Inheritance in Java - Java Inheritance Tutorial - Part 1
Summary
TLDRThe video script explains the concept of inheritance in Java, an object-oriented programming language. It covers how the `extends` keyword is used to implement inheritance, allowing subclasses like `Cat` and `Dog` to inherit properties and methods from a superclass such as `Animal`. The script highlights key benefits of inheritance, like code reusability, and explores access modifiers (public, private, protected) in inheritance scenarios. It also touches on multiple inheritance limitations in Java, type casting, and method overriding, providing simple code examples for better understanding.
Takeaways
- 🟢 Java is an object-oriented programming language, where inheritance is applied using the `extends` keyword.
- 🐱 Inheritance allows classes like `Cat` and `Dog` to inherit properties and methods from a superclass like `Animal`.
- 🔁 A key benefit of inheritance is code reusability, reducing the amount of code needed.
- 🔑 In Java, a superclass (e.g., `Animal`) passes down properties and methods to subclasses (e.g., `Cat`, `Dog`).
- 🔒 Access modifiers (e.g., `private`, `protected`, `public`, and default) control the visibility of variables and methods across classes and packages.
- 🚫 Java does not support multiple inheritance to avoid ambiguity in method selection when two superclasses have the same method.
- 🔼 'Upcasting' allows assigning a subclass object (e.g., `Cat`) to a superclass variable (e.g., `Animal`).
- 🔽 'Downcasting' requires explicit casting, and it must be ensured that the object belongs to the correct type to avoid `ClassCastException`.
- 🔄 Method overriding allows a subclass to modify the implementation of a superclass method while keeping the same method signature.
- ⚠️ The `@Override` annotation should be used to indicate that a method is overriding a superclass method, helping the compiler catch any signature mismatches.
Q & A
What is inheritance in Java?
-Inheritance in Java is a mechanism where one class (the subclass) inherits properties and methods from another class (the superclass). This allows for code reuse and a hierarchical classification.
What keyword is used to implement inheritance in Java?
-The keyword 'extends' is used to implement inheritance in Java. A subclass uses this keyword to inherit from a superclass.
How does inheritance reduce the number of lines of code?
-Inheritance reduces the number of lines of code by allowing subclasses to inherit methods and properties from their superclass, rather than rewriting the same code multiple times.
What is a superclass and a subclass in the context of inheritance?
-A superclass is the parent class from which properties and methods are inherited, while a subclass is the child class that inherits those properties and methods. For example, in the transcript, 'Animal' is the superclass, and 'Cat' and 'Dog' are subclasses.
What are access modifiers in Java, and how do they relate to inheritance?
-Access modifiers in Java, such as public, protected, private, and default, define the visibility of a class's methods and variables. These rules determine whether a subclass can access certain members of its superclass. Public members are accessible to all subclasses, while private members are not.
Why does Java not support multiple inheritance with classes?
-Java does not support multiple inheritance with classes to avoid ambiguity when methods with the same name exist in multiple superclasses. This could lead to confusion as to which method the subclass should inherit.
What is upcasting in the context of Java inheritance?
-Upcasting refers to assigning a subclass object to a superclass reference. This allows the subclass object to be treated as an instance of the superclass, although subclass-specific methods may not be accessible directly.
What is downcasting in Java?
-Downcasting is when a superclass reference is cast back to a subclass reference. It allows access to subclass-specific methods but must be done carefully, as an incorrect cast can lead to a ClassCastException.
What is method overriding in Java?
-Method overriding occurs when a subclass provides a specific implementation of a method already defined in its superclass. The method in the subclass must have the same signature as the one in the superclass.
Why is it important to use the @Override annotation in Java?
-The @Override annotation ensures that the method being overridden matches the method in the superclass. If there are any discrepancies, such as method signature changes, the compiler will generate an error, preventing potential bugs.
Outlines
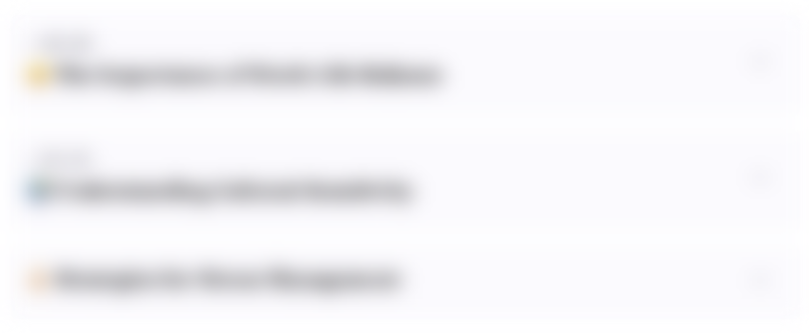
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
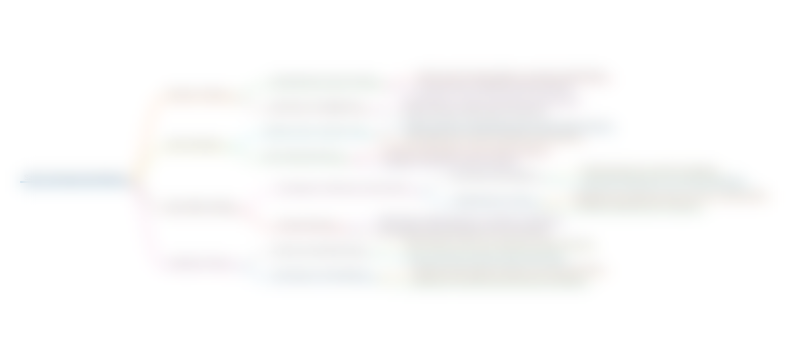
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
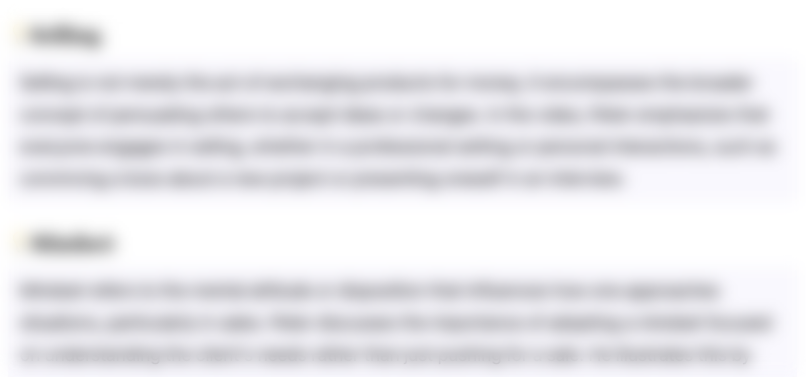
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
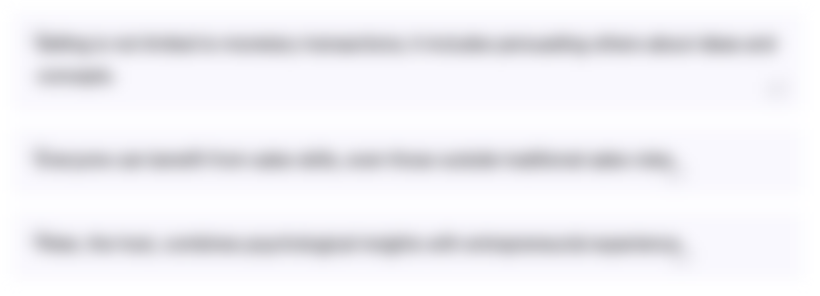
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
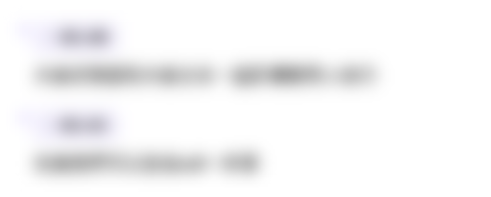
此内容仅限付费用户访问。 请升级后访问。
立即升级浏览更多相关视频
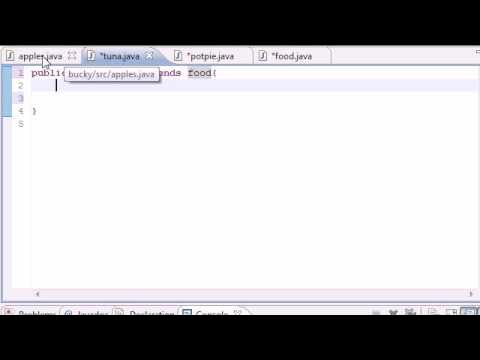
Java Programming Tutorial - 49 - Inheritance
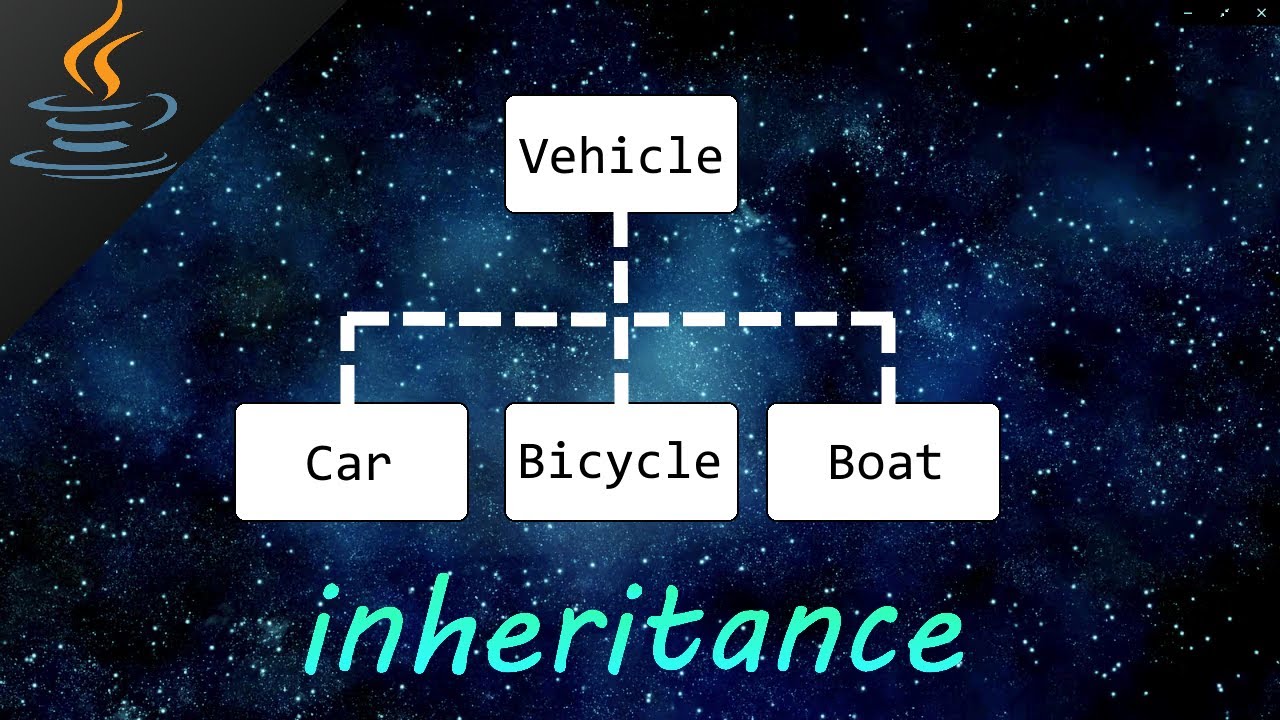
Java inheritance 👪
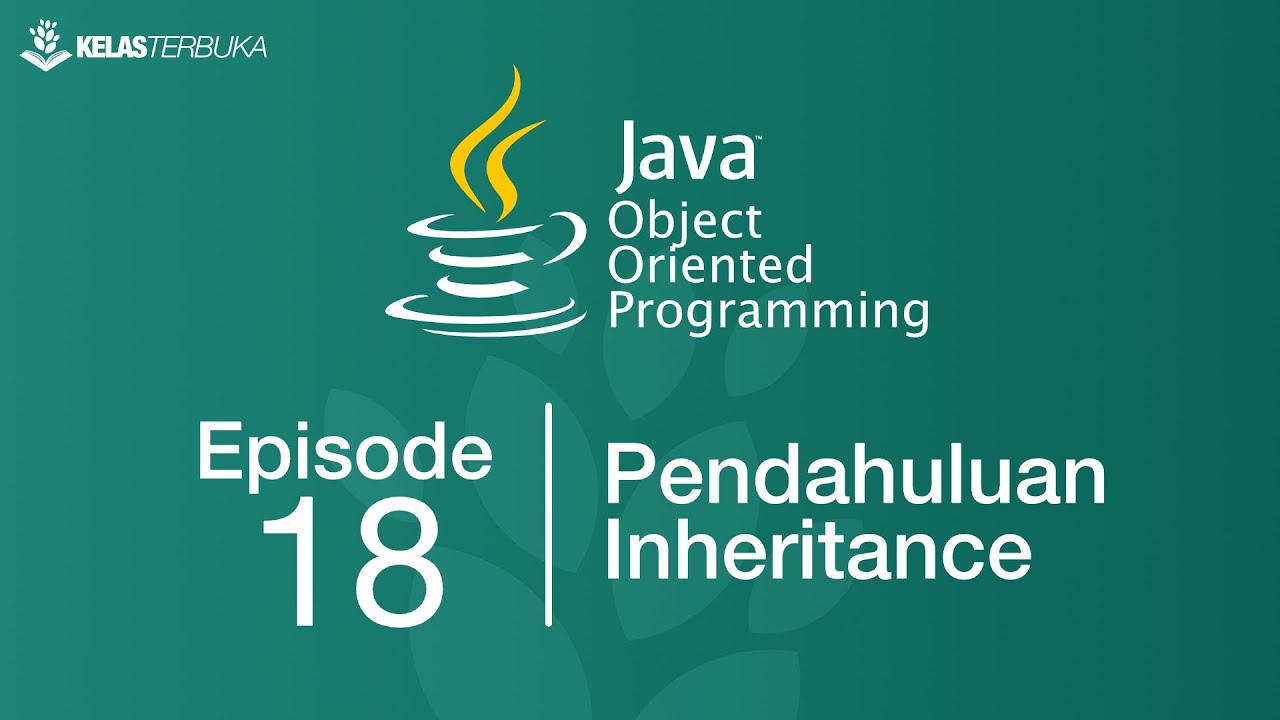
Belajar Java [OOP] - 18 - Pengenalan Inheritance

Java Inheritance | Java Inheritance Program Example | Java Inheritance Tutorial | Simplilearn
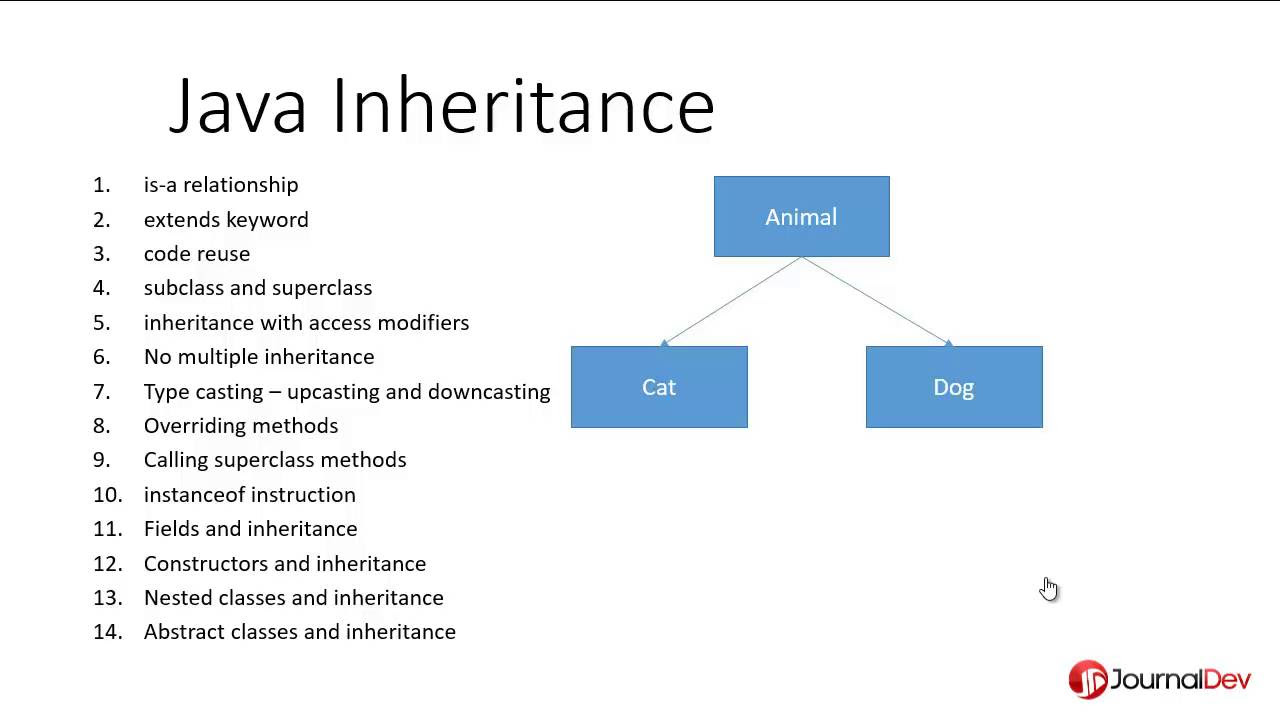
Inheritance in Java - Java Inheritance Tutorial - Part 2
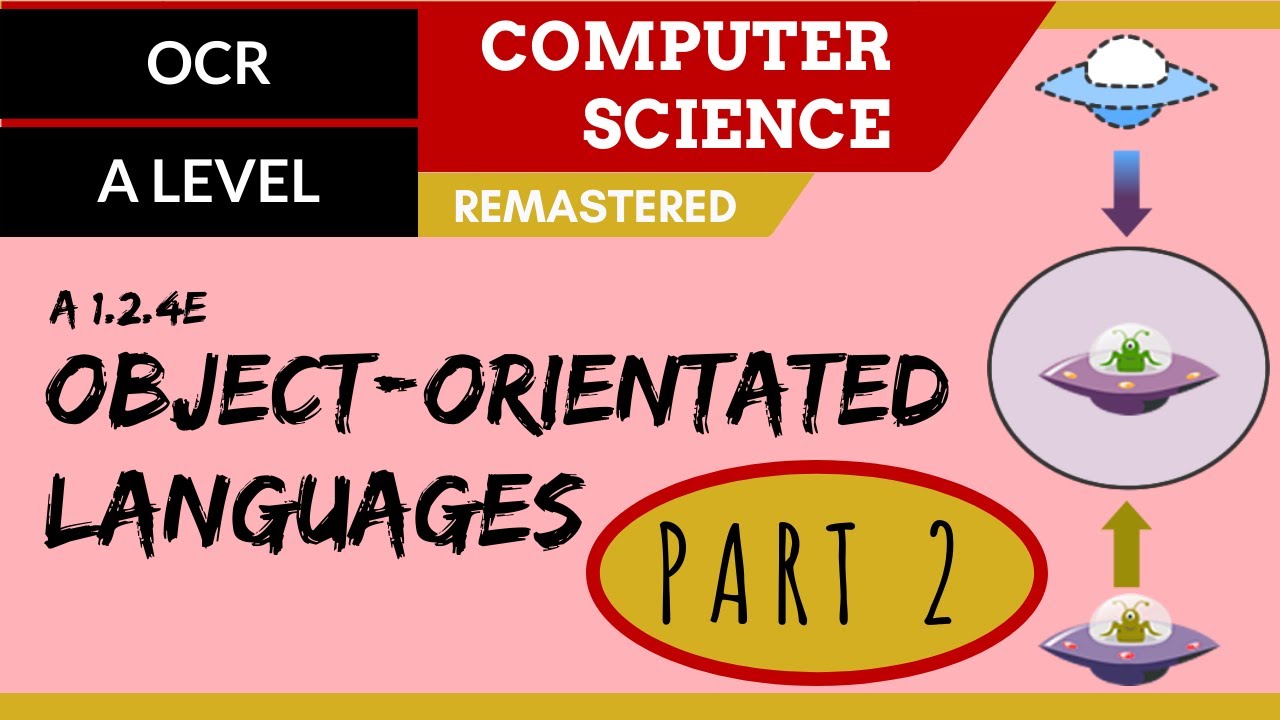
37. OCR A Level (H446) SLR7 - 1.2 Object-oriented languages part 2
5.0 / 5 (0 votes)