COS 333: Chapter 11, Part 2
Summary
TLDRThis lecture delves into abstract data types (ADTs) and encapsulation, focusing on their implementation in various programming languages. It compares Java, C++, C#, and Ruby, highlighting how each language supports ADTs and access control. The discussion also covers parameterized ADTs, or generics, in C++, Java, and C#, explaining how they allow for type-specific classes at compile time. The lecture concludes with an exploration of encapsulation constructs that facilitate organization and partial compilation in large projects, such as namespaces in C# and Java packages.
Takeaways
- 📘 The lecture concludes the discussion on abstract data types (ADTs) and encapsulation, focusing on their implementation in Java, C#, and Ruby, as well as parameterized ADTs.
- 🔄 Java supports ADTs similarly to C++ but with exceptions: all user-defined types are classes, objects are heap-allocated with garbage collection, and access control is specified individually for each member.
- 🗃️ Java uses 'package' as a scope for classes without access modifiers, allowing access within the same package, which is akin to C++'s 'friend' concept but less strict.
- 📑 Java's source files are compiled into bytecode, typically kept in '.class' files, and the '.java' source is not shared with clients to hide implementation details.
- 📝 Javadoc tool in Java is used to generate documentation for classes, which is provided to clients instead of the source code for better encapsulation.
- 🔠 C# is influenced by both C++ and Java, offering public, private, protected, internal, protected internal, and private protected access modifiers, and supports structs in addition to classes.
- 🔄 C# structs differ from classes, being lightweight and not supporting inheritance or heap allocation, limiting their use in building ADTs like linked lists.
- 🏢 C# introduces properties as a convenient mechanism for implementing getters and setters, allowing for direct access to member variables with validation and processing.
- 💠 Ruby uses classes for encapsulation with dynamic interfaces, allowing classes to be modified at runtime, which can be useful for situations requiring flexible programming.
- 🔠 Ruby's instance variables are private by default, and all methods are instance methods, with no standalone functions, reflecting the language's 'everything is an object' philosophy.
- 📝 The script also covers parameterized ADTs, which are necessary in statically typed languages to allow the creation of generic classes that can work with different data types.
Q & A
What are the main topics discussed in the second part of the lecture on Chapter 11?
-The main topics discussed include abstract data types (ADTs), encapsulation, and how these concepts are implemented in Java, C#, and Ruby programming languages. The lecture also covers parameterized abstract data types and their implementation in different programming languages.
How is the support for ADTs in Java similar to C++?
-Java's support for ADTs is similar to C++ in that both languages use classes to define user types. However, Java has a few exceptions, such as all objects being allocated from the heap and accessed through reference variables, automatic de-allocation via garbage collection, and explicit access control modifiers for each class member.
What is the difference between access control modifiers in C++ and Java?
-In C++, access control modifiers can be applied to groups of entities using labels like 'public:', affecting everything under it. In contrast, Java requires explicit access control modifiers for each member, such as 'private' or 'public', and does not have a 'protected' modifier like C++ does.
What is the concept of package scope in Java, and how does it relate to the concept of 'friends' in C++?
-Package scope in Java refers to the visibility of entities without access control modifiers within the current package. It is similar to the concept of 'friends' in C++, but 'friends' in C++ is stricter as it requires explicit granting of friendship by a class, whereas in Java, any class within the same package has access to package scope entities.
How does the implementation of a Stack class in Java differ from its implementation in C++?
-In Java, the entire class definition, including member variables and methods, is placed inside a single source file with a .java extension. Java does not separate header and implementation files. Also, Java uses the 'private' keyword to hide member variables from clients, and the source file is compiled into bytecode, which is usually not shared with clients, providing better encapsulation.
What are the additional access modifiers provided by C# that relate to assemblies?
-C# provides three additional access modifiers related to assemblies: 'internal', 'protected internal', and 'private protected'. These modifiers help control the accessibility of classes and members within assemblies, which are collections of user-defined data types and resources.
How do structs differ from classes in C#?
-In C#, structs are lightweight classes that do not support inheritance and can only exist on the stack, unlike classes. They are used for small, lightweight data structures and cannot reference themselves, limiting their use in building ADTs like linked lists.
What is the purpose of properties in C# and how do they differ from accessor methods?
-Properties in C# provide a more convenient mechanism for implementing accessors and mutators without relying on explicit method calls. They allow for direct access to data members while still allowing for validation and processing code within the get and set blocks, unlike traditional accessor methods.
What is the significance of dynamic interfaces in Ruby, and how do they affect the programming language evaluation criteria?
-Dynamic interfaces in Ruby allow the structure of a class to change at runtime, enabling metaprogramming. This affects the evaluation criteria by introducing flexibility and adaptability in how classes and objects can be modified during execution, which can be beneficial for certain use cases but may also introduce complexity.
Why don't dynamically typed languages require support for parameterized abstract data types?
-Dynamically typed languages don't require parameterized ADTs because they allow for flexibility in the types of data that can be used with objects and functions without explicit type declarations. The language runtime handles type checking, making separate implementations for different types unnecessary.
What is the difference between template classes in C++ and generic classes in Java?
-Template classes in C++ are a compile-time construct that generates a new version of the class for each specified type, while generic classes in Java are a runtime construct with a single class that uses object instances and casts to ensure type safety, with the compiler inserting the necessary casts to catch type errors at compile time.
How do parameterized ADTs, or generic classes, help in organizing large projects and supporting partial compilation?
-Parameterized ADTs or generic classes allow for the creation of flexible, reusable code components that can work with different data types. This modularity helps in organizing large projects by separating concerns and enabling partial compilation, as changes to the implementation of a generic class do not require recompilation of the entire project.
What are the different encapsulation constructs provided by various programming languages to support large projects?
-Different encapsulation constructs include nested sub-programs in languages like Python, JavaScript, and Ruby; header and implementation file separation in C and C++; assemblies in C#; and naming encapsulations like namespaces in C# and C++, and packages in Java, which help in organizing code logically and supporting partial compilation.
Outlines
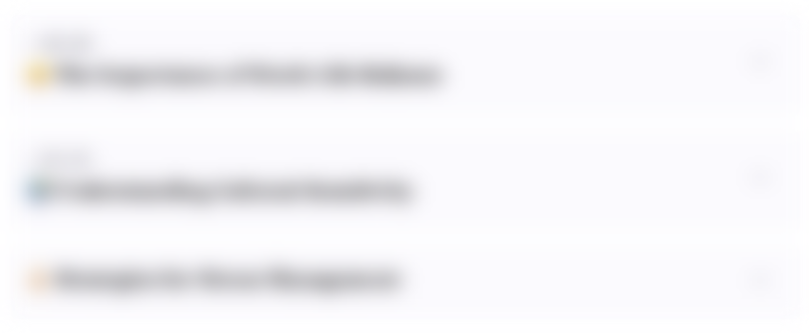
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
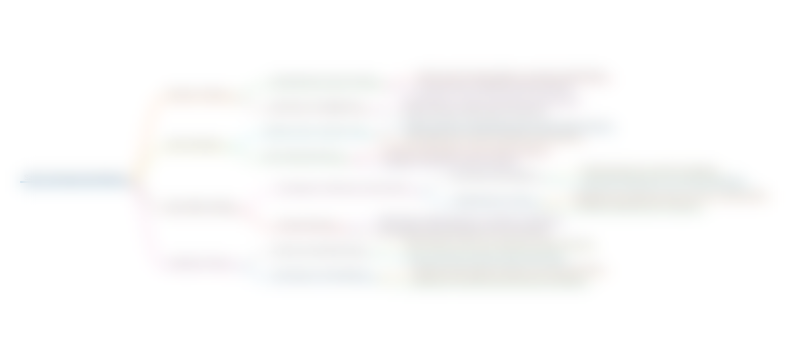
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
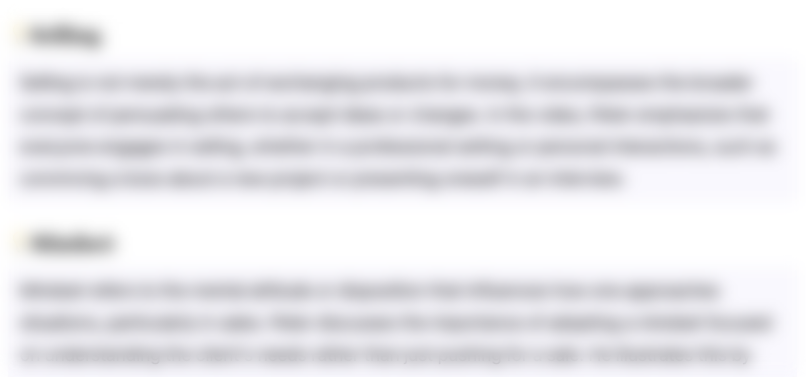
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
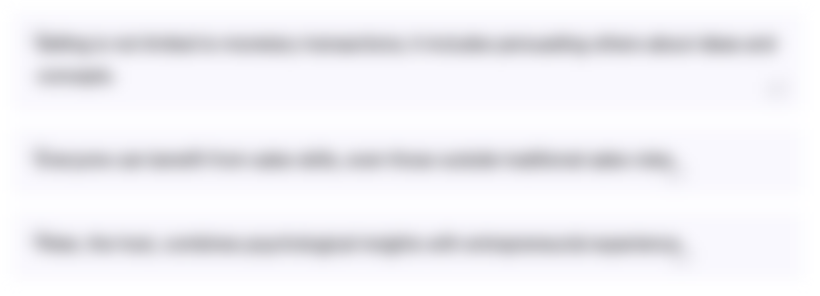
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
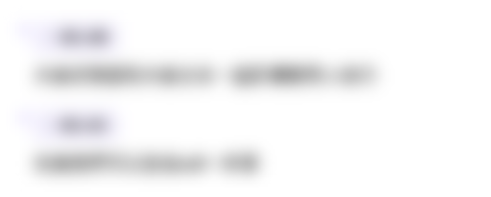
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführen5.0 / 5 (0 votes)