Go (Golang) Tutorial #5 - Arrays & Slices
Summary
TLDRThis video script offers an in-depth tutorial on arrays and slices in Go programming. It explains how to declare and initialize arrays with a fixed length, emphasizing that arrays cannot be resized once created. The script then transitions to slices, which are more flexible and can be appended or shortened. It demonstrates how to create slices, modify their values, append new elements, and create new slices from ranges of existing ones. The tutorial concludes by highlighting the practicality of slices over arrays in most programming scenarios.
Takeaways
- 😀 Arrays in Go are declared with a fixed length and cannot be changed once defined.
- 🔢 To create an array, use the `var` keyword followed by the array name, square brackets for the length, and the data type inside the brackets.
- 👉 The syntax for initializing an array is `var [3]int{20, 25, 30}` where `[3]int` specifies an array of three integers.
- 🚫 Attempting to add more elements than the declared length of an array will result in an error.
- 🔄 You can't change the type of elements in an array once it's declared; all elements must be of the specified type.
- 📝 Shorthand array declaration in Go allows the type to be omitted on the left side of the assignment, as Go infers the type automatically.
- 🔍 The length of an array can be obtained using the `len()` function, which returns the number of elements in the array.
- 📈 Slices in Go are more flexible than arrays, allowing the length to be changed, and new elements to be appended.
- 💡 Slices are created without specifying a length in the square brackets and can be manipulated using methods like `append()`.
- 🔑 Slice ranges allow you to create new slices from a portion of an existing slice, using a start and end index.
- ➕ You can append to a slice range, effectively extending the range with new elements.
Q & A
How do you declare an array in Go?
-You declare an array in Go by using the 'var' keyword followed by the array name, square brackets to specify the length of the array, and the type of elements it will hold. For example, 'var ages [3]int' declares a fixed-length array named 'ages' with three integer elements.
What is the difference between square brackets and curly braces when initializing an array in Go?
-Square brackets are used to declare the length of the array, while curly braces are used to initialize the values of the array. For example, 'var ages [3]int = [20, 25, 30]' uses square brackets to define the array length and curly braces to set the initial values.
Can you change the length of an array in Go once it's declared?
-No, the length of an array in Go is fixed and cannot be changed after it has been declared.
How do you assign a value to an array in Go without explicitly typing it on the left-hand side?
-You can use shorthand assignment by using a colon equal sign (':=') which allows Go to infer the type automatically. For example, 'ages := [3]int{20, 25, 30}' assigns the value without explicitly typing 'int' on the left-hand side.
How do you print the length of an array in Go?
-You can print the length of an array using the 'len' function. For example, 'fmt.Println(len(ages))' will print the length of the 'ages' array.
What is a slice in Go and how is it different from an array?
-A slice in Go is a more flexible, dynamically-sized view into the elements of an array. Unlike arrays, slices do not have a fixed length and can be easily modified, such as appending new elements.
How do you create a slice in Go?
-You create a slice by using square brackets to indicate the type of elements and curly braces to initialize the values without specifying the length, like so: 'scores := []int{150, 60}'.
How do you change the value of an element in a slice?
-You can change the value of an element in a slice by specifying the index of the element and assigning it a new value. For example, 'scores[2] = 25' changes the third element of the 'scores' slice to 25.
How do you append a new element to a slice in Go?
-You use the 'append' function to append a new element to a slice. For example, 'scores = append(scores, 85)' appends the integer 85 to the 'scores' slice.
What are slice ranges and how do you create them?
-Slice ranges allow you to create a new slice from a portion of an existing slice. You create them by specifying the start and end indices within the brackets, like 'range1 := names[1:3]' which creates a new slice containing elements from index 1 to 2 of the 'names' slice.
How do you append a slice with a range of elements from another slice?
-You can append a range of elements from one slice to another by using the 'append' function. For example, 'range1 = append(range1, "cooper")' appends the string 'cooper' to the 'range1' slice.
Outlines
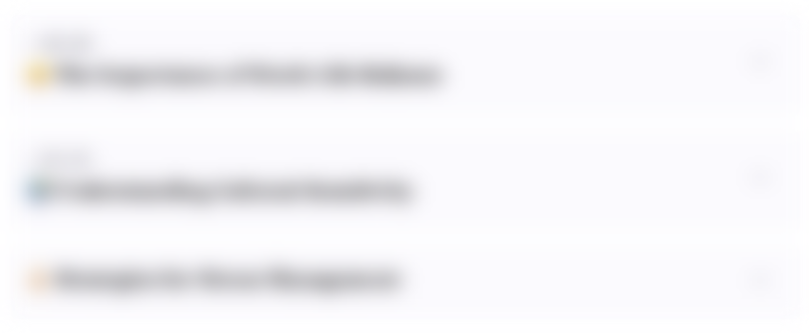
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
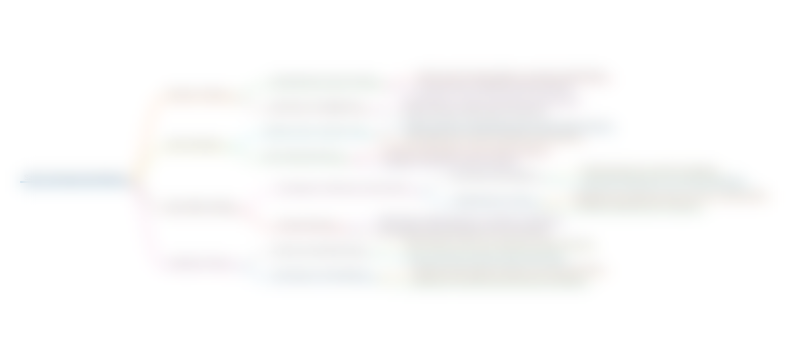
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
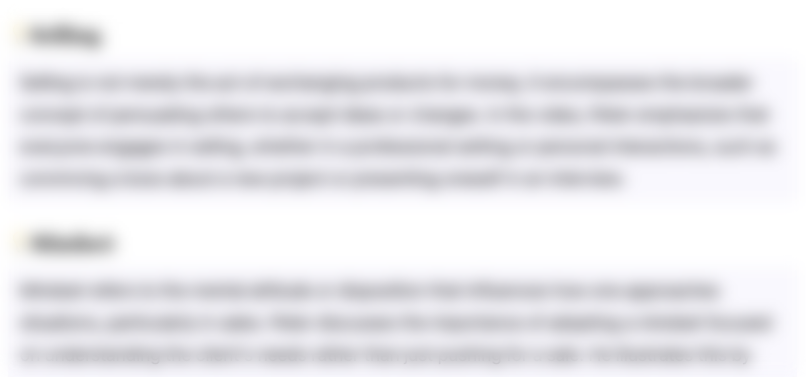
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
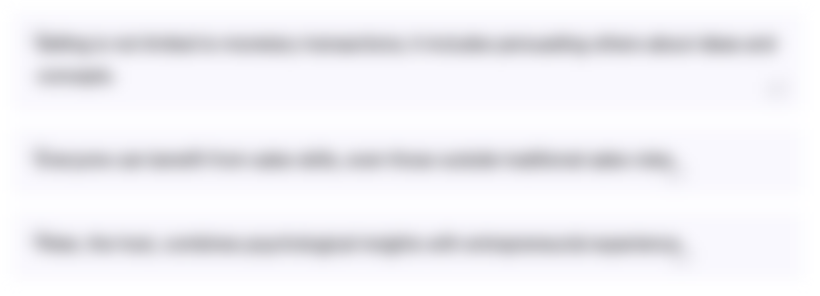
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
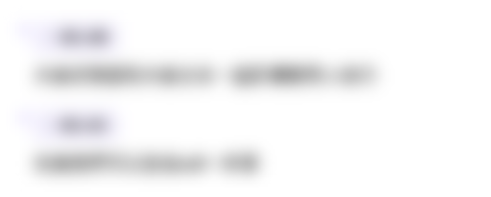
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraVer Más Videos Relacionados

Memahami Fungsi Array || Algoritma dan Struktur Data
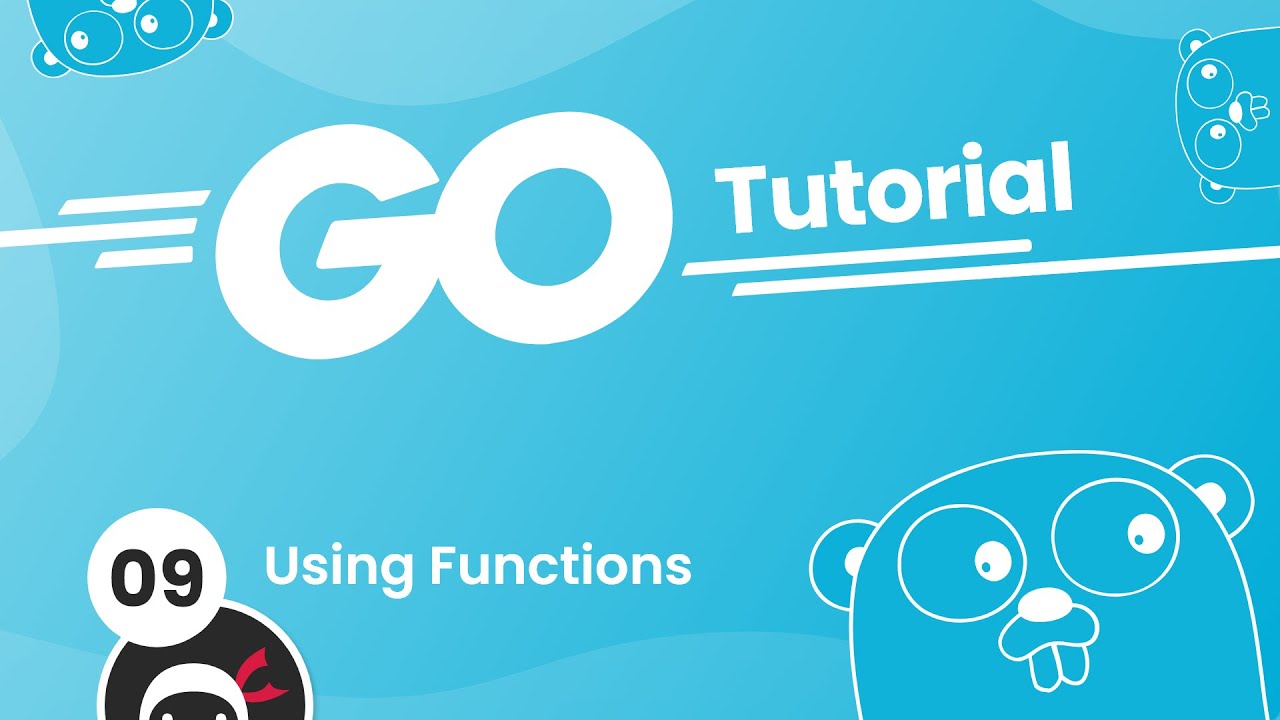
Go (Golang) Tutorial #9 - Using Functions
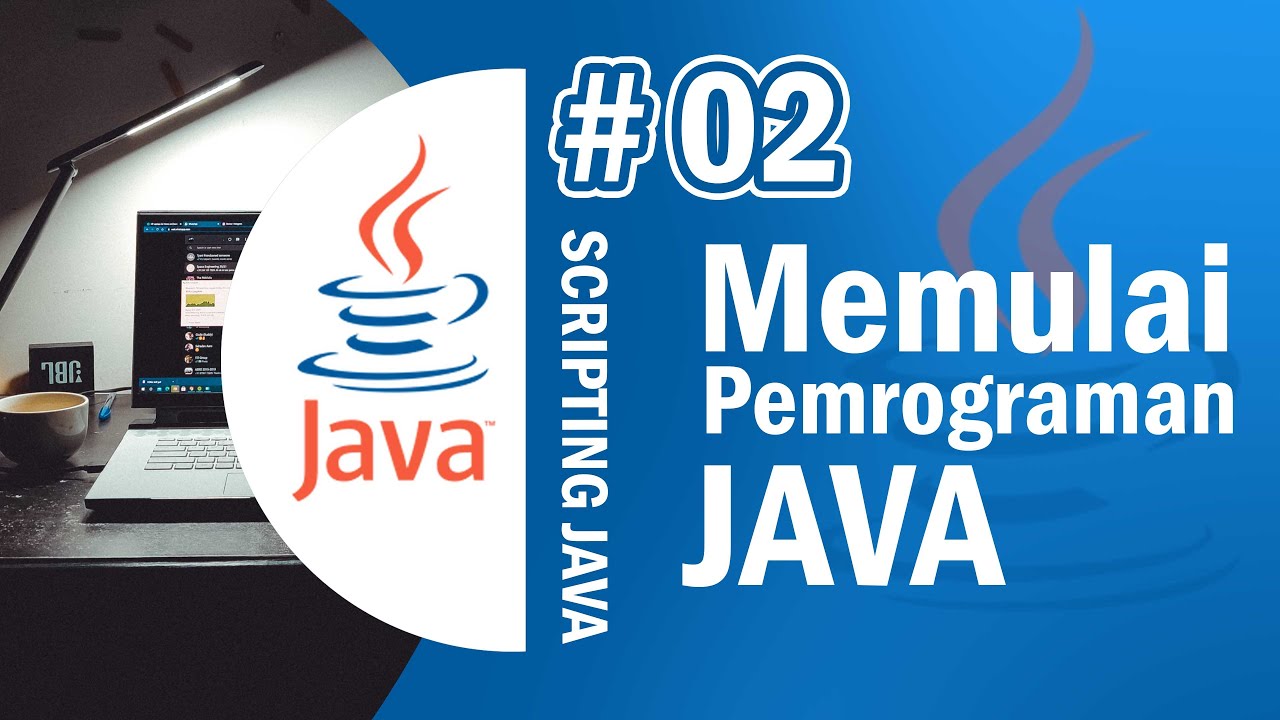
Java 02 - Memulai Pemrograman Java dengan IDE Netbeans - Tutorial Java Netbeans Indonesia
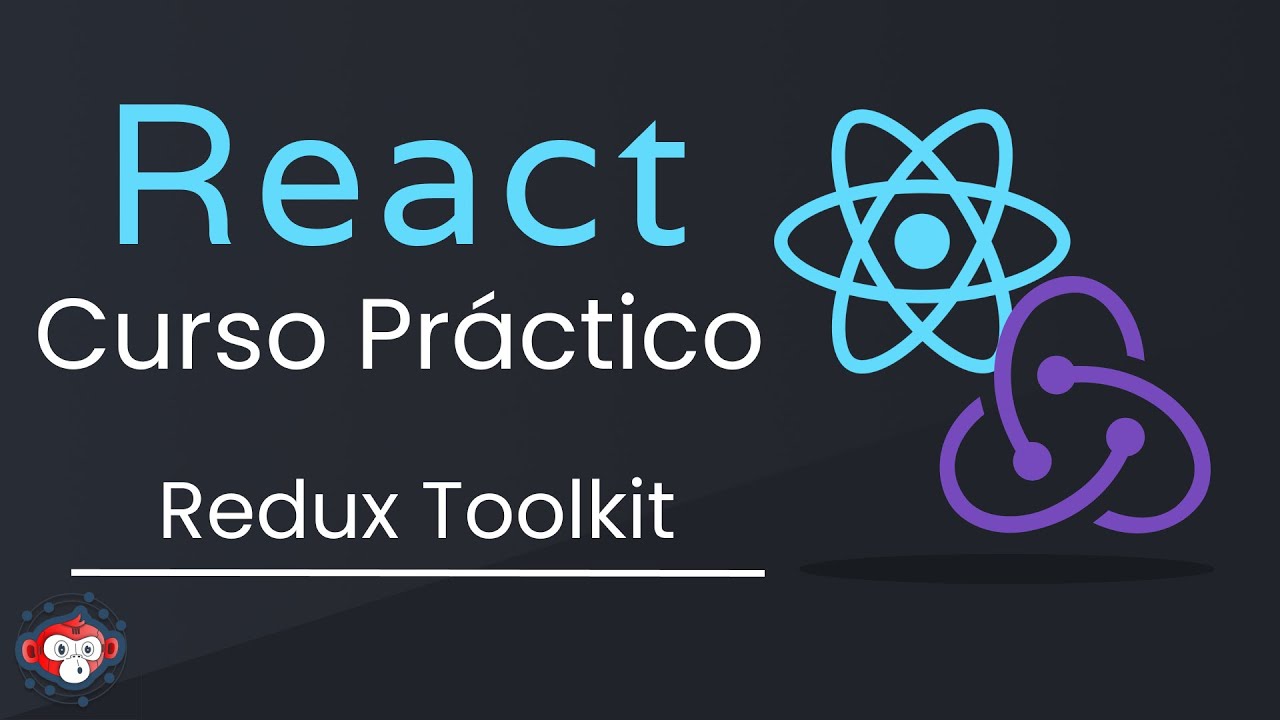
React & Redux Toolkit - Bases y proyecto práctico

Learn C Language In 10 Minutes!! C Language Tutorial
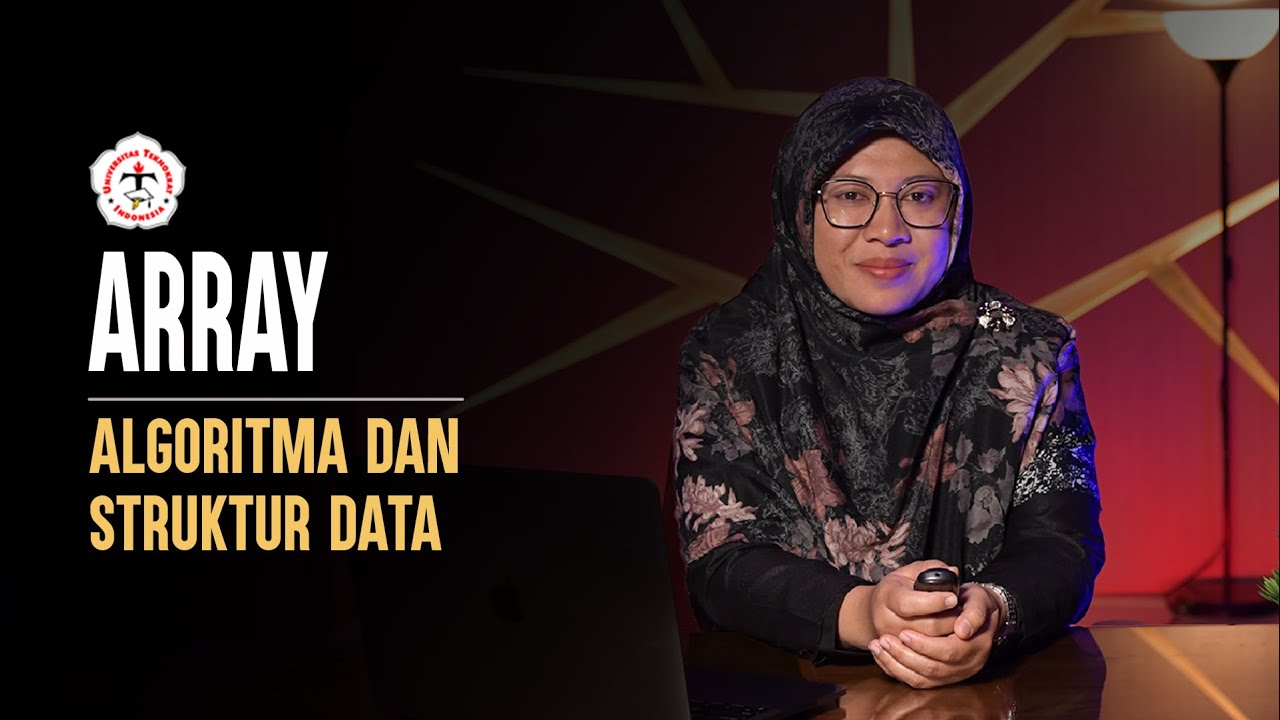
Penerapan ARRAY pada Algoritma dan Struktur Data
5.0 / 5 (0 votes)