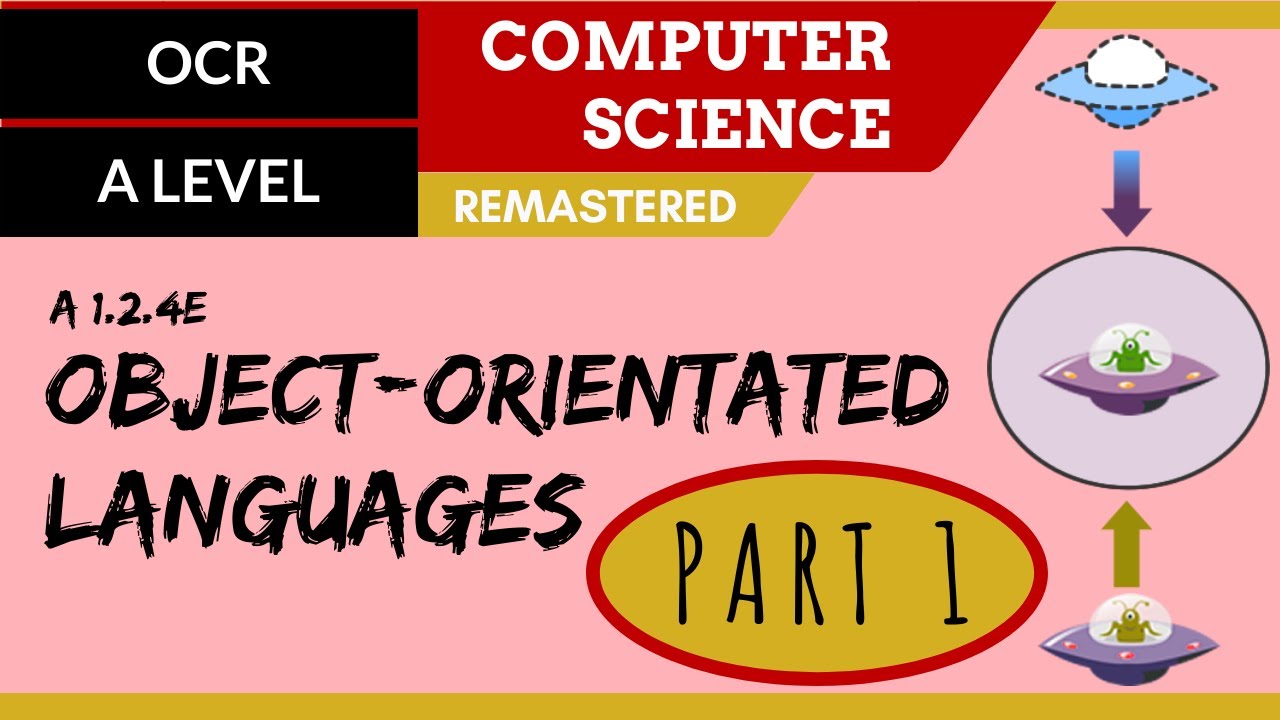
36. OCR A Level (H446) SLR7 - 1.2 Object-oriented languages part 1
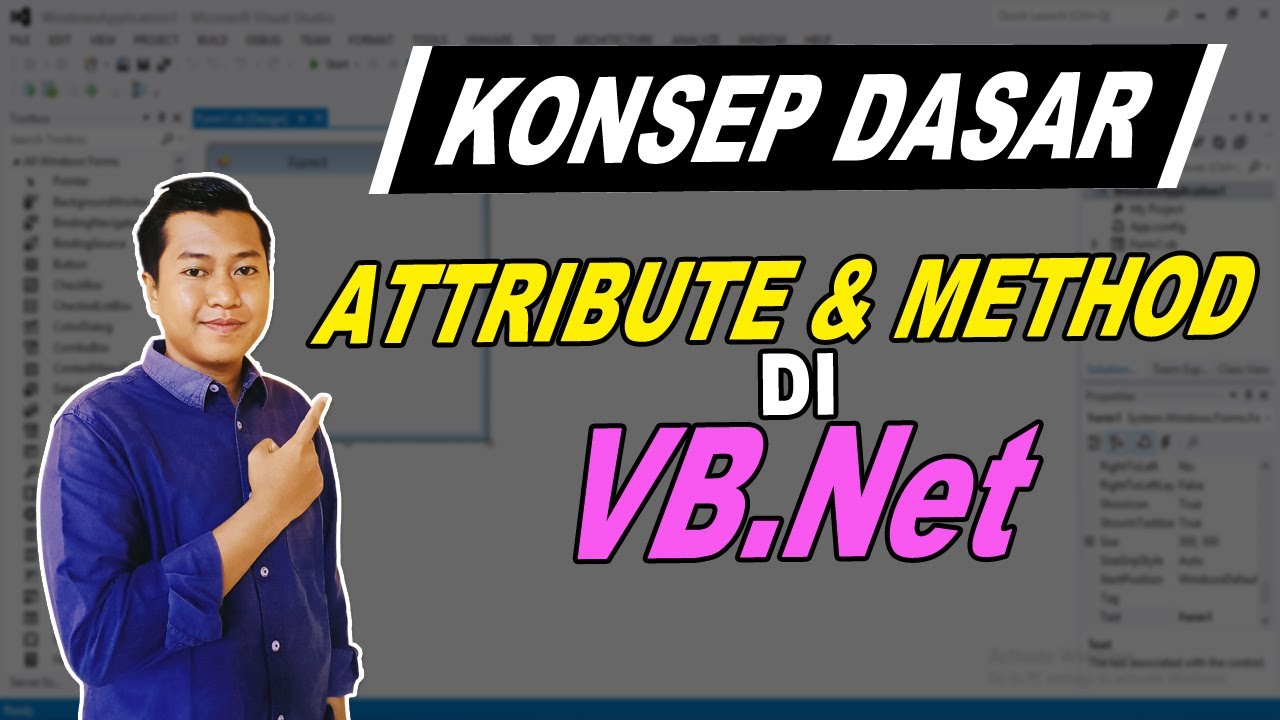
Memahami Konsep OOP di VB.Net: Penjelasan dan Contoh Attribute / Property & Method / Behavior

Criando Métodos de Objetos - Curso Python Orientado a Objetos [Aula 03]

Introduction to OOPs in Python | Python Tutorial - Day #56

Self and __init__() method in Python | Python Tutorials for Beginners #lec86
5.0 / 5 (0 votes)