Optional Types
Summary
TLDRThe video focuses on advanced functional programming concepts, particularly the use of `Optional`, `flatMap`, and handling potential null values in streams. The speaker demonstrates how to work with functions that may return undefined or missing values, such as looking up user IDs in a database. By utilizing `Optional`, the speaker explains how to encapsulate potentially missing values and manipulate them elegantly with functional methods. The video also highlights the modularity and safety of using `Optional` in place of null checks, making the code more robust and easier to maintain.
Takeaways
- 😀 FlatMap is used to combine functions where the first function returns an optional value, and the second function can operate on that value if present.
- 😀 Instead of using null, Optional provides a safe and modular way to represent values that may or may not be present.
- 😀 Functions that return Optional types should be handled carefully, and the user of the function must be aware of how Optional works.
- 😀 The Optional class allows you to encapsulate the concept of missing values, avoiding potential NullPointerExceptions.
- 😀 You can replace missing values with default values using methods like orElse in Optional, making error handling more streamlined.
- 😀 FlatMap is particularly useful in stream processing, as it can be used to handle empty or invalid elements in a stream of data.
- 😀 A lookup function that returns Optional<User> is an example of using Optional to handle the possibility of an invalid or non-existent user ID.
- 😀 Stream processing with Optional and flatMap allows you to transform and filter streams efficiently while accounting for potential missing values.
- 😀 Using Optional can make your code more modular and object-oriented, as it abstracts the representation of missing values and eliminates direct null handling.
- 😀 In contrast to traditional approaches, Optional offers a more expressive way to handle optionality and missing values, making your code easier to maintain and understand.
Q & A
What is the role of `Optional` in Java, as discussed in the transcript?
-In Java, `Optional` is used to represent a value that may or may not be present, offering a cleaner alternative to handling null values. It encapsulates a potentially missing value inside an object, allowing developers to avoid directly working with `null` and providing methods like `orElse` to handle absent values.
Why is `flatMap` used in the context of `Optional`?
-`flatMap` is used when composing two functions where the first function may return an `Optional` type. It ensures that the second function operates on the value inside the `Optional` if present, otherwise skipping the operation. This helps in chaining operations without breaking the flow when values are missing.
What is the difference between `map` and `flatMap` when dealing with `Optional`?
-The key difference is that `map` applies a function to the value inside an `Optional` and returns a new `Optional`. In contrast, `flatMap` also applies a function but expects the function to return an `Optional` as well, allowing for smoother chaining without nesting `Optional` objects.
How does `flatMap` help with stream processing in Java?
-`flatMap` helps in stream processing by allowing you to map values in a stream to `Optional` types and then flatten those `Optional` values into a single stream. This is especially useful when some operations may return empty results, as it eliminates the need for explicit null checks.
What happens if the `lookup` function in the example fails to find a user?
-If the `lookup` function fails to find a user, it returns an empty `Optional`, which means the result of that lookup is absent. The code then uses `flatMap` to handle this absence without breaking the flow of the program.
Can `Optional` be used to handle other types of failure scenarios beyond null values?
-Yes, `Optional` can be used to handle various types of failure scenarios, such as invalid inputs, missing records, or any situation where a result might be absent, without resorting to `null` or error-prone checks.
What are some benefits of using `Optional` instead of returning `null`?
-Using `Optional` reduces the risk of `NullPointerException` by explicitly signaling the absence of a value. It also provides a more expressive API, where the user of the function can easily decide how to handle the absence of a value using methods like `orElse`, `ifPresent`, or `flatMap`.
How does the `stream.ofNullable` method relate to `Optional`?
-`stream.ofNullable` is used to convert a potentially nullable value into a stream. If the value is `null`, it produces an empty stream, while non-null values are wrapped in a stream. This is useful when working with `Optional` types in stream processing, as it handles missing values gracefully.
What is the role of methods like `orElse` when working with `Optional`?
-Methods like `orElse` allow you to define a default value when the `Optional` is empty. This provides a simple way to replace missing values without needing to check explicitly for null or empty results.
What are some challenges or considerations when using `Optional` in Java?
-While `Optional` improves readability and safety, it requires that users understand its usage and methods. Also, it adds a layer of abstraction that may be overkill for simple cases where null is acceptable. Additionally, functions returning `Optional` require other code to properly handle the empty case, which may introduce complexity in certain scenarios.
Outlines
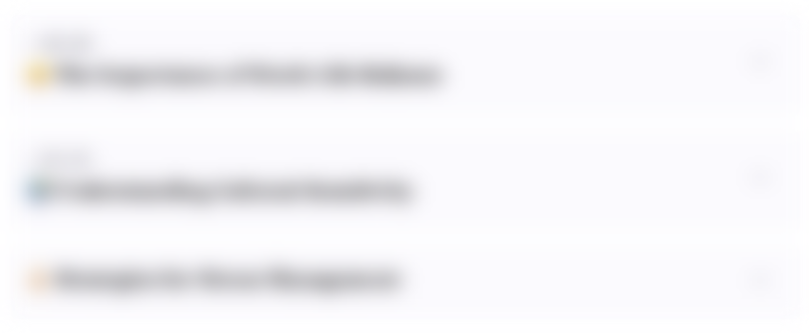
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
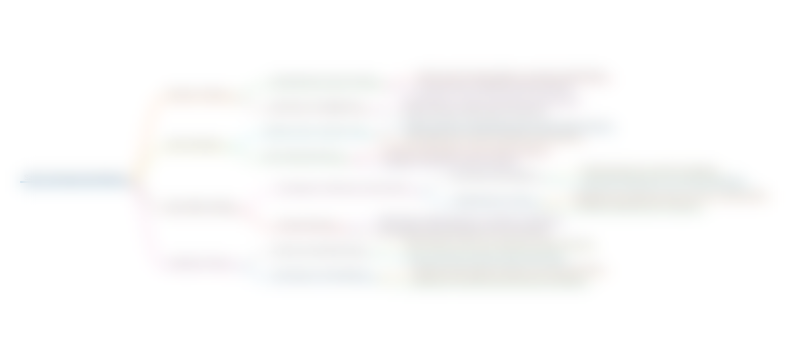
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
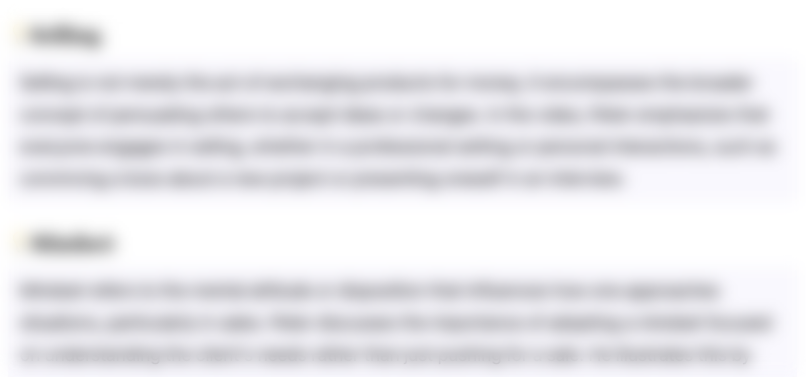
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
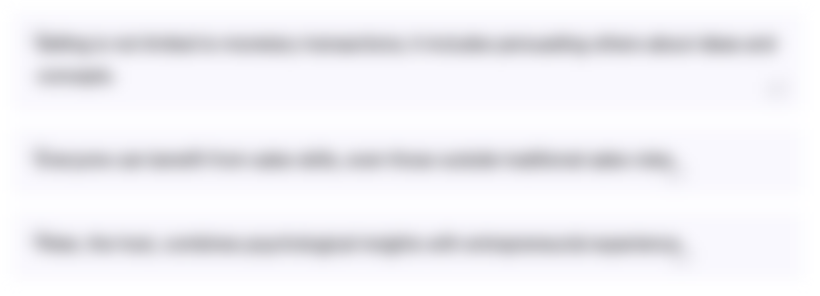
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
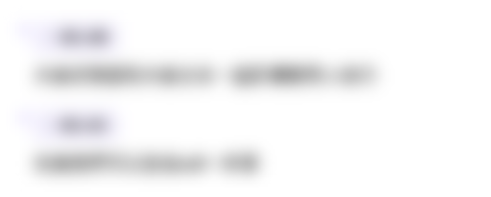
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآن5.0 / 5 (0 votes)