Class Methods in Python | How to add Methods in Class | Python Tutorials for Beginners #lec87
Summary
TLDRThis video tutorial explains how to add methods to a class in Python, building upon previous lessons on attributes and object creation. It highlights the difference between attributes (the characteristics of an object) and methods (the actions an object can perform). The video demonstrates how to define and use the `__init__` method to initialize attributes and explains the importance of the `self` keyword in binding attributes and methods to objects. It also covers default values, class object variables, and provides a practical example of adding and updating followers for an instructor class.
Takeaways
- 🛠️ In this video, methods are added to a class in Python, continuing from the previous video on attributes.
- 📜 Attributes represent the data objects possess, such as name, phone number, and address, while methods represent actions that objects can perform.
- 💡 The `__init__` function is introduced, which is used to initialize attributes when creating an object of a class.
- 🏗️ Methods in a class are similar to functions but are attached to objects, making them distinct from standalone functions.
- 🔗 The `self` keyword is crucial in class methods, binding the method to the specific object it is called on, allowing it to access that object's attributes.
- 📝 Class object variables, like `followers = 0`, are shared by all objects of the class, making them accessible without explicitly initializing them for each object.
- 🔄 The method `update_followers` is created to modify object attributes (like increasing a follower count), showing how methods interact with attributes.
- 📥 Parameters passed to class methods don't need to be attributes of the class (e.g., `subject_name`), but attributes (like `self.name`) must use the `self` keyword to access.
- ⚙️ Methods allow objects to perform actions, such as updating attributes based on interactions (like increasing a follower count when someone follows).
- 🤔 Understanding the difference between passing arguments to methods and using attributes is key when designing classes with both attributes and methods.
Q & A
What are the two main components of a class in Python?
-The two main components of a class in Python are attributes and methods. Attributes represent the properties of the object (e.g., name, address), while methods represent the actions the object can perform (e.g., teaching).
What is the purpose of the __init__ method in a Python class?
-The __init__ method is a constructor used to initialize the attributes of a class when an object is created. It assigns values to the attributes and ensures the object is properly set up with the required properties.
Why is the 'self' keyword important in Python class methods?
-The 'self' keyword refers to the instance of the class on which the method is being called. It allows access to the attributes and methods of the specific object, ensuring that operations are applied to the correct instance.
What is the difference between a class attribute and an instance attribute?
-A class attribute is shared by all instances of the class, while an instance attribute is specific to each object. In the example, 'followers' is a class attribute (shared by all objects), whereas 'name' and 'address' are instance attributes (specific to each object).
How can you call a method of a class in Python?
-To call a method of a class, you use the object name followed by the method name. For example, 'instructor1.display()' calls the 'display' method on the 'instructor1' object.
What happens if you don't provide a return statement in a method?
-If a return statement is not provided, the method will automatically return 'None'. This is why in the script, the output included 'None' until the return statement was adjusted.
Why did the script produce an error when 'self.subject_name' was used instead of 'subject_name'?
-The error occurred because 'subject_name' was passed as a parameter, not an attribute of the class. 'self' is only used to access attributes of the object, and 'subject_name' was just a local variable in the method.
What is the purpose of the 'update_followers' method in the script?
-The 'update_followers' method increases the follower count of an instructor by one each time it's called, simulating someone starting to follow that instructor.
What is the significance of default values in class attributes?
-Default values in class attributes, such as 'followers = 0', ensure that every object starts with the same value for that attribute unless explicitly changed. This avoids needing to pass the same value every time an object is created.
How can the script be extended to handle both 'followers' and 'following' counts for an instructor?
-The script can be extended by adding a new attribute, 'following', to track how many people the instructor is following. A corresponding method could be added to update the 'following' count whenever the instructor starts following someone.
Outlines
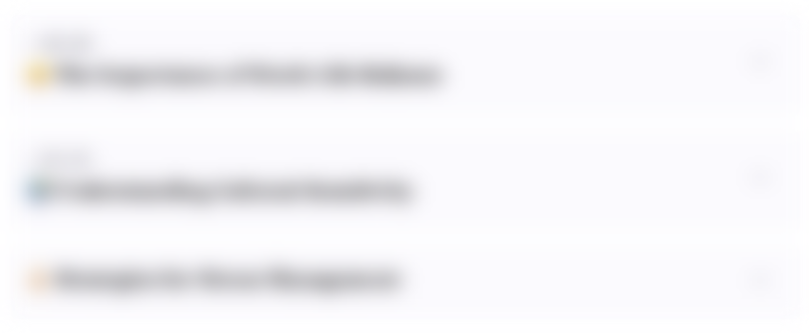
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
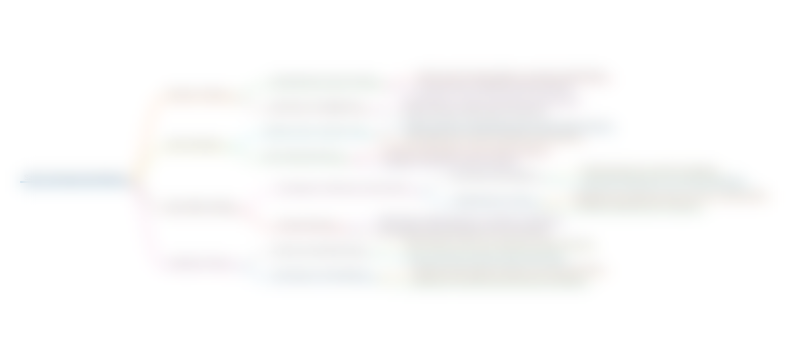
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
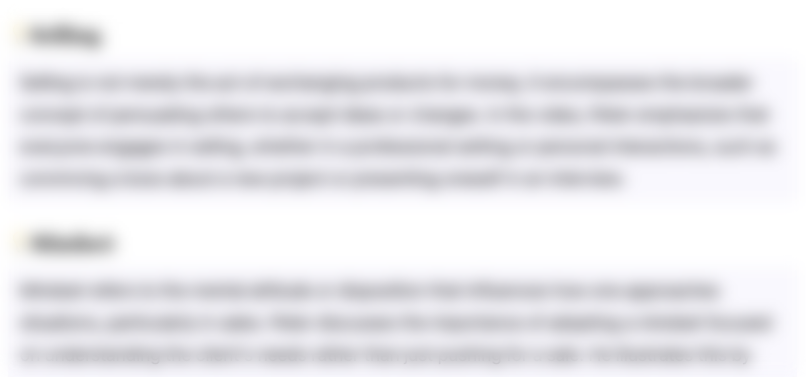
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
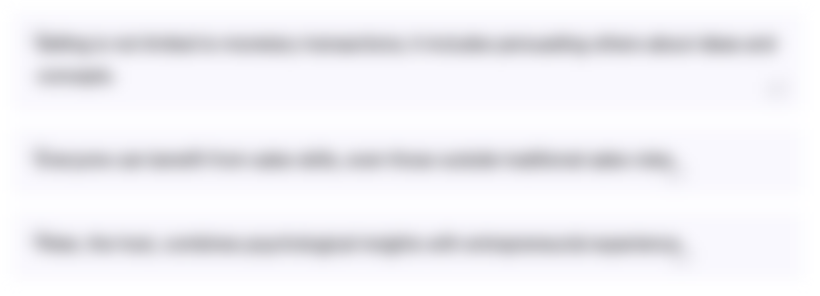
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
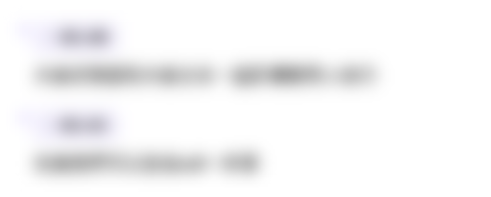
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenWeitere ähnliche Videos ansehen

Self and __init__() method in Python | Python Tutorials for Beginners #lec86
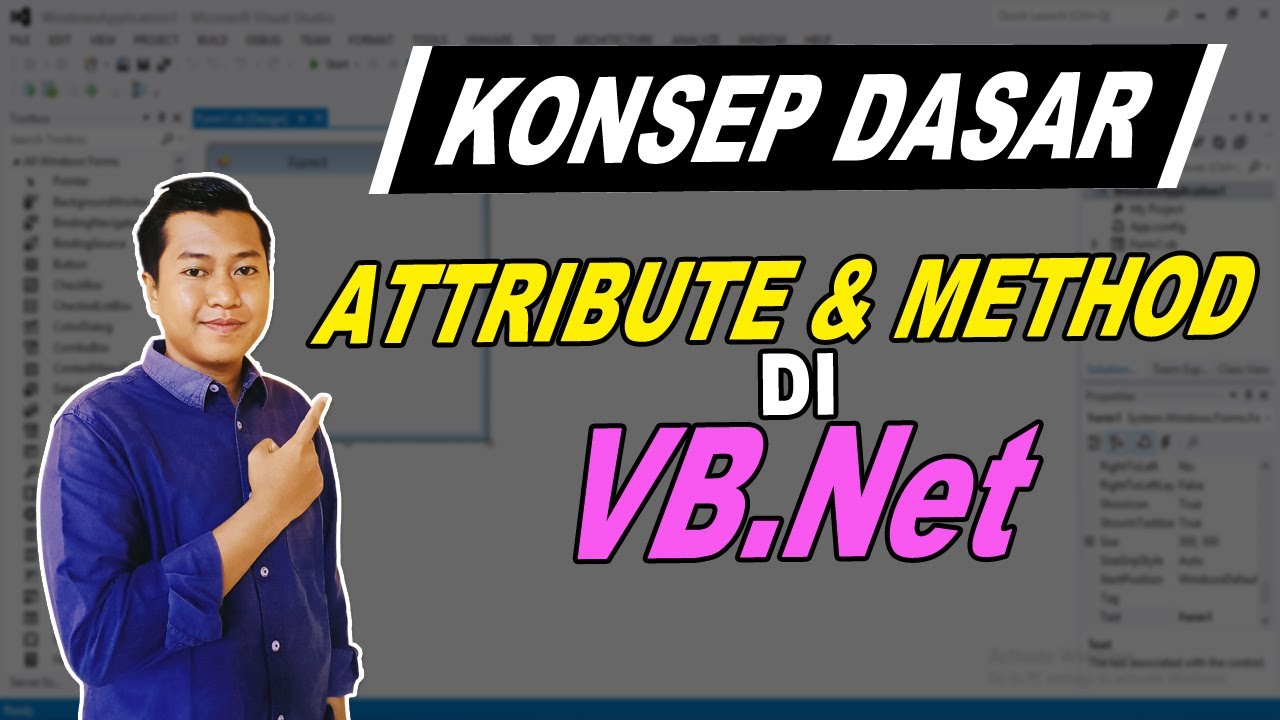
Memahami Konsep OOP di VB.Net: Penjelasan dan Contoh Attribute / Property & Method / Behavior
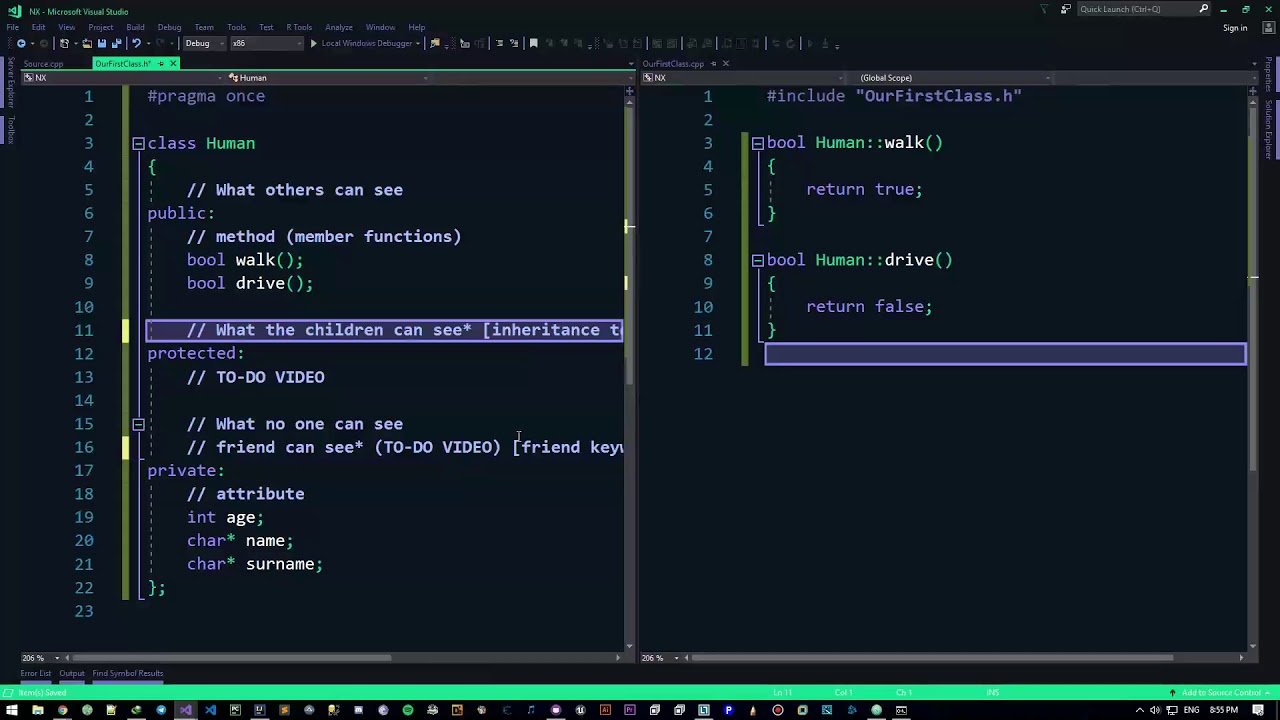
01 - [Classes] C++ Intermediate Programming Tutorial

Classes and Objects in Python | OOP in Python | Python for Beginners #lec85
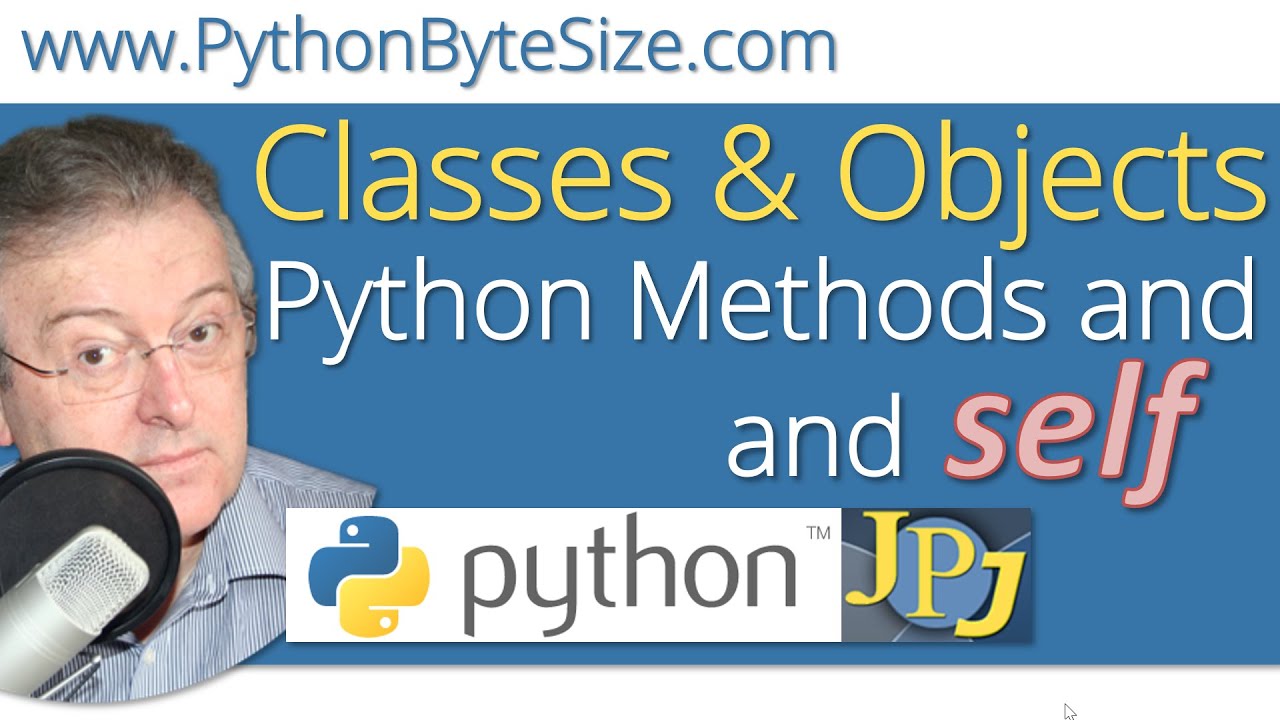
Python Methods and self

Python OOP Tutorial 1: Classes and Instances
5.0 / 5 (0 votes)