Build a Microservice with Go #1 - Getting Started
Summary
TLDRThe video introduces using Go and the Chi web framework to build a microservice API. It starts by setting up a Go development environment, then builds a simple web server that responds "Hello World" to requests. It adds the Chi router for request routing and logging middleware for debugging. The video demonstrates handling requests and errors automatically with Chi. It sets up version control with Git and manages dependencies with Go modules. The goal is to end up with a basic CRUD microservice API connected to a database, built step-by-step over the course of the video series.
Takeaways
- 😀 The video will teach how to build a microservice API with Go and Chi
- 📝 Go is popular for building services like CLI tools, network apps and microservices
- 👨💻 The video will walk through building an API from start to finish
- 🛠️ Go has a rich standard library to help build web servers easily
- 🚦 Chi router matches paths and methods like GET and POST to handler functions
- 🔧 Logging middleware from Chi logs requests and responses out of the box
- 📦 go.mod manages dependencies and versions using decentralized Git repos
- 🖥️ The course is available ad-free on netninja.dev for $2
- 💳 Or get access to all Net Ninja courses with Net Ninja Pro for $9/month
- 👍 Don't forget to like, share and subscribe to support the channel
Q & A
What is the purpose of this video tutorial series?
-The purpose is to teach how to make a microservice with Go and the Chi framework, walking through building an API microservice from start to finish.
What version of Go is used in the tutorial?
-Version 1.20.5 of Go is used in the tutorial.
What is the go.mod file used for?
-The go.mod file is used for dependency management in Go. It specifies the Git repo that relates to the Go project.
How does the tutorial application print "Hello World"?
-It uses the fmt.Println function from the fmt package to print "Hello World".
What does the Chi router package provide?
-The Chi router package provides HTTP routing functionality that conforms to Go's HTTP handler interface in the standard library.
Why is the 404 response seen when first testing the Chi router?
-There were no handlers set up for the root path yet, so Chi automatically handles that and returns a 404 response.
What is middleware used for with the Chi router?
-Middleware packages from Chi, like the logging middleware, can be easily added to the router using the router.Use() method.
How are errors handled in the Go code?
-Errors are checked for nil values using if error syntax, and then handled in the code block, often by logging them.
Why is the API binary added to .gitignore?
-Build artifacts like binaries should not be committed to git, as they can quickly add unwanted files and bloat the repo size.
What is a pointer in Go syntax?
-A pointer in Go is denoted by an asterisk * and takes the memory address of a value rather than the value itself.
Outlines
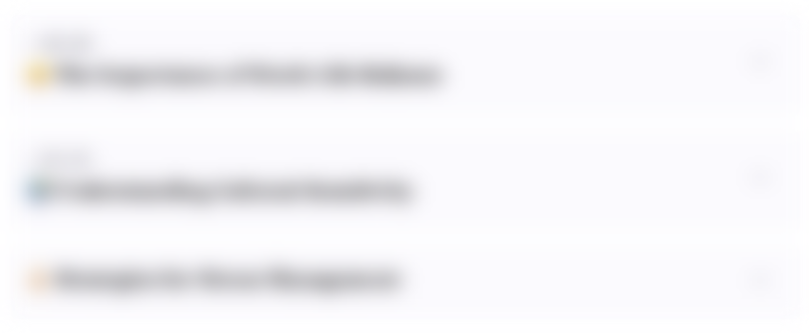
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
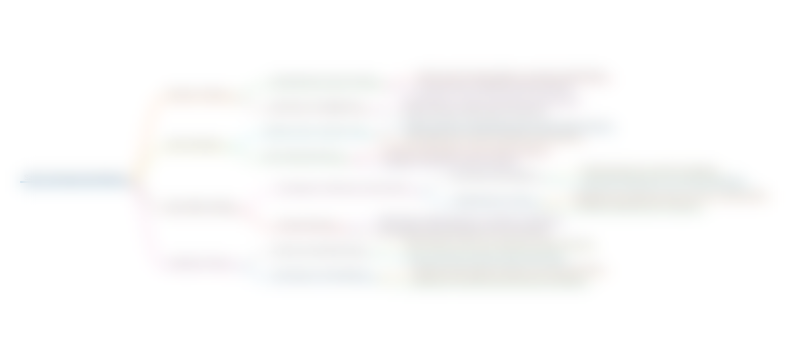
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
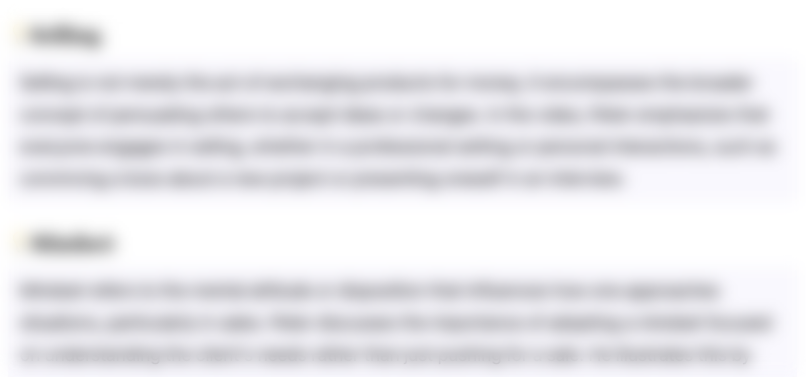
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
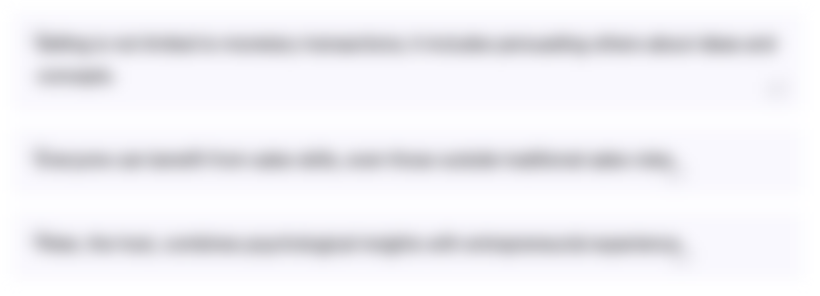
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
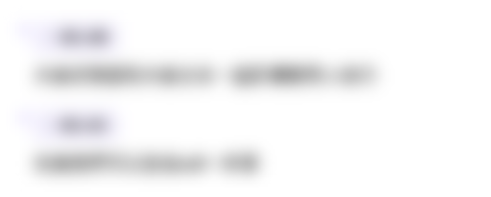
此内容仅限付费用户访问。 请升级后访问。
立即升级5.0 / 5 (0 votes)