HTTP | Mastering React: An In-Depth Zero to Hero Video Series
Summary
TLDRIn this React JS tutorial for beginners, the instructor explains how to make HTTP requests using Fetch and Axios. The video covers the basics of HTTP, different types of requests, and the differences between Fetch and Axios. The instructor demonstrates fetching data from an API using both methods in a React application, replacing hardcoded data with dynamic content. A practical task is suggested, involving creating a card that links to a GitHub repository using the GitHub API, and viewers are encouraged to try it themselves.
Takeaways
- ๐ HTTP is the protocol used to access data on the World Wide Web, and it's essential for data retrieval from backend databases in applications.
- ๐ Common HTTP request methods include GET, POST, PUT, and DELETE, each serving a specific purpose in data handling.
- ๐ React lacks built-in tools for making HTTP requests, unlike frameworks like Angular, which have an inbuilt HTTP module.
- ๐ ๏ธ Axios and Fetch are popular choices for making HTTP requests in React; Axios is a third-party library, while Fetch is a built-in browser API.
- ๐ Axios automatically handles data serialization and provides JSON-formatted responses by default, simplifying data handling.
- ๐ฆ Fetch requires manual conversion of responses to JSON, adding an extra step compared to Axios.
- ๐ก The video demonstrates how to use both Fetch and Axios to perform GET requests and retrieve data for a React application.
- ๐ฏ The tutorial includes a practical example using an API to fetch data and dynamically render it in a React application.
- ๐ The video suggests a task for viewers to practice: creating an application that fetches user data from a GitHub API and displays it.
- ๐ The video provides a GitHub repository link for viewers to access the solution code for the tutorial and the suggested task.
Q & A
What is the main topic of the React JS - Zero to Hero series?
-The main topic of the React JS - Zero to Hero series is to teach beginners how to learn React JS from scratch.
What was explained in the previous video of the series?
-In the last video, the useContext hook was explained and how it can be used to avoid props drilling.
What is the purpose of the HyperText Transfer Protocol (HTTP)?
-HTTP is a protocol used to access data on the World Wide Web, typically to get data from backend databases.
What are the different types of HTTP requests mentioned in the script?
-The different types of HTTP requests mentioned are GET, POST, PUT, and DELETE.
How does one perform a GET request in React according to the video?
-To perform a GET request in React, one can use either Axios or Fetch. The video demonstrates using Fetch with useEffect and then converting the response to JSON.
What are the differences between Axios and Fetch when making HTTP requests in React?
-Axios is a third-party library that automatically stringifies data and provides JSON responses by default, while Fetch is a built-in API that requires manual serialization of data and conversion of responses to JSON.
Why might someone prefer Axios over Fetch according to the video?
-The video suggests that Axios might be preferred due to its ease of use, automatic JSON handling, and because it is a third-party library specifically designed for HTTP requests.
How does the video demonstrate fetching data from an API?
-The video demonstrates fetching data by using the useEffect hook to call Fetch or Axios, then setting the state with the fetched data to render it in the React application.
What is the task suggested by the video for practice?
-The suggested task is to create a small application where clicking a card takes the user to the respective GitHub repo, using the GitHub users API with Axios or Fetch.
How can one access the solution code for the suggested task?
-The solution code for the suggested task can be accessed from a repository, the link to which is provided in the video description.
What is the final call to action from the video presenter?
-The presenter asks viewers to like the video, subscribe to the channel, and support them.
Outlines
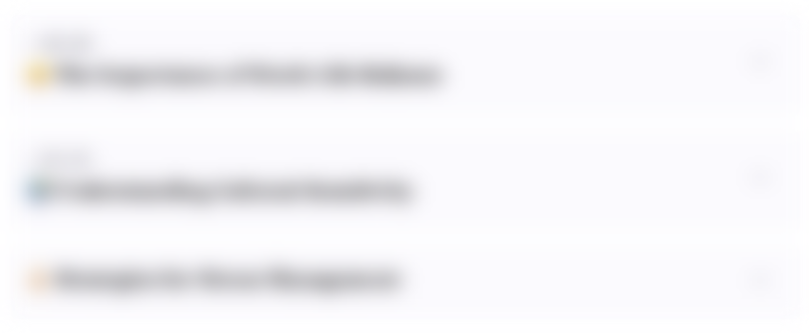
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
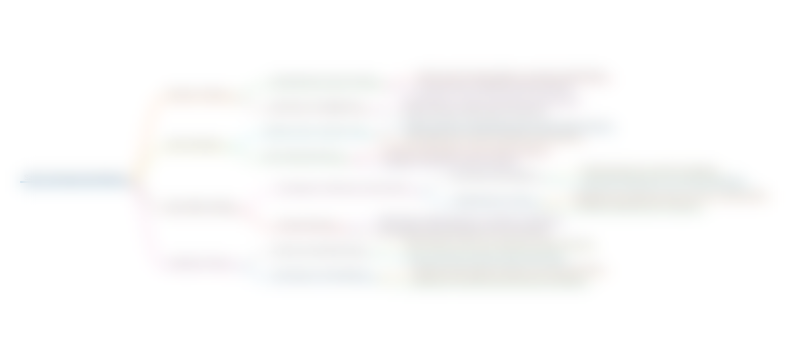
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
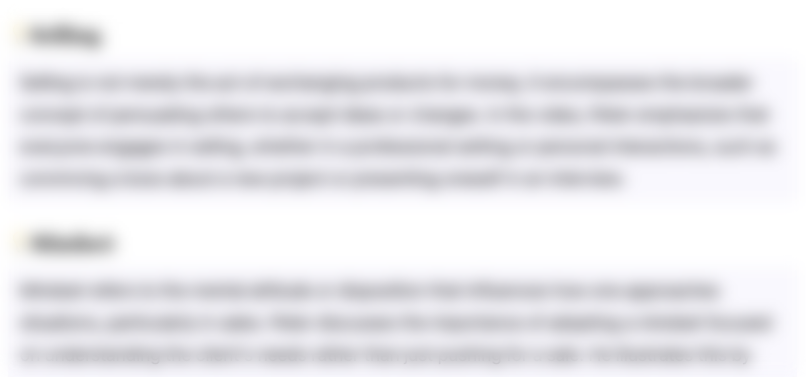
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
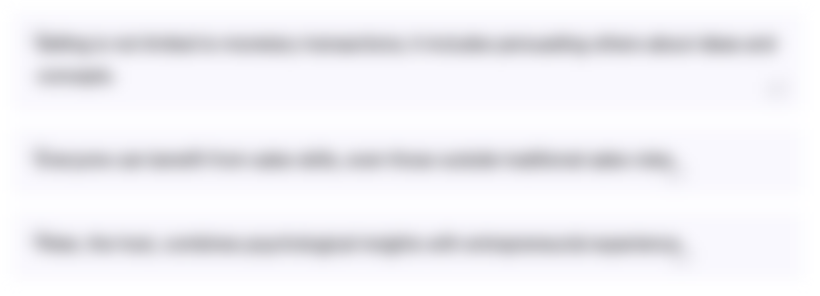
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
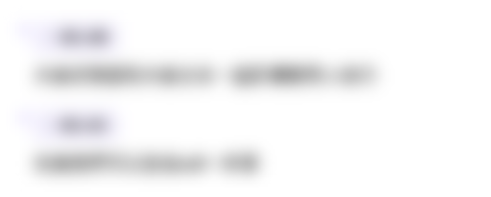
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
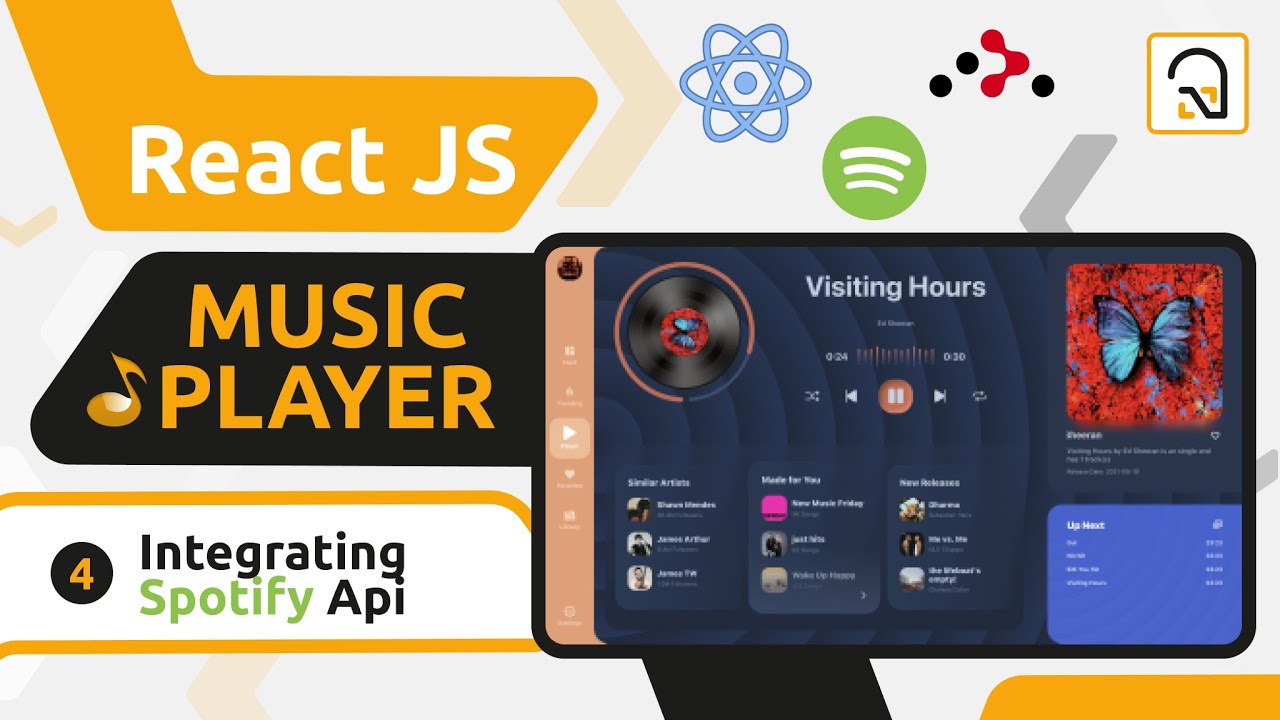
ReactJS Music Player #4: Integrating the Spotify Api in our React App
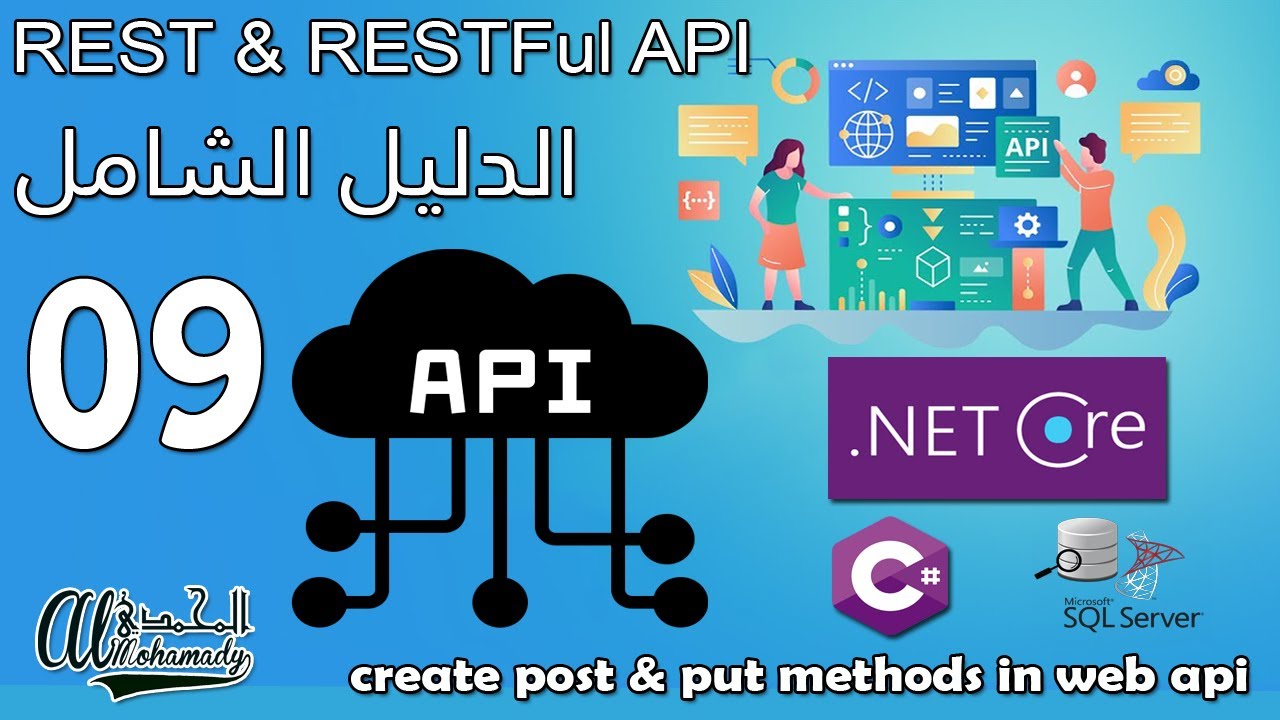
09 ุฅูุดุงุก ุฏูุงู ุงูุญูุธ ู ุงูุชุนุฏูู create post & put methods in web api
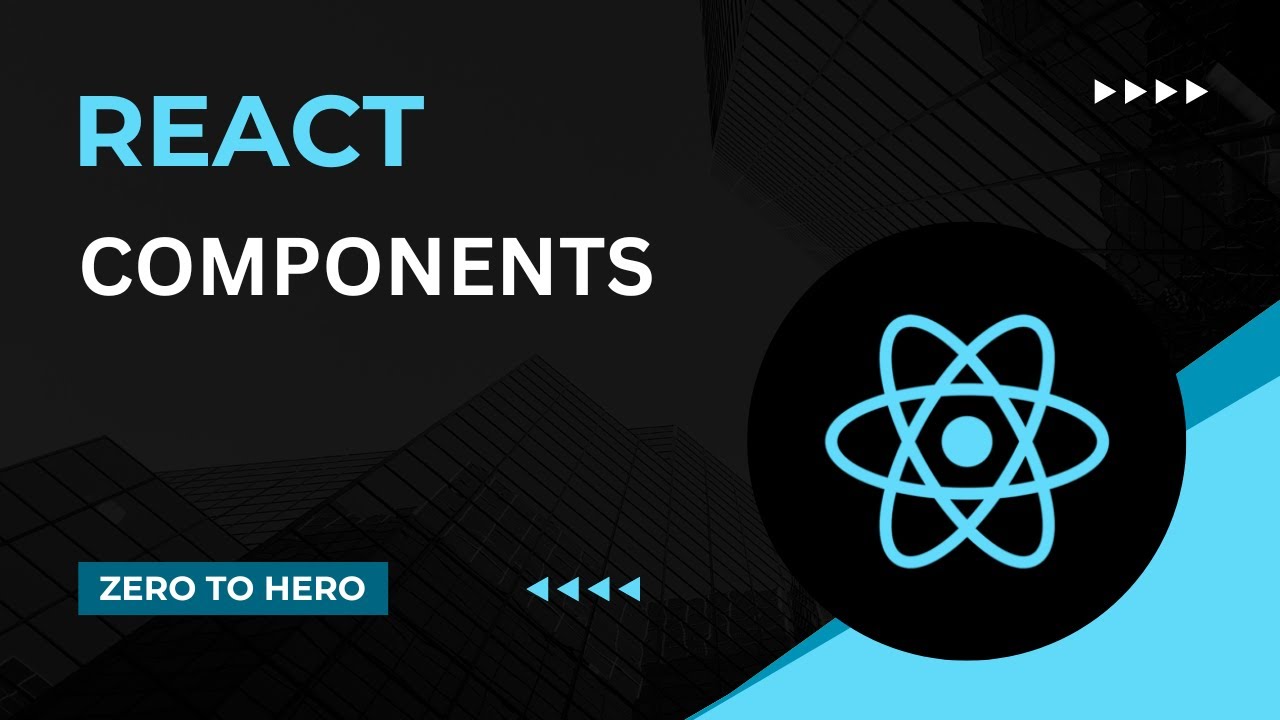
Components | Mastering React: An In-Depth Zero to Hero Video Series
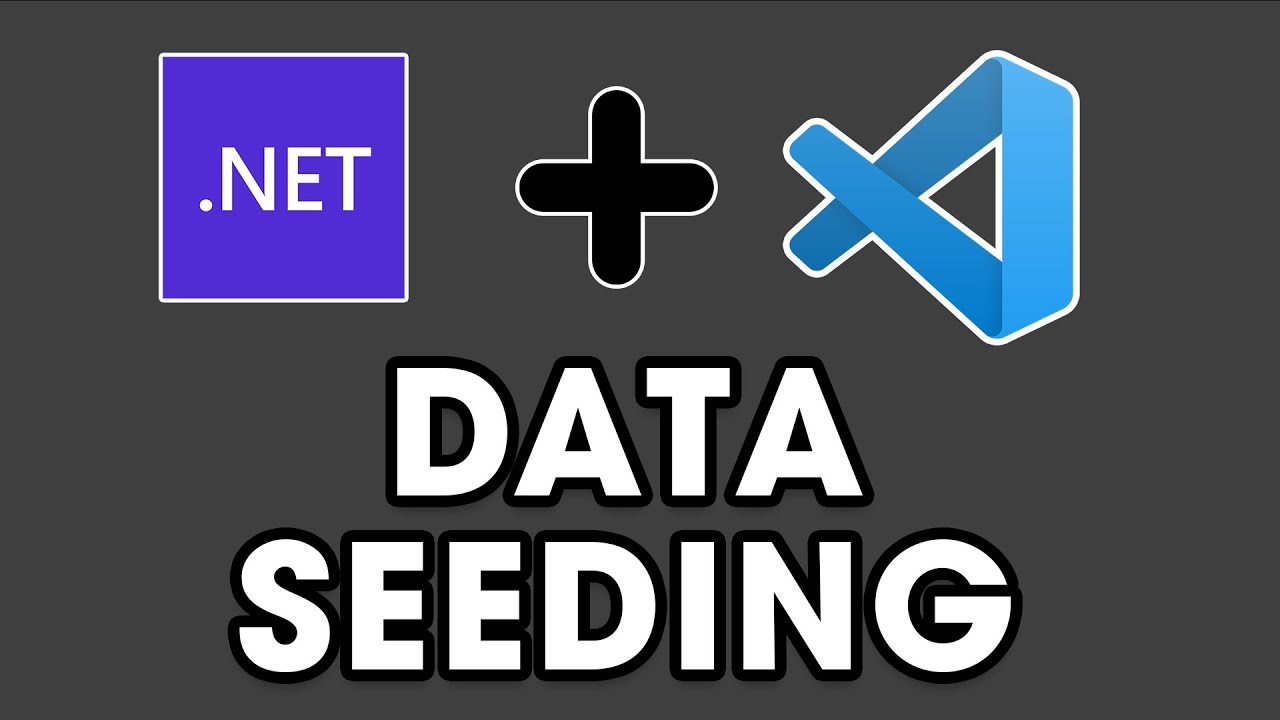
ASP.NET Core Web API .NET 8 2024 - 31. Data Seeding
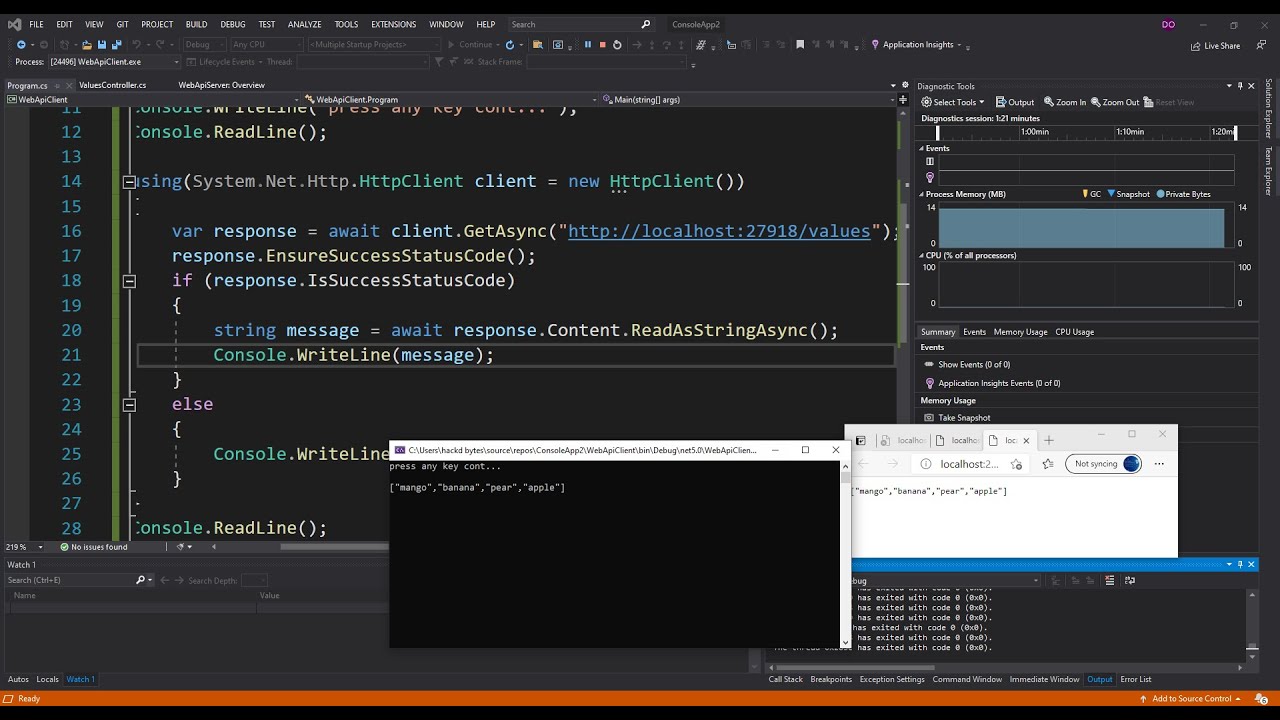
Asp.Net Core Web API Client/Server Application | Visual Studio 2019
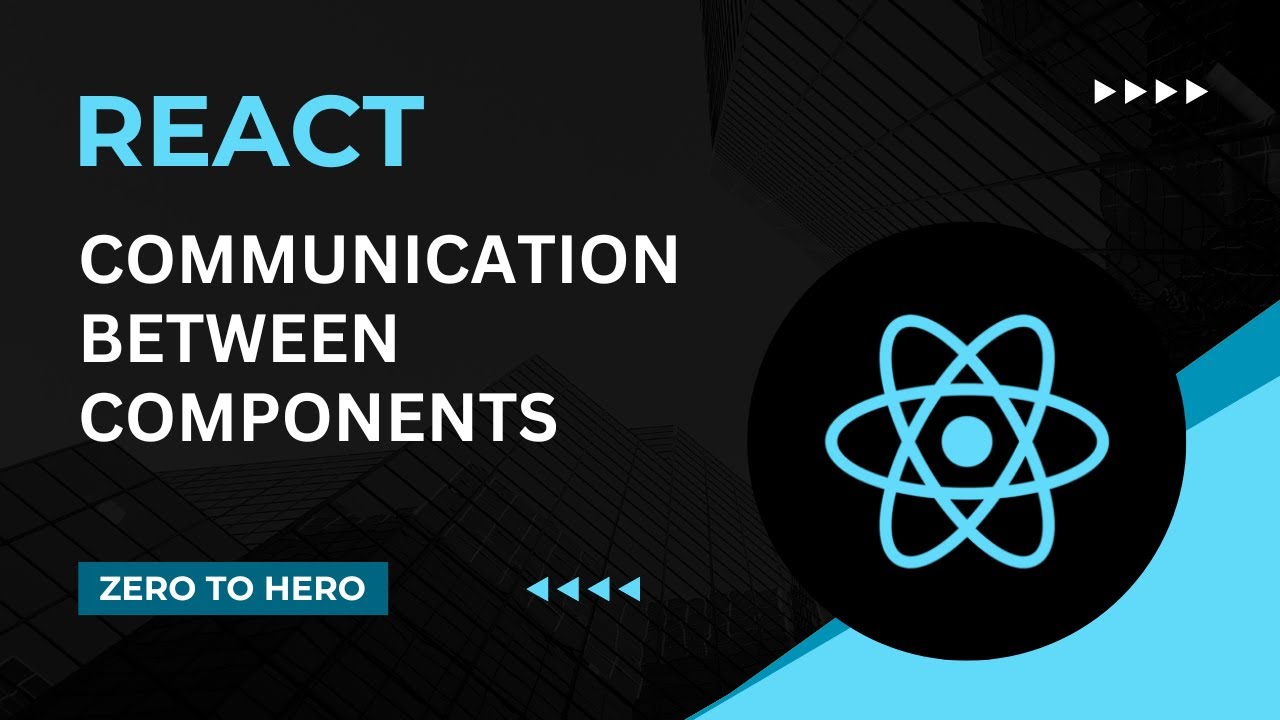
Communication between components | Mastering React: An In-Depth Zero to Hero Video Series
5.0 / 5 (0 votes)