Object Oriented Programming Using Python [With Examples] - Part 2 | The Knowledge Academy
Summary
TLDRThis video from the Knowledge Academy delves into the four fundamental concepts of object-oriented programming: encapsulation, abstraction, inheritance, and polymorphism. It explains encapsulation's role in data protection through controlled access, abstraction's ability to simplify complex systems, inheritance for promoting code reuse, and polymorphism allowing diverse classes to be treated uniformly. With practical Python examples, viewers gain insights into how these principles enhance code modularity and maintainability. The session concludes with a preview of an upcoming project that will further illustrate these concepts in action.
Takeaways
- 😀 Encapsulation bundles data and methods into a class, hiding the internal state and ensuring controlled interaction through interfaces.
- 😀 It enhances code modularity and maintainability, making it easier to manage changes in dynamic environments.
- 😀 In Python, encapsulation is achieved using classes, which define attributes and methods for objects.
- 😀 Directly modifying an object's attributes without encapsulation can lead to unintended consequences, like incorrect data values.
- 😀 Abstraction hides complex implementation details and presents only essential features, simplifying system management.
- 😀 Abstract classes in Python serve as blueprints for child classes, ensuring they implement specific methods.
- 😀 Inheritance allows new classes to inherit properties and behaviors from existing classes, promoting code reuse.
- 😀 The 'super' function simplifies inheritance by allowing subclasses to call parent class methods, ensuring proper initialization.
- 😀 Polymorphism enables different classes to be treated as instances of a common superclass, allowing them to respond uniquely to the same method.
- 😀 The video concludes with an invitation to explore a beginner-level project that will apply the concepts of object-oriented programming.
Q & A
What is encapsulation in object-oriented programming?
-Encapsulation is the bundling of data and methods that operate on the data into a single unit, known as a class, which helps hide the internal state and requires all interactions to be performed through well-defined interfaces.
Why is encapsulation important?
-Encapsulation is crucial as it promotes modular and maintainable code, allowing developers to modify data in a controlled manner and making the code easier to understand and debug.
How can encapsulation be achieved in Python?
-In Python, encapsulation is achieved using classes, which define the attributes and methods of objects. Private attributes can be created using name mangling with an underscore prefix.
What is abstraction in the context of object-oriented programming?
-Abstraction is the process of hiding complex implementation details and exposing only the essential features of an object, enabling developers to focus on what an object does rather than how it does it.
How does inheritance facilitate code reuse?
-Inheritance allows a new class to inherit properties and behaviors from an existing class, reducing code duplication and promoting a more modular and organized codebase.
What role does the 'super' function play in inheritance?
-The 'super' function allows a subclass to call methods from its parent class, ensuring that the initialization logic from the base class is executed and maintaining consistency.
Can you explain polymorphism?
-Polymorphism allows objects of different classes to be treated as objects of a common superclass, enabling flexibility in the code as different objects can respond to the same method in unique ways.
How is polymorphism implemented in Python?
-Polymorphism in Python is often achieved through method overriding and duck typing, where objects are evaluated based on their behavior rather than their specific type.
What is the significance of abstract classes in abstraction?
-Abstract classes serve as blueprints for other classes, defining essential methods that must be implemented by subclasses, thus enforcing a common interface for code reusability and organization.
How does encapsulation improve data integrity?
-By encapsulating attributes and providing controlled access methods, encapsulation ensures that data manipulation occurs through defined channels, safeguarding the integrity of the data against unwanted changes.
Outlines
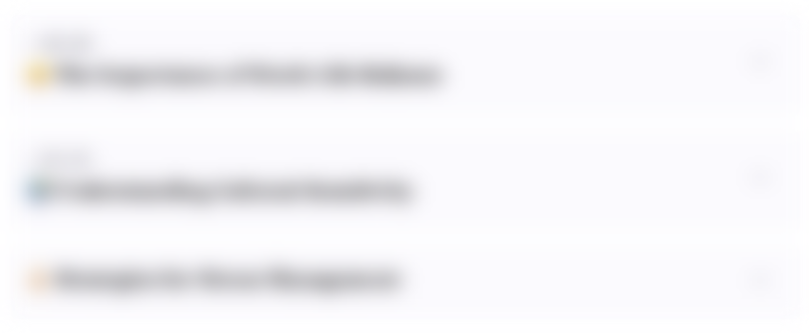
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
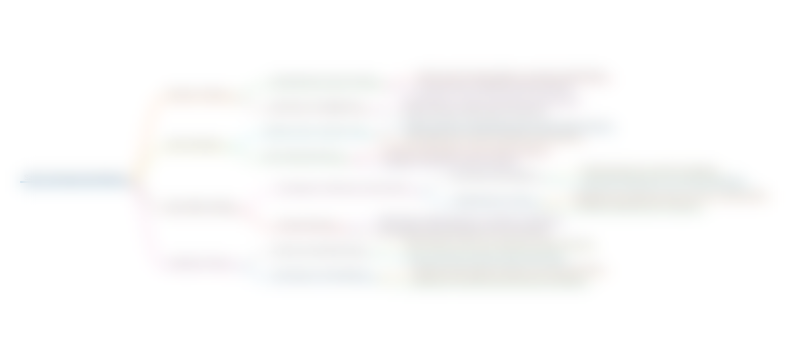
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
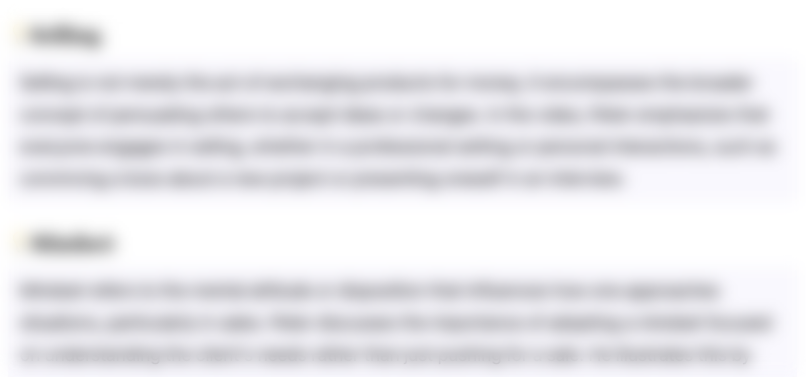
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
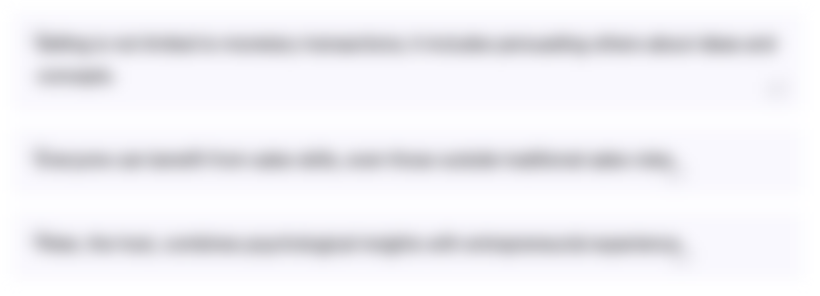
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
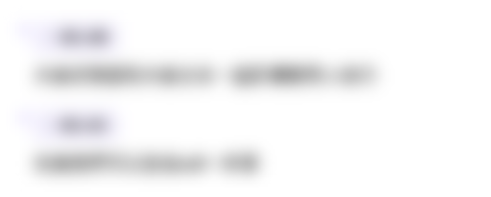
此内容仅限付费用户访问。 请升级后访问。
立即升级浏览更多相关视频
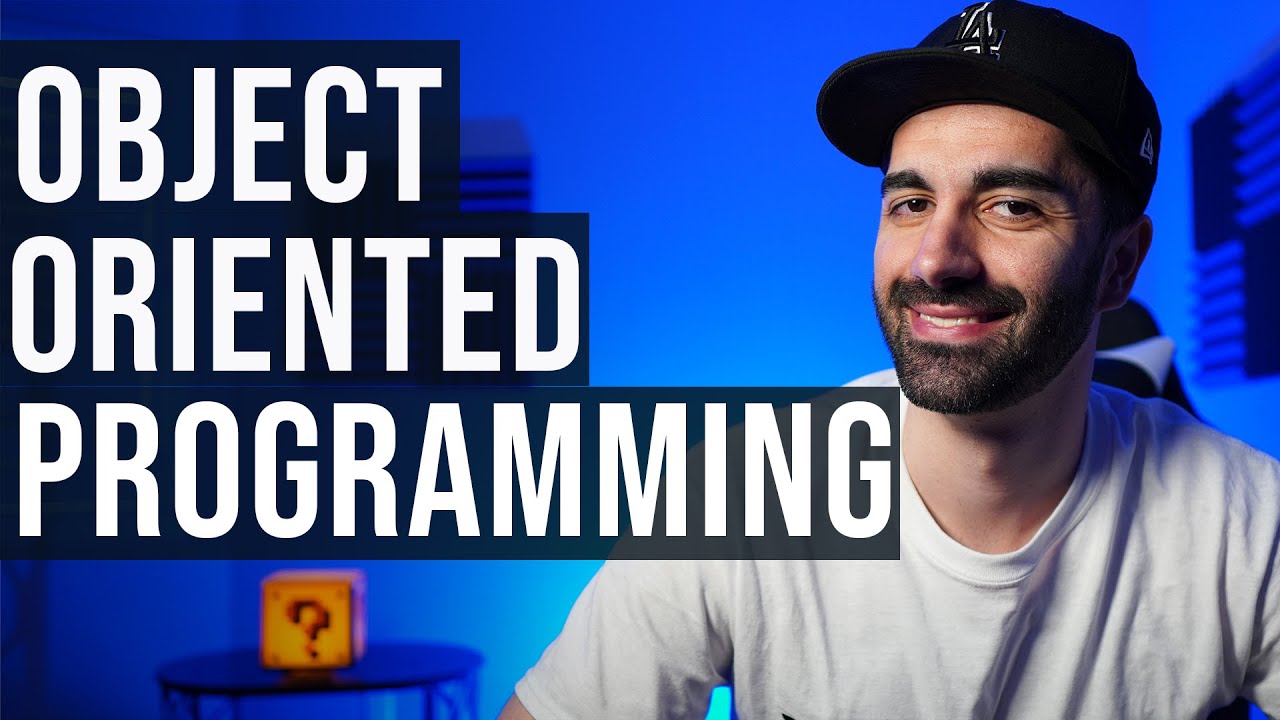
Object Oriented Programming - The Four Pillars of OOP
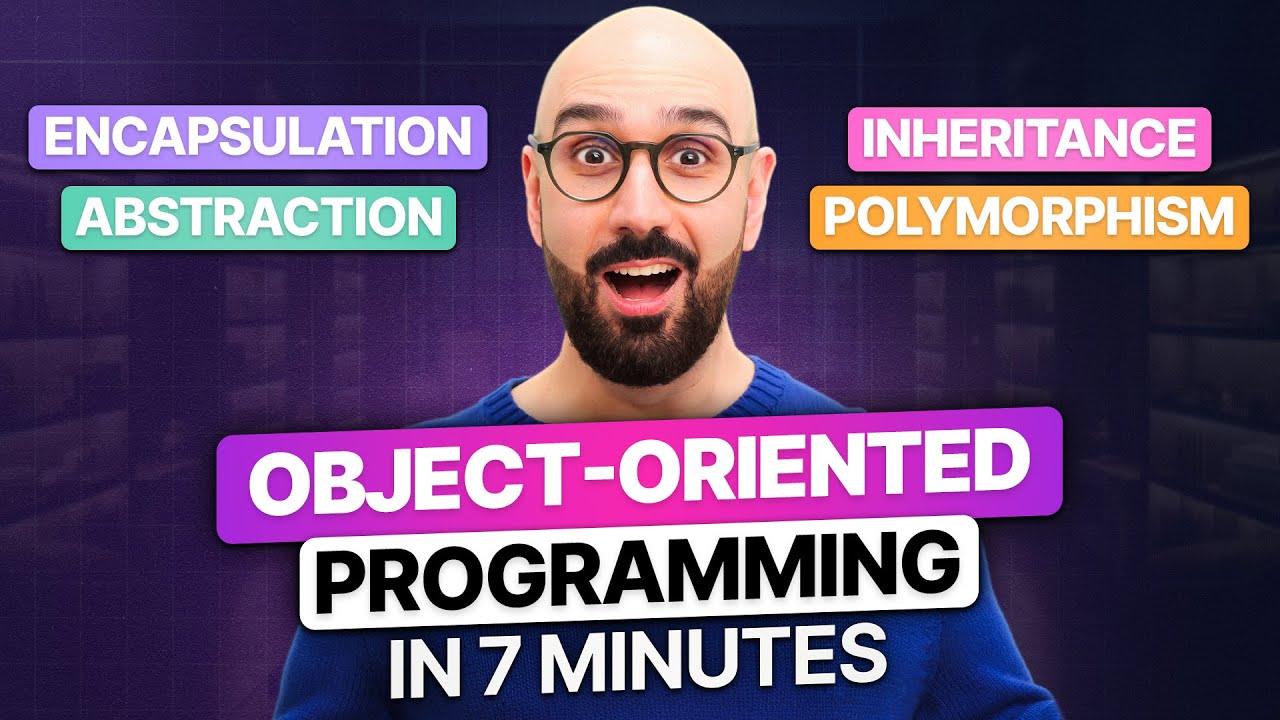
Object-oriented Programming in 7 minutes | Mosh
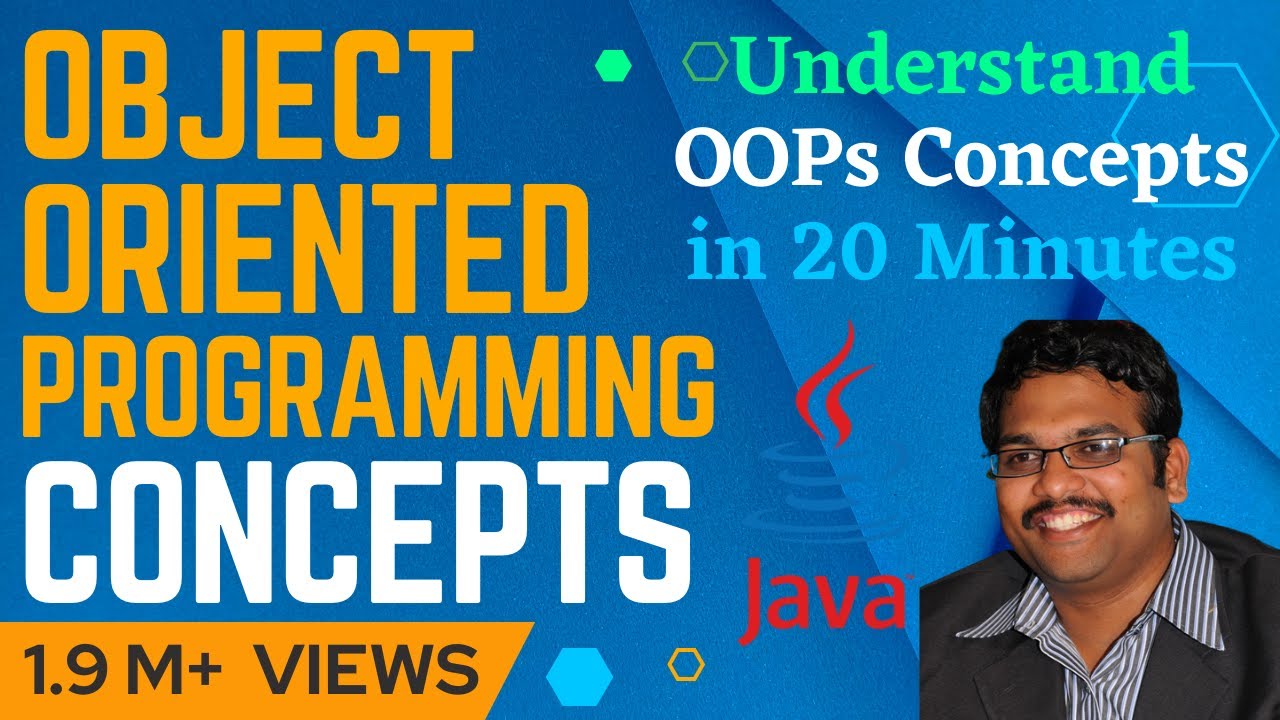
OOPS CONCEPTS - JAVA PROGRAMMING

Fundamental Concepts of Object Oriented Programming
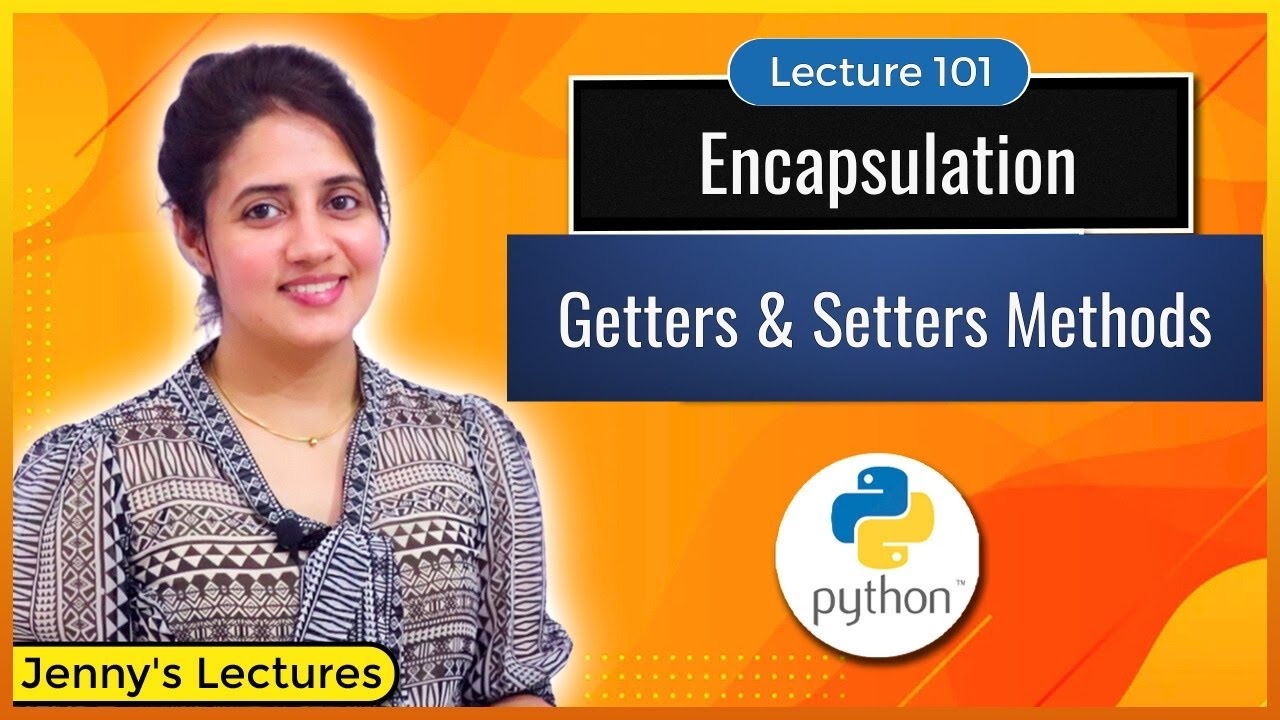
Encapsulation in Python | Getters & Setters methods | Python Tutorials for Beginners #lec101

Lec 3: OOPs Concepts in C++ | Object Oriented Programming Pillars | C++ Tutorials for Beginners
5.0 / 5 (0 votes)