#69. How To Use Project Lombok To Remove Boilerplate code Getter And Setter Methods From POJO Class
Summary
TLDRIn this tutorial, the speaker, Amudh, introduces Lombok, a Java library that automates the creation of boilerplate code such as getters and setters. By demonstrating the manual process of generating these methods in a POJO class, the video highlights the tediousness of this task. The speaker then shows how to integrate Lombok into a Maven project, explaining the installation of the Lombok plugin for IDEs like Eclipse. After installation, the video illustrates how Lombok annotations can be used to automatically generate getter and setter methods, streamlining the development process and reducing code redundancy.
Takeaways
- 😀 The video is about using Lombok to reduce boilerplate code in Java POJO classes.
- 🛠 Lombok is a library that can automatically generate getters, setters, and other methods when new fields are added or removed from a class.
- 📦 To use Lombok, one needs to add it as a dependency in their Maven project and also install the Lombok plugin in their IDE.
- 🔍 The video provides a demonstration of how to add Lombok to a project and use it to automatically generate getters and setters.
- 📝 The script explains the manual process of creating getters and setters before introducing Lombok as a solution.
- 🔑 Lombok annotations like `@Getter` and `@Setter` can be used to specify the generation of only getters or setters as needed.
- 🔄 The video shows how Lombok handles the addition and removal of fields in a class without requiring manual updates to the getter and setter methods.
- 🔍 The presenter mentions that Lombok is particularly useful in reducing lines of code and avoiding repetitive tasks.
- 🔗 The video script includes instructions on how to install the Lombok plugin for Eclipse IDE, either through the marketplace or by manually downloading the `lombok.jar`.
- 📌 The script also mentions that Lombok will be covered in more depth in future videos, including its builder feature for creating JSON bodies.
- 📢 The video concludes with an invitation for viewers to comment, like, subscribe, and share if they found the content helpful.
Q & A
What is the main purpose of the Lombok library discussed in the video?
-The main purpose of the Lombok library is to eliminate the need for manually creating boilerplate code such as getters and setters in Java classes, thus reducing the lines of code and simplifying the development process.
How does Lombok help in reducing boilerplate code when creating POJO classes?
-Lombok provides annotations that automatically generate getters, setters, and other methods at compile time, so developers do not need to write these methods manually for each field in a class.
What is the process of adding Lombok as a dependency to a Maven project?
-To add Lombok as a dependency to a Maven project, one needs to search for 'lombok' in the Maven Central Repository, find the latest version, and copy the dependency into the project's pom.xml file.
Why is it necessary to install the Lombok plugin in the IDE after adding the Lombok dependency?
-The Lombok plugin is necessary in the IDE to recognize the Lombok annotations and generate the corresponding methods (getters, setters, etc.) during the development process, without requiring explicit method definitions.
What are the two ways to install the Lombok plugin in Eclipse mentioned in the video?
-The two ways to install the Lombok plugin in Eclipse are by downloading the lombok.jar and running it, which opens an installer window, or by using the Eclipse Marketplace if available and working in the user's network environment.
How can the Lombok plugin be installed via the command prompt?
-The Lombok plugin can be installed via the command prompt by navigating to the directory containing the lombok.jar file, and then running the command 'java -jar lombok.jar'. This will launch the Lombok installer window, where the user can specify the IDE installation path and install the plugin.
What is the advantage of using Lombok when adding or removing fields in a class?
-The advantage of using Lombok is that it automatically handles the addition or removal of getters and setters for new or removed fields, without the developer needing to manually update the method definitions.
Can Lombok be used without other dependencies like Jackson in a project?
-Yes, Lombok can be used independently of other dependencies like Jackson. The video mentions Jackson as part of a reactive tutorial, but Lombok's primary function is to reduce boilerplate code and does not require Jackson or any other specific libraries.
What is the 'Builder' pattern mentioned in the video, and how is it related to Lombok?
-The 'Builder' pattern is a design pattern used to create objects step by step, often used in frameworks to construct JSON bodies. Lombok provides an annotation for the Builder pattern, which simplifies object construction and will be discussed in a subsequent video.
What should developers do if they face issues with the Lombok plugin installation via the Eclipse Marketplace?
-If developers face issues with the Lombok plugin installation via the Eclipse Marketplace, they can download the lombok.jar file directly from the Lombok website or the Maven Central Repository and install it manually using the command prompt or by running the jar file.
How can the Lombok library help in reducing the development time for creating POJO classes?
-Lombok library can help in reducing the development time by automatically generating the necessary boilerplate code like getters and setters, allowing developers to focus on the core logic of the application rather than repetitive code tasks.
Outlines
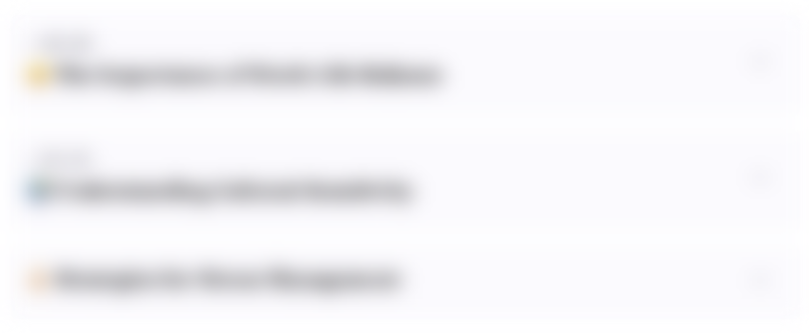
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
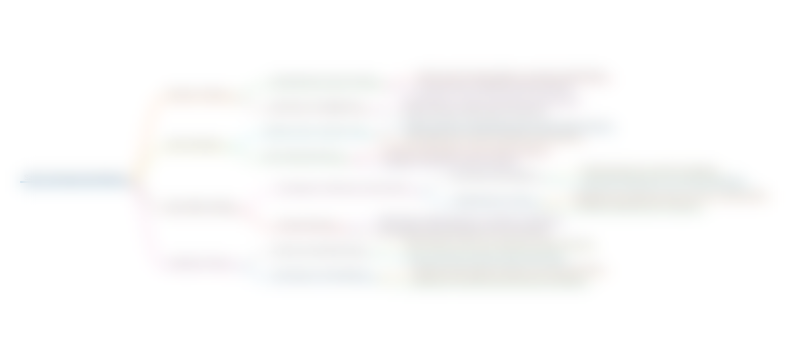
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
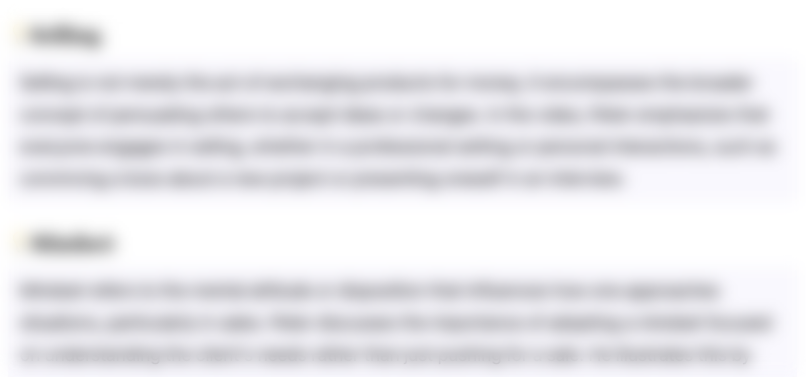
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
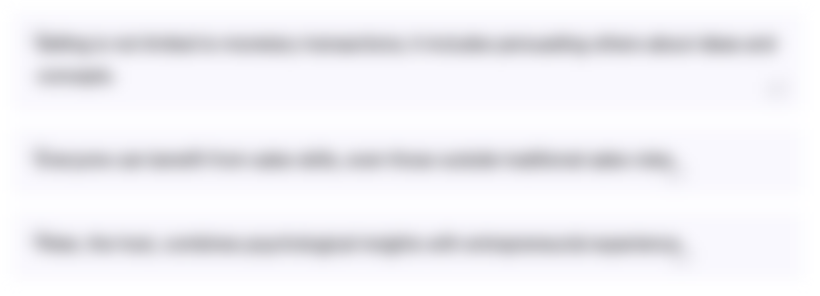
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
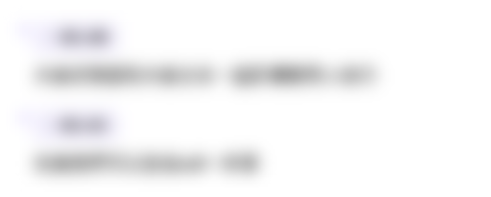
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة

JavaScript Classes #2: Getters & Setters - JavaScript OOP Tutorial
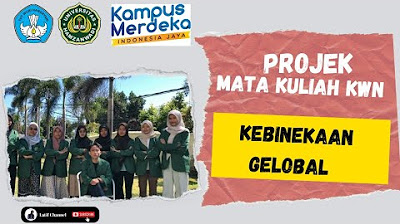
Mata Kuliah Kewarganegaraan berbasis Projek..(MKWK) #universitashamzanwadi #mkwk #budaya
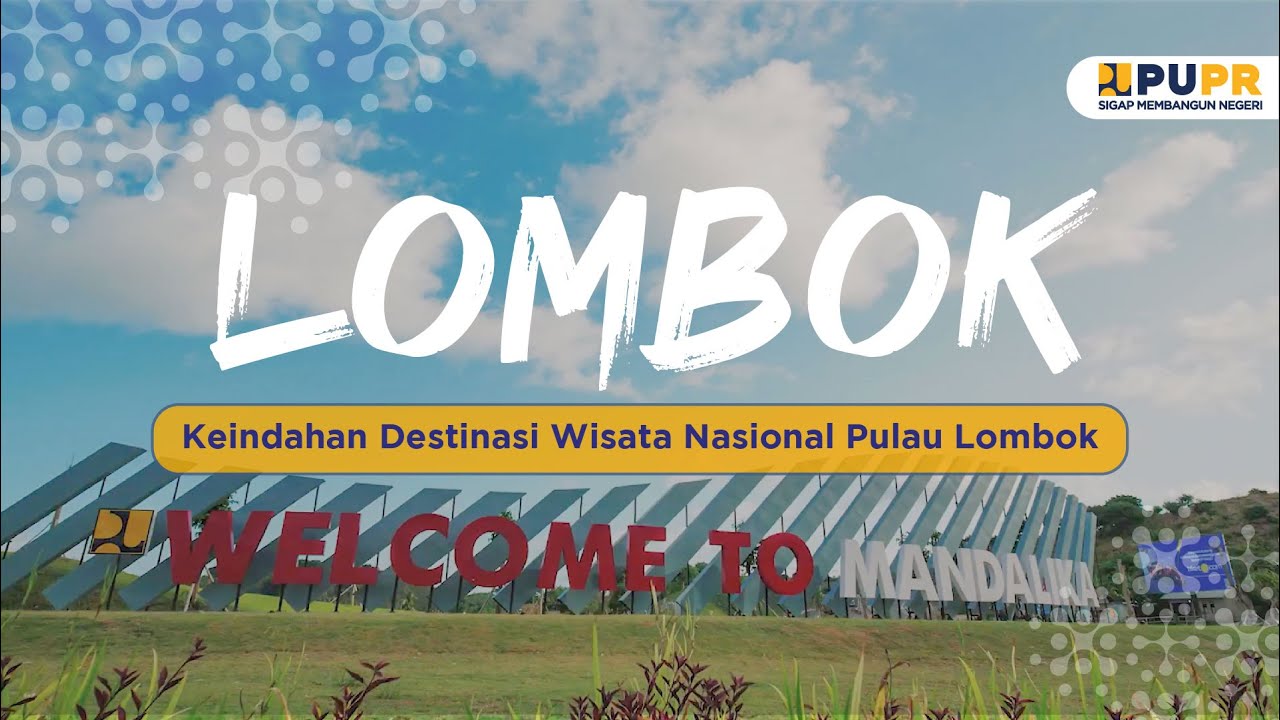
Integrated Tourism Master Plan (ITMP) Lombok
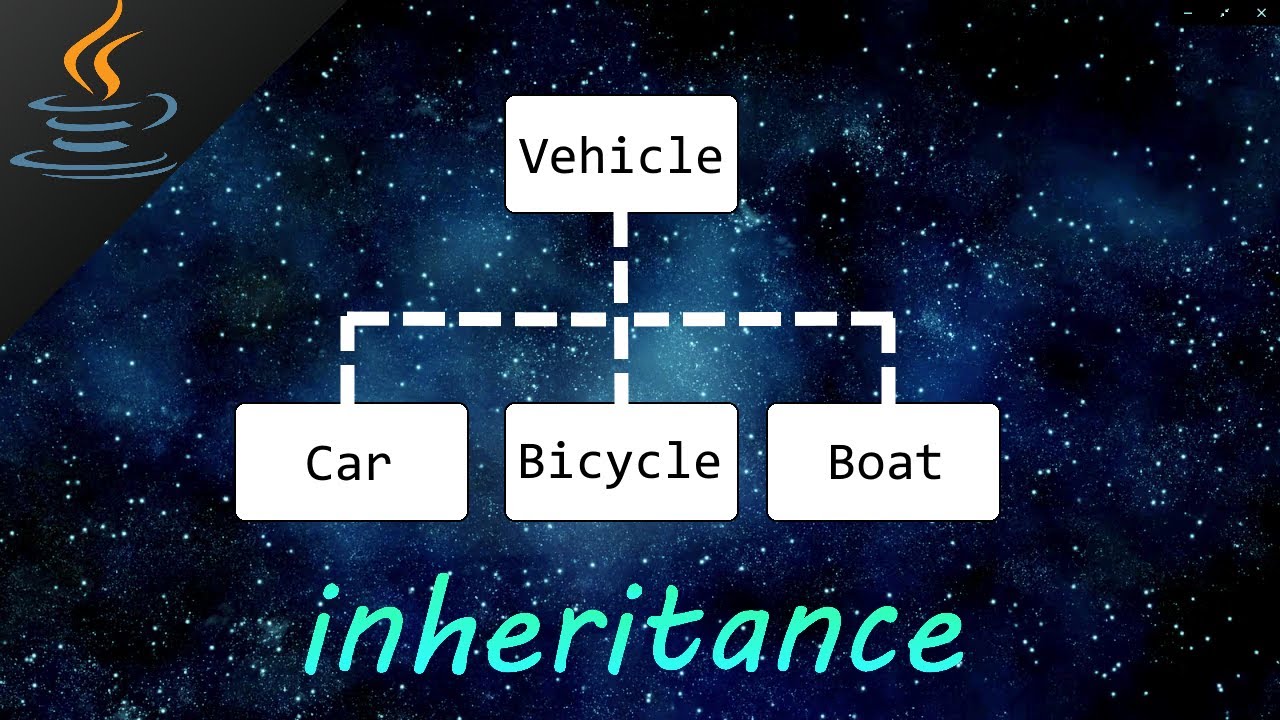
Java inheritance 👪
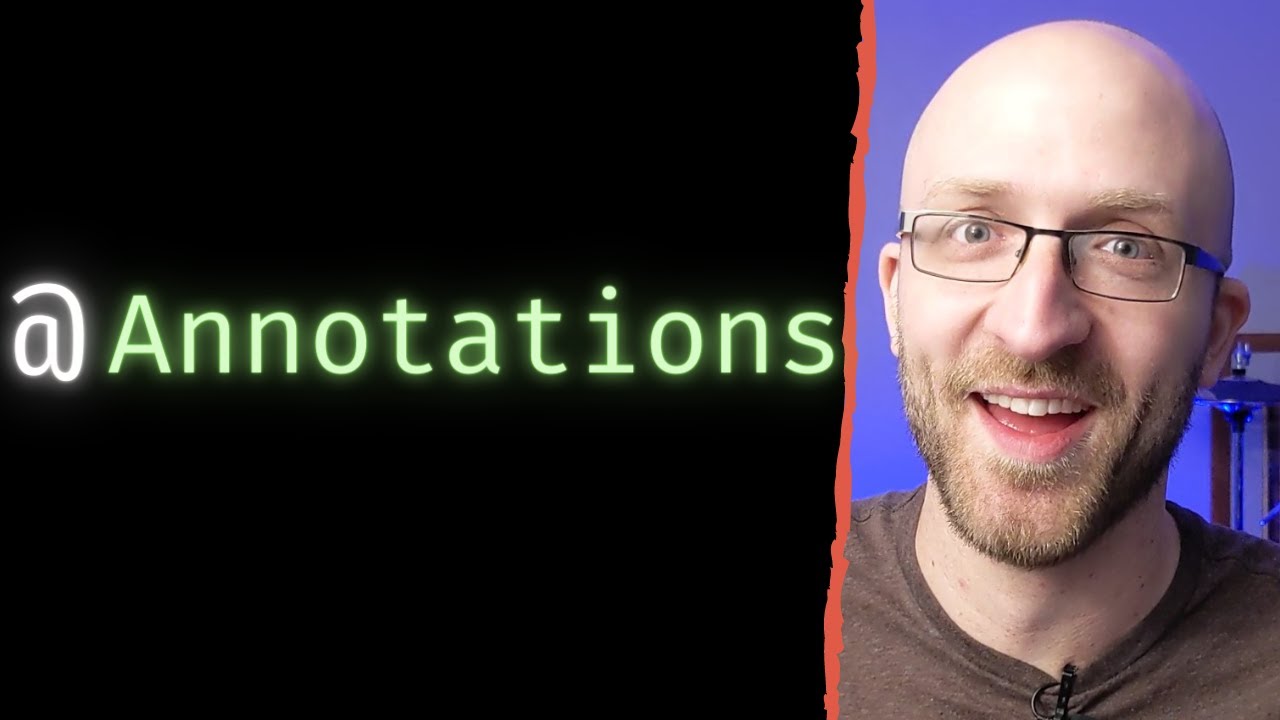
Annotations In Java Tutorial - How To Create And Use Your Own Custom Annotations
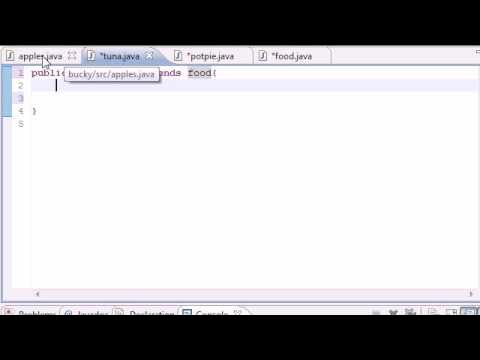
Java Programming Tutorial - 49 - Inheritance
5.0 / 5 (0 votes)