WHAT IS ARRAY? | Array Data Structures | DSA Course | GeeksforGeeks
Summary
TLDRThis video offers a clear introduction to arrays, one of the most fundamental data structures in computer science. It explains the basics of arrays, including their definition as collections of similar items in contiguous memory, and discusses both static and dynamic arrays. The video also covers how to declare, insert, and access array elements in C, C++, and Java. Additionally, it explains why accessing array elements has a constant time complexity of O(1) by showing how memory addressing works. Viewers will gain a foundational understanding of arrays and their importance.
Takeaways
- 📦 Arrays are a collection of similar items stored in contiguous memory locations.
- 🧰 Arrays are the foundation of many data structures like stacks and queues.
- 🔢 An array is like a row of boxes, each capable of storing an element of the same data type.
- 🧮 Array indices start at 0 and go up to size minus 1, mapping values to specific indexes.
- 🏢 Contiguous memory allocation means that each array element is stored in adjacent memory locations.
- 🗂️ Arrays can be static (fixed size) or dynamic (can grow in size).
- 🖥️ Syntax for declaring arrays varies between languages like C, C++, and Java, with differences in how the size is declared and assigned.
- 💾 Elements in an array can be inserted using their index, and the values can be accessed by iterating over the array.
- ⏱️ Accessing elements in an array takes constant time, O(1), because the memory address is calculated using a base address and an offset.
- 📊 Arrays are a core concept in computer science due to their efficiency and structure.
Q & A
What is an array?
-An array is a collection of similar items stored in contiguous memory locations. It is a fundamental data structure used to store elements of the same data type, like integers or floats.
Why are arrays considered a fundamental data structure?
-Arrays are considered fundamental because they are the basis for other data structures like stacks and queues. They are commonly taught first in introductory computer science courses due to their simplicity and wide usage.
How are the elements in an array accessed?
-Array elements are accessed using indices, which typically start from 0 and go up to the size of the array minus one. You can access elements by specifying the index inside square brackets.
What is meant by 'contiguous memory allocation' in arrays?
-Contiguous memory allocation means that the memory for array elements is allocated in a continuous block. Each element is stored next to the previous one in memory, similar to adjacent apartments in a building.
What is the difference between static arrays and dynamic arrays?
-Static arrays have a fixed size that is determined at compile time and cannot be changed. Dynamic arrays, on the other hand, can grow in size during runtime by reallocating memory and copying existing elements into a new, larger array.
How is an array declared in C/C++?
-In C/C++, an array is declared by first specifying the data type, followed by the array name and the size inside square brackets. For example: `int arr[3];` declares an array of size 3.
How is an array declared in Java?
-In Java, the array declaration syntax is slightly different. It includes the `new` keyword. For example: `int[] arr = new int[3];` declares an array of size 3.
How do you insert an element into an array?
-To insert an element, you specify the array name followed by the index inside square brackets and assign a value. For example: `arr[5] = 13;` assigns the value 13 to the index 5 of the array.
How does accessing an array element take constant time (O(1))?
-Accessing an array element takes constant time because the address of any element can be calculated using a simple formula: `Base Address + (Index * Size of Element)`. This direct calculation allows immediate access without looping or searching.
Why does an array use memory in a contiguous manner?
-An array uses contiguous memory to ensure that elements are stored together, making it easy to compute their addresses and access them quickly. This helps in efficient memory usage and faster access times.
Outlines
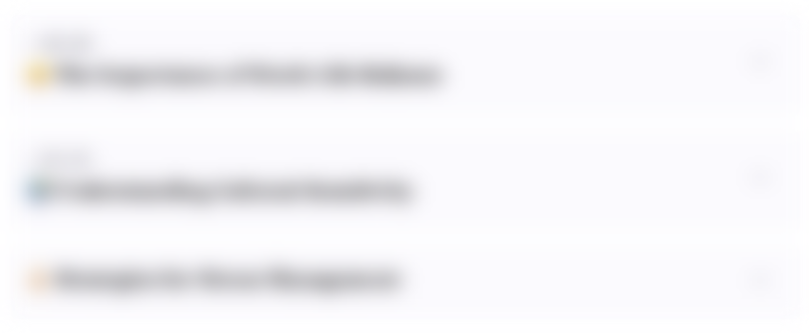
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
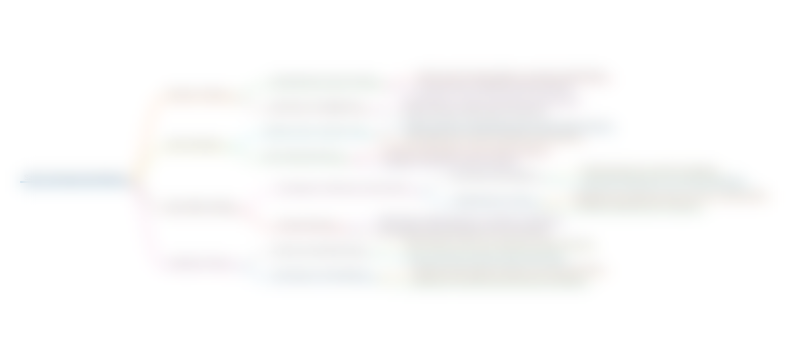
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
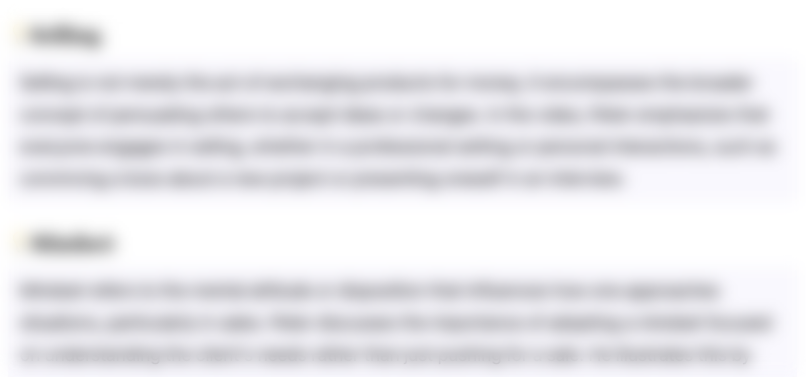
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
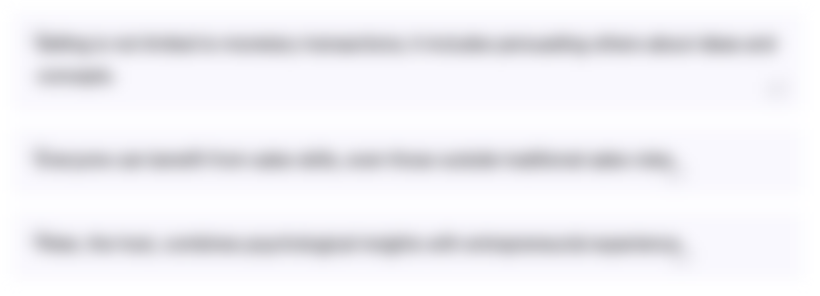
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
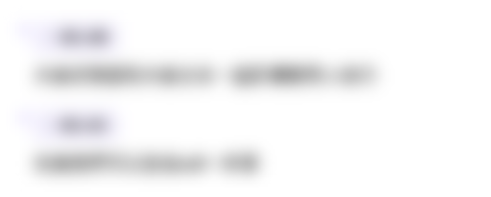
此内容仅限付费用户访问。 请升级后访问。
立即升级5.0 / 5 (0 votes)