How to render lists | Mastering React: An In-Depth Zero to Hero Video Series
Summary
TLDRIn this React JS tutorial, the instructor guides beginners on rendering lists using React components. They cover creating lists with arrays, using loops like 'for of' and 'forEach', and emphasize the preference for 'map' due to its ability to return a new array. The importance of unique 'key' props for list items is discussed, with recommendations for using unique identifiers or indexes when necessary. The instructor also demonstrates rendering a list of components, managing keys, and accessing keys within components. Finally, a task is set to practice adding and removing list items in a table format, encouraging hands-on learning.
Takeaways
- 😀 This video is part of a React JS tutorial series aimed at beginners.
- 📚 The focus of the video is on rendering lists in React.
- 🔧 The presenter demonstrates creating an unordered list using a numbers array.
- 🔄 Different JavaScript loops are mentioned, but the map function is preferred in React for rendering lists.
- 🛠 The map function is favored because it returns a new array, unlike forEach which does not return anything.
- ⚠️ React warns about the importance of using unique 'key' props for list items to help identify changes in the list.
- 🔑 It's recommended to use a unique identifier from the data as a key, rather than the index, unless no other unique identifier is available.
- 🚫 React does not pass the 'key' prop into the components; it's only used internally by React.
- 💻 The video includes a practical example of rendering a list of 'Student' components with unique keys.
- 📝 The presenter assigns a task to the viewers to practice rendering lists in a table format, adding, and removing items.
Q & A
What is the main topic of the video?
-The main topic of the video is how to render lists in React JS, specifically for beginners learning React from scratch.
Why does the instructor prefer using the 'map' function over other loops in React?
-The instructor prefers using the 'map' function because it returns a new array by applying the callback function on each element of an array, which is more efficient and concise compared to other loops like 'for of' or 'forEach' that do not return a new array.
What is the significance of using a unique 'key' prop in a list of elements in React?
-Using a unique 'key' prop in a list of elements in React helps React to identify which items have changed, added, or removed, thus improving performance and providing hints for efficient updates to the DOM.
What should be the best practice for choosing a key in a list?
-The best practice for choosing a key is to use a unique identifier from the data, such as an ID from a database, as it helps maintain the identity of elements even when the order changes.
Why does React warn against using indexes as keys if the order of items may change?
-React warns against using indexes as keys if the order of items may change because it can negatively impact performance and cause issues with component state, as indexes do not provide a stable identity for elements.
How can you add a 'key' to an element in a list in React?
-You can add a 'key' to an element in a list by using the 'key' property on the element, such as `<li key={item.id}>`, where 'item.id' is a unique identifier for each list item.
What is the task given at the end of the video?
-The task is to create a table using the provided data, with text boxes for inputting a student's name, age, and country, and buttons to add and delete data from the table.
Why is it important to keep the students array in a state for the given task?
-It is important to keep the students array in a state for the task because it allows the UI to update dynamically when a student is added or removed, reflecting the changes in the list.
What is the purpose of the 'key' prop in React components?
-The 'key' prop in React components serves as a hint to React for identifying which items have changed, added, or removed, but it is not passed into the components as a prop. It is used internally by React for performance optimization.
How can you access the 'key' in a component if needed?
-If you need to access the 'key' inside a component, you should pass it as a different prop, as the 'key' prop itself is not passed to the component.
Outlines
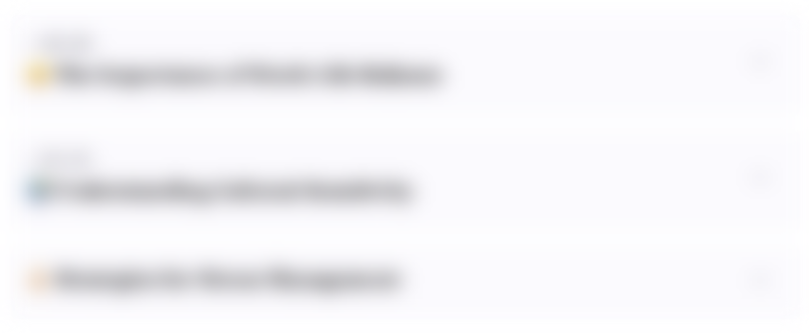
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
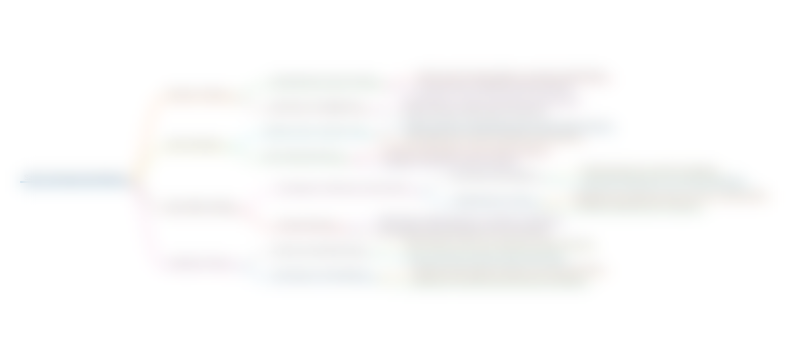
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
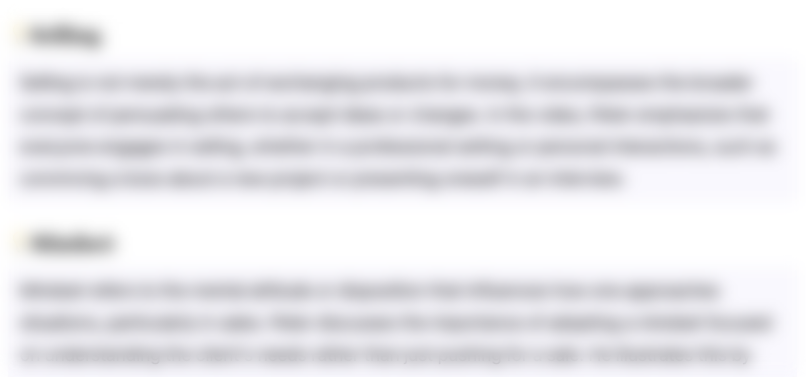
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
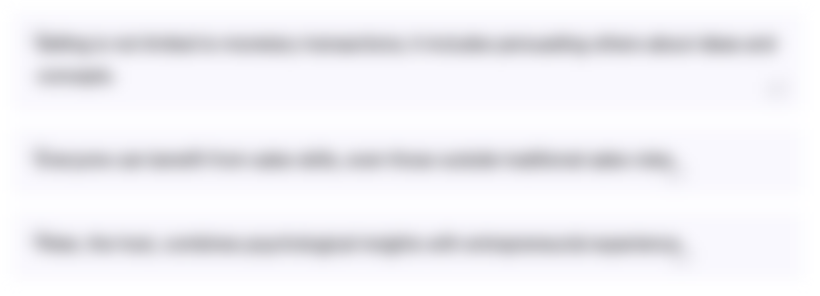
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
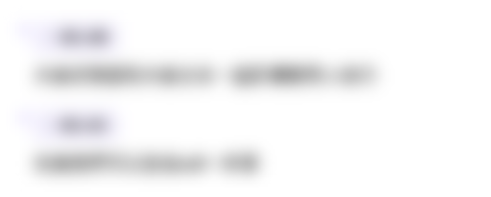
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
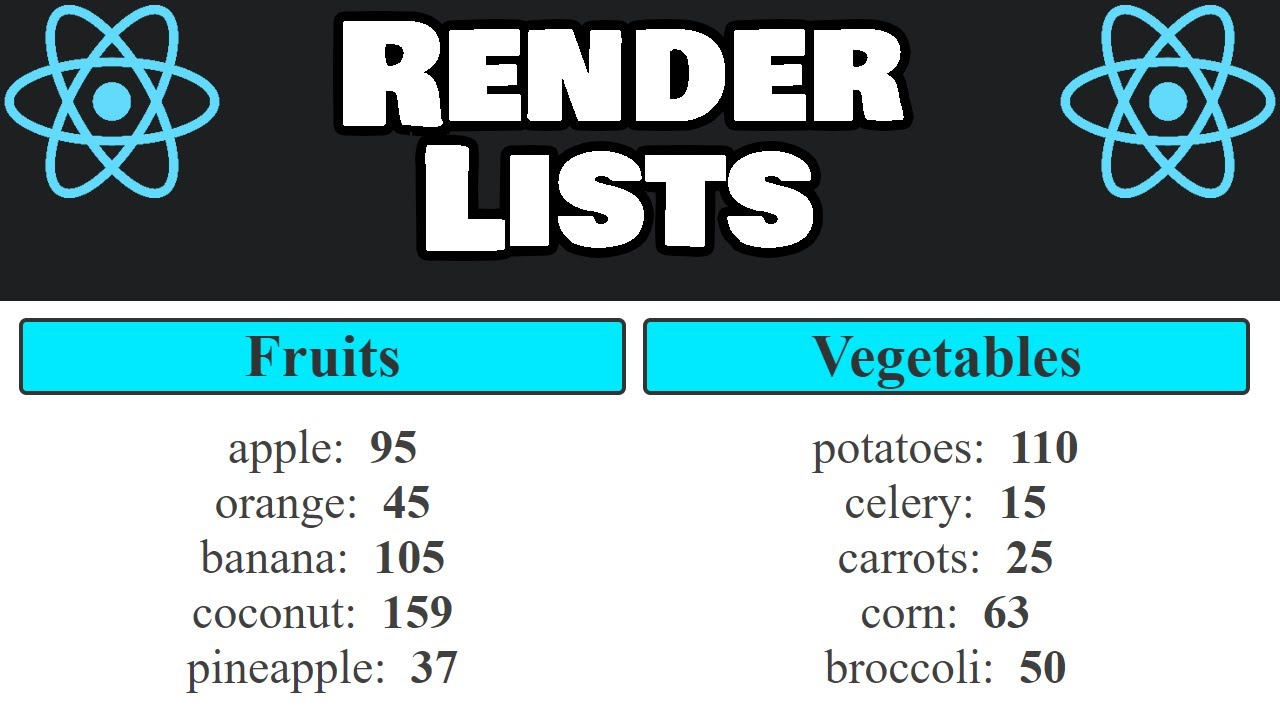
How to render LISTS in React 📃
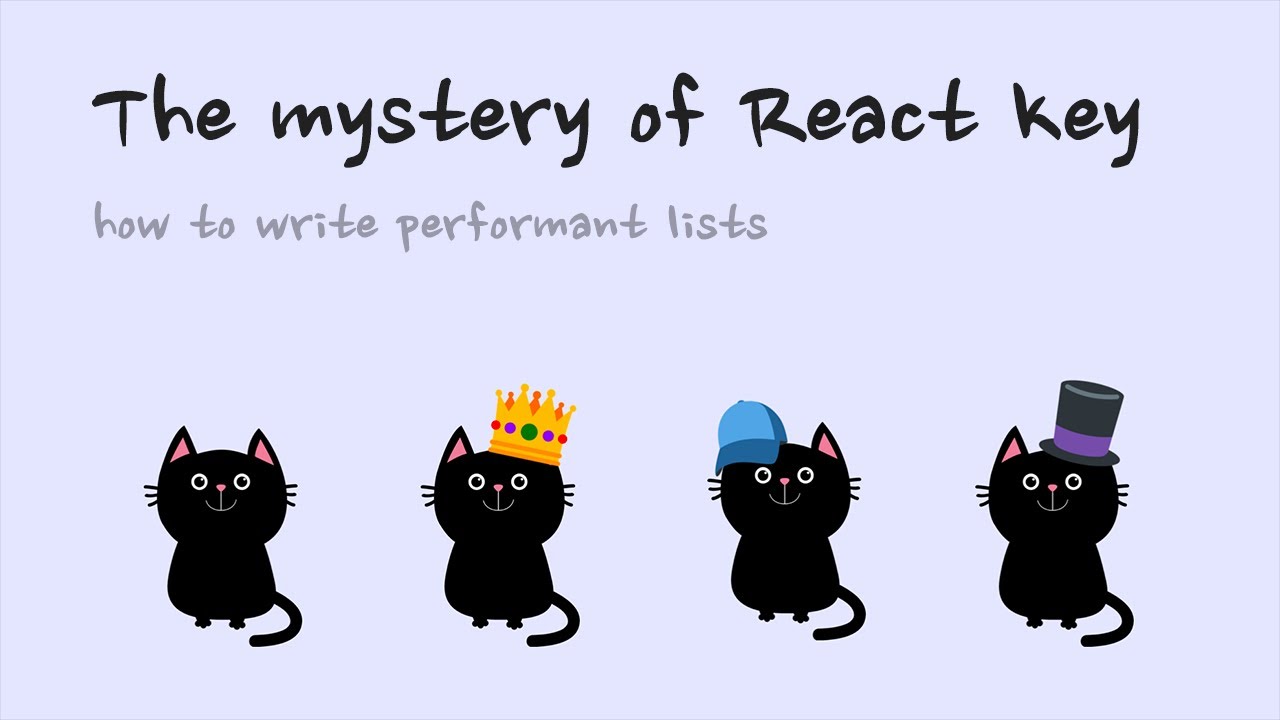
The mystery of React key: how to write performant lists
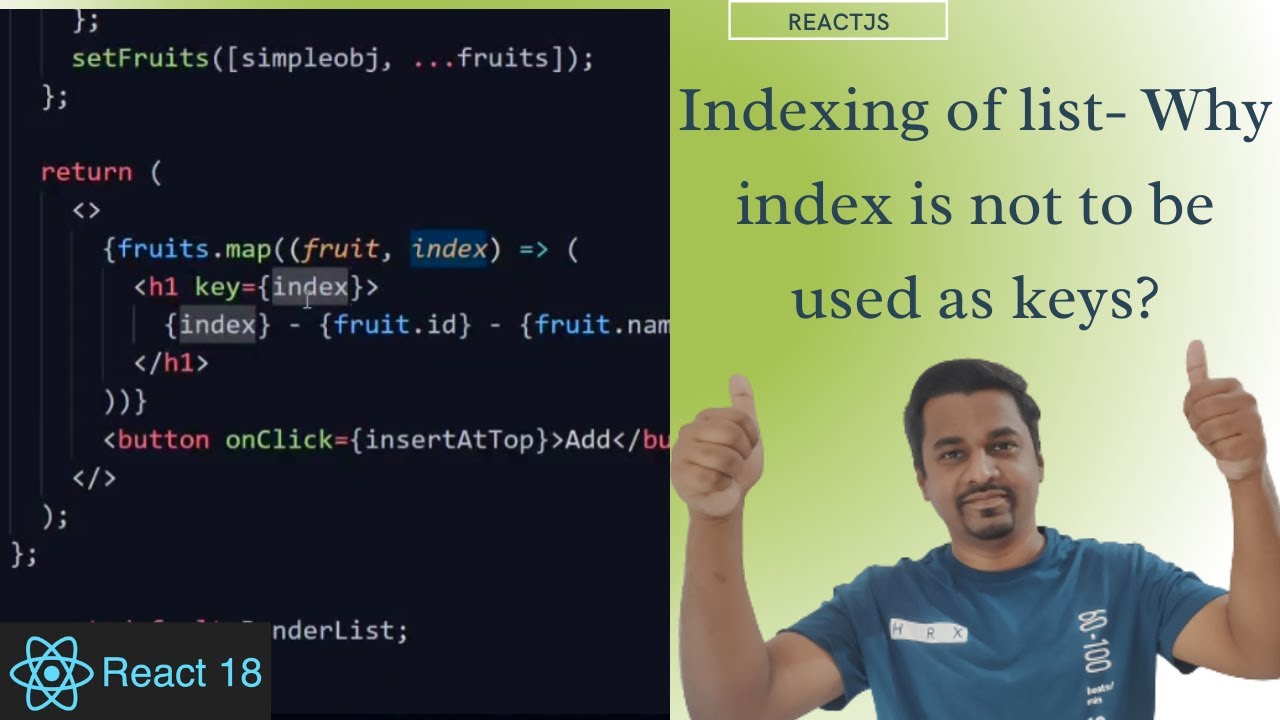
What is indexing of list? | Avoid using index as keys

React Keys and Lists - Complete Tutorial!

Conditional Rendering | Mastering React: An In-Depth Zero to Hero Video Series

useContext Hook | Mastering React: An In-Depth Zero to Hero Video Series
5.0 / 5 (0 votes)