Learn C# Scripting For Unity in 15 Minutes - Part 2
Summary
TLDRIn this video, Raja from Charger Games continues his 'C# in Unity' series, focusing on essential C# scripting techniques for game development. He demonstrates how to spawn game objects like a ball, manage spawn points, and trigger actions using keyboard and mouse inputs. Additionally, he explains how to use collision detection to change object colors and automate repeated object spawning with randomization. By the end, viewers will understand how to create dynamic interactions and spawn objects at random positions. The video is part of a series with more tutorials available via links in the description.
Takeaways
- 🎮 Learn C# scripting for Unity game development in a short span.
- 🌐 Part 2 of a series, check out the first part and other courses for more learning.
- 🔵 Understand how to spawn game objects in Unity using C# scripting.
- 🏐 Create a 3D object (ball), add color and a Rigidbody component for physics.
- 📍 Set up a spawn point for the game object using an empty GameObject.
- 💾 Create a GameManager object and a GameController script to handle spawning.
- 🔧 Write a function to instantiate the ball object at the spawn point's position and rotation.
- 🔄 Save the ball as a prefab for easy reuse in Unity.
- ⌨️ Implement functionality to spawn the ball when the space key is pressed.
- 🖱️ Learn to spawn the ball at the position of a mouse click on the screen.
- 🎯 Change the color of the ball upon collision with another object using a script.
Q & A
What is the main focus of this video?
-The video focuses on teaching C# scripting in Unity, specifically covering essential functionalities needed for game development within 15 minutes.
What is the first task demonstrated in the video?
-The first task is creating and spawning a game object (a sphere) in Unity, which is later used as a ball in the game.
How does the video explain adding color to the game object?
-The video explains how to create a material and apply it to the game object by dragging and dropping the material onto the object to change its color.
What is the purpose of adding a 'rigid body' component to the ball?
-Adding a 'rigid body' component enables the ball to behave according to physics, allowing it to fall when dropped into the game world.
How does the instructor demonstrate the spawning of the ball at a specific location?
-The instructor creates a spawn point by making an empty game object, assigns it a position, and then writes a script that spawns the ball at that location when the game starts.
How can the ball be spawned when pressing a specific key?
-The instructor modifies the script to spawn the ball when the space bar is pressed using `Input.GetKeyDown(KeyCode.Space)` inside the update function.
How does the video explain spawning the ball at the mouse click position?
-The video explains converting the mouse position from screen coordinates to world coordinates and using that position to spawn the ball at the clicked location using `Camera.main.ScreenToWorldPoint(mousePos)`.
What method is used to detect collision and change the ball's color?
-The method `OnCollisionEnter` is used to detect when the ball collides with another object, and the ball's color is changed using the `Renderer` component to make it red upon collision.
How is the ball set to spawn repeatedly in the game?
-The `InvokeRepeating` method is used in the `Start` function to repeatedly call the `SpawnBall` function at a regular interval, allowing balls to spawn continuously.
How does the instructor randomize the spawn position of the ball?
-The instructor creates random x and z values within a specified range and uses these to spawn the ball at random positions across the plane in the game.
Outlines
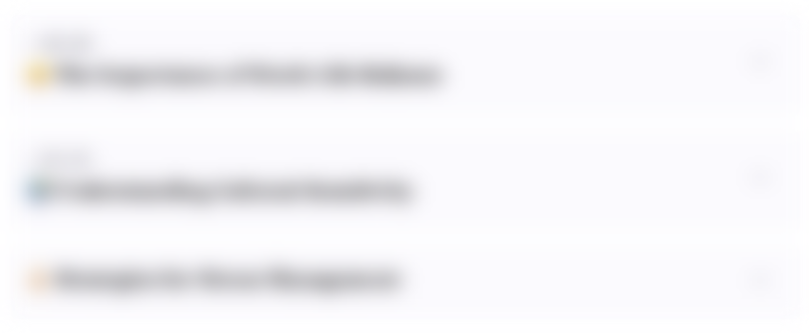
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
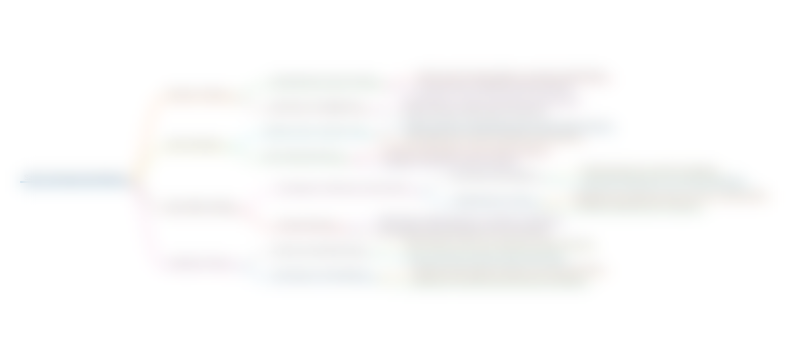
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
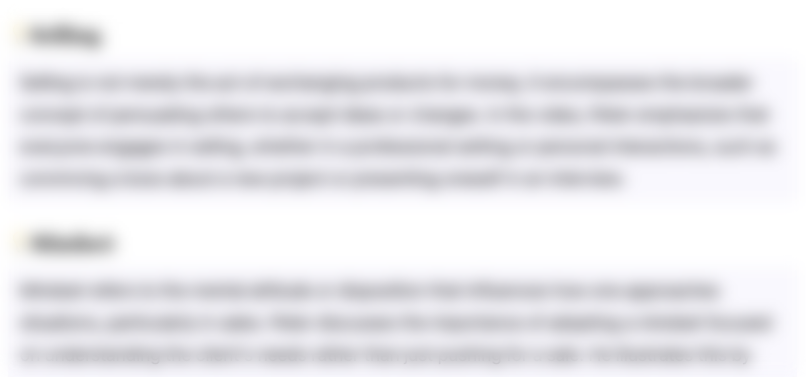
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
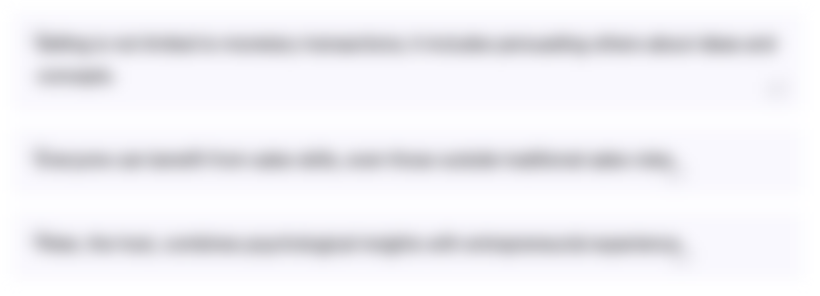
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
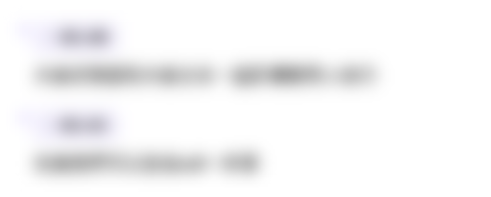
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraVer Más Videos Relacionados
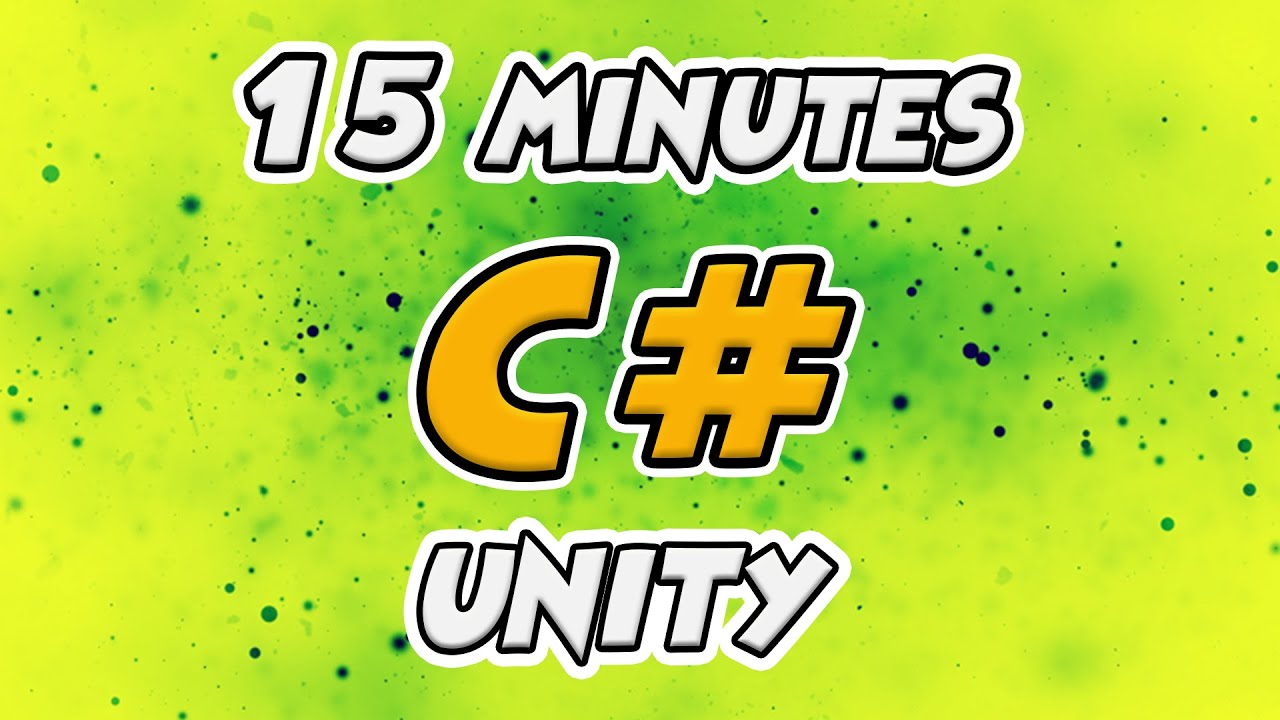
Learn C# Scripting for Unity in 15 Minutes (2024 Working)
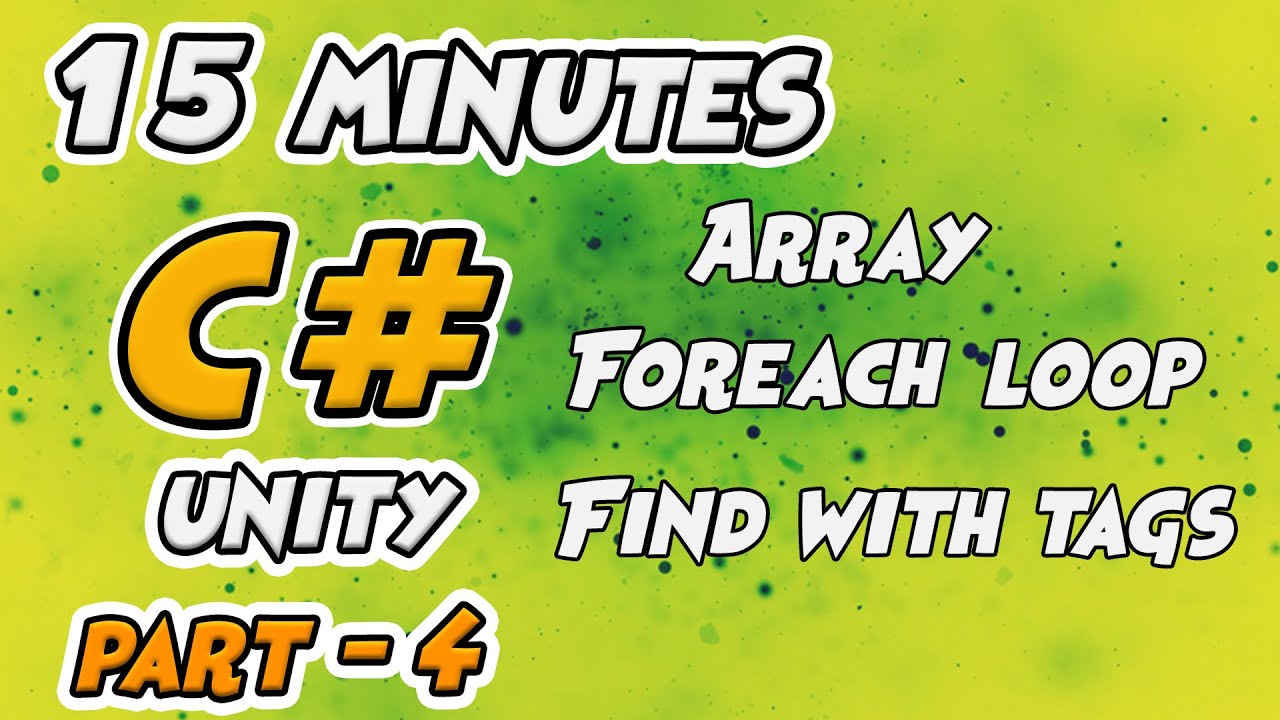
Learn C# Scripting for Unity 15 Minutes - Part 4 - Foreach Loop, Array, Find Tags
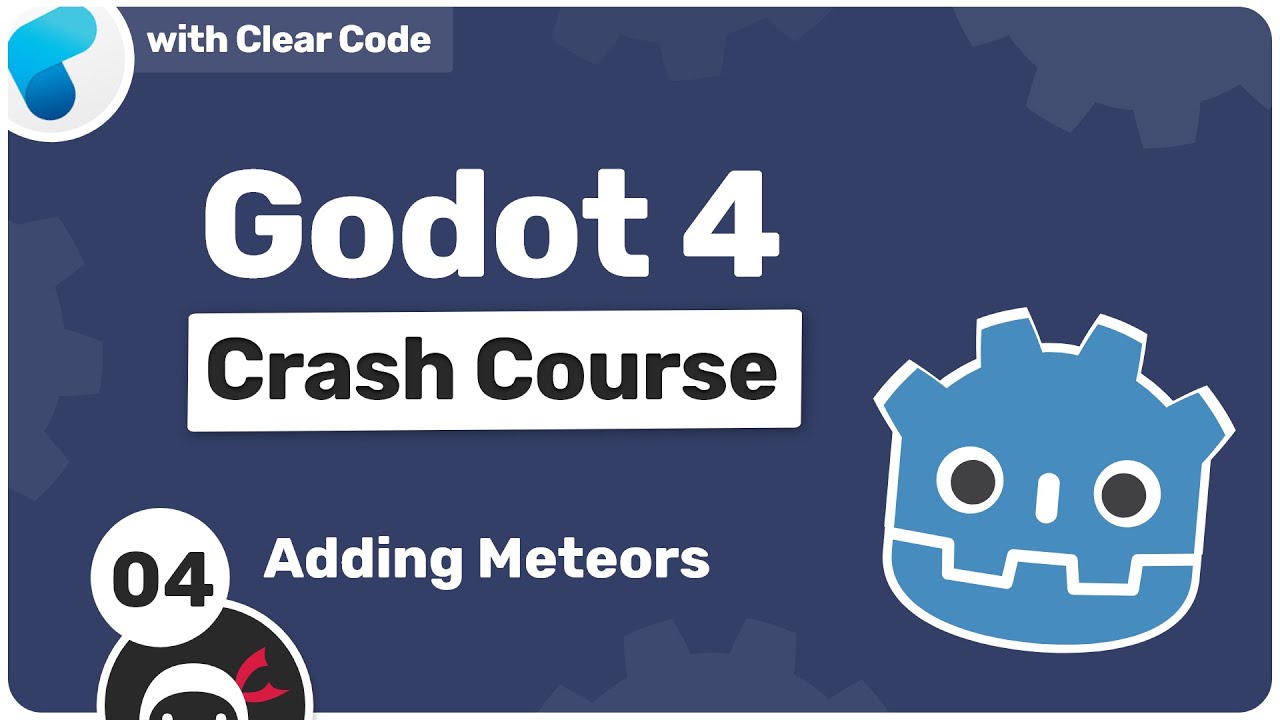
Godot 4 Crash Course #4 - Adding Meteors
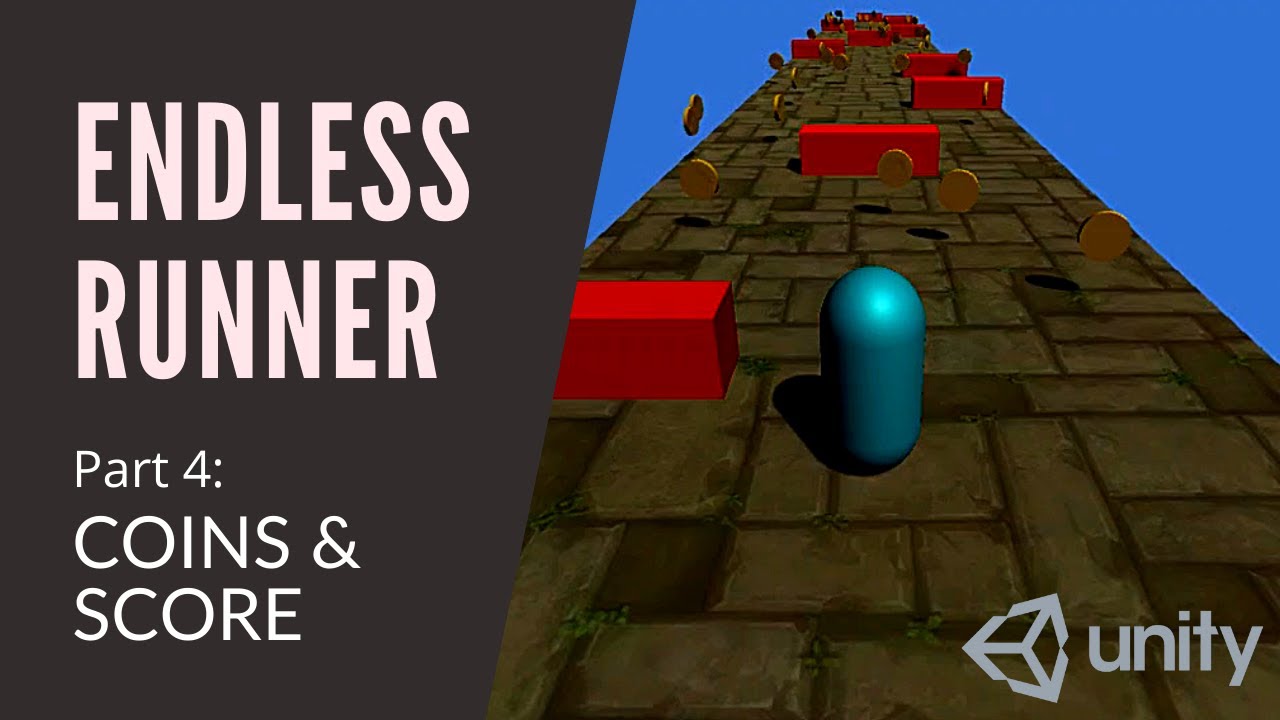
3D ENDLESS RUNNER IN UNITY - COINS (Pt 4)
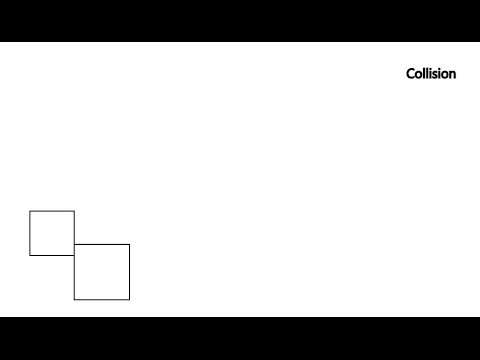
Game Dev: Collision Detection - Bounding Box
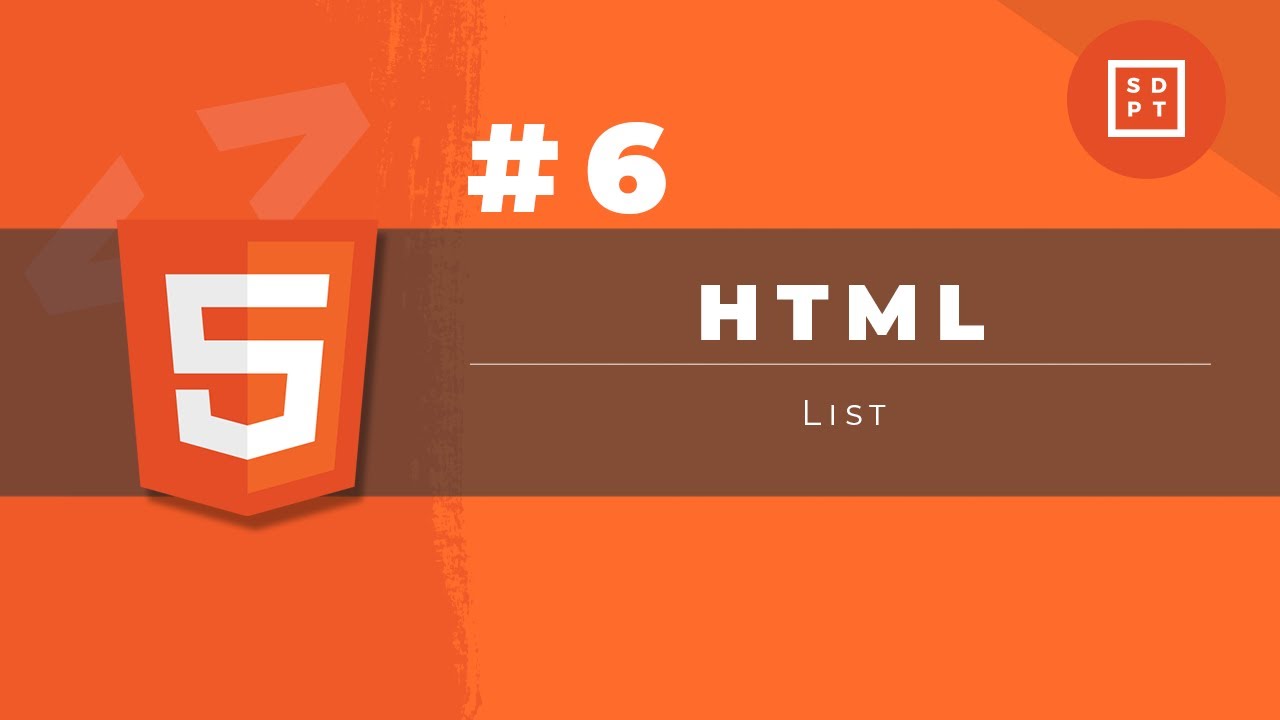
HTML Tutorial #6: List | Web Development | Filipino | Tagalog
5.0 / 5 (0 votes)