Handling Permission in Flutter Apps | Permission Handlers | The right way to check for permissions
Summary
TLDRThis FL tutorial explains how to handle permissions in a Flutter app using the 'permission_handler' package. The video demonstrates how to request and manage permissions for accessing device-specific APIs, such as the camera or gallery. It covers how to track permission status, display alerts, and guide users to system settings to modify denied permissions. The tutorial walks through implementing this feature, handling exceptions, and ensuring a smooth user experience by rendering the appropriate UI components based on permission status.
Takeaways
- 📱 The tutorial focuses on handling permissions in a Flutter app, specifically for accessing device-specific APIs like the camera, microphone, and gallery.
- 🔑 The 'permission_handler' package is introduced as a tool to manage permission requests and track their status across Android and iOS platforms.
- 📸 An example is provided where the app uses the 'permission_handler' to access the device's gallery, with an elevated button to trigger the permission request.
- 🚫 If the user denies the permission request, the app uses the 'permission_handler' to check the status and displays an alert dialog to educate the user about the denied permission.
- 🔄 The tutorial demonstrates how to handle exceptions that occur when a permission is denied, using a try-catch block to manage the flow of the app.
- 🛠️ The 'image_picker' package is used in conjunction with 'permission_handler' to open the device gallery and select a photo.
- 📝 The tutorial guides through adding dependencies in the 'pubspec.yaml' file, which are essential for image picking and permission handling.
- 🔔 It emphasizes the importance of informing users when a permission is denied and providing options to change the permission settings manually.
- 🔄 The 'open_app_settings' method from the 'permission_handler' package is highlighted as a way to direct users to the app's settings page to modify permissions.
- 💻 The tutorial concludes with a coding segment that implements the discussed features, enhancing the app's user interface based on permission status.
- 🎥 The video script is designed to help developers create more intuitive apps by effectively managing permissions and providing a better user experience.
Q & A
What is the purpose of the 'permission_handler' package in Flutter?
-The 'permission_handler' package is used to manage device-specific permissions, such as access to the camera, microphone, or gallery. It raises permission requests and tracks their status, allowing developers to control app behavior based on the user's response.
How does the 'permission_handler' package help in handling denied permissions?
-When a permission is denied, the 'permission_handler' package keeps track of the permission status and can notify the user. It allows the app to show a dialog informing the user that the permission was denied and gives an option to open system settings to manually change the permission.
What happens when a user denies a permission in a Flutter app using 'permission_handler'?
-If a user denies a permission, the app won't be able to access the requested device feature (like gallery or camera). The permission status is tracked, and the app can inform the user, providing an option to navigate to the system settings to change the permission manually.
What is the difference in app behavior when a permission is granted versus when it is denied?
-When permission is granted, the app follows a sequential flow, allowing access to the requested feature, such as opening the gallery. If permission is denied, the app will handle the exception by showing a dialog with options to open settings and change the permission.
How can developers prevent platform exceptions when a permission is denied?
-Developers can prevent platform exceptions by wrapping the permission request logic inside a try-catch block. This ensures that any permission denial is caught and handled gracefully without crashing the app.
What is the purpose of the 'Image Picker' package in the example?
-The 'Image Picker' package is used to open the device gallery and allow users to choose an image. It works in conjunction with the 'permission_handler' package to request access to the gallery and handle permissions.
How does the app notify the user when a permission is denied?
-When a permission is denied, the app uses an alert dialog to notify the user. The dialog includes information about the denied permission and offers options such as 'Cancel' or 'Settings' to allow the user to navigate to system settings and change the permission.
What role does the 'openAppSettings' method play in the permission flow?
-The 'openAppSettings' method allows the app to directly open the system settings, enabling the user to manually change the permission that was previously denied. This is helpful when the app needs access to a specific device feature.
What should developers add to the 'pubspec.yaml' file to implement the features discussed in the video?
-Developers should add the 'permission_handler' and 'image_picker' packages as dependencies in the 'pubspec.yaml' file to implement permission handling and image selection from the gallery.
How does the app behave after a denied permission is manually granted through system settings?
-Once the denied permission is manually granted through system settings, the app behaves as if the permission was granted initially. For instance, if the gallery permission was granted, the app will directly open the gallery the next time the user clicks the corresponding button.
Outlines
📱 Introduction to Handling Permissions in a Flutter App
The video introduces handling permissions in a Flutter app using the 'Permission Handler' package. This package allows the app to request access to device-specific APIs like the camera, microphone, or gallery, and helps track the permission status across platforms (iOS and Android). The tutorial demonstrates a simple use case where a button triggers the gallery access, raising a permission request. If the request is denied, the user is informed through the package's built-in status tracking, which also enables them to revoke or modify permissions through system settings.
🚫 Managing Denied Permissions and Handling Exceptions
The second part explains how to handle denied permissions and related exceptions using try-catch blocks. If the user denies the permission, the app throws a platform exception, which is caught and handled gracefully. By using the Permission Handler package, the app tracks permission status, showing an alert dialog to notify the user if a permission is denied. The user can then navigate to system settings to change the permissions manually. The flow is demonstrated for gallery access but can also be applied to other device-specific APIs like the camera or microphone.
Mindmap
Keywords
💡Permissions
💡Permission Handler
💡Device-specific APIs
💡Image Picker
💡Exception Handling
💡Alert Dialog
💡Settings Button
💡Elevated Button
💡UI Components
💡Cross-platform API
Highlights
Introduction to handling permissions in a Flutter app, focusing on accessing device-specific APIs like camera, microphone, and gallery photos.
Introduction of the 'permission_handler' package in Flutter to request, track, and manage permissions across Android and iOS platforms.
Using the 'permission_handler' package to handle permission requests when accessing the device gallery, either granting or denying access.
Description of the process for granting or denying permissions for device-specific APIs, such as the gallery, and how the app reacts to each.
Explanation of how the app can keep track of permission status and provide users with information about denied permissions through alerts.
Illustration of how the 'permission_handler' package allows the user to revoke or change permissions manually through system settings.
Walkthrough of creating the user interface with a custom app bar and a primary button that triggers the permission request.
Implementation of the 'getFromGallery' method using the 'image_picker' package to open the device gallery and select a photo.
Detailed explanation of wrapping permission logic inside a try-catch block to handle exceptions and display error messages if permissions are denied.
The app ensures that once permission is denied, the user will not be asked again to grant the permission, making it a one-time process.
Handling denied permissions through an alert dialog that offers users options to either cancel or navigate to system settings to modify permissions.
Introduction of the 'openAppSettings' method from the 'permission_handler' package to directly guide users to the app’s settings page.
Explaining how the app checks permission status, educates users about permission denial, and updates the UI accordingly.
Demonstration of how denied permissions can be re-enabled through system settings, allowing the app to access the requested API again.
Clarification that the same permission handling process applies to other device-specific APIs like the camera and microphone, making the app more intuitive for users.
Transcripts
[Music]
hey everyone welcome back again to
another FL tutorial and in this s we
will look into how to handle permissions
in our f app so the permission here what
we talk about is the ability to access
the device specific apas like the camera
microphone or the gallery photos Etc and
to make this possible we'll be using one
such package available in pb. called the
permission handless and you can also
take a look at that and this is going to
be the package which we have been
talking right now the permission
handless which actually does the job of
raising the request whenever the flat
appap invokes any device specific AP
access like the camera microphone and
also at the same time this package keeps
track of the permission status and you
can also see that this package provides
a close platform Android and iOS API to
request and check for the permissions so
this package actually comes in handy
while you're dealing with uh the process
of accessing the device specific AP in
your frap now let's head back to our vs
code and try to understand this with the
help of a simple example what we have
here is a running example of the same we
have used the permission Handler package
to access the device Gallery we have a
elevated button placed right to the
center and which when click it should
take us to the device gallery and allow
us to choose a particular photo consider
we have opened the app for the very
first time and if I try to click this
button it rises the request whether to
approve or deny the request for the
particular AP which which is the photo
in this case you can either go for
approving the request or you can also
deny the request if you go for approving
the request the process is going to be
linear nothing is going to change rather
consider if you try to deny the request
where we click this don't allow button
now we have deny the request either
knowingly unknowingly and if we try to
click this button again we will no
longer see the request to revoke the
permission or to change the permissions
rather with the help of the permission
handers you'll be able to keep track
with the status and since we are using
this package in a f app it try to
provide the user with the relevant
information of the permission status
that the permission has been denied for
the photo or the gallery and this is
done possible with the help of the
package permission handers just with the
help of the status we'll be able to
enter this alert box and educate the
user about the permission status where
the user can go directly to the system
settings and revoke the permission or
change the permission manually in this
case the permission is being denied
let's change it to all photos and head
back to our FL appap and this time if I
try to click this button you will no
longer see that alert box rather it
takes us directly to the device gallery
and allow us to choose the photo since
the permission has been granted so this
is where the usage of the package comes
in it keeps track of the permission
status either it is approved or denied
based upon which we can render the UI
accordingly either to ask for a
permission again or to go with the flow
of the app itself hope you got a better
understanding of how to make use of the
the permission handlers to process the
request and keep track of the permission
status in a fredra with this idea and
without any further delay let's directly
jump into the coding part and start
implementing this feature in our
flra first let's try to add two
dependencies in our perspect and the
dependencies are the image picker and
the permission Handler the image Pier is
the package that helps us to open the
device gallery and choose the photo and
the permission Handler is what we have
been talking earlier all right so make
sure you add these two dependencies in
your perspect and after adding these two
dependencies let's head over to the
main.
file here in the main. file the home
points to my homepage the my homepage is
nothing but a stateless wiget class with
the empty scaold first let's start by
building up the uis let's create AB bar
right so this abar is nothing but a
custom defined function which is written
down separately inside the components
folder here inside the components we
have the app bar defined which is going
to be a generic app bar so we just need
to pass the title and few other optional
parameters and the rendering will be
done accordingly so we'll make use of
this build AB bar wiet which is a user
defined
function and after the app bar let's
start with the body where inside the
body we have a primary button which is
an elevated button technically just the
same way this primary button is nothing
but a custom defined function that
internally renders the elevated button
widget okay so we make use of this
primary button which is written down
inside the same components folder just
like the app bar
okay and the button function is going to
be the get from gallery that we will
Define shortly second parameter is going
to be the button text now let's try to
Define this get from Gallery
method here this get from Gallery method
is where we will be writing the logic
for opening the device gallery and try
to pick a photo so let's make use of the
image picker and make use of the method
pick image
now we have written down the code for
picking the image from the gallery we
haven't made use of the permission
Handler here so let's try running this
app and see how it
works since I am opening the app for the
first time it asks for allowing or
denying the request if I click allow the
flow is going to be sequential no ex is
going to happen but consider I click
this don't allow that is I deny the
request you see that a platform
exception has occurred in order to
handle this exception we need to wrap
this code inside the catch
block and inside the catch block let's
try to just print the exception and if I
refresh this app you no longer get the
permission request again it is a one
time process so
let's delete this
app and try to reun the entire project
once
again here the only change is that we
have wrapped this code inside the TR
cast block and if I click this and try
to deny the request you will no longer
see the except extion rather you will be
able to see the exception here in the
debug
console this is one decent way of
handling the exceptions while dealing
with the permissions but this is not
something which we are about to discuss
here so so let's get of that and here
inside the cat block which means that
the permission has been denied so this
catch block get executed only if there
is an exception happening here during
the process of granting or denying the
request considering the possibility of
denying the request this catch block
gets executed right therefore inside
this cat block let's make use of the
permission status with help of the
permission Handler we'll be able to
check for the status of the permission
here in this case we use the photos you
can also check for the
camera or the
microphone EXC thanks to the permisson
Handler package that helps us keep track
of the status and based upon the status
if it is denied if the status is denied
we try to show alert dialogue or else we
try simply try to print the exception
here and let's now try to Define this so
aler
dialogue this so alert dialog is going
to be a Cino dialogue and inside which
we try to define the title as permission
denied since the status is denied we try
to provide the user with the relevant
information of the same and we try to
have two Cino action dialogue one is
going to be the cancel button and second
is going to be the settings button which
will in turn take us to the system
settings to do the process of changing
the permission manually whenever the
cancel buttons press we are not going to
do anything just we will try to close
the alert dialog here inside the
settings whenever the button is pressed
we try to make use of the open app
settings method which we get as a result
of the permisson Handler package okay so
this open app settings will take us
directly to the system settings and do
the process of reworking or either
changing the permission based upon the
user choice so let's refresh this
app so consider that we have dened the
request for the first time and try to
click this button the second time we try
to get this s dialog based upon the
status since there is an exception
occurring here as the has been denied
for opening the device Gallery this cat
block get executed and here inside the
cat block as the permission is denied we
try to S the alert dialog this alert
dialog is going to have two options
either to cancel or open the system
settings to do the manually let's go to
the system settings that will take us
directly to the particular flat app
setting and here you see that since we
have denied the request we have no
access to any of the photos let's click
this and change it to all photos and now
let's move back to our f app and let's
try to click this button again this time
we will no longer see the alert dialogue
as the permission has been granted so
this is how it works the same way for
the camera microphone and all of the
device specific apis therefore with the
help of this permission handlers be able
to keep track of the request and also
educate the user about the Peron
statuses and render the UI components
accordingly thereby making your app more
intuitive for the users hope you guys
found this tutorial useful if you do so
consider subscribing and I will see you
in the next video
a
[Music]
Weitere ähnliche Videos ansehen
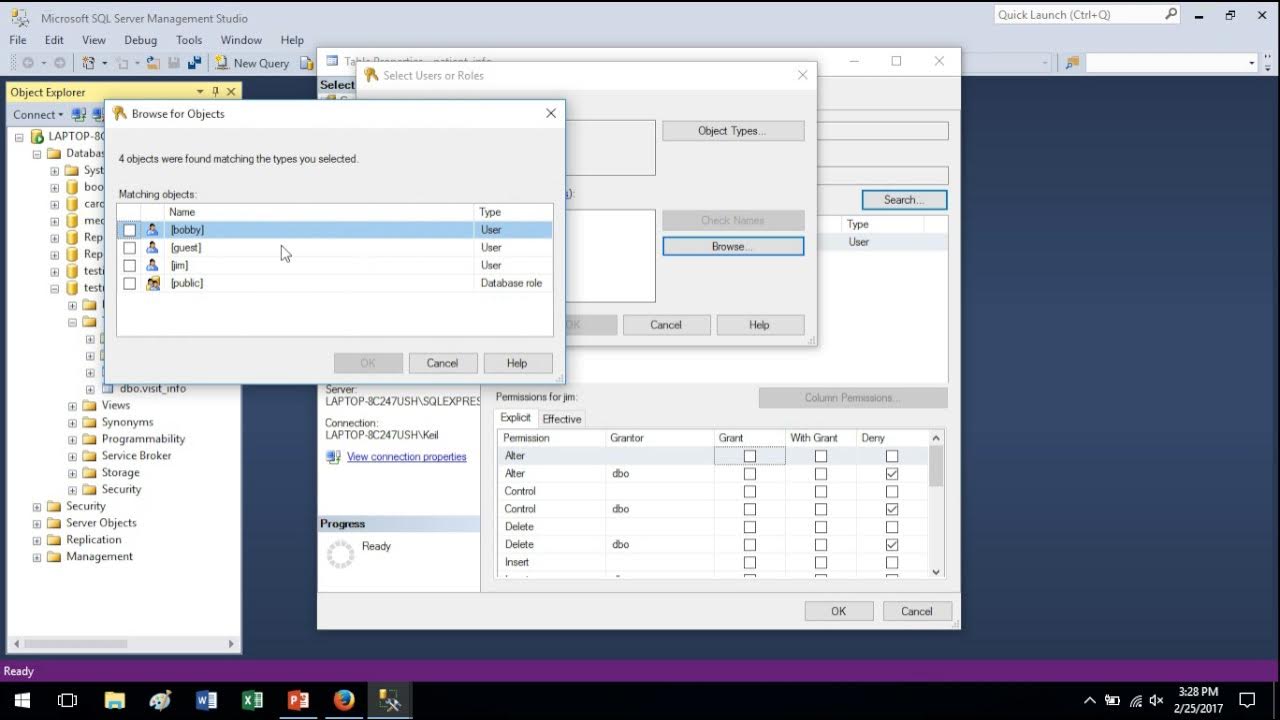
Quick Tutorial - Users and Permissions in SQL Server
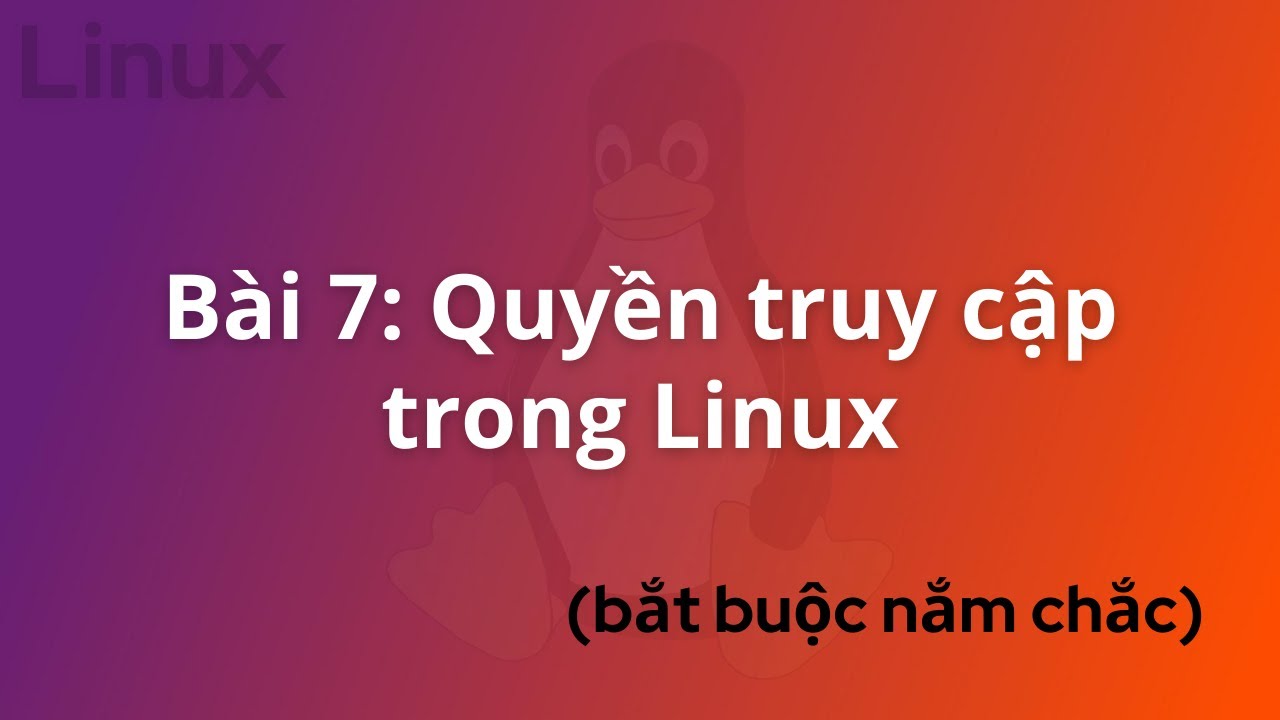
DevOps for Freshers | Bài 7: Quyền truy cập trong Linux | DevOps cho người mới bắt đầu
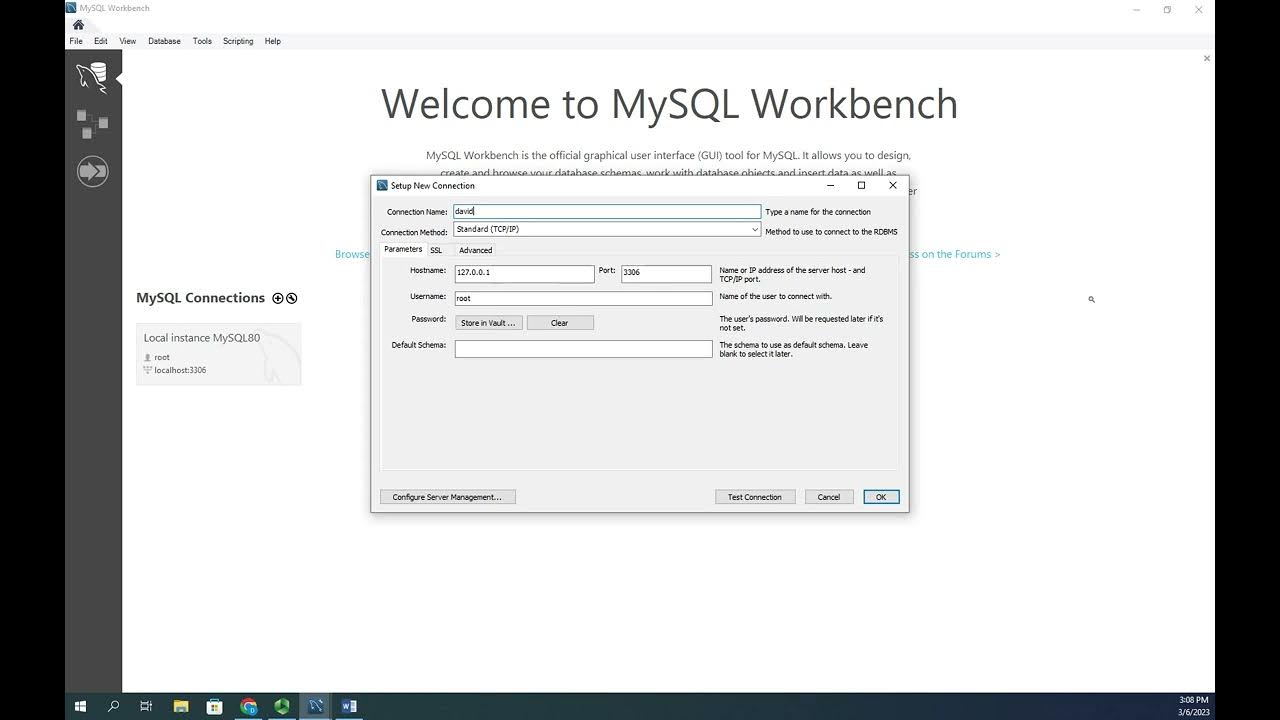
Creating and granting permission to MySQL users

COMO USAR A DIGISAC PSICÓLOGAS PSICOGATTI LTDA
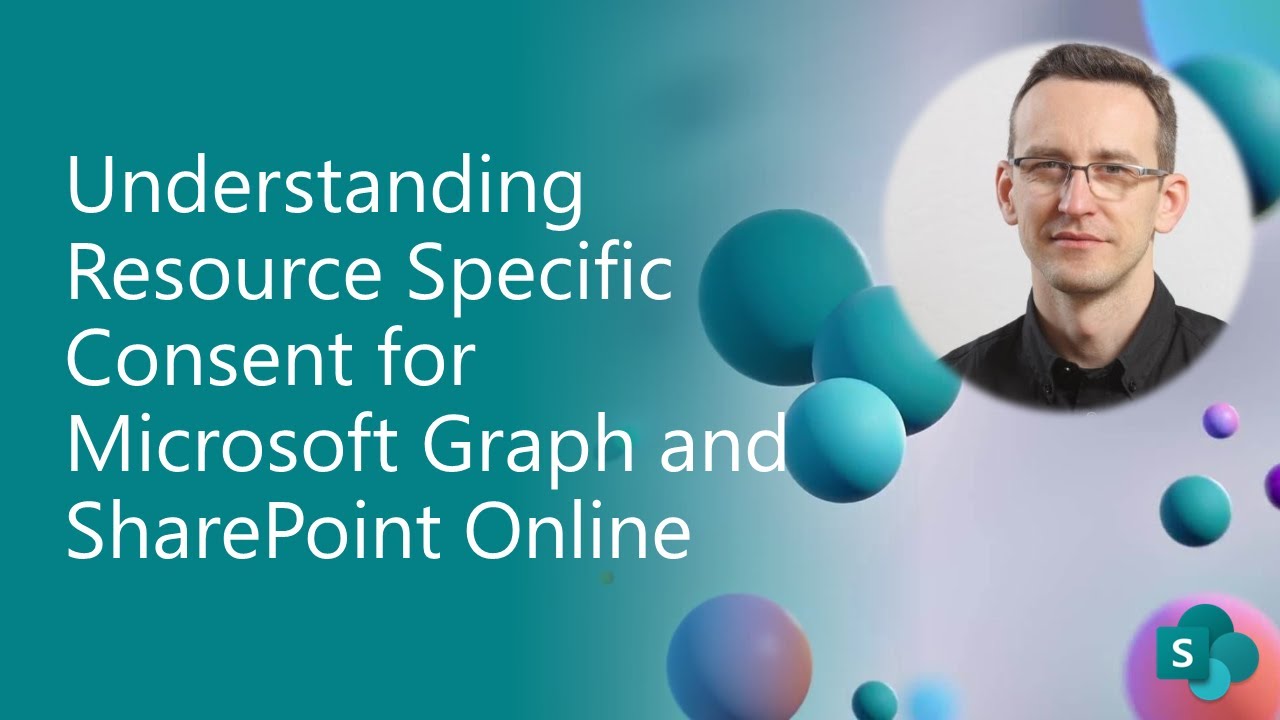
Understanding Resource Specific Consent for Microsoft Graph and SharePoint Online
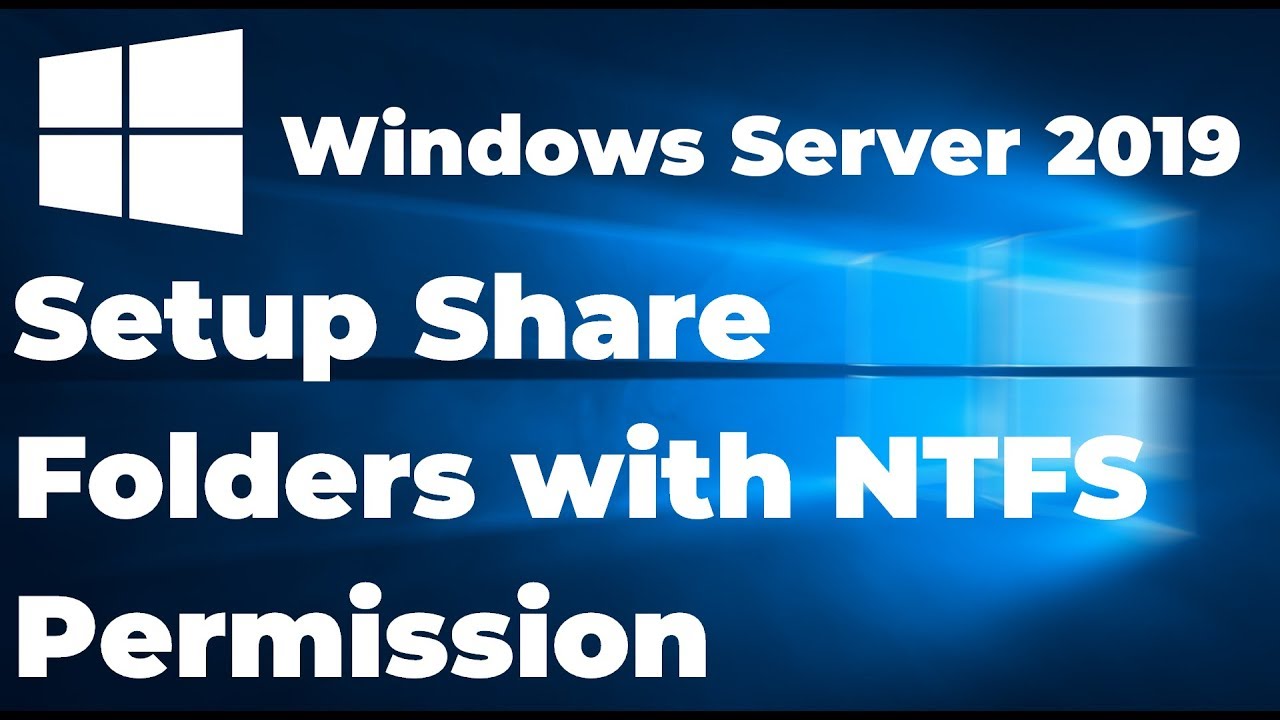
Setup Share Folders with NTFS Permission in Windows Server 2019
5.0 / 5 (0 votes)