10 Design Patterns Explained in 10 Minutes
Summary
TLDRIn this video, the presenter delves into 10 essential software design patterns, offering a mix of theory and practical examples. These patterns, originating from the influential book 'Design Patterns' by the Gang of Four, cover key concepts like Singleton, Prototype, Builder, Factory, and Observer. The presenter highlights the importance of using design patterns thoughtfully, emphasizing how they can help solve common programming challenges. With examples from JavaScript and TypeScript, the video explores how patterns can simplify code, reduce complexity, and improve maintainability, while also acknowledging the potential pitfalls of overuse.
Takeaways
- 😀 Software design patterns help programmers solve recurring problems, improving code quality and efficiency.
- 😀 The book *Design Patterns* by the Gang of Four is influential in software engineering, categorizing 23 patterns into creational, structural, and behavioral types.
- 😀 Being a proficient software engineer is more about problem-solving with programming languages than memorizing syntax.
- 😀 Design patterns can be overused and add unnecessary complexity if not applied correctly, so they should be used thoughtfully.
- 😀 The Singleton pattern ensures only one instance of an object exists, but in some languages, built-in features like global objects might suffice.
- 😀 The Prototype pattern allows for creating objects based on existing ones, offering an alternative to traditional inheritance with a flatter structure.
- 😀 The Builder pattern enables method chaining to incrementally build objects, useful for handling complex objects like hot dog orders.
- 😀 The Factory pattern helps avoid conditional logic when instantiating objects, making code more maintainable by centralizing object creation.
- 😀 The Facade pattern simplifies complex systems by providing a unified interface to a set of subsystems, hiding unnecessary complexity from the user.
- 😀 The Proxy pattern substitutes an object with a proxy that controls access to the original object, often used in frameworks like Vue.js for reactivity.
- 😀 Behavioral patterns like Iterator and Observer help manage how data flows through a system, with the Iterator pattern enabling custom loops and the Observer pattern providing event-driven communication.
Q & A
What is the primary purpose of design patterns in software development?
-Design patterns are solutions to recurring problems that developers face in software design. They provide standardized approaches to solving common challenges, making code more maintainable, scalable, and understandable.
How does the Singleton pattern work, and why might it be unnecessary in JavaScript?
-The Singleton pattern ensures that only one instance of an object is created. In JavaScript, this can be achieved with global objects, which eliminates the need for the extra boilerplate code that the Singleton pattern introduces.
What is the Prototype pattern, and how does it differ from inheritance?
-The Prototype pattern clones objects instead of inheriting from a class. Unlike inheritance, which creates complex class hierarchies, the Prototype pattern allows for a flat structure, making it easier to share functionality between objects.
What is the Builder pattern, and how does it improve object creation in JavaScript?
-The Builder pattern allows for step-by-step object creation rather than relying on a constructor. It enables method chaining, making it easier to manage complex object creation, though it has become less common in modern JavaScript.
What is the advantage of using a Factory pattern instead of directly instantiating objects?
-The Factory pattern centralizes object creation and removes conditional logic scattered throughout the code. This enhances maintainability and flexibility, particularly when working with cross-platform applications.
How does the Facade pattern simplify complex subsystems in software development?
-The Facade pattern creates a simplified interface for complex subsystems, allowing users to interact with a higher-level API without needing to understand the intricate details of the underlying systems.
What role do Proxies play in software design, and how are they used in Vue.js?
-A Proxy substitutes for an object and can intercept and modify operations on that object. In Vue.js, proxies are used to reactively update the UI whenever data changes, by intercepting access or modifications to data properties.
What is the difference between the Iterator and Observer patterns?
-The Iterator pattern allows for sequentially accessing elements in a collection, while the Observer pattern provides a way to notify multiple objects about state changes, creating a one-to-many relationship between the subject and its observers.
How does the Mediator pattern reduce complexity in communication between objects?
-The Mediator pattern introduces a central controller that manages communication between objects, reducing the need for direct interactions and preventing complex dependencies, as seen in middleware systems like those in Express.js.
What is the State pattern, and how does it address the limitations of using conditional logic?
-The State pattern allows an object to change its behavior based on its internal state, replacing complex conditional logic. It improves scalability by organizing state-specific behavior into separate classes, making code more maintainable.
Outlines
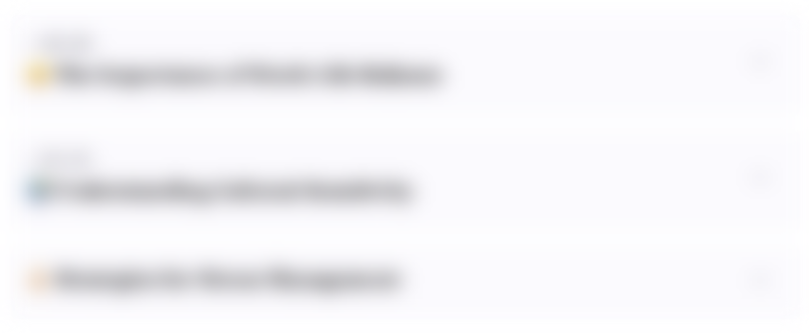
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
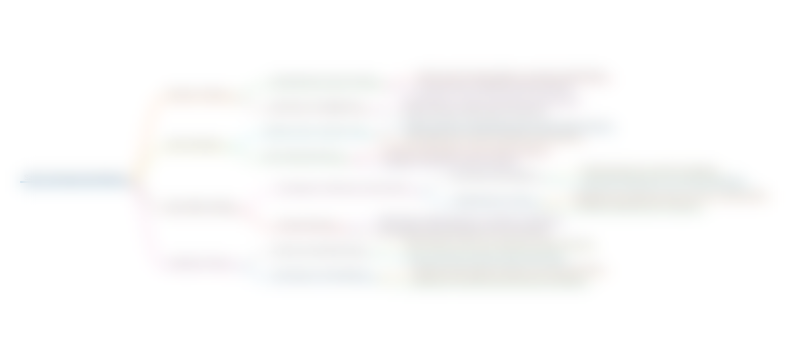
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
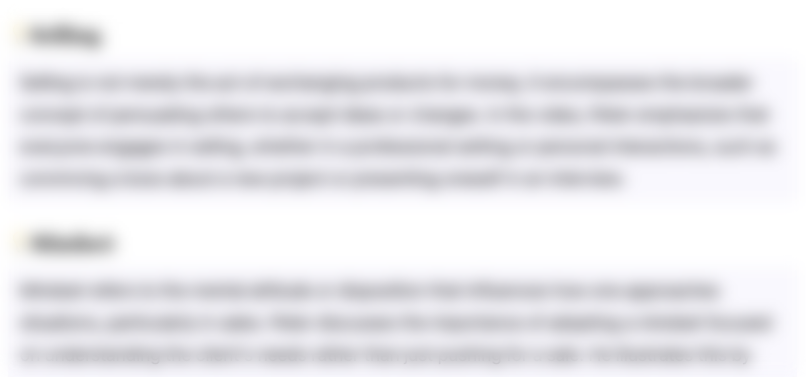
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
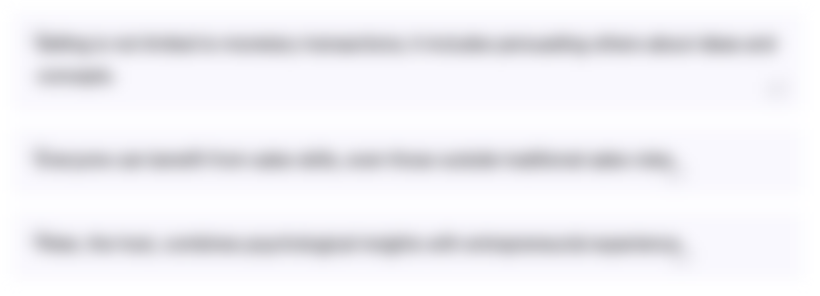
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
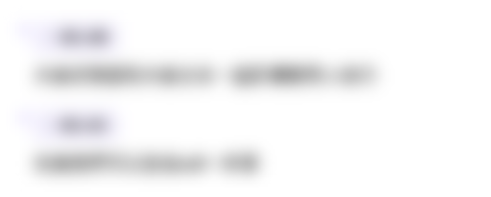
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenWeitere ähnliche Videos ansehen
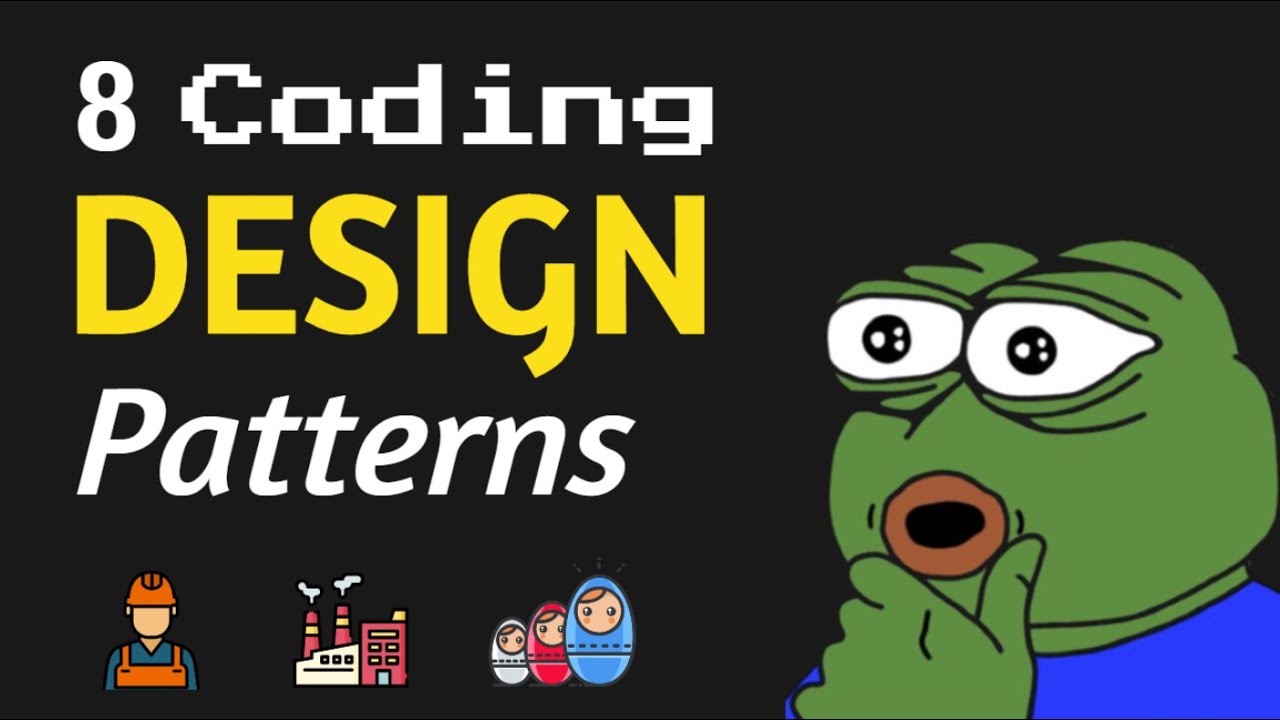
8 Design Patterns EVERY Developer Should Know
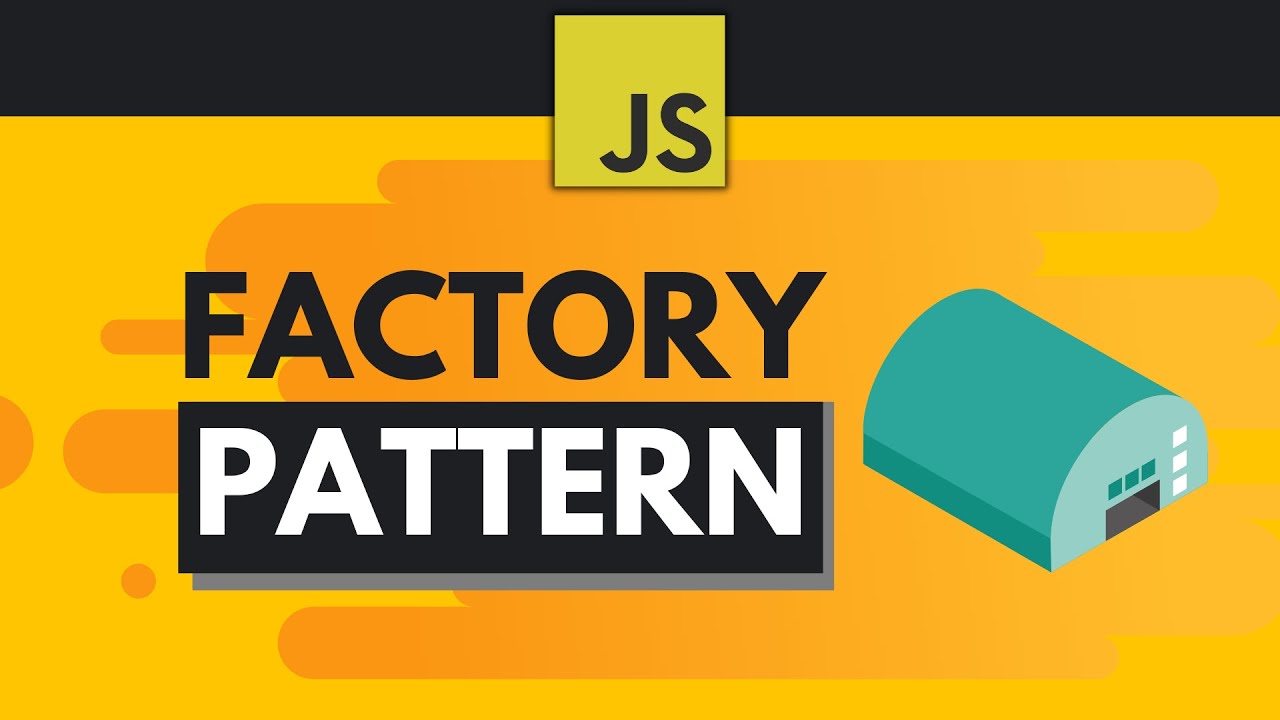
Javascript Design Patterns #1 - Factory Pattern
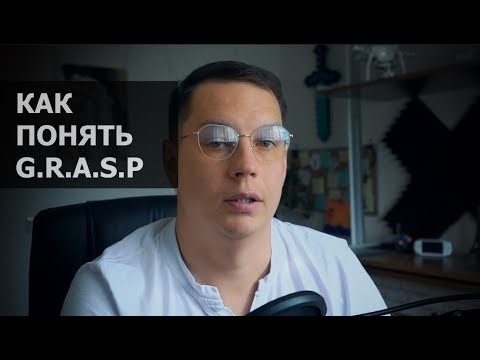
G.R.A.S.P | шаблоны проектирования

Parking Lot Design | Grokking The Object Oriented Design Interview Question

Why I focus on patterns instead of technologies
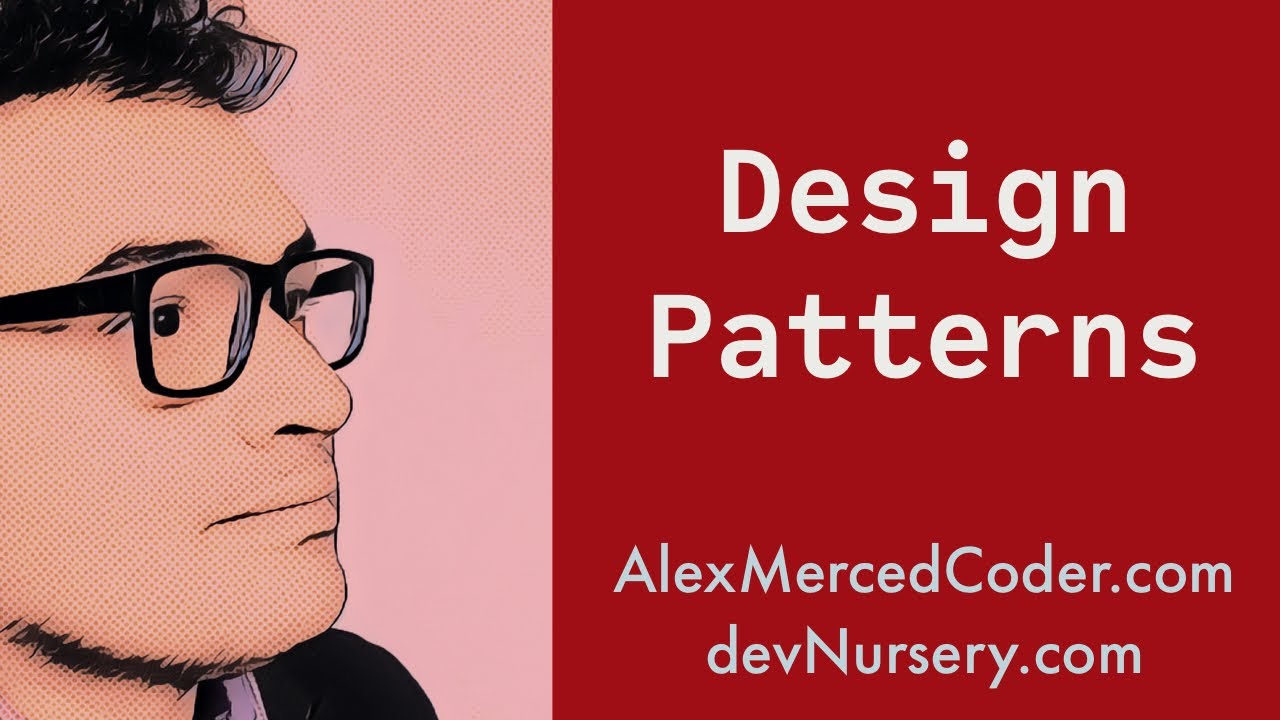
Programming Concepts - Design Patterns (Creational, Structural and Behavioral)
5.0 / 5 (0 votes)