Iterators in c++ | STL | Part-1/2 | OOPs in C++ | Lec-58 | Bhanu Priya
Summary
TLDRThis video explains iterators in the C++ Standard Template Library (STL), focusing on their role in accessing and manipulating container elements efficiently. The tutorial covers the syntax for declaring iterators for various containers (like vectors, lists, and maps) and demonstrates common operations such as `advance`, which moves iterators forward or backward by a specified number of steps. The video emphasizes the use of iterators to reduce program complexity and execution time, and provides practical code examples to help viewers understand how to implement and use these operations effectively in their own programs.
Takeaways
- 😀 Iterators in C++ are used to access and manipulate elements in STL containers without knowing their underlying structure.
- 😀 The primary purpose of iterators is to reduce program complexity and execution time.
- 😀 Iterators can be used with various STL containers like vectors, lists, maps, and more.
- 😀 To use an iterator, you first need to specify the container type and declare the iterator.
- 😀 The general syntax to declare an iterator is `container_type::iterator iterator_name;`.
- 😀 Iterators can point to different container types, such as `vector<int>`, `list<string>`, etc.
- 😀 The `advance()` function moves the iterator by a specified number of steps, either incrementing or decrementing it.
- 😀 The `next()` function returns an iterator one step ahead of the current iterator, without modifying the original iterator.
- 😀 The `prev()` function returns an iterator one step behind the current iterator, similar to `next()` but in the opposite direction.
- 😀 The `distance()` function calculates the number of steps between two iterators, helping to determine the size of a portion of a container.
- 😀 Iterators can be used to traverse and manipulate all types of sequences in C++ (integers, characters, strings, etc.), making them an essential tool for efficient programming.
Q & A
What are iterators in the Standard Template Library (STL)?
-Iterators in STL are objects that allow us to traverse through the elements of containers (like vectors, lists, and maps) by pointing to the memory addresses of these elements. They enable efficient access and manipulation of data within containers.
Why do we use iterators in STL?
-Iterators are used primarily to reduce the complexity and execution time of a program. They provide a uniform and efficient way to access and manipulate elements in STL containers.
What is the basic syntax for declaring an iterator in C++?
-The basic syntax for declaring an iterator is: `container_type::iterator iterator_name;`. For example, `vector<int>::iterator i;` declares an iterator `i` for a vector of integers.
What is the difference between `begin()` and `end()` in STL containers?
-`begin()` returns an iterator pointing to the first element of a container, while `end()` returns an iterator pointing just past the last element, essentially marking the end of the container.
What does the `advance()` function do with iterators?
-The `advance()` function increments or decrements an iterator by a specified number of positions. If the distance is positive, the iterator moves forward; if negative, it moves backward.
What will happen if a negative value is passed to the `advance()` function?
-If a negative value is passed to the `advance()` function, the iterator is decremented by that value, effectively moving it backwards in the container.
What is the purpose of the `distance()` function when working with iterators?
-The `distance()` function calculates and returns the number of elements between two iterators, helping to determine the size or the position difference between two points in a container.
How does the `next()` function work in STL iterators?
-The `next()` function moves the iterator forward by a specified number of steps and returns the new iterator. It is useful for accessing the next element without modifying the original iterator.
What is the `prev()` function used for in STL iterators?
-The `prev()` function moves the iterator backward by a specified number of steps and returns the new iterator. It is often used when you need to access previous elements in a container.
Can iterators be used with all STL containers?
-Yes, iterators can be used with most STL containers such as vectors, lists, deques, maps, and others. However, the types of iterators (e.g., random access, bidirectional) depend on the type of container.
Outlines
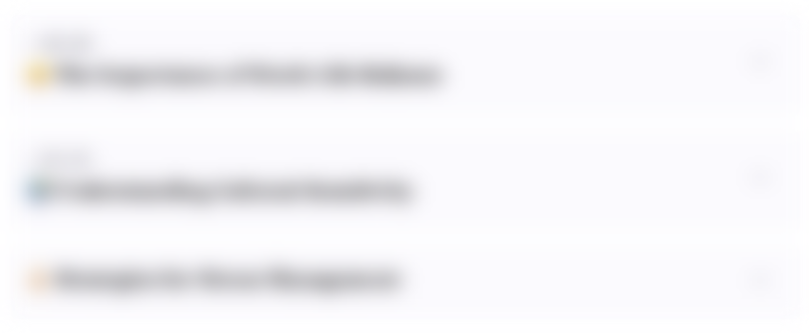
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
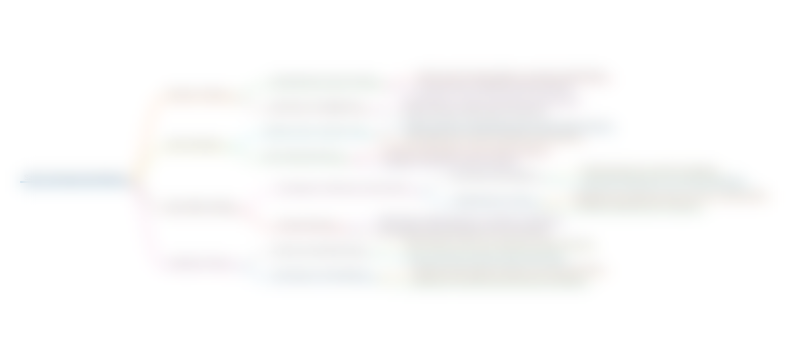
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
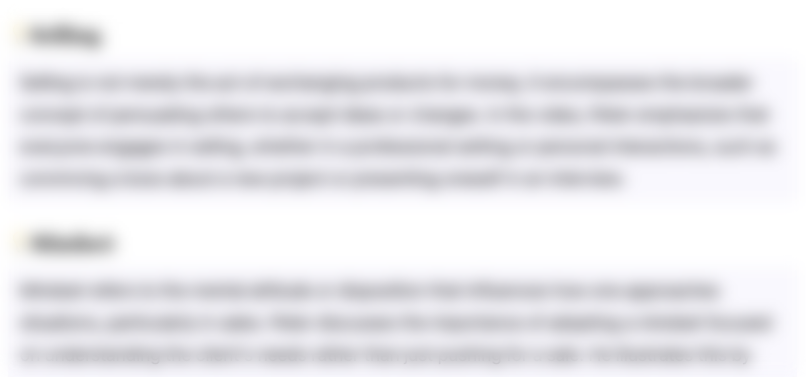
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
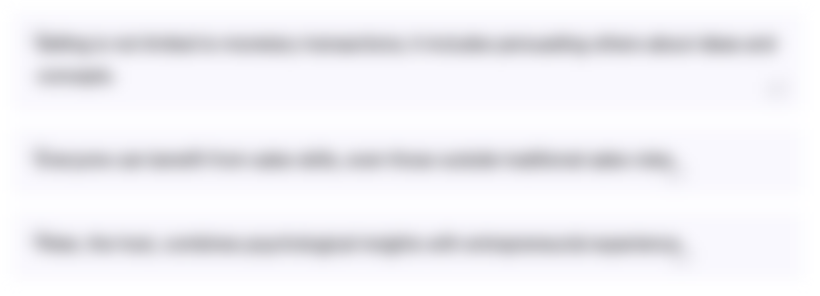
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
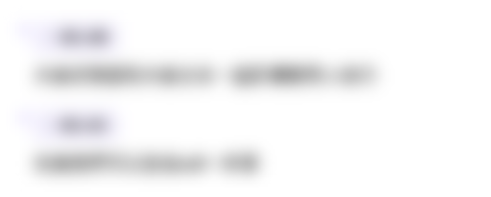
此内容仅限付费用户访问。 请升级后访问。
立即升级浏览更多相关视频

Pointers and Dynamic Memory in C++ (Memory Management)

Streams

#17 C Standard Library Functions | C Programming For Beginners

How are Containers Loaded? | Cargo Operations on Container Ship
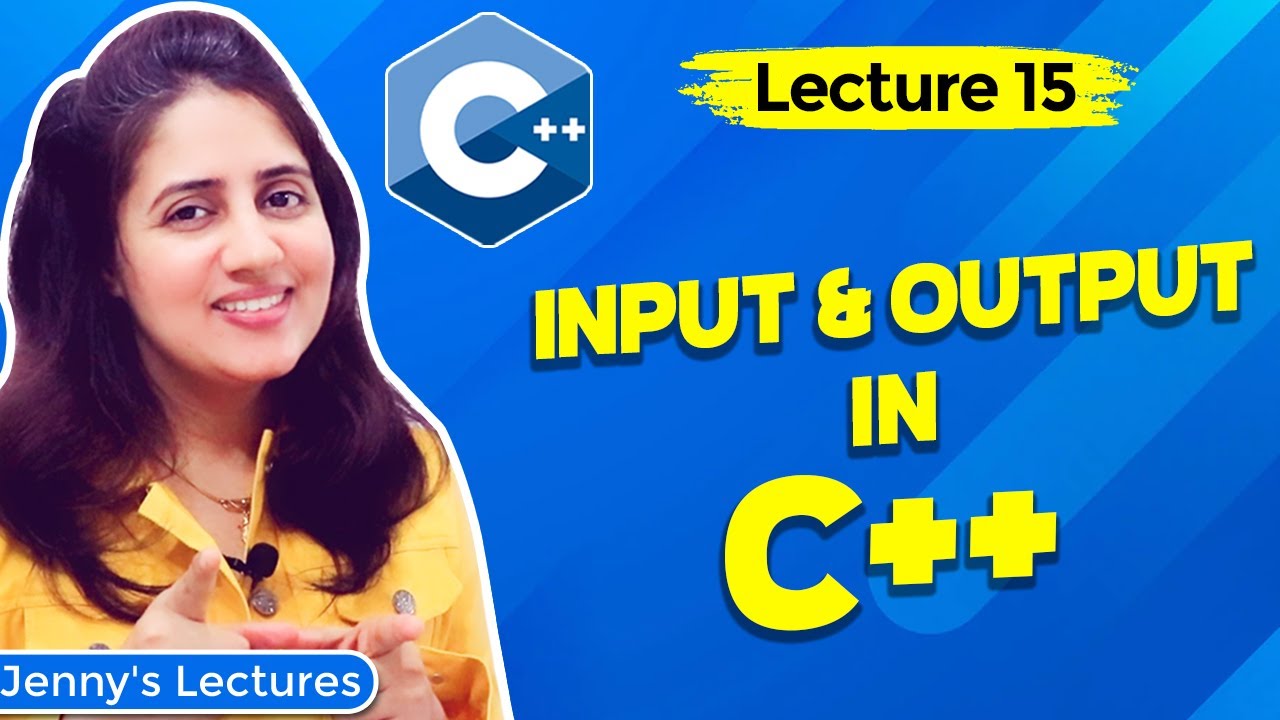
Lec 15: Input and Output in C++ | C++ Tutorials for Beginners
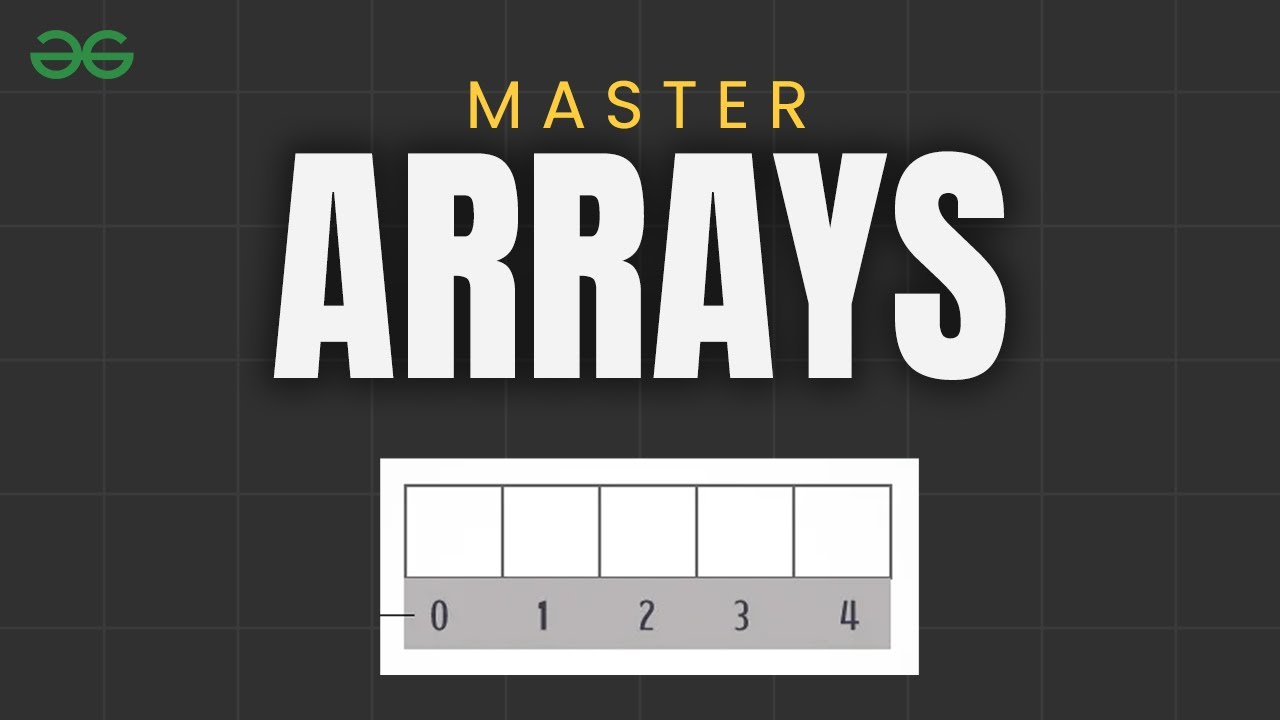
WHAT IS ARRAY? | Array Data Structures | DSA Course | GeeksforGeeks
5.0 / 5 (0 votes)