Dataframes - Part 01
Summary
TLDRThis tutorial introduces the Pandas library in Python, widely used for data manipulation and analysis. It begins with instructions on installing and importing Pandas, followed by an explanation of DataFrames, a key object that functions like a table similar to Excel or SQL. The tutorial demonstrates how to load data from various sources like CSV files and SQL databases, and provides a hands-on example of reading and manipulating a CSV file. Additionally, it touches on the integration of Pandas with machine learning libraries like Scikit-learn for data analysis and modeling.
Takeaways
- 🐍 Python's pandas library is essential for data manipulation.
- 📚 You can install pandas via pip if it's not included with Python.
- 🔌 Importing pandas is done using 'import pandas as pd'.
- 📊 A DataFrame is the primary object in pandas, akin to a spreadsheet or SQL table.
- 💾 DataFrames are optimized for data aggregation and calculations.
- 🔄 DataFrames can be inputted into machine learning models, not just arrays.
- 🌐 Data can be sourced from various formats like CSV, Excel, SQL, and APIs.
- 📁 The script demonstrates how to read a CSV file into a DataFrame.
- 🔍 The script shows how to specify the path to a file for data reading.
- 📈 The script also touches on loading datasets from libraries like scikit-learn.
Q & A
What is pandas and why is it important for data manipulation?
-Pandas is a Python library that provides data structures and data analysis tools for Python programs. It is widely used for data manipulation and analysis because it allows for efficient and easy handling of structured data.
How can you install pandas if it's not already available in your Python environment?
-You can install pandas via the terminal using the command 'pip install pandas'.
What is the typical way to import pandas in a Python script?
-The typical way to import pandas is by using the line 'import pandas as pd'.
What is a DataFrame in pandas?
-A DataFrame is a 2-dimensional labeled data structure with columns of potentially different types. It is similar to a table or a spreadsheet and is the primary data structure used in pandas.
How does pandas relate to Excel and SQL?
-Pandas can perform operations similar to both Excel and SQL. It allows for data manipulation like Excel and can execute operations similar to SQL queries.
Can pandas DataFrames be used in machine learning models?
-Yes, pandas DataFrames can be used as input for machine learning models. You can provide the DataFrame as an entry instead of an array.
What are some different data sources from which pandas can read data?
-Pandas can read data from various sources including CSV files, APIs, SQL queries, Excel files, and clipboard.
How do you read a CSV file into a pandas DataFrame?
-You can read a CSV file into a DataFrame using the function 'pd.read_csv(filepath)' where 'filepath' is the location of the CSV file.
What does the 'pd.read_csv()' function do?
-The 'pd.read_csv()' function reads a comma-separated values (CSV) file into a pandas DataFrame.
How can you specify the separator in a CSV file when reading it into a DataFrame?
-You can specify the separator in a CSV file by using the 'sep' parameter in the 'pd.read_csv()' function. For example, if the separator is a tab, you would use 'pd.read_csv(filepath, sep='\t')'.
What does the 'pd.read_csv()' function return?
-The 'pd.read_csv()' function returns a DataFrame object containing the data from the CSV file.
Can you provide an example of how to load a dataset from a machine learning library using pandas?
-Yes, you can load datasets from libraries like scikit-learn using pandas. For example, you can load the diabetes dataset from scikit-learn using 'from sklearn.datasets import load_diabetes' and then convert it to a DataFrame.
Outlines
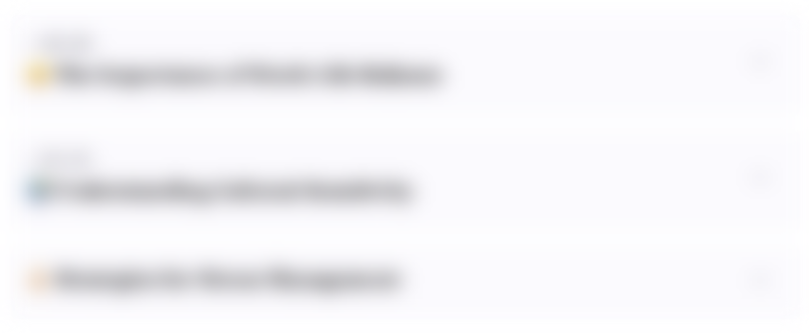
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
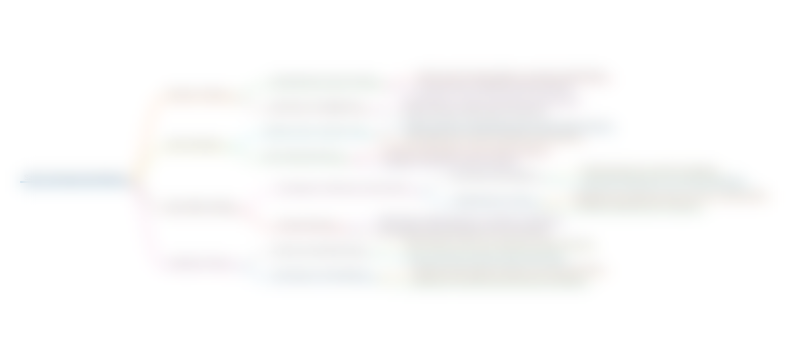
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
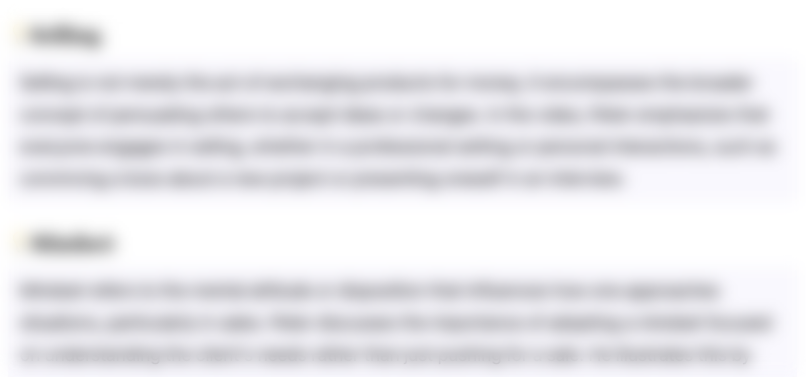
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
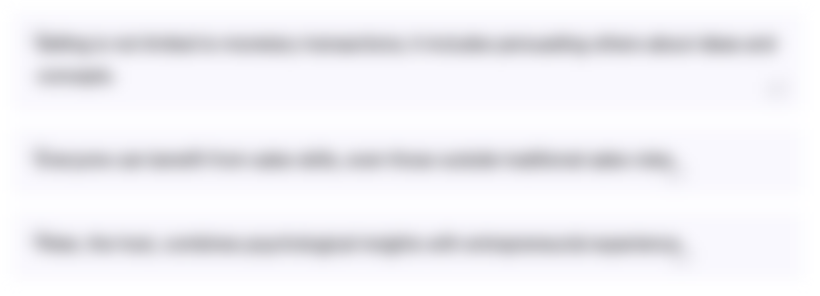
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
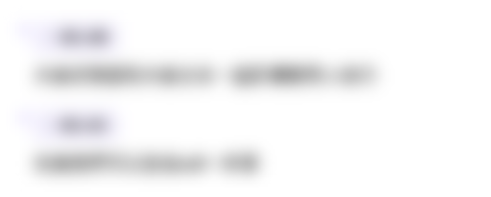
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraVer Más Videos Relacionados
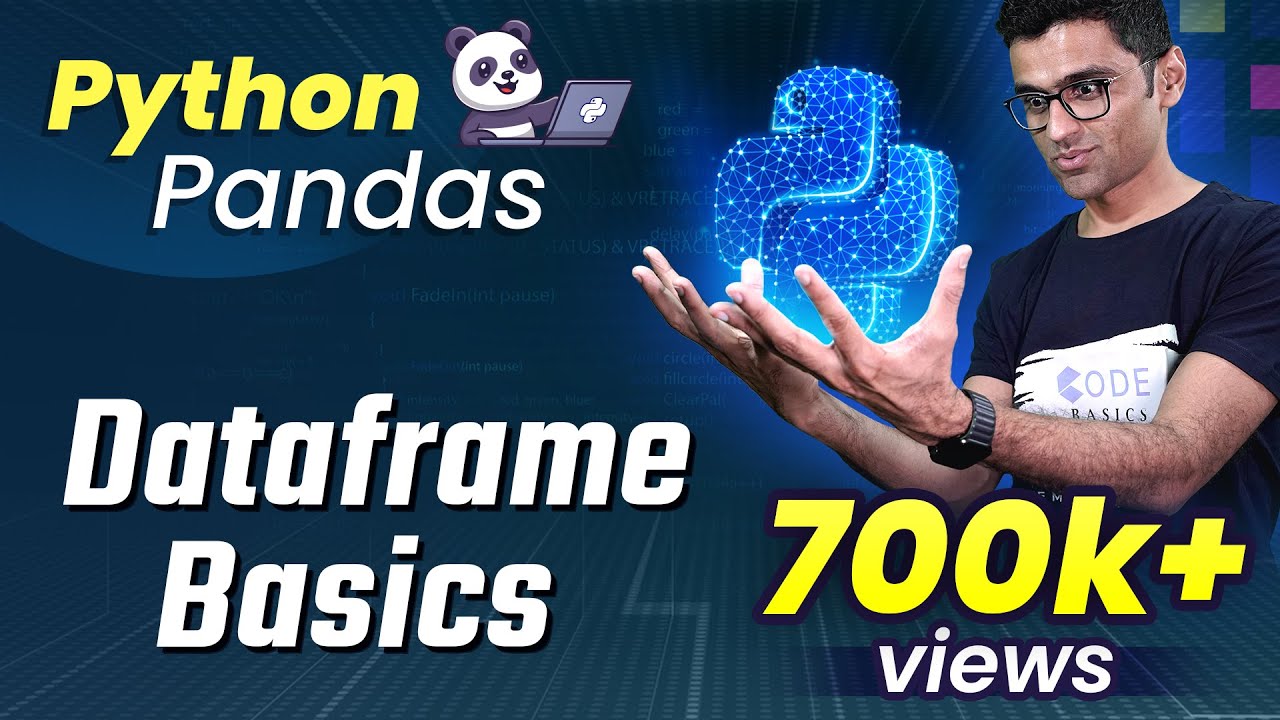
Python Pandas Tutorial 2: Dataframe Basics
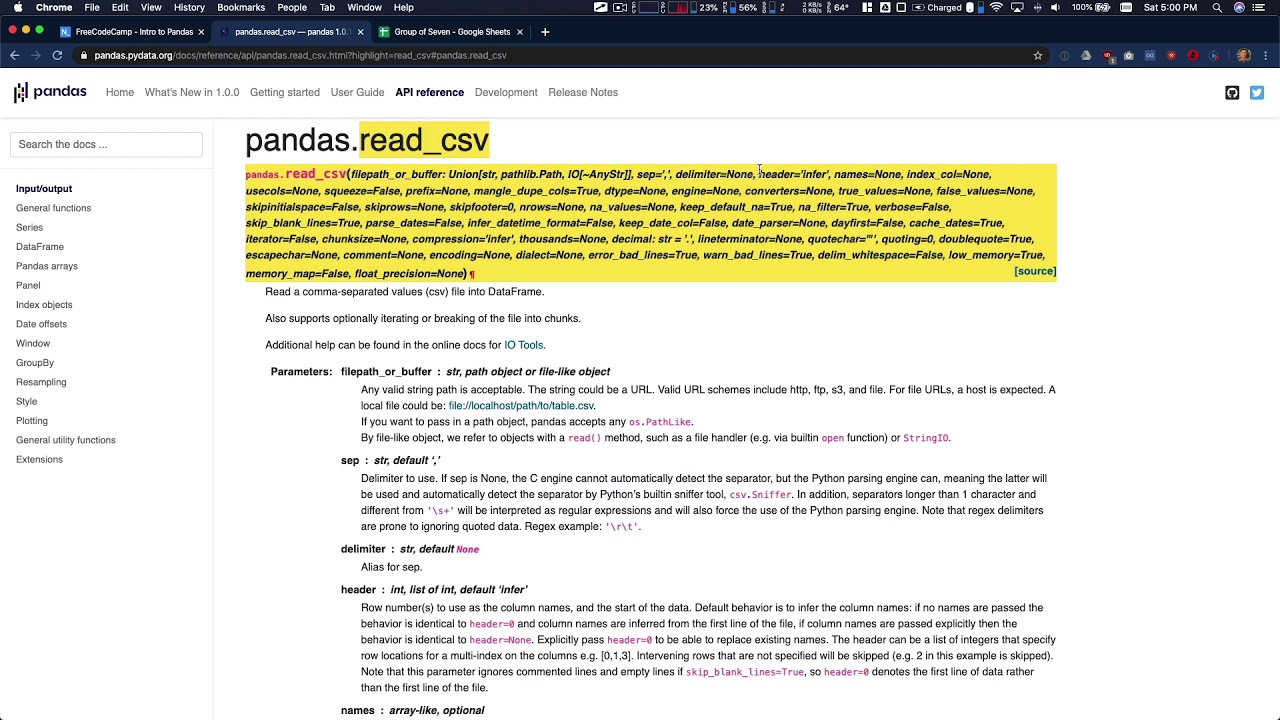
Pandas Creating Columns - Data Analysis with Python Course
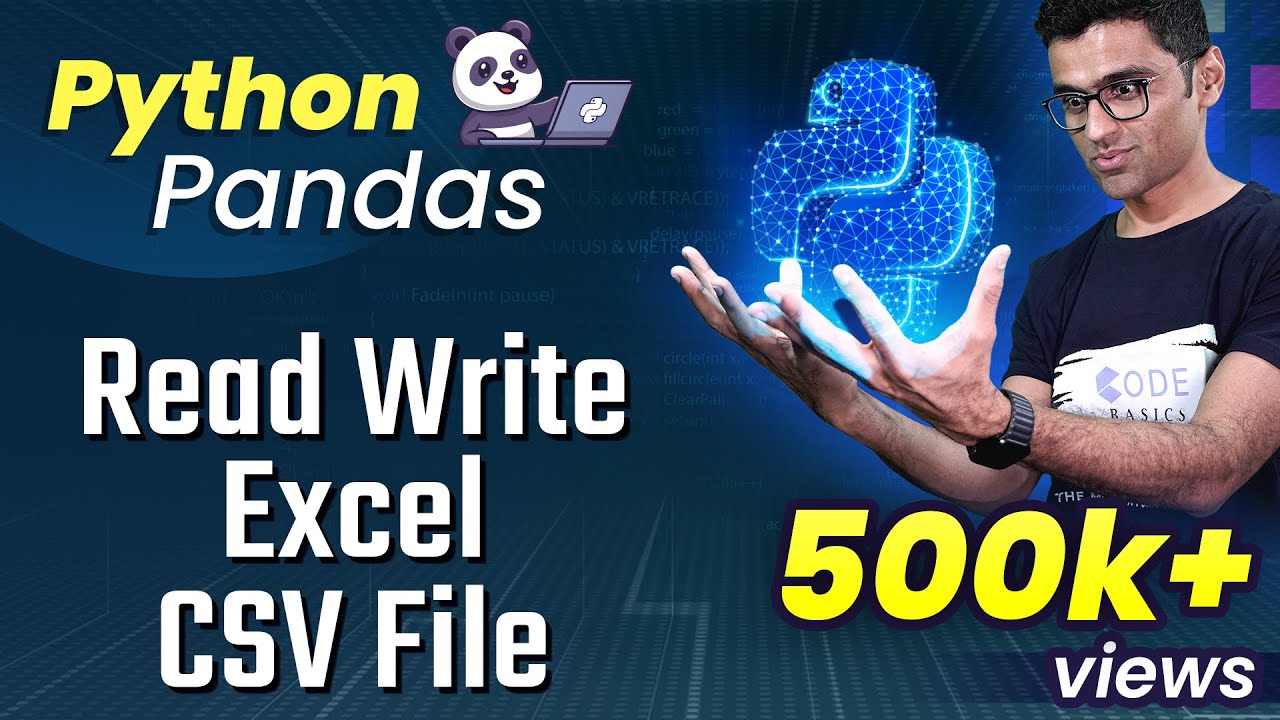
Python Pandas Tutorial 4: Read Write Excel CSV File

The Exact Skills and Certifications for an Entry Level Machine Learning Engineer
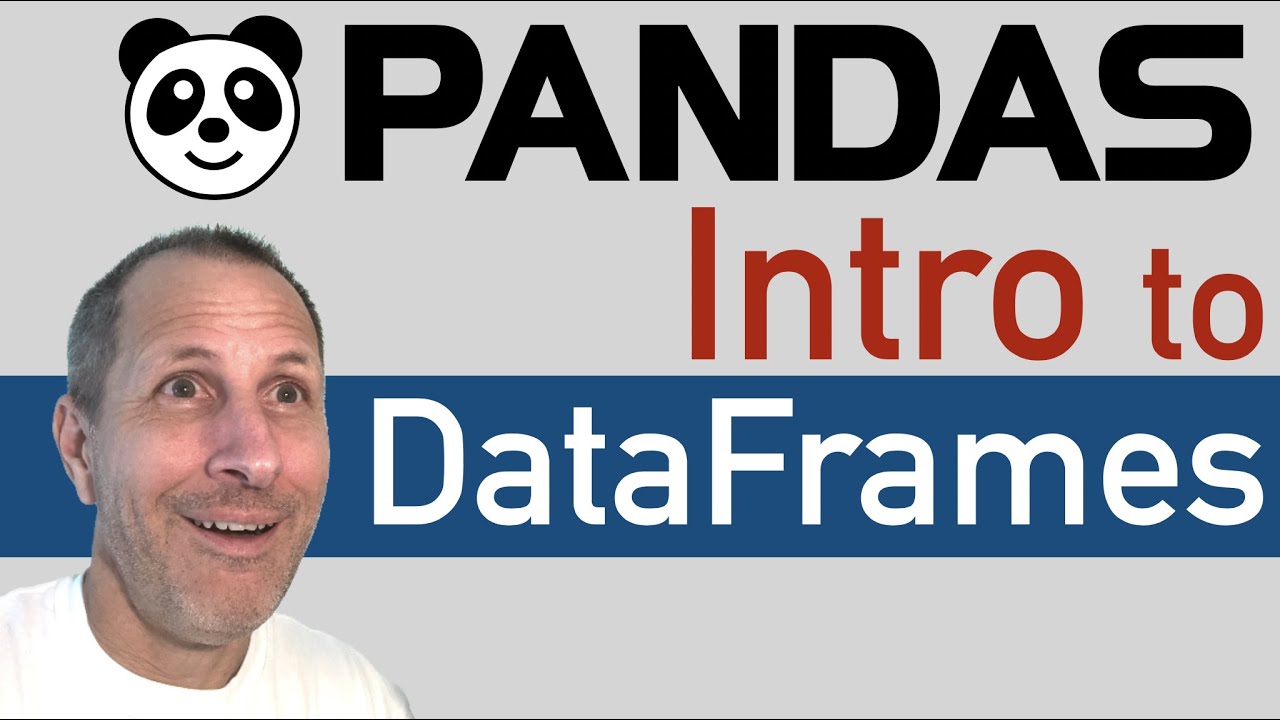
Python: Pandas Tutorial | Intro to DataFrames
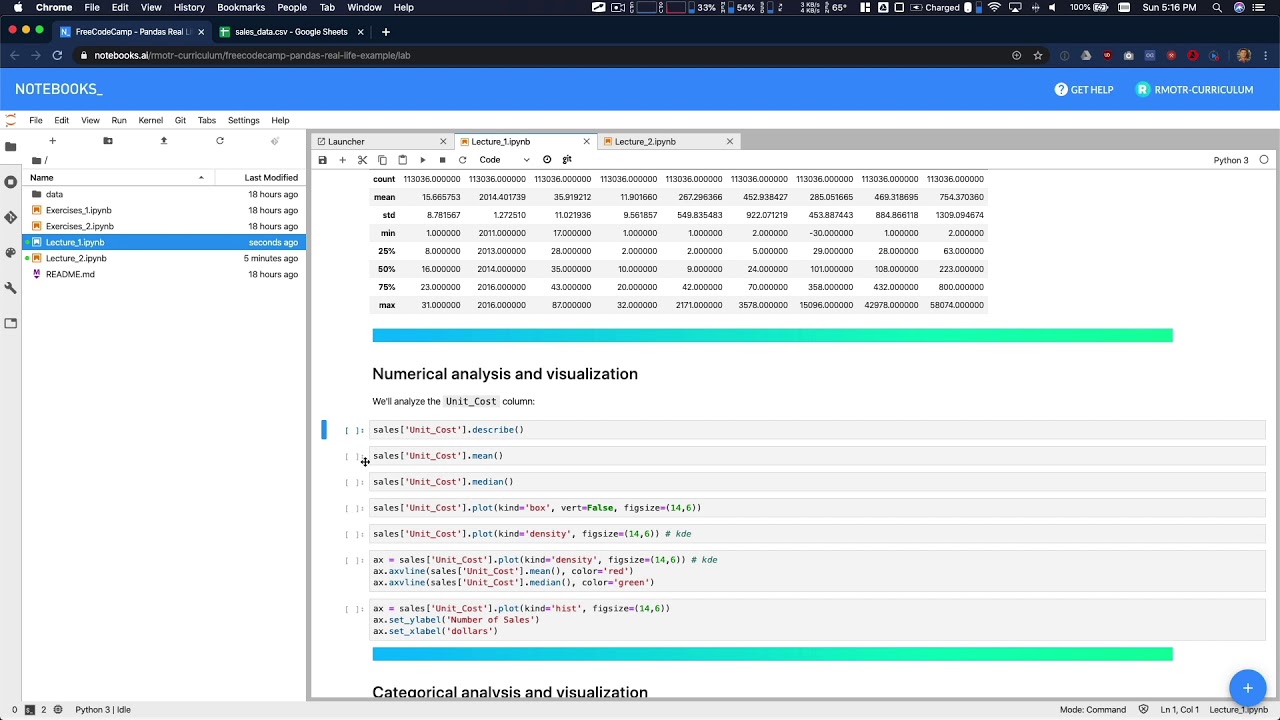
Data Analysis Example A - Data Analysis with Python
5.0 / 5 (0 votes)