Life Cycle Methods | Mastering React: An In-Depth Zero to Hero Video Series
Summary
TLDRIn this React JS tutorial, the instructor dives into component lifecycle methods, essential for beginners learning React from scratch. The video explains the three phases of a component's lifecycle: mounting, updating, and unmounting. Key methods like constructor, render, componentDidMount, componentDidUpdate, and componentWillUnmount are covered with examples to illustrate their use. The instructor also touches on best practices, such as avoiding setState in componentWillUnmount and using shouldComponentUpdate to control rendering. The session sets the stage for understanding the useEffect hook in future lessons.
Takeaways
- 📚 This video is part of a React JS tutorial series aimed at beginners learning React from scratch.
- 🔄 The focus of the video is on component lifecycle methods, which are crucial for understanding component behavior in React.
- 🎯 Lifecycle methods are categorized into mounting, updating, and unmounting, corresponding to different stages of a component's existence.
- 🌱 Mounting methods are called when a component is first rendered, in the order of constructor, getDerivedStateFromProps, render, and componentDidMount.
- 🔄 The render method is central to a component's lifecycle, being called on the initial render and subsequent updates.
- 🔁 The updating methods, including getDerivedStateFromProps, shouldComponentUpdate, render, getSnapshotBeforeUpdate, and componentDidUpdate, are involved in subsequent renders triggered by state or props changes.
- 🛑 The componentWillUnmount method is invoked right before a component is removed from the DOM, making it suitable for cleanup activities.
- 🚫 It's important not to call setState inside componentWillUnmount as it won't trigger a re-render.
- 🔍 The video uses a class component example to illustrate the lifecycle methods, emphasizing the render method's purity and the constructor's role in state initialization and method binding.
- 🔄 The video also covers the use of componentDidUpdate for handling updates and the potential pitfalls of causing infinite rerenders if not used carefully.
- 🔄 The shouldComponentUpdate method is highlighted as a way to control whether a component should re-render, with an example showing its interaction with the render and componentDidUpdate methods.
Q & A
What are the three main categories of lifecycle methods in React?
-The three main categories of lifecycle methods in React are mounting, updating, and unmounting.
What happens during the mounting phase of a React component?
-During the mounting phase, a component renders for the first time, and the following methods are called in this order: constructor, getDerivedStateFromProps, render, and componentDidMount.
Why is the constructor method important in a React class component?
-The constructor method is important for initializing local state and binding event handlers. It is also the only place where you should assign this.state directly.
What is the purpose of the componentDidMount lifecycle method?
-componentDidMount is invoked immediately after a component is mounted, making it a good place to instantiate network requests or perform any setup that requires DOM nodes.
Why should you avoid calling setState inside the constructor?
-You should avoid calling setState inside the constructor because it can lead to an infinite loop of renders and updates. Instead, you should assign state directly to this.state in the constructor.
What is the role of the componentDidUpdate lifecycle method?
-componentDidUpdate is called after a component has re-rendered with new props or state. It is used for operations that need to be performed after updates, such as network requests or DOM manipulations, but care should be taken to avoid causing infinite re-renders.
Why is shouldComponentUpdate used and how does it affect componentDidUpdate?
-shouldComponentUpdate is used to determine whether a component should re-render when receiving new props or state. If it returns false, the componentDidUpdate method will not be called for that update cycle.
What is the significance of componentWillUnmount in the lifecycle of a React component?
-componentWillUnmount is called just before a component is unmounted from the DOM. It is used for cleanup activities like clearing timeouts, canceling network requests, or unsubscribing from subscriptions.
Why should you not call setState in componentWillUnmount?
-You should not call setState in componentWillUnmount because the component will never be re-rendered after it is unmounted, and calling setState would be a no-op.
How does the order of lifecycle methods differ between the initial render and subsequent renders?
-During the initial render, the methods are called in the order: constructor, getDerivedStateFromProps, render, and componentDidMount. For subsequent renders, the order is: getDerivedStateFromProps, shouldComponentUpdate, render, getSnapshotBeforeUpdate, componentDidUpdate.
Outlines
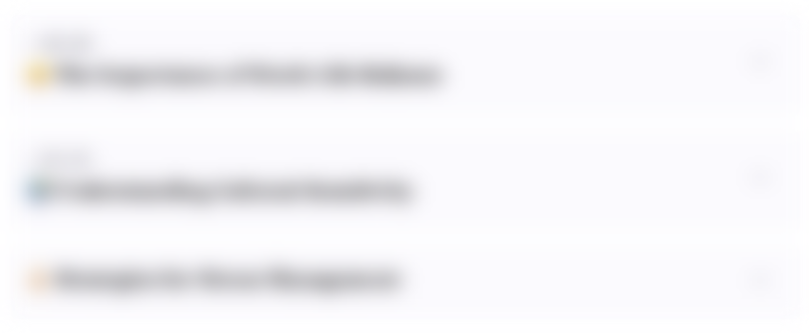
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
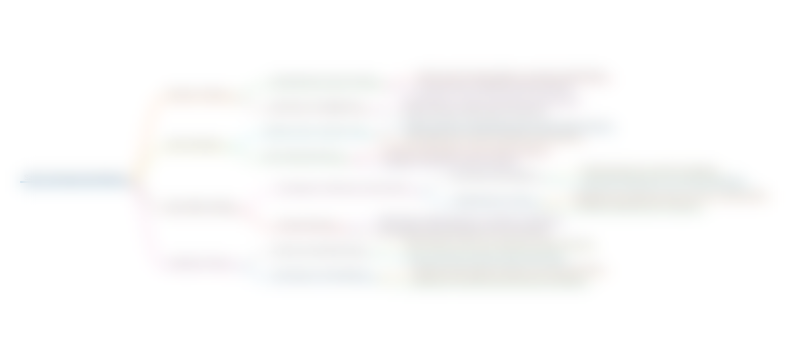
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
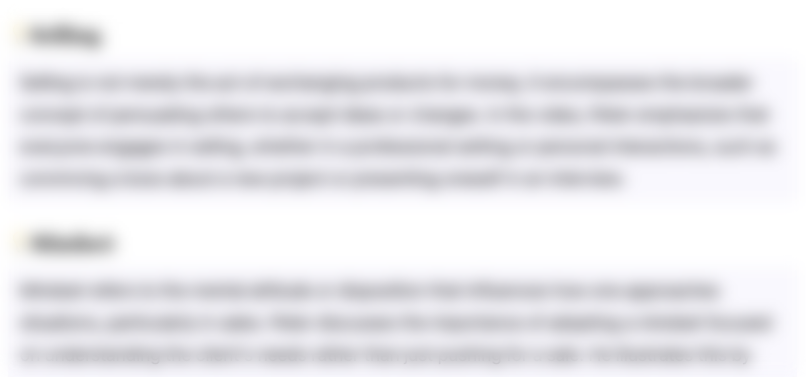
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
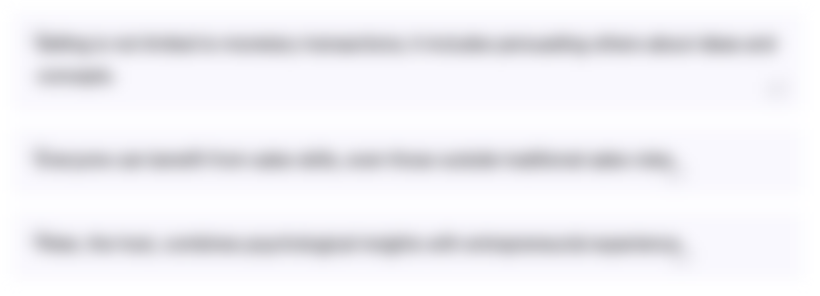
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
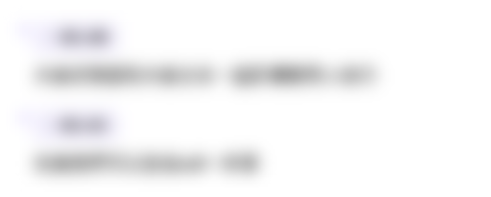
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
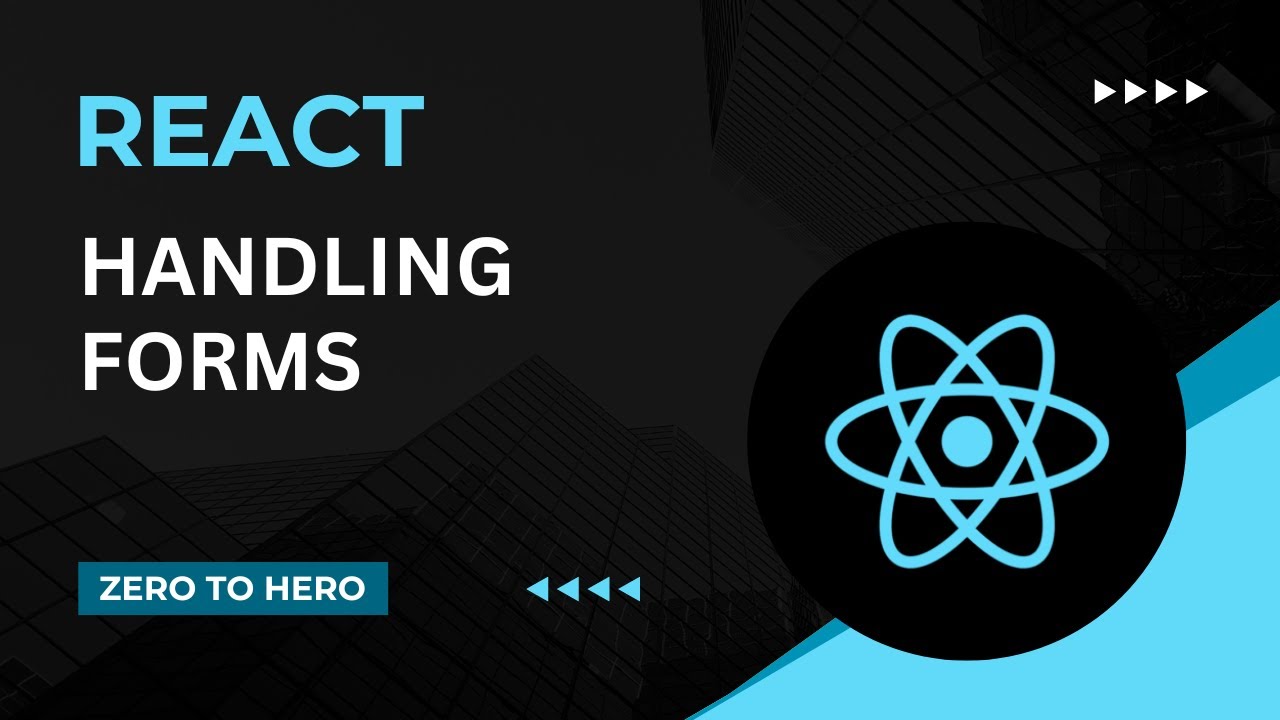
Handling forms | Mastering React: An In-Depth Zero to Hero Video Series
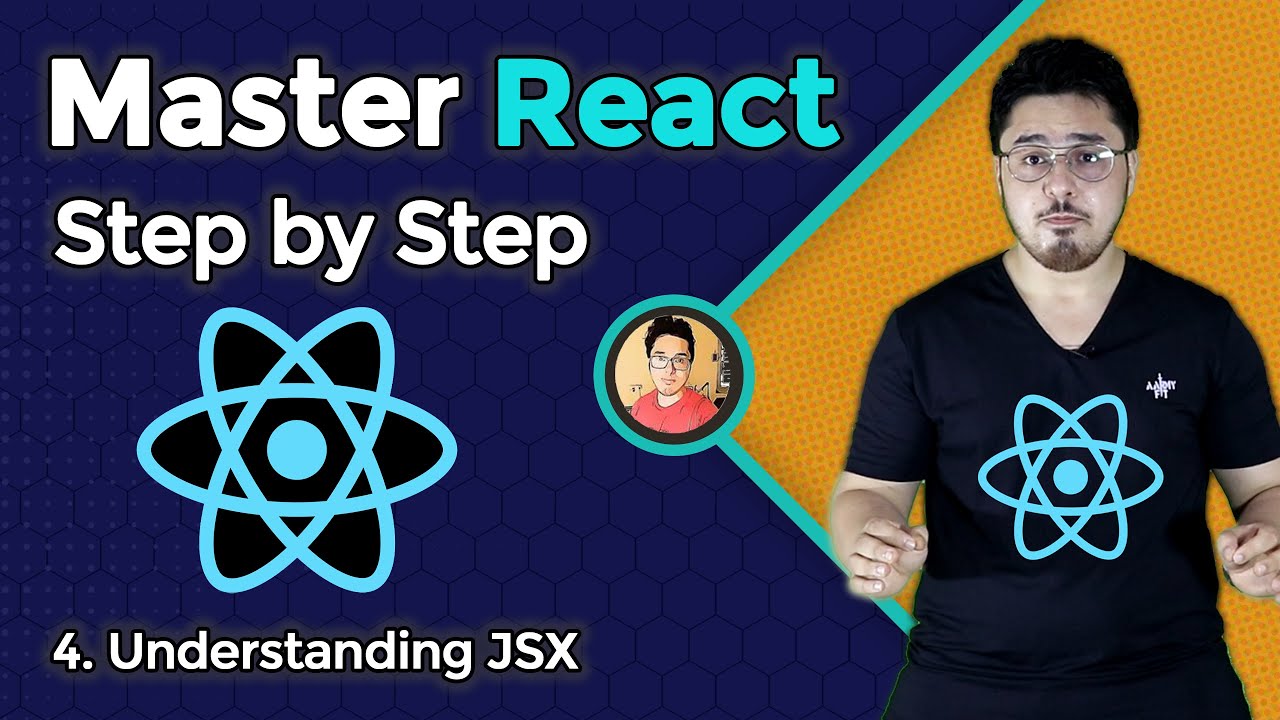
Understanding JSX | Complete React Course in Hindi #4
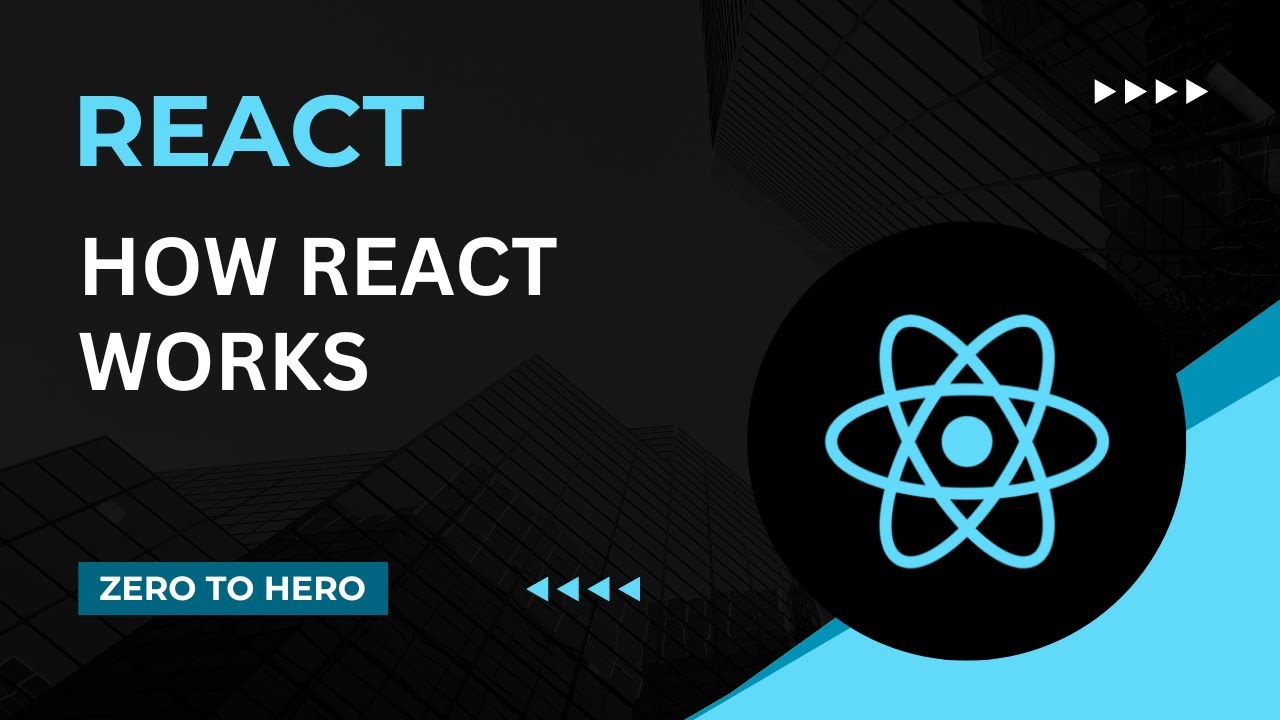
How React Works | Mastering React: An In-Depth Zero to Hero Video Series
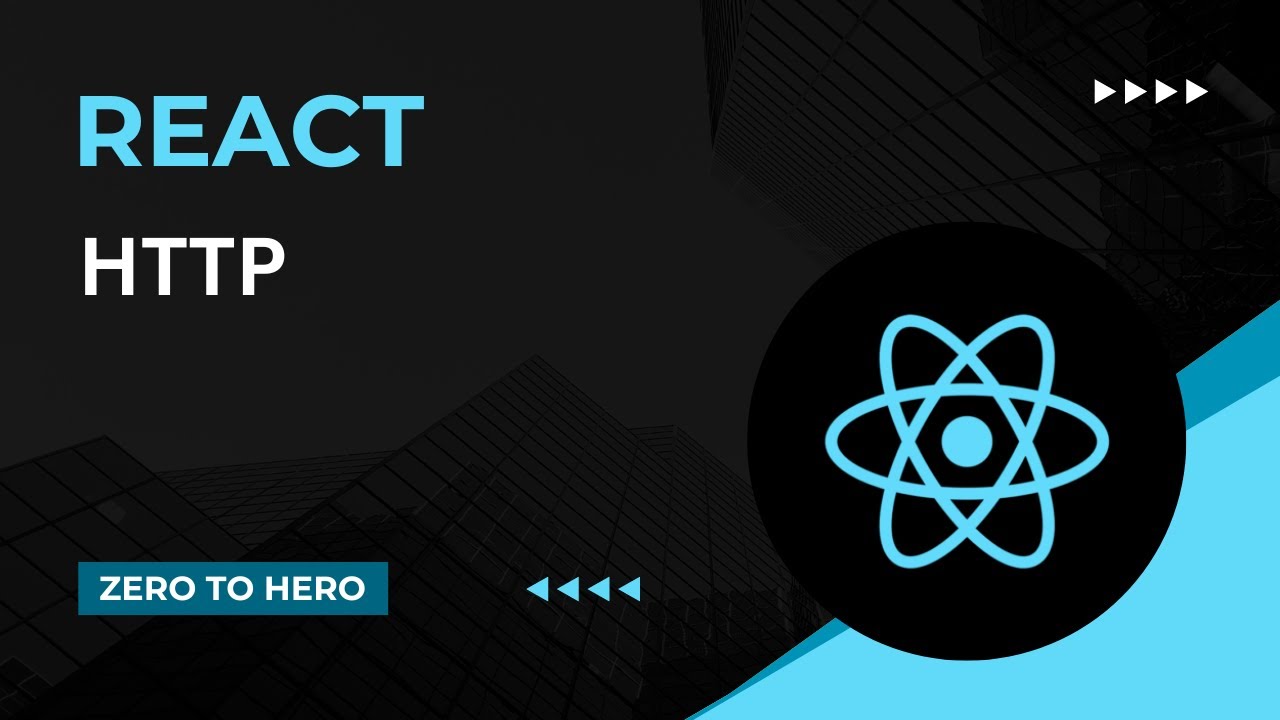
HTTP | Mastering React: An In-Depth Zero to Hero Video Series
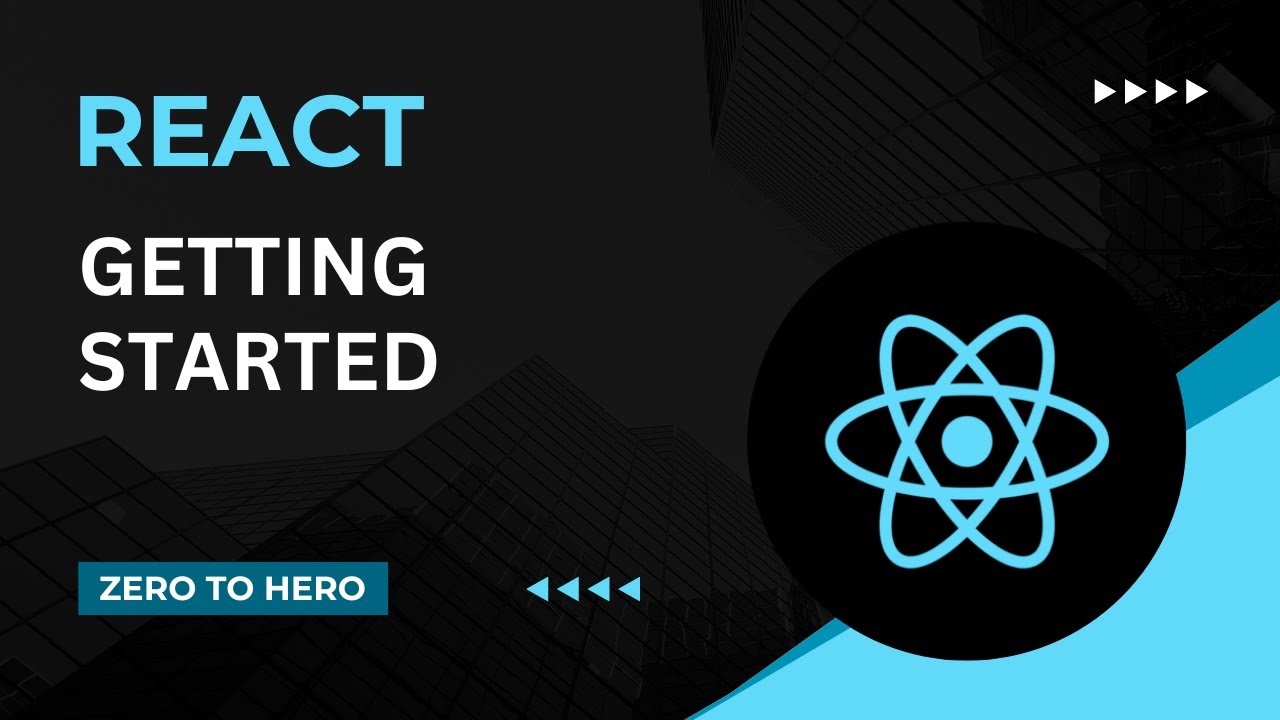
Getting Started | Mastering React: An In-Depth Zero to Hero Video Series
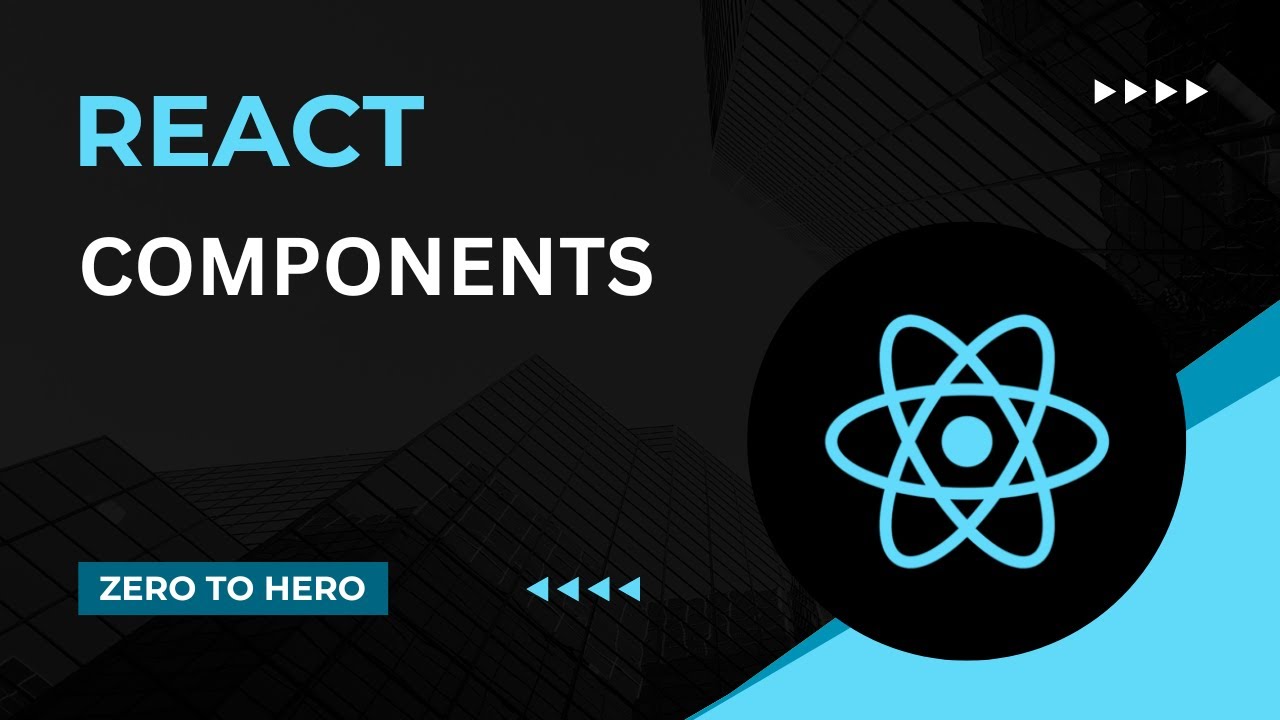
Components | Mastering React: An In-Depth Zero to Hero Video Series
5.0 / 5 (0 votes)