Top 7 Data Structures for Interviews Explained SIMPLY
Summary
TLDRThis video script offers an insightful overview of seven fundamental data structures, essential for coding interviews, computer science studies, and project development. It simplifies concepts like arrays, linked lists, hash maps, stacks, queues, trees, and graphs, highlighting their unique properties and use cases. The script also touches on time complexity and memory allocation, providing a foundational understanding for beginners and a refresher for experienced programmers.
Takeaways
- ๐งฉ Arrays are ordered collections of data, typically of similar types, and are essential for beginners to learn.
- ๐ Arrays use zero-based indexing, making it easy to read elements but slower for inserting and deleting elements.
- ๐ Linked lists store ordered data with pointers to the next element, making insertion and deletion faster but reading slower.
- ๐ Hash maps (or hash tables/dictionaries) allow for custom keys, making searching, inserting, and removing elements very fast.
- ๐ง Stacks are LIFO (last in, first out) structures, similar to a stack of plates, with fast operations for adding, removing, and viewing elements.
- ๐ Queues are FIFO (first in, first out) structures, like a grocery store line, with efficient operations for adding to the back and removing from the front.
- ๐ณ Binary search trees are a type of binary tree where left children are less than the parent and right children are greater, facilitating quick searches.
- ๐ Binary search trees are useful for searching through large ordered datasets efficiently, like looking up words in a dictionary.
- ๐ Graphs are models of connections with nodes and edges, which can be directed or undirected, weighted, and can include cycles, making them complex but versatile.
- ๐ Graphs are practical for real-world applications like finding the shortest route for errands or optimizing ride-sharing services like Uber.
Q & A
What are the 7 most important data structures mentioned in the script?
-The 7 most important data structures mentioned are Arrays, Linked Lists, HashMap, Stacks, Queues, Trees (specifically Binary Search Trees), and Graphs.
Why are arrays considered the most important data structure to learn first?
-Arrays are considered the most important data structure to learn first because they are used all the time for pretty much everything, and they make it easy to read elements with a time complexity of O(1).
What is the main advantage of using an array?
-The main advantage of using an array is that it's very easy to find any element due to the index assigned to each element, allowing for constant time complexity (O(1)) when accessing elements.
What is the time complexity for inserting or deleting elements in an array?
-The time complexity for inserting or deleting elements in an array is O(n), as it may require shifting elements and potentially reallocating memory.
How does the memory allocation of arrays differ from linked lists?
-Arrays are stored in contiguous memory locations, while elements in a linked list are not required to be stored next to each other due to the use of pointers to the next element.
What is the main advantage of linked lists over arrays in terms of element manipulation?
-The main advantage of linked lists over arrays is that they are faster at inserting or deleting elements, as they do not require reallocation or shifting of elements.
How does a hash map differ from an array in terms of accessing elements?
-A hash map differs from an array in that it uses keys to access elements instead of indexes, and it allows for quick searching with a time complexity of O(1) on average.
What is the time complexity for searching in a hash map?
-The time complexity for searching in a hash map is O(1) on average, assuming a good hash function and no excessive collisions.
What is the main characteristic of a stack in terms of element order?
-A stack is a LIFO (Last In, First Out) structure, meaning the last element added is the first one to be removed.
What are the three common operations associated with stacks?
-The three common operations associated with stacks are push (adding an element), pop (removing the top element), and peek (viewing the top element without removing it).
How does a queue differ from a stack in terms of element order?
-A queue is a FIFO (First In, First Out) structure, meaning the first element added is the first one to be removed, which is the opposite of a stack.
What are the three common operations associated with queues?
-The three common operations associated with queues are enqueue (adding an element to the back), dequeue (removing the front element), and front (viewing the front element without removing it).
What is the main advantage of using binary search trees for searching through ordered values?
-The main advantage of using binary search trees for searching through ordered values is that they allow for efficient searching with a time complexity of O(log n) by eliminating half of the remaining elements with each comparison.
What is a real-life example of a data structure that resembles a graph?
-A real-life example of a data structure that resembles a graph is a transportation network, where nodes represent locations and edges represent the roads connecting them, with weights indicating distances or travel times.
What is the main characteristic that distinguishes directed graphs from undirected graphs?
-The main characteristic that distinguishes directed graphs from undirected graphs is that in directed graphs, the edges have a direction, indicating that the connection between nodes is one-way, whereas in undirected graphs, the edges have no direction, indicating a two-way connection.
Outlines
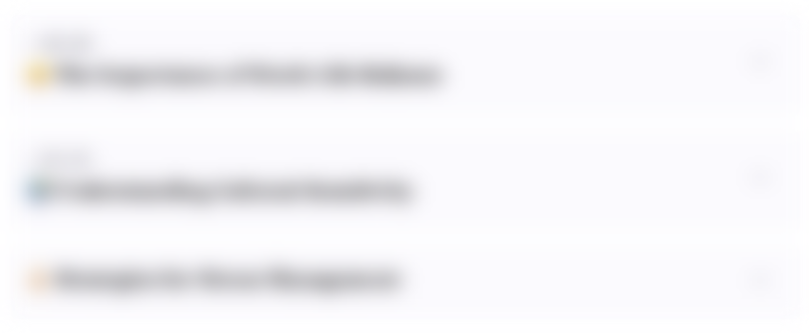
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
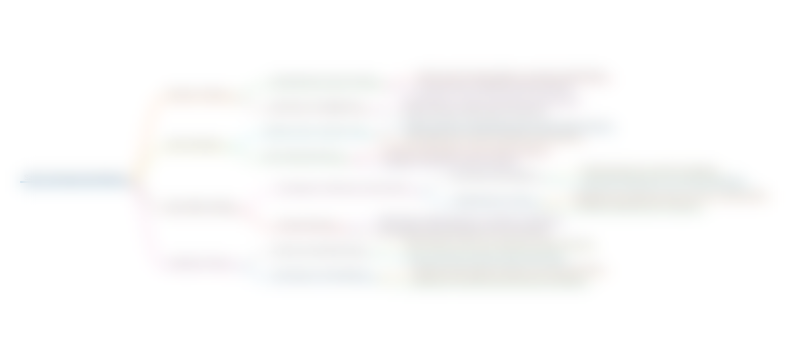
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
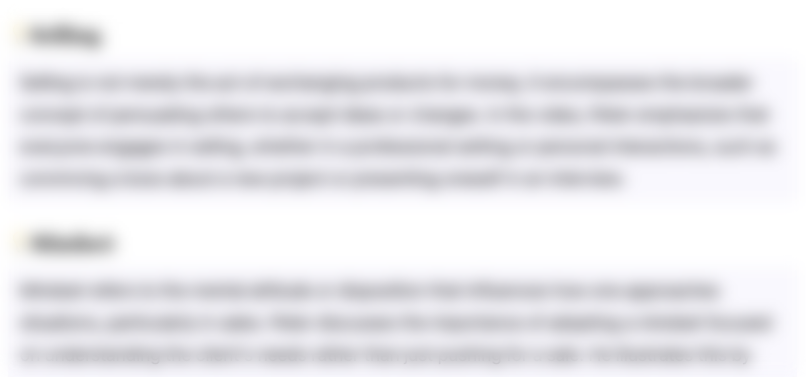
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
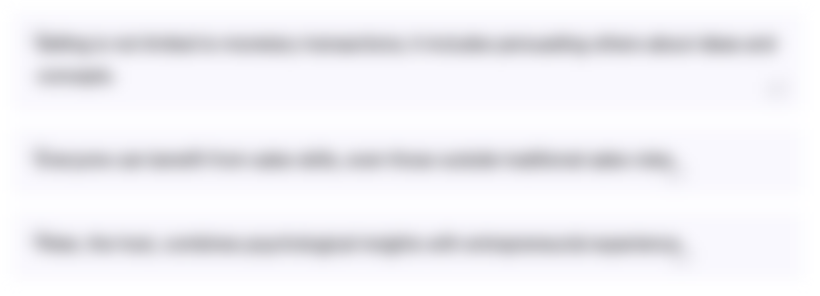
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
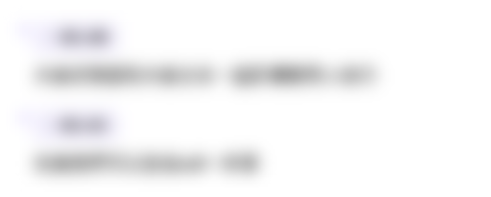
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

8 Data Structures Every Programmer Should Know
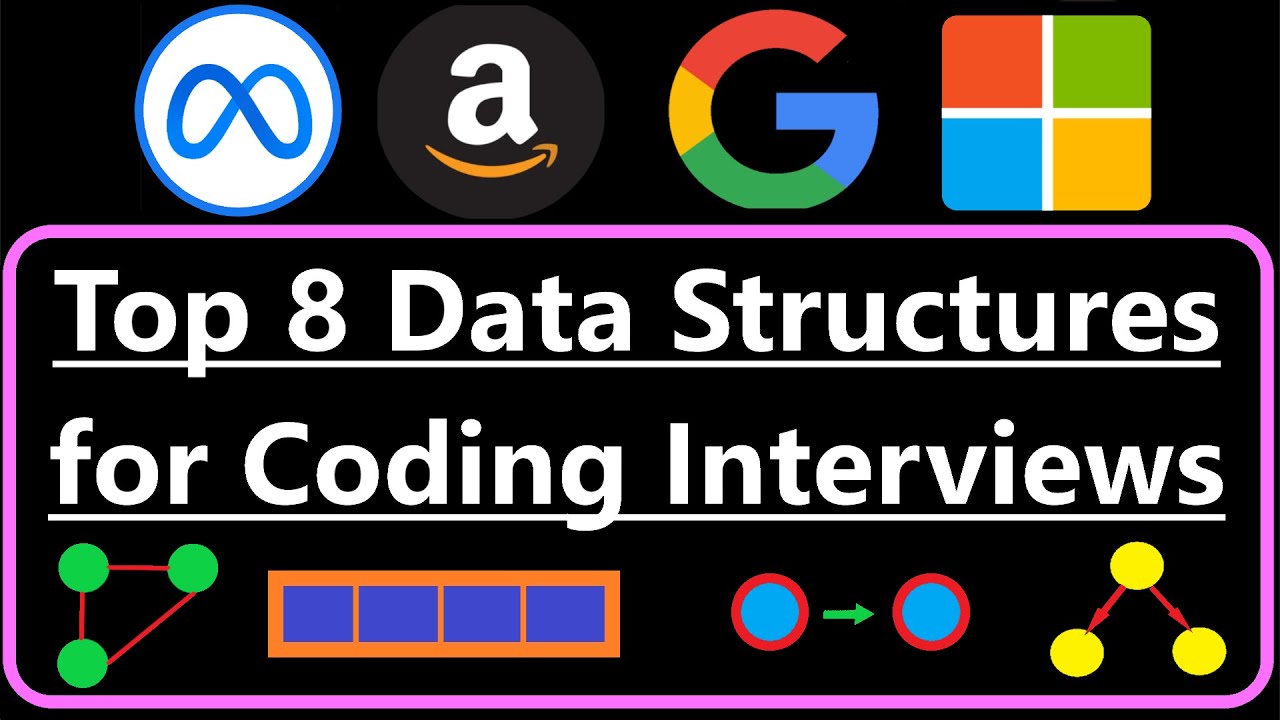
Top 8 Data Structures for Coding Interviews
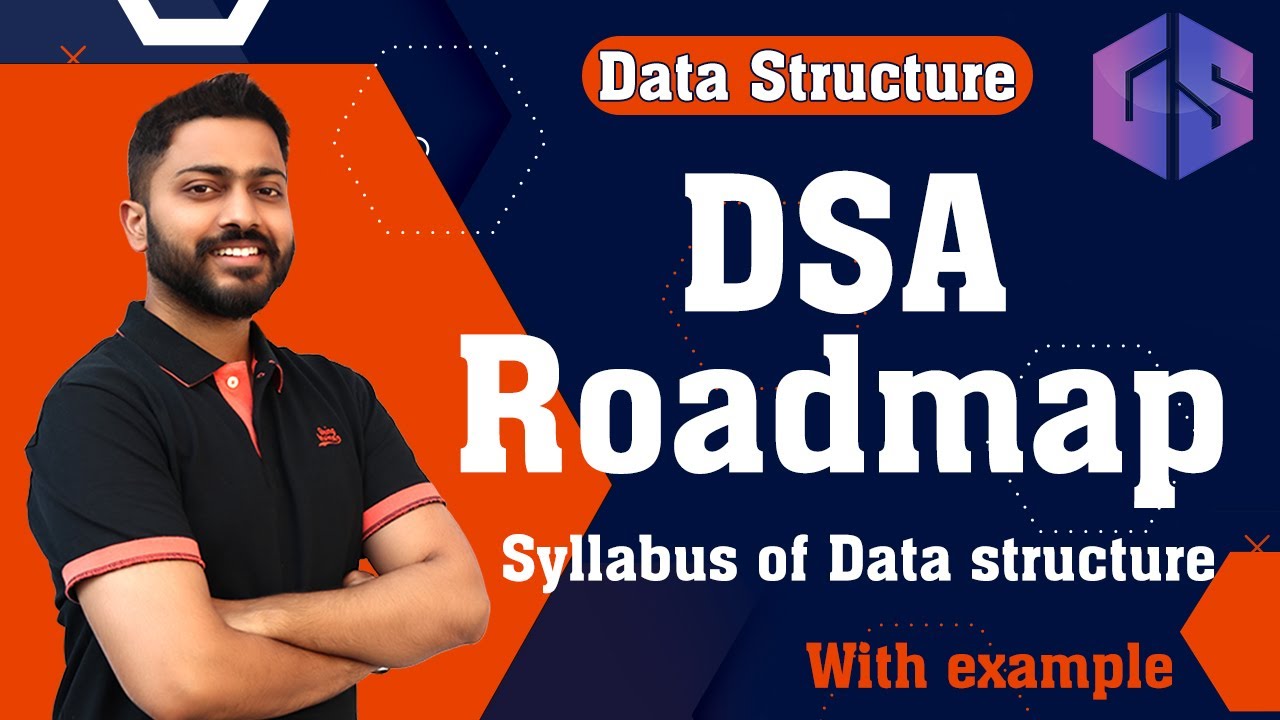
Roadmap ๐ฃ๏ธ of DSA | Syllabus of Data structure | Data Structure for Beginners

10 Key Data Structures We Use Every Day
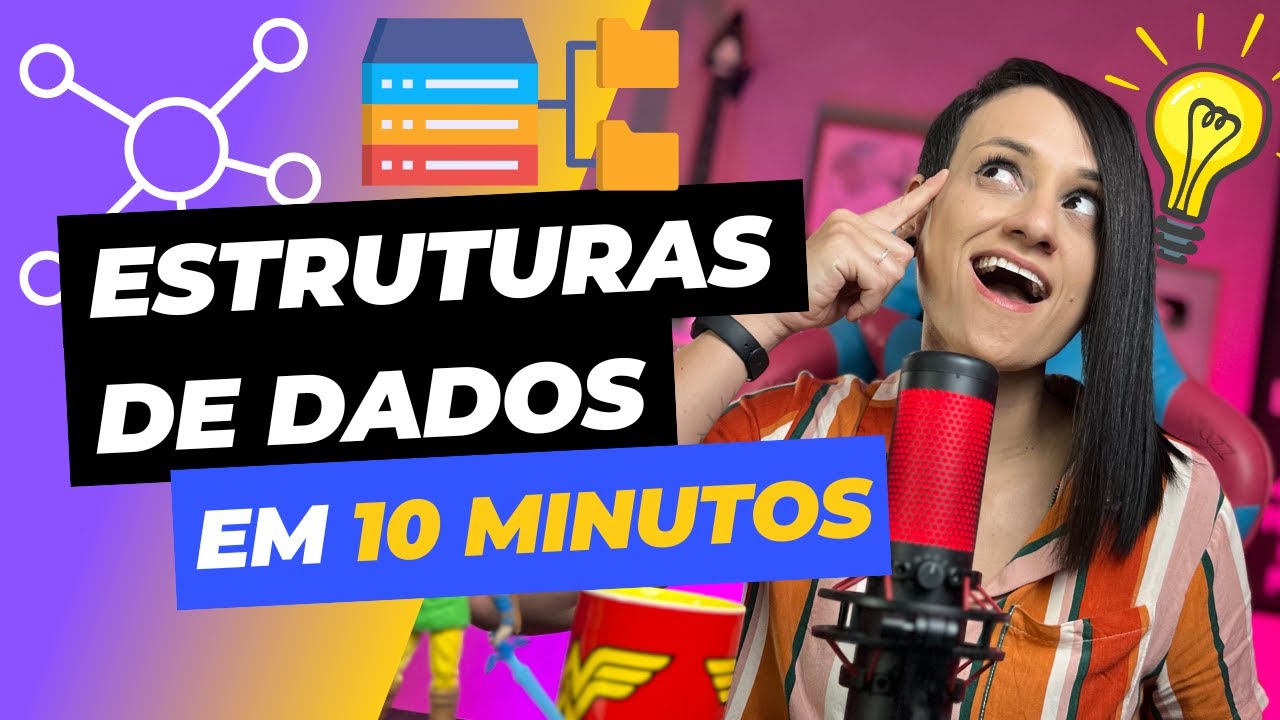
ESTRUTURAS DE DADOS de um jeito fรกcil! (Guia rรกpido para programadores iniciantes)
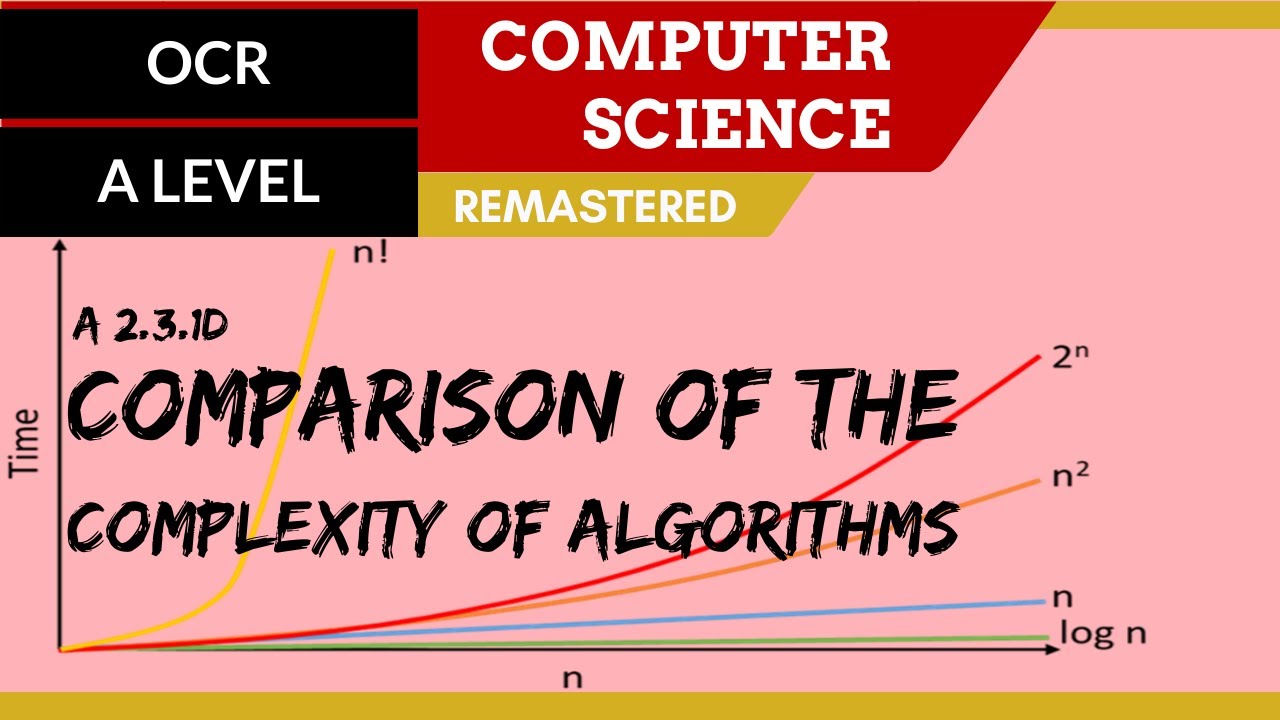
161. OCR A Level (H446) SLR26 - 2.3 Comparison of the complexity of algorithms
5.0 / 5 (0 votes)