Self and __init__() method in Python | Python Tutorials for Beginners #lec86
Summary
TLDRIn this video, the speaker continues their Python Object-Oriented Programming series by discussing how to add attributes and initialize objects in a class using the `__init__` method. They explain the role of constructors, the `self` keyword, and how to set default values for attributes like Instagram followers. The video emphasizes simplifying object creation by reducing code lines and concludes with an assignment for viewers to practice creating new objects with attributes. The next video will cover adding methods to the class.
Takeaways
- ๐ The video continues a Python programming series focusing on object-oriented programming.
- ๐งโ๐ซ The instructor discusses creating classes and objects, building on concepts from the previous video.
- ๐๏ธ The key topic in this video is adding attributes to a class and introducing the `__init__` method, which serves as a constructor.
- ๐ The `self` keyword is explained as a way to reference the current object when creating attributes or methods within a class.
- ๐ก The purpose of the `__init__` method is to initialize the attributes of an object automatically when the object is created.
- ๐ Each time an object is created, the `__init__` method is called to set up the initial state of that object with the given parameters.
- ๐ Examples are provided on how to add attributes like `name`, `address`, and `followers` to a class and initialize them using the constructor.
- โ The instructor emphasizes that attributes must be accessed using `object.attribute` syntax and demonstrates how this works.
- ๐ Default values for attributes (like setting default Instagram followers to 0) can be added, reducing the need for repetitive code.
- ๐ An assignment is given at the end, asking viewers to create more instructors with name and address attributes, and print their information.
Q & A
What is the purpose of the `__init__` method in Python classes?
-The `__init__` method in Python is a special function that acts as a constructor. Its primary purpose is to initialize the attributes of a class when an object is created.
Why is the `self` keyword used in class methods?
-The `self` keyword is used to refer to the current instance of the class. It allows the method to access and modify the object's attributes and ensures the method operates on the correct object.
How do you assign attributes to an object of a class?
-Attributes can be assigned directly to an object using dot notation, like `object.attribute = value`. Alternatively, attributes can be initialized inside the `__init__` method by assigning values to `self.attribute`.
What happens if you don't pass any parameters to the `__init__` method?
-If no parameters are passed to the `__init__` method, and it expects them, Python will raise a `TypeError`, stating that required positional arguments are missing.
What is the advantage of setting default values for parameters in the `__init__` method?
-Setting default values for parameters allows you to create objects without providing values for those parameters. This is useful when certain attributes are optional or have a standard default value.
Can you modify the attributes of an object after it has been created?
-Yes, attributes of an object can be modified after its creation using dot notation, such as `object.attribute = new_value`.
What is the difference between a class attribute and an instance attribute?
-An instance attribute is specific to each object of a class and is set using `self.attribute`, while a class attribute is shared across all instances of the class.
What does the error 'missing required positional argument' indicate?
-This error occurs when a function or method, like `__init__`, is called without providing the necessary arguments it expects, leading to an incomplete call.
Why is using a class beneficial for managing multiple similar objects?
-Using a class allows for better organization and reusability of code, as it provides a blueprint for creating multiple objects with similar attributes and methods, reducing repetition and potential errors.
How would you create a new object with attributes in a Python class?
-To create a new object with attributes, define the class with an `__init__` method that initializes the attributes. Then, create an object by calling the class and passing the necessary parameters to the `__init__` method.
Outlines
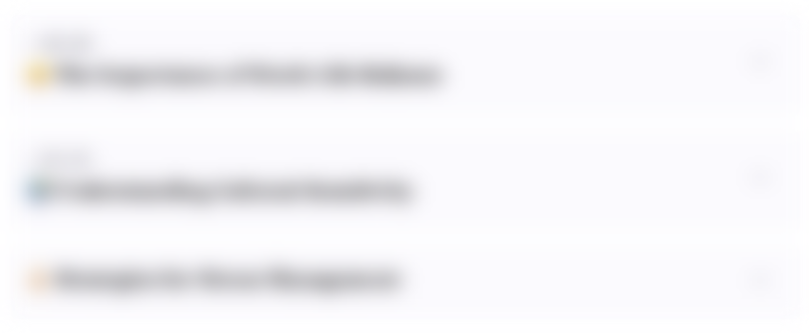
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
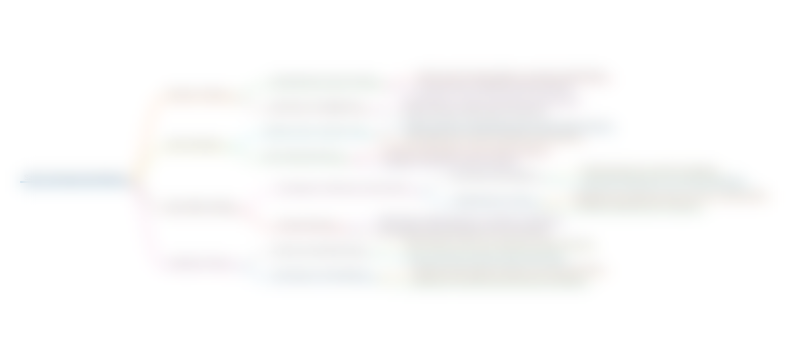
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
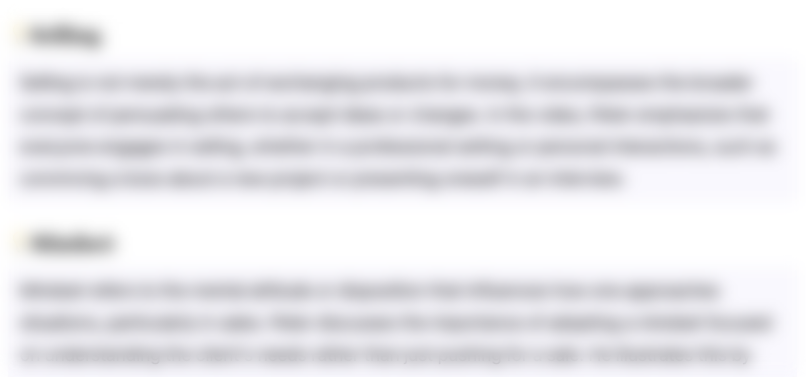
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
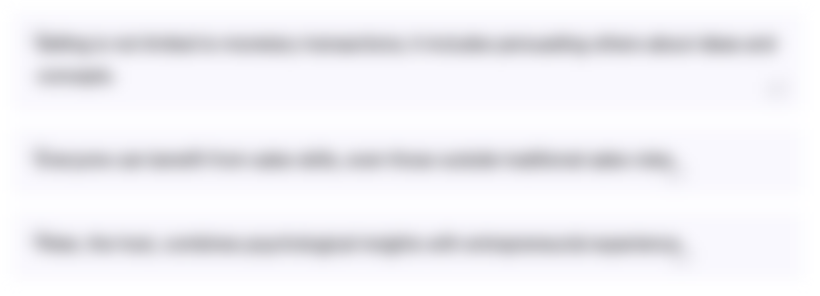
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
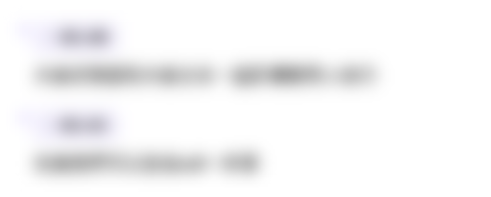
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
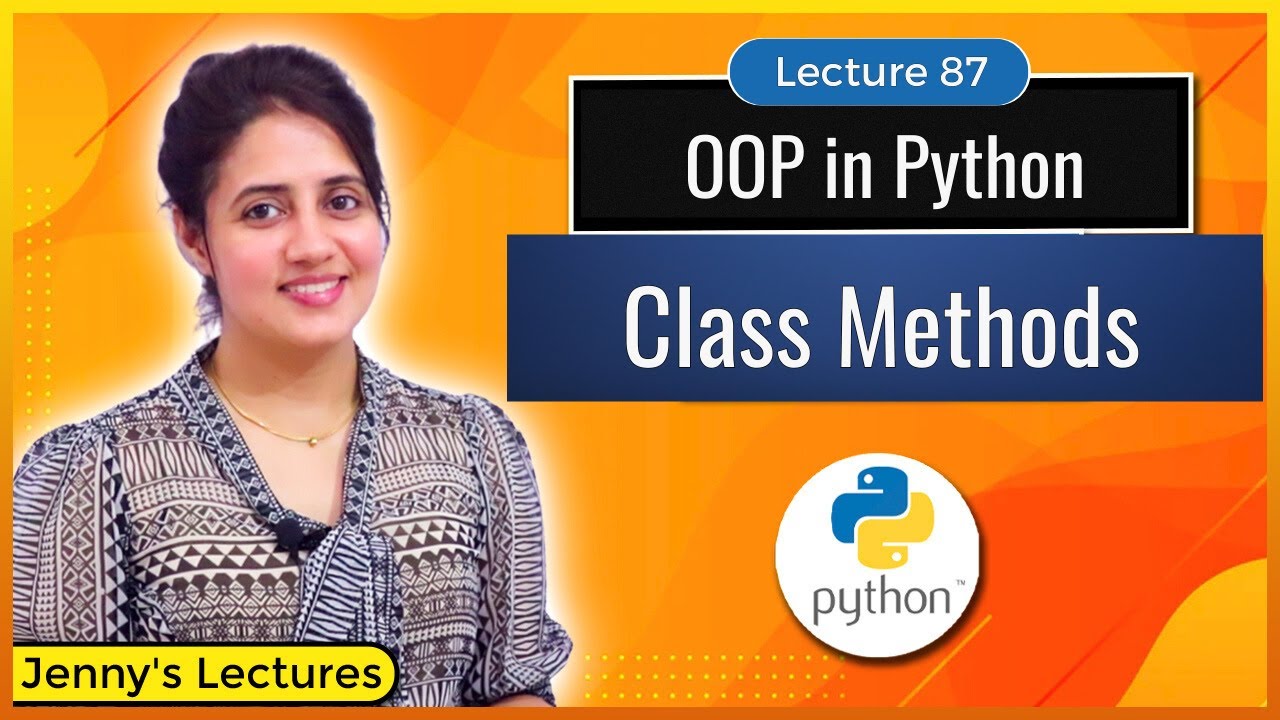
Class Methods in Python | How to add Methods in Class | Python Tutorials for Beginners #lec87
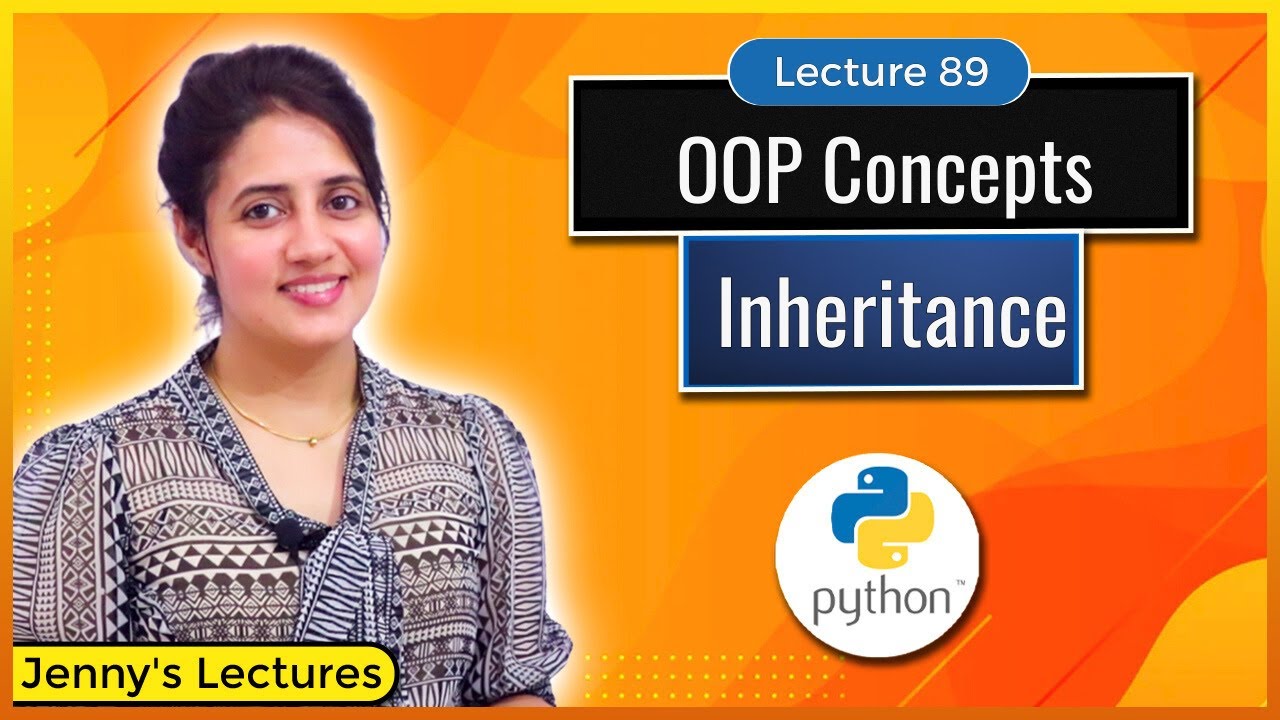
Inheritance in Python | Python Tutorials for Beginners #lec89

Object Oriented Programming Dasar pada C++ (Class, Object, Method, dan Constructor)

Criando Mรฉtodos de Objetos - Curso Python Orientado a Objetos [Aula 03]
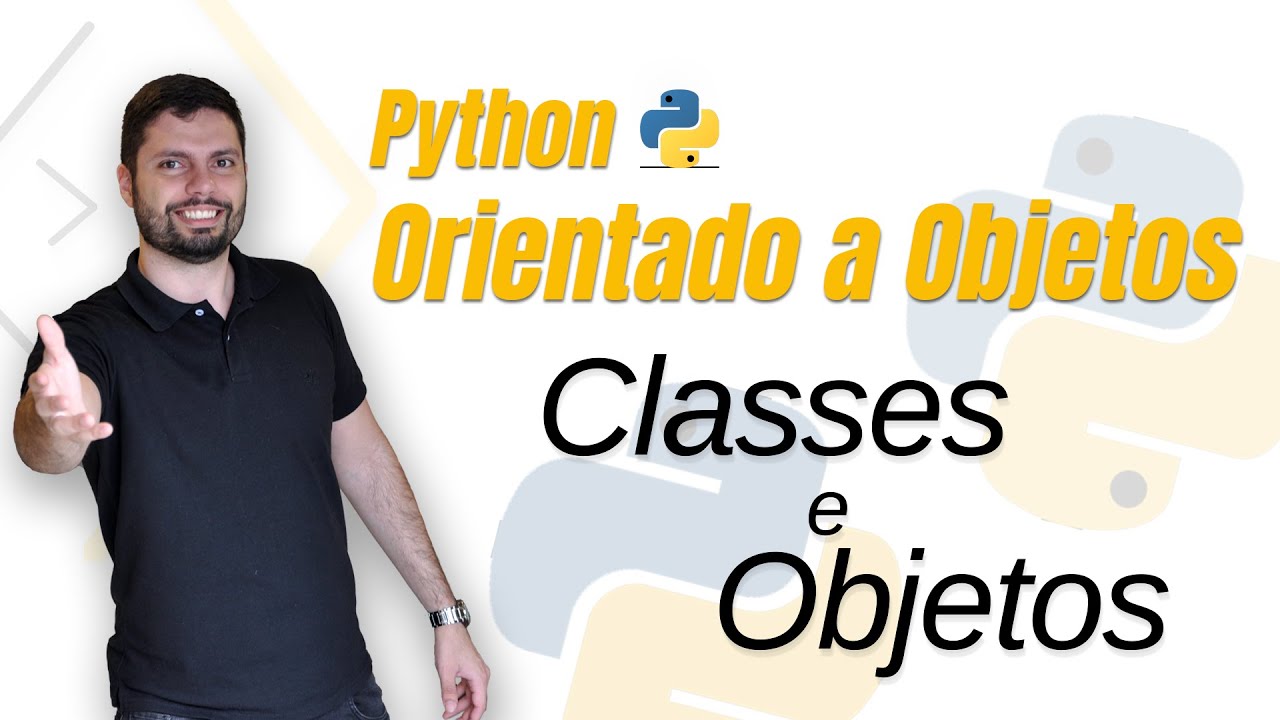
Como criar Classes e Objetos - Curso Python Orientado a Objetos [Passo a Passo]
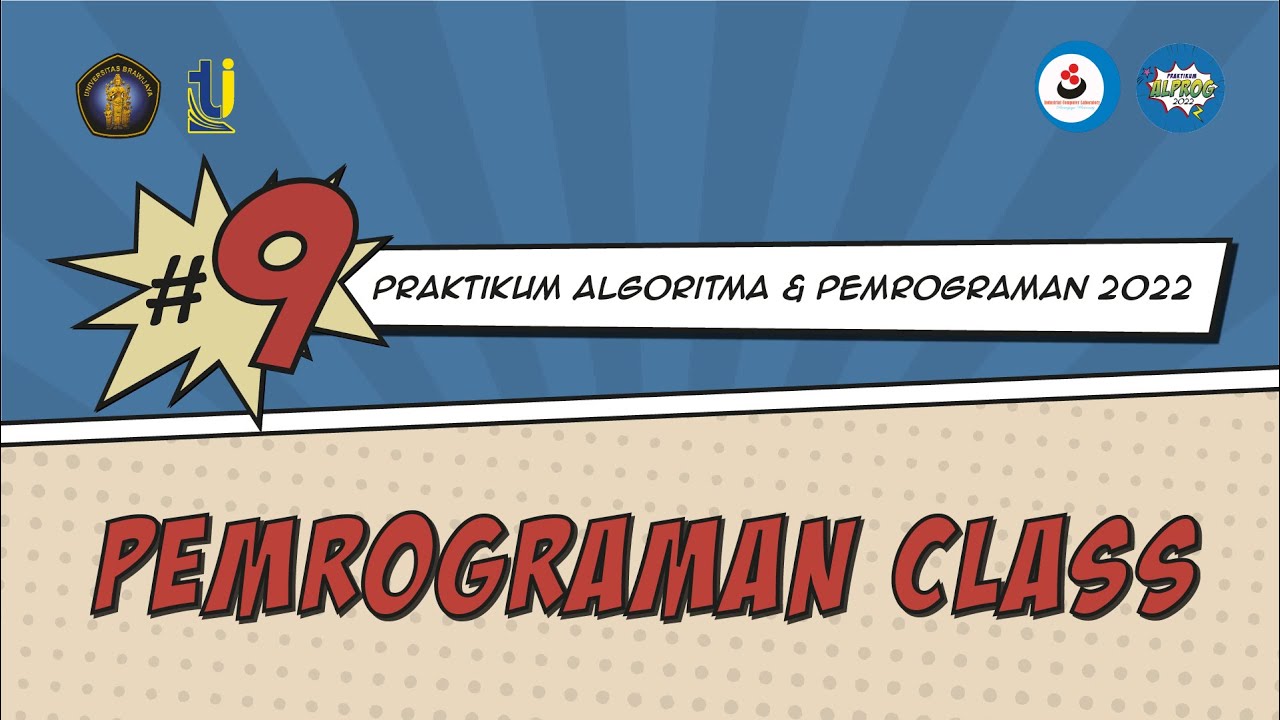
9. Pemrograman Class - Praktikum Algoritma dan Pemrograman 2022
5.0 / 5 (0 votes)