JavaScript - Reference vs Primitive Values/ Types
Summary
TLDRThis video explains the difference between primitive and reference types in JavaScript, focusing on common errors that arise when dealing with data assignments. It covers how primitive types like strings and numbers are copied by value, while reference types like objects and arrays are copied by reference. The video highlights how assigning an object to a new variable doesn't duplicate the data but rather copies the memory reference, leading to changes being reflected across variables. It also addresses solutions for deep copying, such as using `Object.assign` or array methods like `slice`, and emphasizes the need to manage references carefully.
Takeaways
- 😀 Primitive types in JavaScript include strings, numbers, booleans, undefined, null, and symbols. They are immutable and stored directly in memory on the stack.
- 😀 Reference types include objects and arrays, which are stored in memory on the heap. These types are mutable, and variables store references (pointers) to the data rather than the data itself.
- 😀 When you assign a primitive type to a new variable, the value is copied. Changes to one variable will not affect the other.
- 😀 When you assign a reference type to a new variable, only the reference (pointer) is copied. Changes to the object or array affect all variables pointing to the same reference.
- 😀 The stack is a fast but limited memory space where primitive types are stored. The heap is slower but has more space for reference types.
- 😀 JavaScript variables do not store the actual object but store the reference to the object in memory. This is why modifying a reference type affects all variables pointing to it.
- 😀 When you copy an object or array, only the reference is copied. To create a real copy, you need to use methods like Object.assign() or array.slice().
- 😀 Objects and arrays are reference types, meaning changes to properties or elements will be reflected in all variables referring to the same memory location.
- 😀 To deeply clone an object or array (including nested structures), use tools like Lodash's cloneDeep method, as Object.assign() and array.slice() only perform shallow copies.
- 😀 It's important to understand the difference between primitive and reference types to prevent unexpected behavior when copying variables or passing objects/arrays around in your code.
Q & A
What is the main difference between primitive and reference types in JavaScript?
-Primitive types in JavaScript, such as strings, numbers, and booleans, are copied by value, meaning when they are assigned to a new variable, a separate copy of the value is made. Reference types, such as objects and arrays, are copied by reference, meaning the variable stores a pointer to the same memory location where the object or array is stored.
How do primitive types behave when assigned to new variables?
-When a primitive type is assigned to a new variable, the value itself is copied, meaning both variables hold the same value but are independent. If one variable is changed, the other remains unaffected.
Why does modifying one variable of a reference type affect all variables referencing the same object?
-Because reference types are copied by reference, when a reference type is assigned to another variable, both variables point to the same memory location. Modifying the object via one variable affects the object in memory, which reflects in all variables that reference the same object.
What is the difference in memory storage between primitive types and reference types?
-Primitive types are stored on the stack, a fast but limited memory space, whereas reference types are stored on the heap, which can hold larger amounts of data and is slower to access.
Why does changing the value of a primitive type in one variable not affect another variable?
-Primitive types are copied by value, so when one variable is assigned a primitive value, a separate copy is made. Changing the original variable does not affect the copied value in the second variable.
What happens when you assign one object to another object in JavaScript?
-When an object is assigned to another object, the reference to the original object is copied, not the actual object itself. Both variables will point to the same memory location, so changes made to one object will affect the other.
What is the stack and heap, and how are they used in memory management for primitive and reference types?
-The stack is a fast memory area used for storing primitive types, which are small and independent. The heap is a slower memory area used for reference types, which can hold larger and more complex data like objects and arrays.
What is the purpose of the 'Object.assign' method in JavaScript?
-'Object.assign' is used to create a shallow copy of an object. It copies the properties from one object into a new object. However, it does not deeply copy nested objects or arrays; if the object contains reference types, the reference will still point to the original memory location.
What is the issue with copying an object using 'Object.assign' when the object contains reference types like arrays?
-When using 'Object.assign' to copy an object, if the object contains reference types like arrays, those reference types are not deeply copied. Instead, the reference to the original array is copied, meaning any changes to the array in one object will affect the array in the copied object.
How can you properly create a deep copy of an object that includes reference types like arrays?
-To create a deep copy of an object with reference types, you can use libraries like Lodash with the 'cloneDeep' function or manually iterate through the object and recursively copy each property, ensuring that nested reference types are also copied.
Outlines
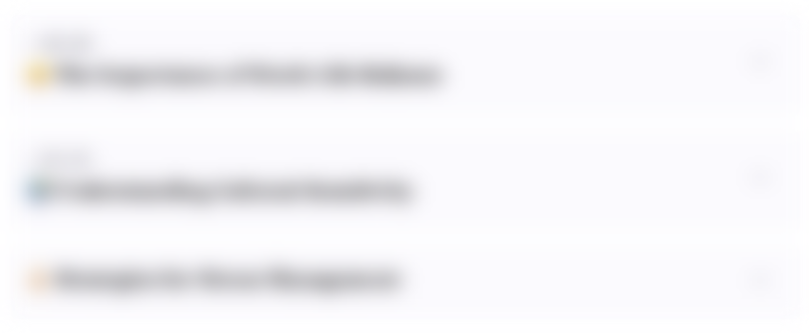
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
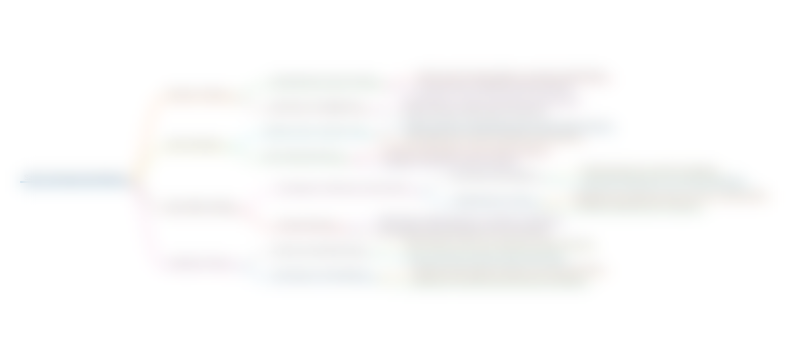
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
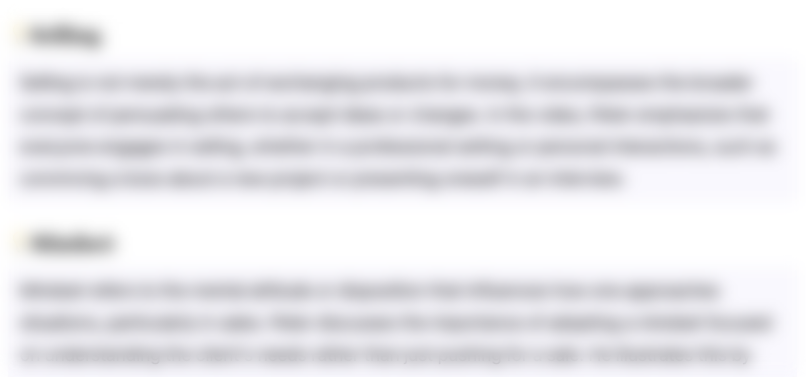
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
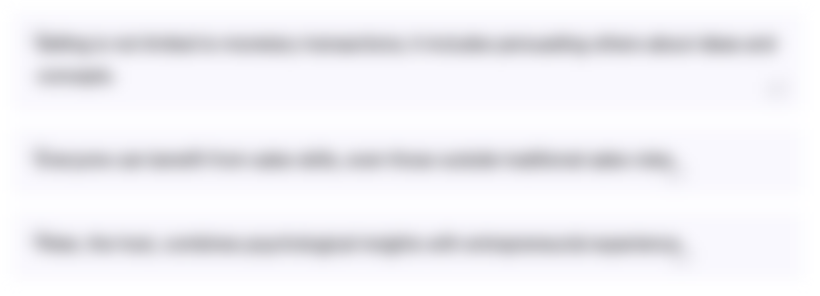
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
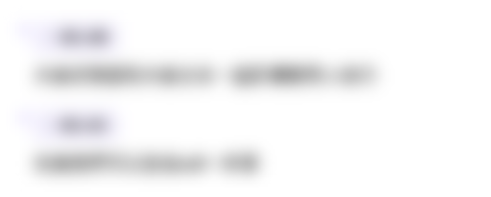
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
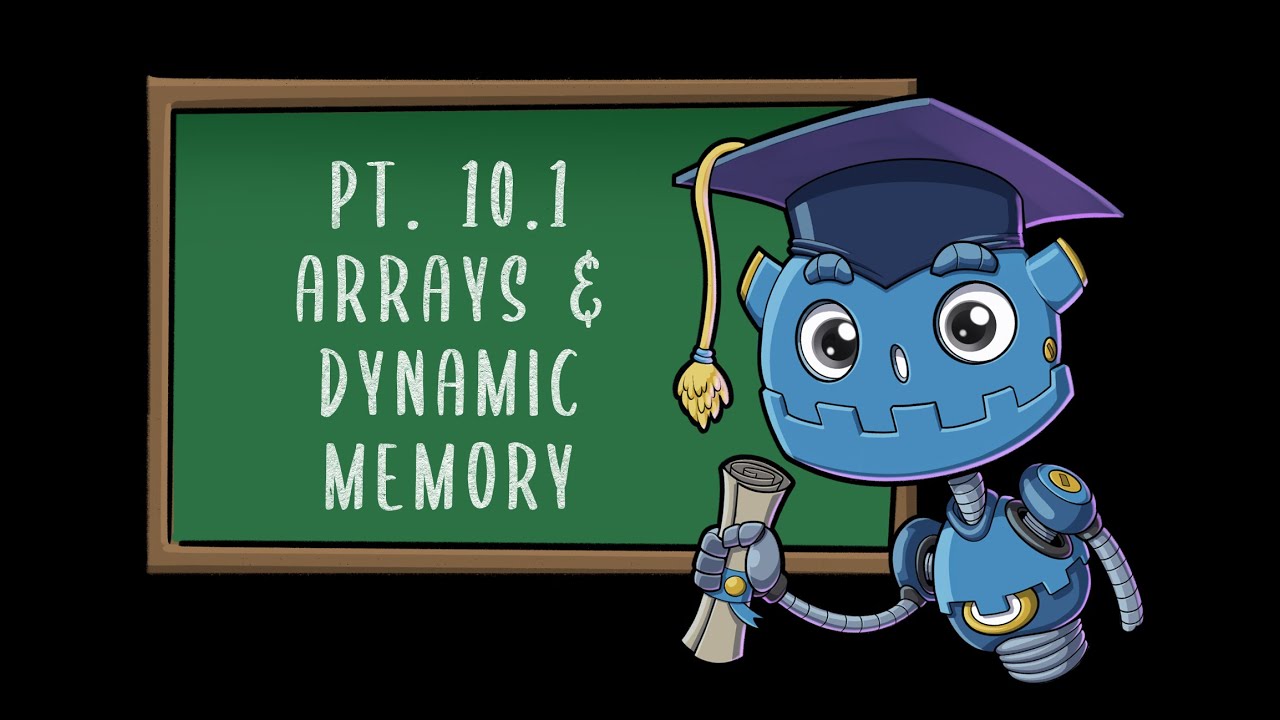
Arrays & Dynamic Memory Address | Godot GDScript Tutorial | Ep 10.1

Variables in Java ✘【12 minutes】

ССЫЛОЧНЫЕ И ЗНАЧИМЫЕ ТИПЫ C# | СТЕК И КУЧА C# | REFERENCE AND VALUE TYPES C# | C# Уроки | # 38

How variables works in Python | Explained with Animations

How Passing Arguments Works_ Value vs Reference | JavaScript 🔥 | Lecture 120
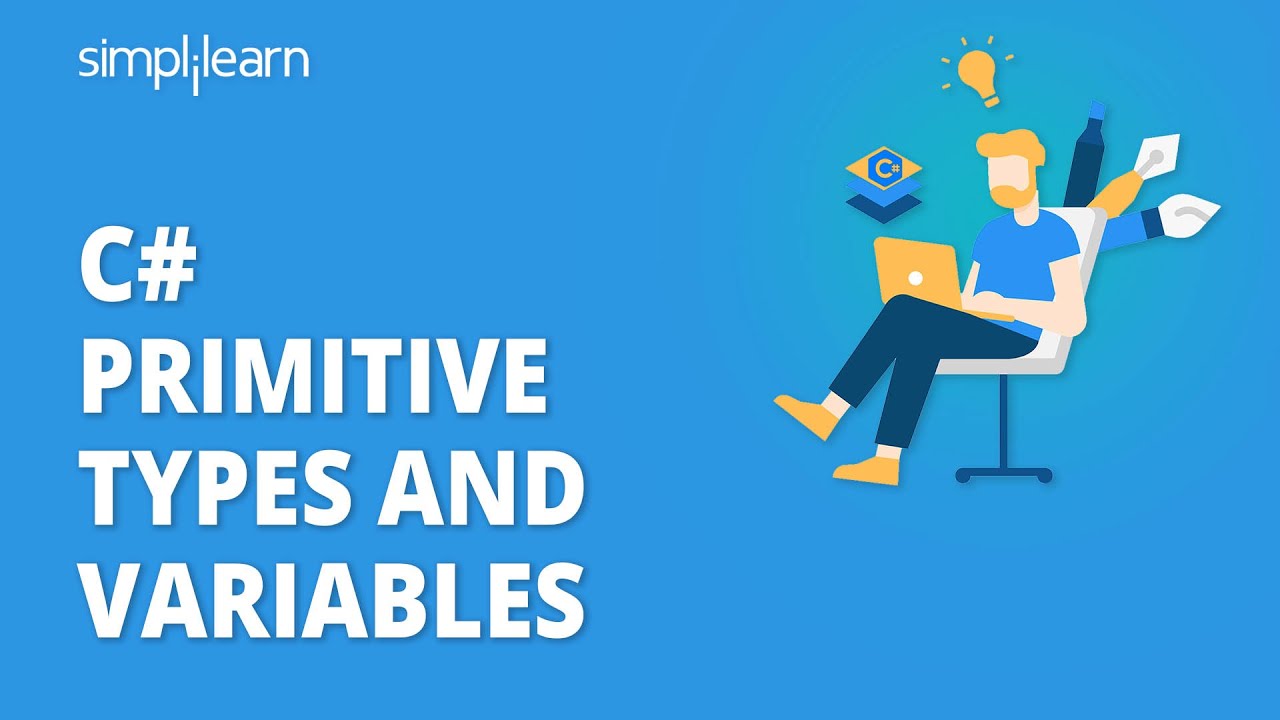
C# Primitive Types and Variables | Datatype Literals and Variables | C# Tutorial | Simplilearn
5.0 / 5 (0 votes)