Variables in C++
Summary
TLDRIn this video, Cherno explores the fundamentals of variables in C++ programming. He explains how variables are used to store and manipulate data, highlighting the importance of understanding memory allocation in the stack or heap. Cherno covers various primitive data types like int, char, float, and bool, discussing their memory sizes and typical use cases. He also touches on the concept of signed and unsigned integers, and the significance of data type sizes in programming. The video is an informative introduction to variable usage in C++, setting the stage for deeper dives into pointers and references in future episodes.
Takeaways
- 📝 Variables in C++ are used to store data that can be manipulated, read, and written in a program.
- 💾 Variables are stored in memory, either on the stack or the heap, with the exact location depending on the programming context.
- 🔢 C++ provides primitive data types which are the building blocks for storing data, each with a specific size determining how much memory they occupy.
- 👉 The 'int' data type is typically four bytes in size and can store integer values ranging from approximately -2 billion to 2 billion.
- 🔄 The size of data types can vary depending on the compiler used, but the 'int' type commonly represents a signed integer with a 32-bit size.
- ➕ By using 'unsigned' before an integer type, you can create an unsigned integer that can store larger positive values without a sign bit.
- 🔤 The 'char' type is traditionally one byte and used for storing characters, but it can also hold numeric values corresponding to ASCII codes.
- 🔢 Other integer types include 'short', 'long', and 'long long', each with varying sizes and capable of holding different ranges of integer values.
- 📉 For storing decimal values, C++ offers 'float' and 'double', with 'float' being four bytes and 'double' eight bytes, and 'double' being the default for decimal values if not specified.
- 🔴 The 'bool' type represents Boolean values and occupies one byte, using 1 for true and 0 for false, despite the actual value only requiring one bit.
- 🔍 The 'sizeof' operator in C++ can be used to determine the size in bytes of a data type or a variable.
- 🔑 Pointers and references are advanced features in C++ that allow for more complex data manipulation, but they are covered in separate videos due to their complexity.
Q & A
What is the primary purpose of variables in programming?
-Variables are used to store data in memory so that it can be manipulated, read, and written to as needed during the execution of a program.
Where are variables typically stored in a C++ program?
-Variables in C++ can be stored in either the stack or the heap, depending on their declaration and usage.
What is the difference between a signed and unsigned integer in C++?
-A signed integer can represent both positive and negative values, while an unsigned integer can only represent non-negative values. The signed integer uses one bit for the sign, limiting the range, whereas an unsigned integer uses all bits for the value, effectively doubling the positive range.
What is the significance of the 'int' data type in C++?
-The 'int' data type in C++ is used to store integer values and typically occupies four bytes of memory, allowing it to store values from about negative two billion to positive two billion.
How can you determine the size of a data type in C++?
-You can use the 'sizeof' operator in C++ to determine the size of a data type, which will return the number of bytes allocated for that type.
What is the purpose of the 'char' data type in C++?
-The 'char' data type in C++ is typically used for storing characters, but it can also store integer values. It occupies one byte of memory.
Why does the 'bool' data type in C++ occupy one byte of memory?
-The 'bool' data type occupies one byte of memory because memory is addressed in bytes, not bits. This allows for easier memory management and access, even though a boolean value technically only requires one bit.
How do you declare a variable of type 'float' in C++?
-To declare a variable of type 'float' in C++, you append an 'f' or 'F' to the numerical value, such as '5.5f', to indicate that it is a floating-point number of type 'float'.
What is the difference between 'float' and 'double' in C++?
-A 'float' in C++ typically occupies four bytes and has a smaller range and precision compared to a 'double', which occupies eight bytes and offers a larger range and higher precision.
What are pointers and references in C++, and how are they declared?
-Pointers in C++ are declared with an asterisk '*' next to the data type, and references with an ampersand '&'. They are advanced features used for direct memory access and aliasing variables, respectively.
Outlines
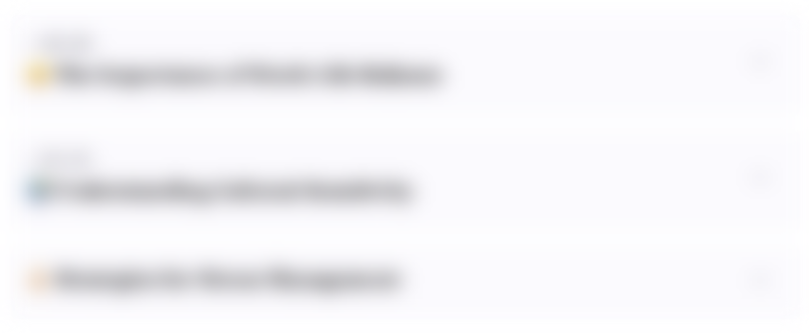
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
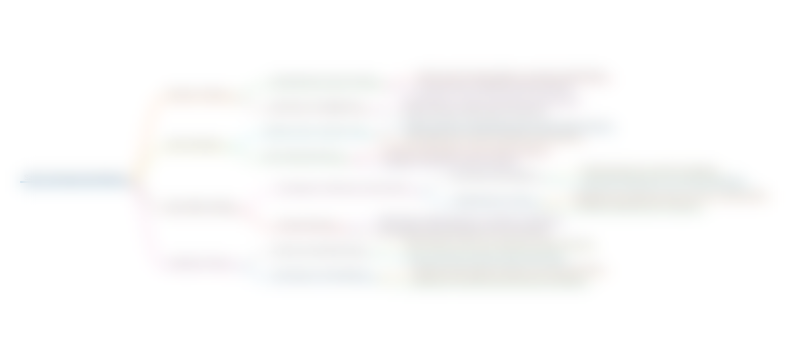
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
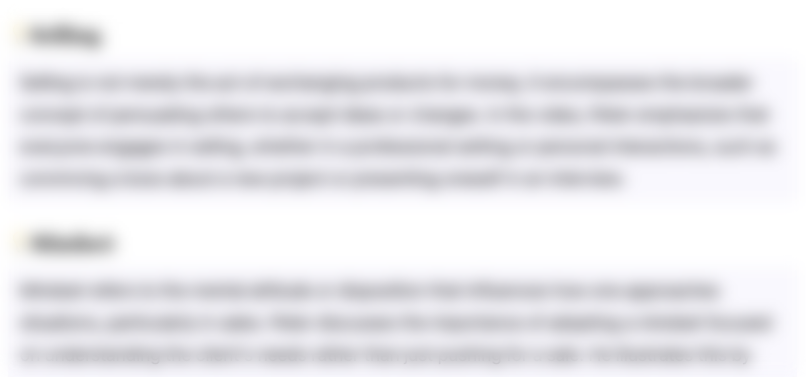
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
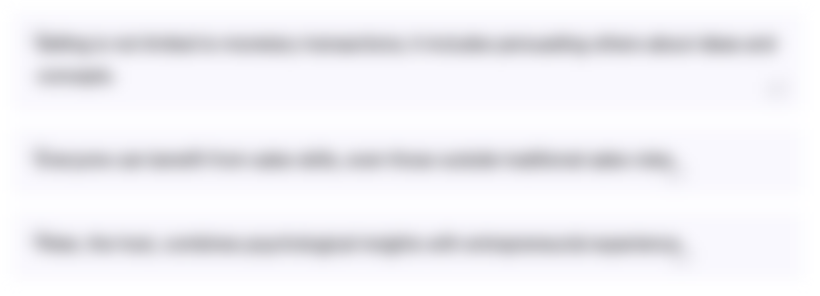
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
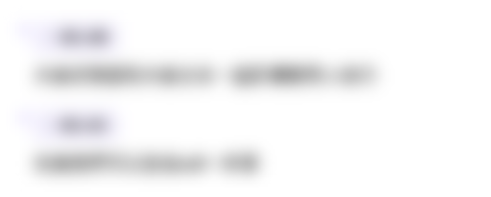
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenWeitere ähnliche Videos ansehen

Variables & Data Types In C: C Tutorial In Hindi #6
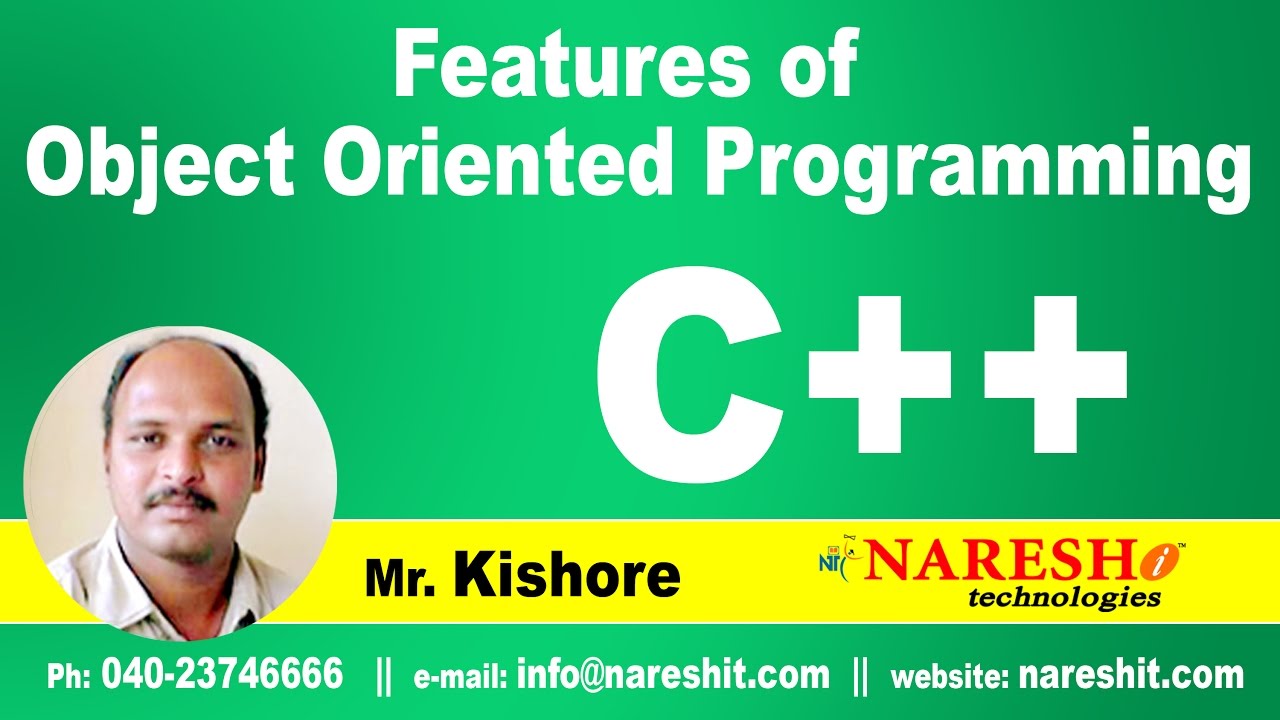
Object Oriented Programming Features Part 3 | C ++ Tutorial | Mr. Kishore
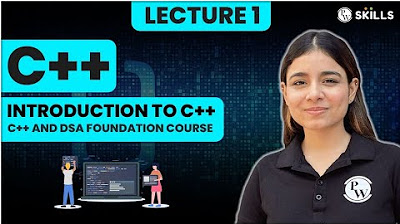
Introduction to C++ | Lecture 1 | C++ and DSA Foundation Course
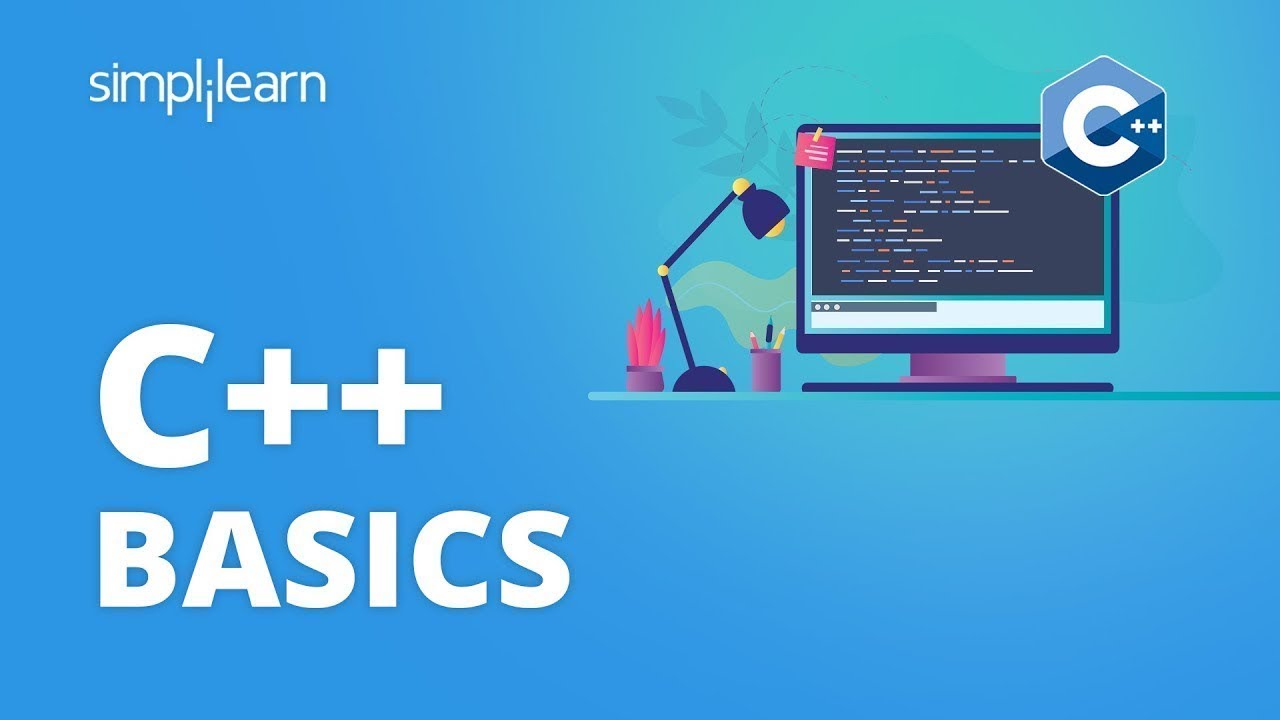
C++ Tutorial For Beginners | C++ Programming | C++ | C++ Basics | C++ For Beginners | Simplilearn
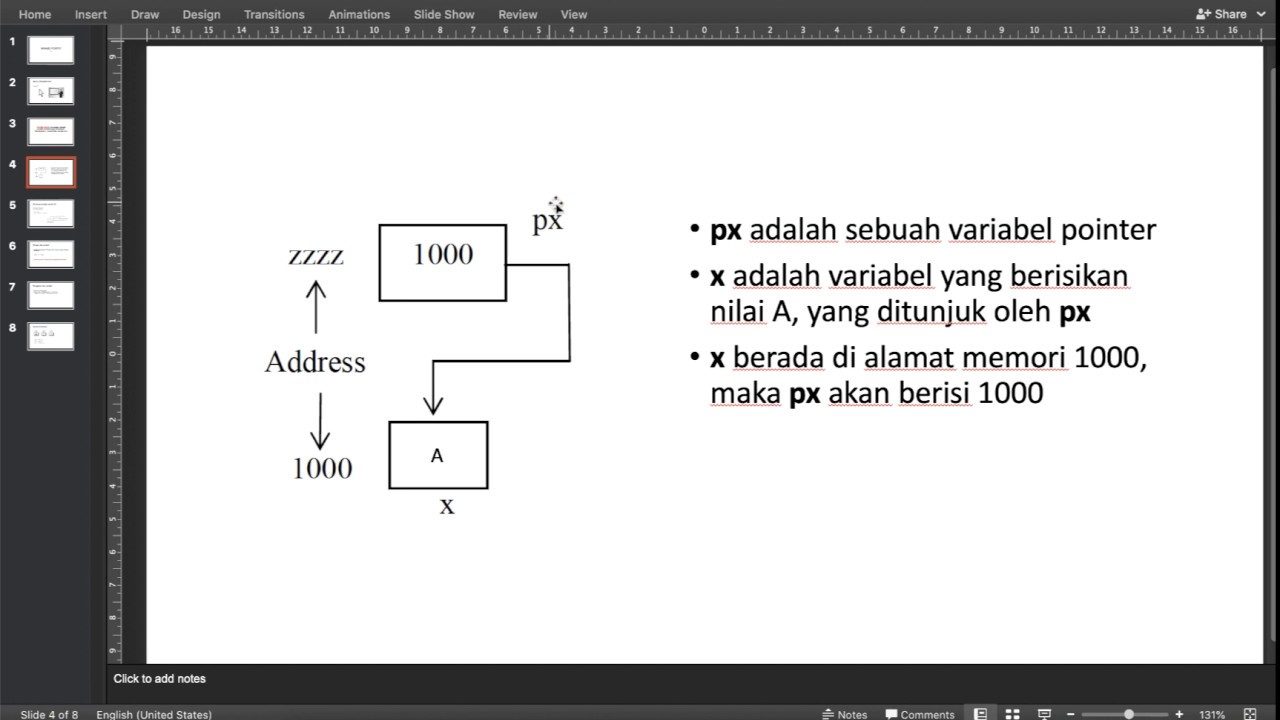
Variabel Pointer
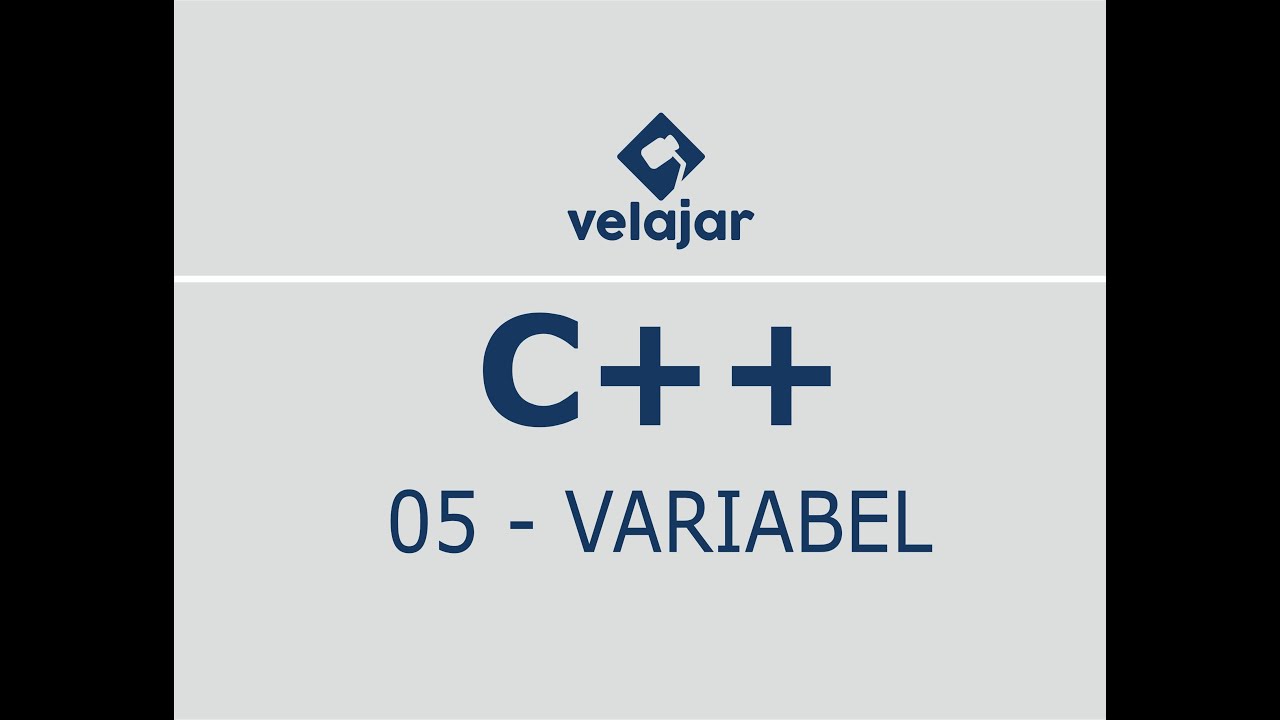
Variabel #5 | C++ | Bahasa Indonesia
5.0 / 5 (0 votes)