Creating a Class in Java
Summary
TLDRIn this tutorial, the process of creating a class in Java is explored by defining a 'Circle' class. The video covers how to define the class, its attributes (center as a point and radius as a double), and its methods, such as calculating the area and perimeter, setting the radius, and updating the center. The tutorial emphasizes how the class will be used to create circle objects, with each object having its own attributes. The use of Java's Math class for calculations like Pi is also discussed. The video serves as an introduction to object-oriented programming concepts in Java.
Takeaways
- 😀 Define a class in Java using the 'class' keyword followed by the class name, adhering to Pascal naming convention.
- 😀 A circle class has two main attributes: a 'center' (Point type) and a 'radius' (double type).
- 😀 A circle class doesn't contain a 'main' method. It's used to create circle objects in another class that contains the 'main' method.
- 😀 Methods in the circle class should include functionality like calculating the area, perimeter, and modifying the radius or center.
- 😀 The 'getArea' method calculates and returns the area of the circle using the formula πr².
- 😀 The 'getPerimeter' method calculates and returns the perimeter (circumference) of the circle using the formula 2πr.
- 😀 The 'setRadius' method allows you to change the radius of the circle, modifying its size.
- 😀 The 'setCenter' method allows you to change the center of the circle, updating its position.
- 😀 Instance variables like 'center' and 'radius' are declared directly inside the class and are accessible throughout the class methods.
- 😀 Attributes in a class can be assigned default values. In this case, the 'center' is set to null and 'radius' to 0.0 by default.
Q & A
What is the purpose of the Circle class in this tutorial?
-The purpose of the Circle class is to define the structure of a circle object, which includes attributes like center and radius, and methods like calculating the area, perimeter, and setting new values for the radius and center.
What attributes are defined in the Circle class?
-The Circle class defines two attributes: 'center', which is a Point object representing the center of the circle, and 'radius', a double representing the radius of the circle.
What does the getArea() method do in the Circle class?
-The getArea() method calculates and returns the area of the circle using the formula: π * radius^2.
What does the getPerimeter() method calculate in the Circle class?
-The getPerimeter() method calculates and returns the perimeter (circumference) of the circle using the formula: 2 * π * radius.
What is the significance of the setRadius() method?
-The setRadius() method allows us to update the radius of the circle object by assigning a new value to it, passed as an argument to the method.
How does the setCenter() method work in the Circle class?
-The setCenter() method takes a new Point object as an argument and assigns it to the 'center' attribute of the circle, effectively changing the circle's center.
What type of method is setRadius(), and why?
-The setRadius() method is a void method because it does not return any value. Its purpose is to modify the radius of the circle, not to provide a return result.
Why does the Circle class not include a main method?
-The Circle class does not include a main method because it is intended to be used as a blueprint for creating circle objects. The main method is typically placed in another class that uses the Circle class to instantiate objects.
What does it mean that the attributes of the Circle class are instance variables?
-Instance variables are attributes that belong to each specific object created from the class. In this case, each Circle object will have its own 'center' and 'radius', and they will not be shared between different Circle objects.
Why are default values assigned to the attributes in the Circle class?
-Default values are assigned to the attributes to ensure that when an object is created, the attributes have an initial value. For example, the 'center' is initialized to null (since it's a reference type) and the 'radius' is set to 0.0 (as it’s a numeric type).
Outlines
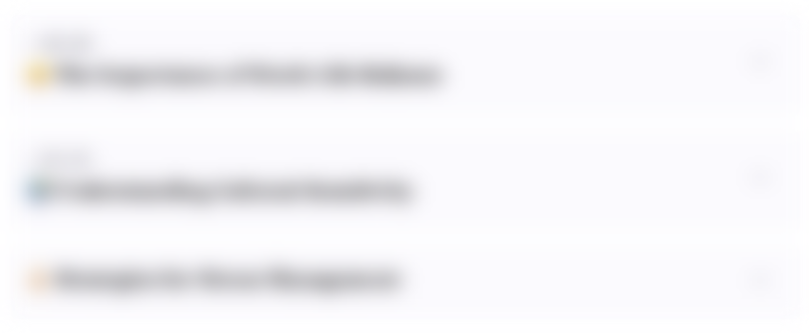
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
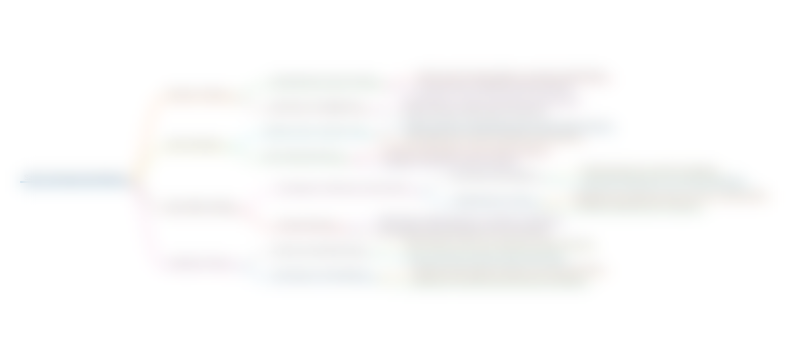
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
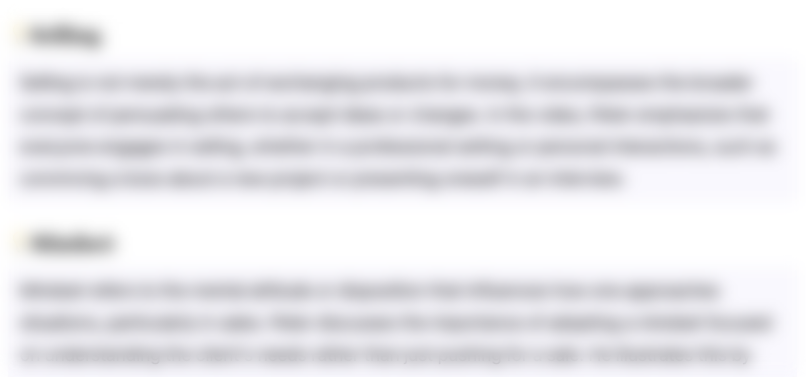
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
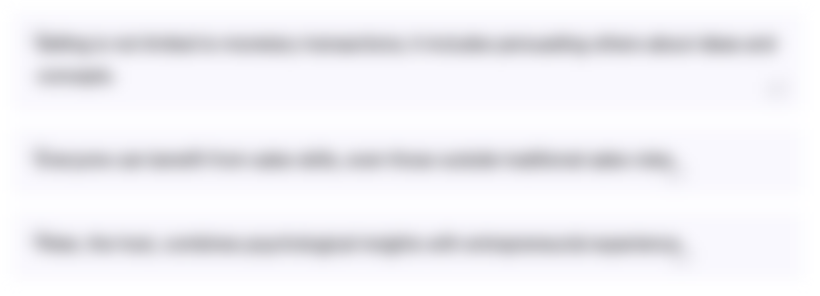
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
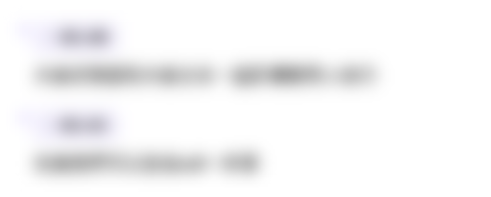
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
5.0 / 5 (0 votes)