JavaScript Classes #2: Getters & Setters - JavaScript OOP Tutorial
Summary
TLDRIn this JavaScript tutorial, the speaker explains how to use getters and setters within classes to dynamically calculate and modify object properties. Using a 'Square' class as an example, the video demonstrates how to create a getter for area, which behaves like a property, and a setter that adjusts the width and height based on a given area. Additionally, the tutorial shows how to track how many times the area getter has been accessed. This step-by-step guide offers clear, practical examples to help users understand how to implement and use getters and setters in their own code.
Takeaways
- π Getters and setters in JavaScript allow defining methods that act like properties of a class.
- π A getter computes a value based on class properties and can be accessed like a property without parentheses.
- π A setter allows assigning new values to class properties using a property-like syntax.
- π The script demonstrates getters and setters using a `Square` class that calculates and modifies the area of a square.
- π The getter for area is defined using the `get` keyword, which computes the area by multiplying width and height.
- π You can test getters by creating an instance of the class and accessing the getter like a property.
- π The setter for area computes the square root of the value passed and assigns it to both width and height.
- π Using setters, the width and height of a square can be dynamically adjusted based on the area value.
- π The script includes tracking the number of times the getter has been accessed by adding a counter.
- π By calling the getter multiple times, the counter increments, showing how often the area property was requested.
- π The main goal of getters and setters is to provide controlled access to object properties while hiding internal logic.
Q & A
What are getters and setters in JavaScript?
-Getters and setters in JavaScript are special methods that allow you to define and manage properties in a class, while adding custom logic to access or modify their values. A getter retrieves a value, and a setter sets a value, but both appear as regular properties to the outside world.
How do you define a getter in JavaScript?
-A getter in JavaScript is defined using the `get` keyword followed by the name of the property you want to create. Inside the getter method, you write the logic to return the value, which will be treated like a property when accessed.
How does the getter for the 'area' property in the Square class work?
-The getter for the 'area' property in the Square class calculates the area by multiplying the `width` and `height` of the square. Itβs accessed like a property (without parentheses), but under the hood, it executes the function to calculate and return the value.
Can getters take parameters in JavaScript?
-No, getters in JavaScript cannot take parameters. They are designed to return a value based on the internal state of the object, but they are accessed like properties without passing any arguments.
How do you define a setter in JavaScript?
-A setter in JavaScript is defined using the `set` keyword followed by the name of the property you want to modify. The setter method takes one parameter, which represents the value being assigned to the property, and you can perform any additional logic before updating the property.
What is the purpose of the setter for the 'area' property in the Square class?
-The setter for the 'area' property in the Square class allows the user to set the area of the square. It takes the new area as input, calculates the square root to determine the width and height, and updates both dimensions accordingly.
How do you assign a new value to a property using a setter?
-To assign a new value to a property using a setter, you use the property name on an object and assign a value, just like any other property. The setter method is automatically invoked, and it will handle the assignment logic inside.
What happens when the 'area' setter is called with a new value in the example?
-When the 'area' setter is called with a new value, the setter calculates the square root of the area to determine the new width and height of the square. This is done using the `Math.sqrt()` method and then assigns the calculated width and height to the object.
What is the purpose of the 'numOfRequests' variable in the Square class?
-The `numOfRequests` variable in the Square class is used to track how many times the 'area' getter has been accessed. Each time the getter is called, `numOfRequests` is incremented, providing a count of the requests made for the area.
What is the advantage of using getters and setters in object-oriented JavaScript?
-Using getters and setters in object-oriented JavaScript provides a clean and controlled way to access or modify an object's properties. It encapsulates the logic for getting or setting values, ensuring that changes to the objectβs state are handled properly and consistently, without directly exposing internal details.
Outlines
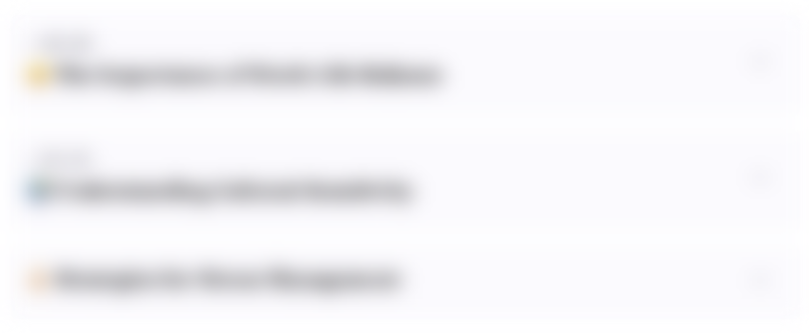
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
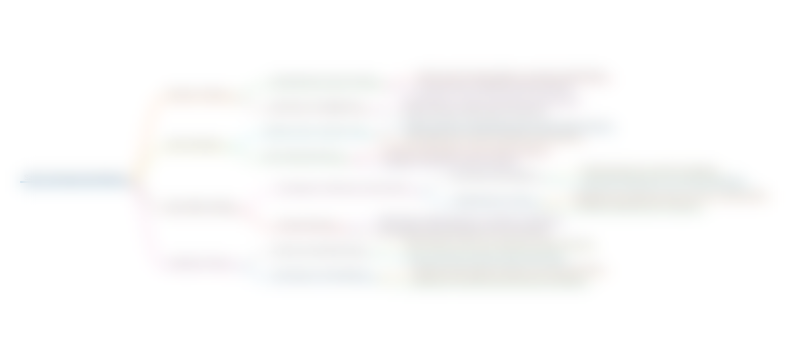
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
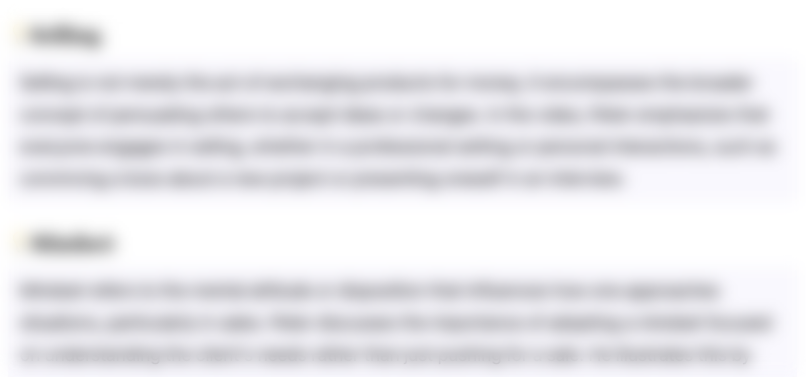
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
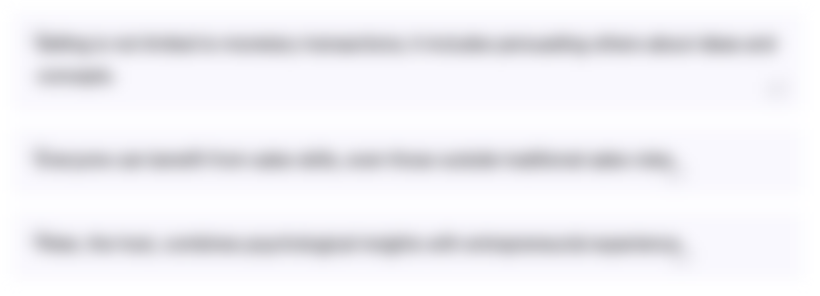
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
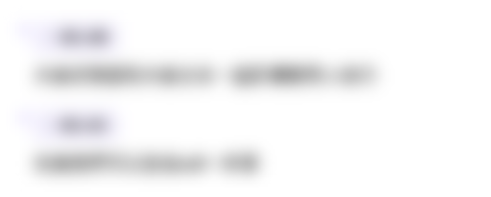
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

Java Tutorial #8: Getters and Setters Explained

#5 Konsep Method Setter dan Getter pada Pemrograman Berorientasi Objek | KONSEP PBO / OOP

Java Inheritance | Java Inheritance Program Example | Java Inheritance Tutorial | Simplilearn

Dot vs Bracket Notation | JavaScript π₯ | Lecture 040
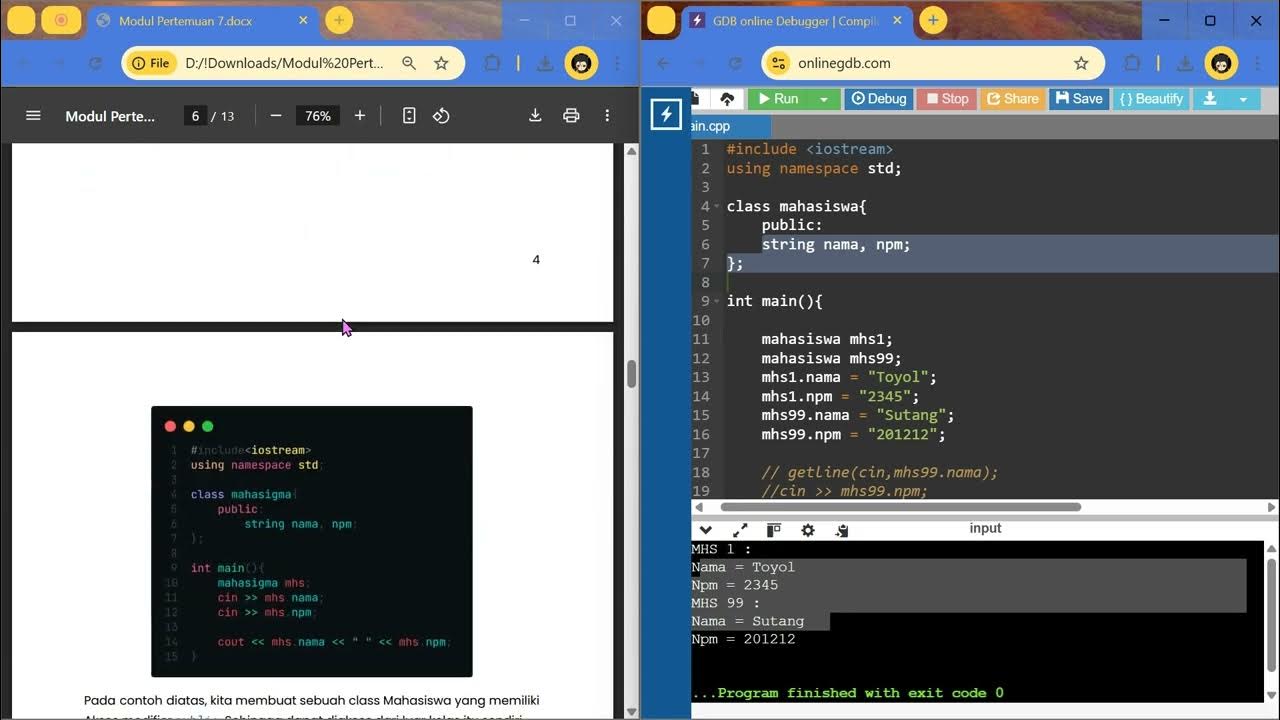
Pertemuan 7 Pemrograman Terstruktur 2025
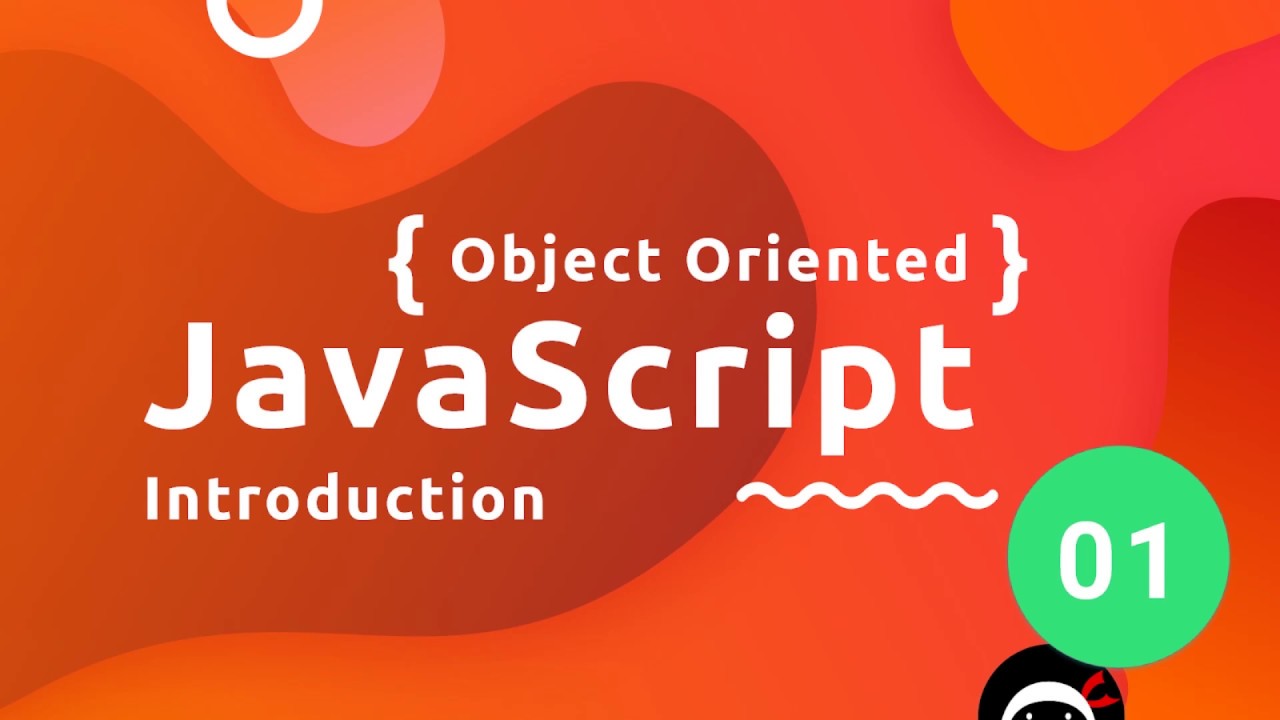
Object Oriented JavaScript Tutorial #1 - Introduction
5.0 / 5 (0 votes)