PBO 2 - JAVA - Liskov Substitution
Summary
TLDRIn this programming lesson, the instructor delves into the Liskov Substitution Principle (LSP) using Java, focusing on inheritance and interfaces. Through practical examples, such as vehicles and documents, the lesson emphasizes the importance of maintaining functional consistency in subclasses and the proper use of interfaces to ensure code reliability. Key concepts include the correct implementation of methods in subclasses and avoiding violations of LSP, illustrated through real-world coding scenarios that enhance understanding and application of object-oriented programming principles.
Takeaways
- 😀 The Liskov Substitution Principle (LSP) emphasizes that subclasses should be substitutable for their base classes without altering the desirable properties of the program.
- 😀 The importance of proper inheritance and ensuring that derived classes adhere to the functionality of their base class was discussed.
- 😀 Practical coding examples were provided to illustrate how to implement LSP using Java, including the creation of vehicle classes and methods.
- 😀 The concept of interfaces was introduced as a solution for classes that may not share common functionality, allowing for greater flexibility in design.
- 😀 When extending classes, the functionality of the superclass should not be compromised, especially regarding method parameters and behavior.
- 😀 The difference between classes that represent vehicles with engines and those without (like bicycles) highlights the necessity of clear class design.
- 😀 Interfaces can be used to define common behaviors while allowing for specific implementations in subclasses, promoting better coding practices.
- 😀 The session also covered practical issues in document management, differentiating between editable and read-only documents as an analogy for LSP.
- 😀 Code snippets demonstrated how to structure classes and interfaces effectively, reinforcing concepts through hands-on examples.
- 😀 Overall, the session emphasized that adherence to LSP is crucial for maintaining code quality and preventing future issues in software development.
Q & A
What is the main topic discussed in the transcript?
-The main topic is the Liskov Substitution Principle (LSP) in object-oriented programming, specifically how it relates to inheritance and method overriding in Java.
What does the term 'substitution' refer to in the context of LSP?
-In the context of LSP, 'substitution' refers to the idea that objects of a superclass should be replaceable with objects of a subclass without altering the correctness of the program.
Why is it important to ensure subclasses do not alter the functionality of superclass methods?
-It is important to maintain the expected behavior of superclass methods in subclasses to ensure that code remains reliable and can be used interchangeably without introducing errors.
What example is used to illustrate the principle in the lesson?
-The example used is a class hierarchy involving vehicles, specifically a superclass 'Vehicle' with subclasses like 'Car' and 'Bicycle'. The issue arises when a subclass does not align with the expected functionality of its superclass.
What is the problem with having a 'Bicycle' class inherit from 'Vehicle' when using LSP?
-The problem is that a 'Bicycle' does not have an engine, which makes it inappropriate to implement a method like 'startEngine' that is expected to be in the 'Vehicle' superclass.
How does the lesson suggest resolving the issue with inappropriate inheritance?
-The lesson suggests creating an interface for vehicles with engines, allowing classes like 'Car' to implement it while keeping 'Bicycle' separate, thus adhering to the LSP.
What is the significance of using interfaces in this context?
-Using interfaces helps to define a contract for functionality that certain classes must fulfill, allowing for more flexible and appropriate inheritance structures that respect LSP.
What is an example of a class that can extend 'Document' as mentioned in the transcript?
-An example given is a 'ReadOnlyDocument' class that can open documents but cannot save them, contrasting with a 'EditableDocument' class that can both open and save documents.
What coding principle does the transcript emphasize through its examples?
-The transcript emphasizes the importance of adhering to the Liskov Substitution Principle to ensure that subclasses maintain the integrity and expected behavior of their superclasses.
How can violations of LSP impact software development?
-Violations of LSP can lead to unexpected behaviors, bugs, and a fragile codebase, making it harder to maintain and extend the software reliably.
Outlines
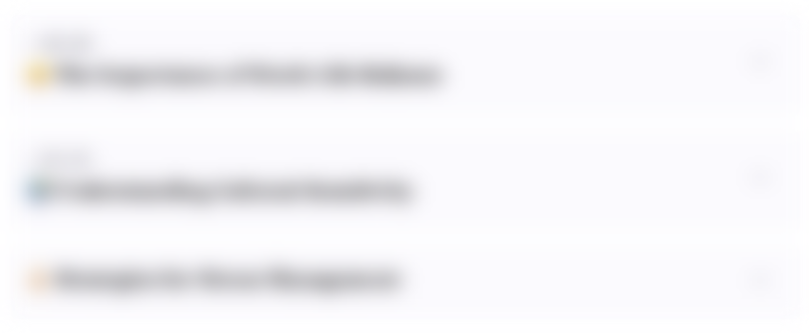
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
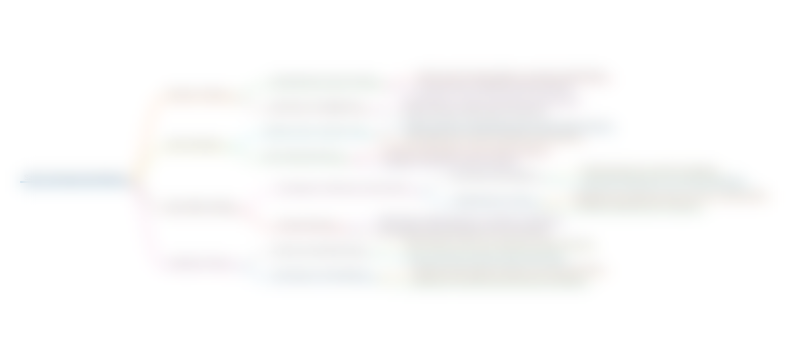
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
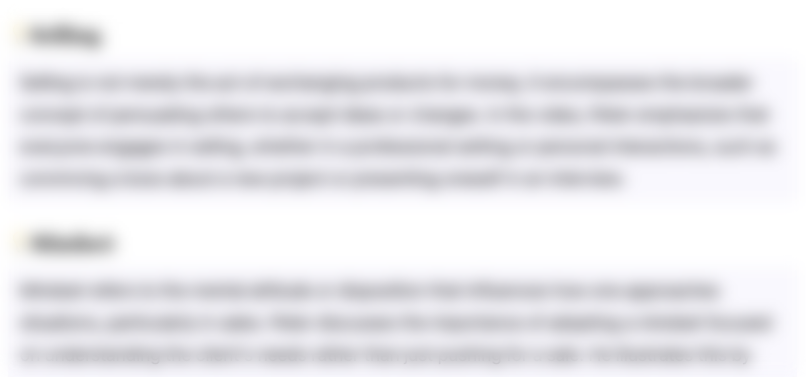
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
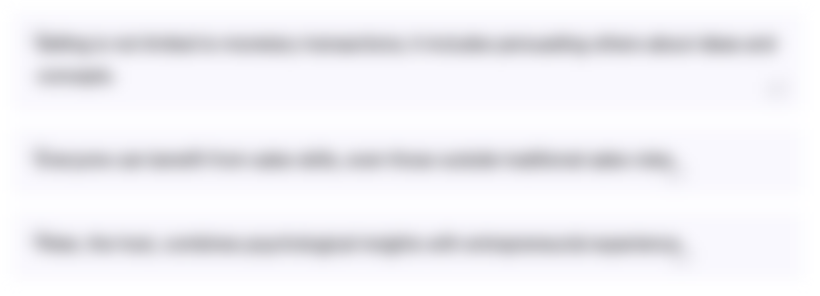
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
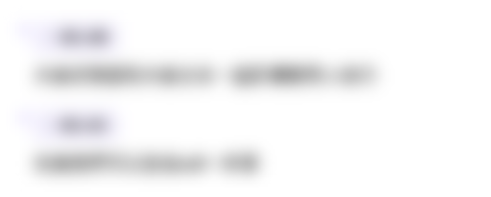
此内容仅限付费用户访问。 请升级后访问。
立即升级浏览更多相关视频
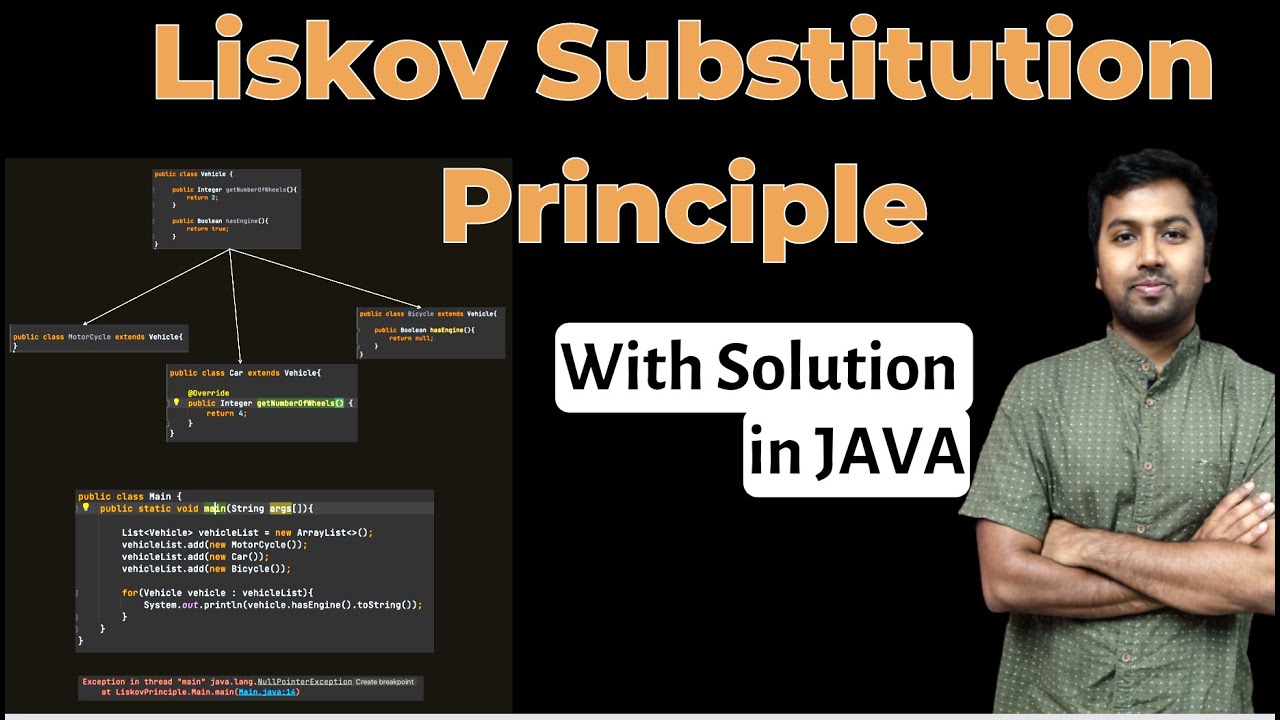
1.1 Liskov Substitution Principle (LSP) with Solution in Java - SOLID Principles of Low Level Design

Inheritance in Java - Java Inheritance Tutorial - Part 1
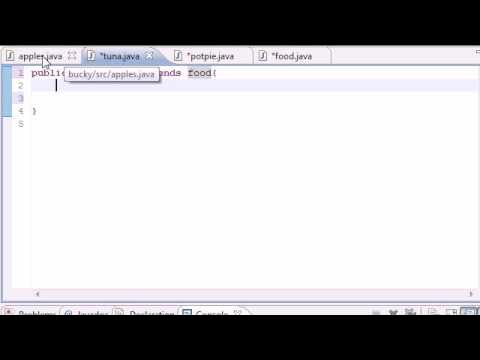
Java Programming Tutorial - 49 - Inheritance
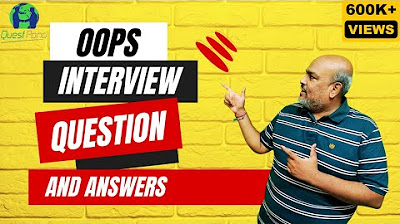
OOPS Interview Questions and Answers | Object Oriented Programming Interview Questions C#
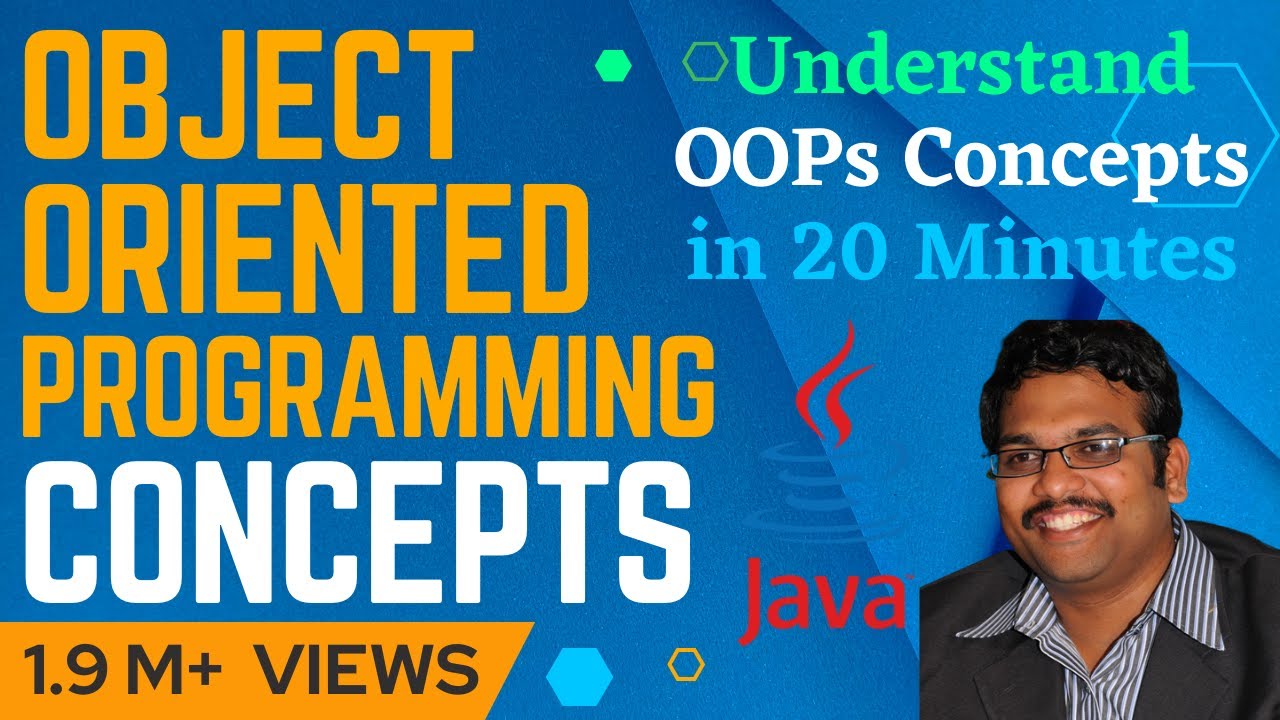
OOPS CONCEPTS - JAVA PROGRAMMING
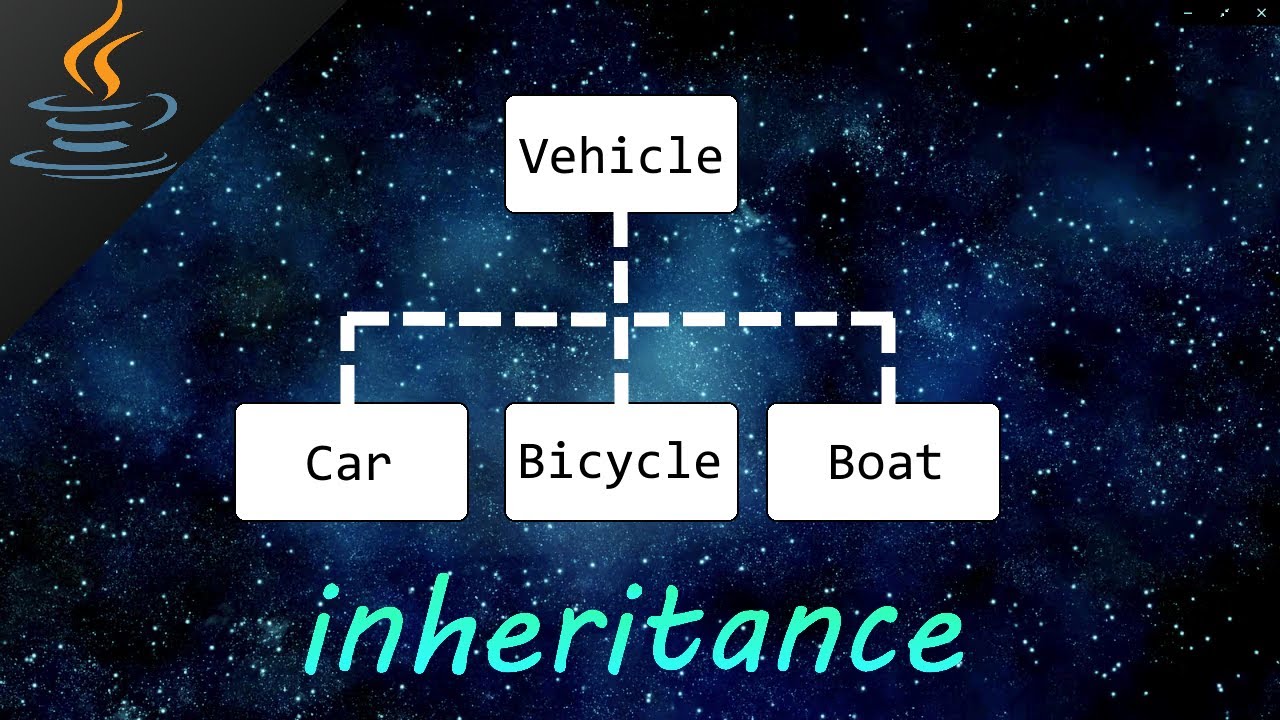
Java inheritance 👪
5.0 / 5 (0 votes)