01 - [Classes] C++ Intermediate Programming Tutorial
Summary
TLDRThis tutorial provides an introduction to classes in C++ programming. It explains what classes are, how to create them, and their key components such as attributes (like age, name) and methods (like walking or driving). The video walks through creating a simple 'Human' class with attributes and member functions, showing how to declare and define these. It also covers access modifiers (public, private, and protected) and their importance in controlling visibility. Finally, the instructor demonstrates object creation and method usage, helping beginners grasp the fundamentals of object-oriented programming in C++.
Takeaways
- 📚 A class in C++ is essentially a structure used to store data, defined by a header and C++ file.
- 📝 To create a class, you can either add a new item or class in Visual Studio, then name the class and its files.
- 👥 Classes represent real-world or conceptual objects with attributes (like age or name) and methods (like walking or driving).
- 🔧 Methods in a class are functions that define what actions an object can perform, and attributes define its properties.
- ⚙️ Inside a class, there are access modifiers: public, private, and protected, which control access to attributes and methods.
- 🚶 Methods in the class can be created within the header file or defined in a separate CPP file by including the class name with two colons (::).
- 👤 Objects are instances of classes, meaning that a class defines the blueprint, and an object represents an actual instance (e.g., John is an object of the class Human).
- 🛡️ By default, class members are private unless specified otherwise with access modifiers.
- 📝 Methods inside a class are referred to as member functions, and attributes as member variables.
- 💡 Classes are versatile and can represent anything, from real-world objects like humans to conceptual ideas like rocks or mathematical objects.
Q & A
What is a class in C++?
-A class in C++ is a structure that represents data. It serves as a blueprint for objects, allowing you to define attributes (data) and methods (functions) that represent real-world entities or conceptual models.
How do you create a class in C++?
-To create a class in C++, you can either manually add a header file and a C++ file with the same name or use Visual Studio to create a class through the 'Add Class' option. The class declaration starts with the 'class' keyword followed by the class name.
What are the attributes and methods of a class?
-Attributes are the data or properties that define a class, such as age or name. Methods (or member functions) are the actions that objects of the class can perform, such as 'walk' or 'drive'.
What is the difference between functions and methods in C++?
-In C++, functions that are declared outside of a class are called functions, whereas functions declared inside a class are called methods or member functions.
What is the purpose of access modifiers in a class?
-Access modifiers in a class (public, private, and protected) determine the visibility and accessibility of the class members. Public members can be accessed by any code, private members can only be accessed within the class itself, and protected members can be accessed by the class and its derived classes.
How do you access the attributes or methods of a class object?
-You can access the attributes or methods of a class object using the dot operator (.). For example, if you have an object 'John' of the class 'Human', you can access the age attribute using 'John.age' and call a method like 'John.walk()'.
What is the difference between a class and an object in C++?
-A class is a blueprint or template that defines the structure and behavior of objects, while an object is an instance of a class. For example, 'Human' is a class, and 'John' is an object created from that class.
What are the default access levels for class members in C++?
-By default, the members of a class in C++ are private. If no access modifier is specified, the members are treated as private, meaning they are only accessible from within the class.
How do you separate class declarations and definitions?
-Class declarations (including the definition of member functions) are typically placed in a header file (.h), while the implementation or definition of member functions is done in a corresponding .cpp file. This separation improves code organization and maintainability.
What are the key things to remember when working with classes in C++?
-Key points include understanding attributes and methods, using proper access modifiers (public, private, protected), recognizing the difference between classes and objects, and knowing how to initialize and use class objects.
Outlines
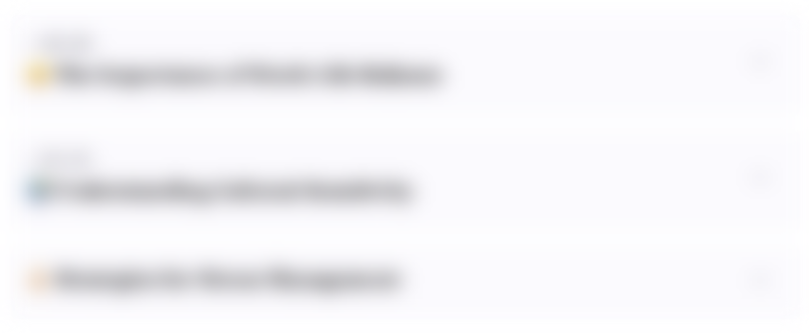
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
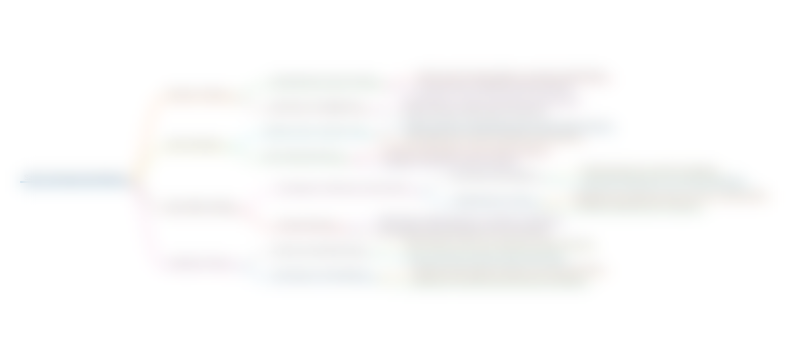
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
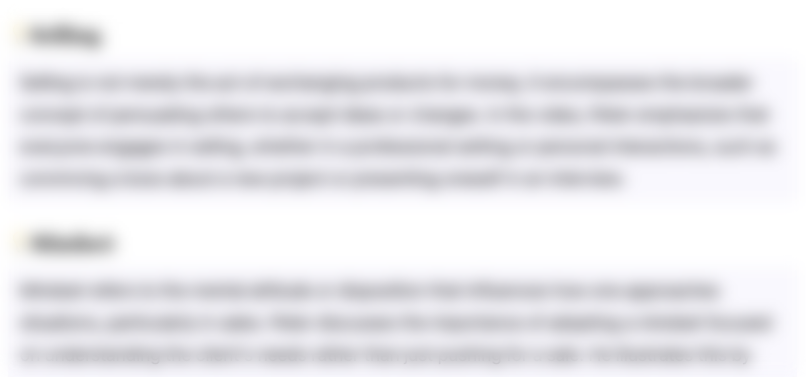
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
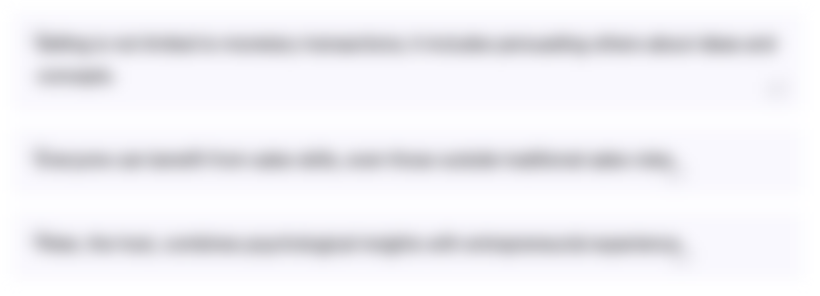
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
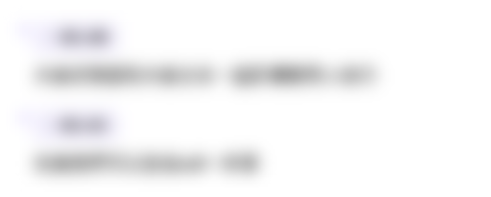
此内容仅限付费用户访问。 请升级后访问。
立即升级浏览更多相关视频
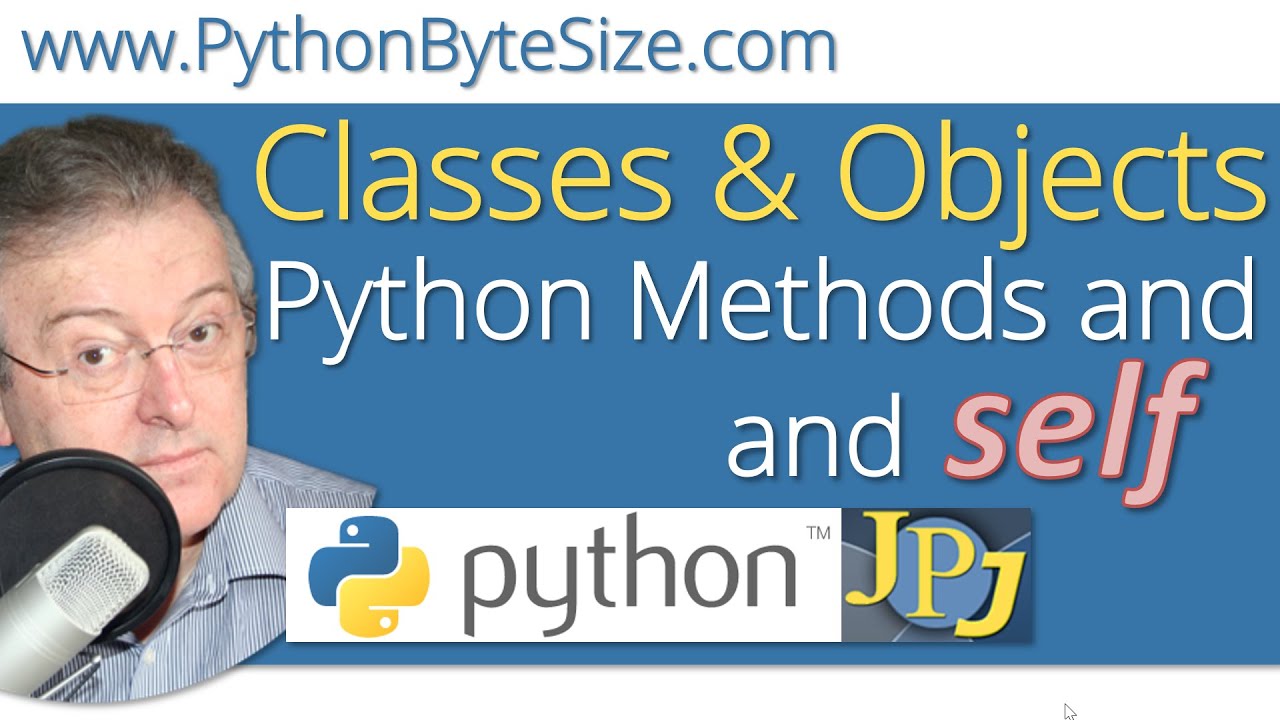
Python Methods and self

Classes and Objects in Python | OOP in Python | Python for Beginners #lec85
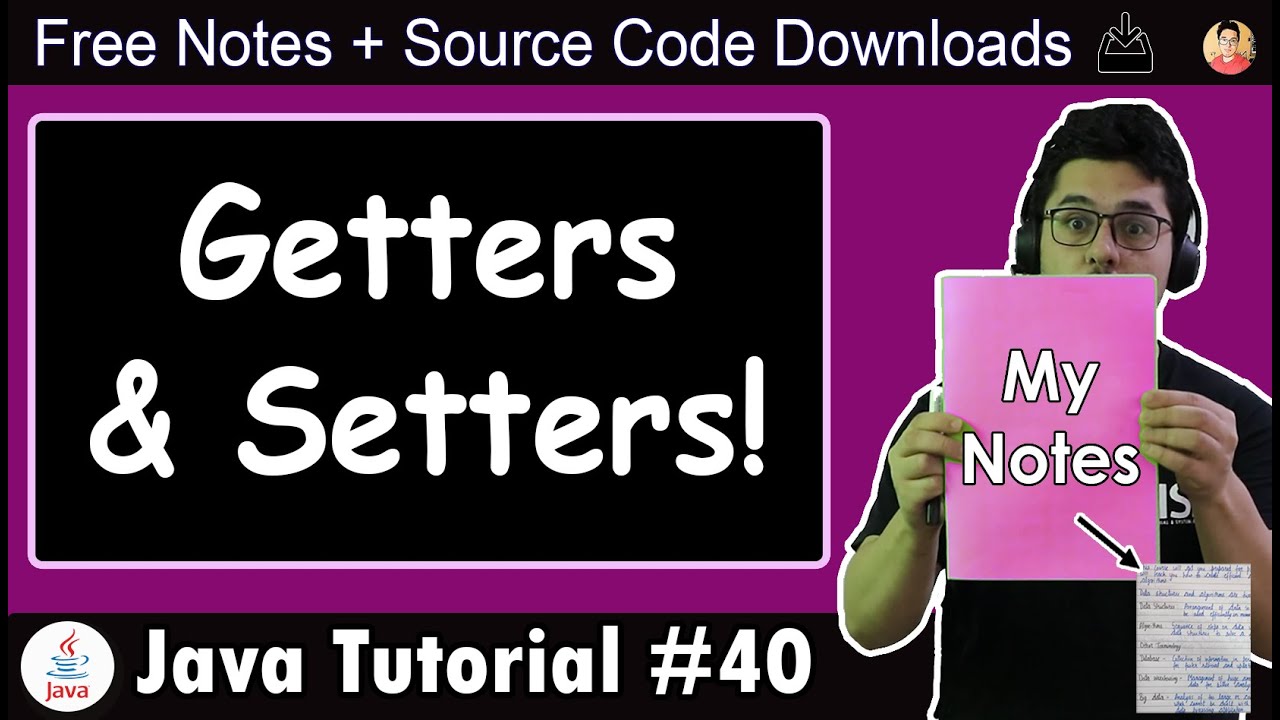
Java Tutorial: Access modifiers, getters & setters in Java
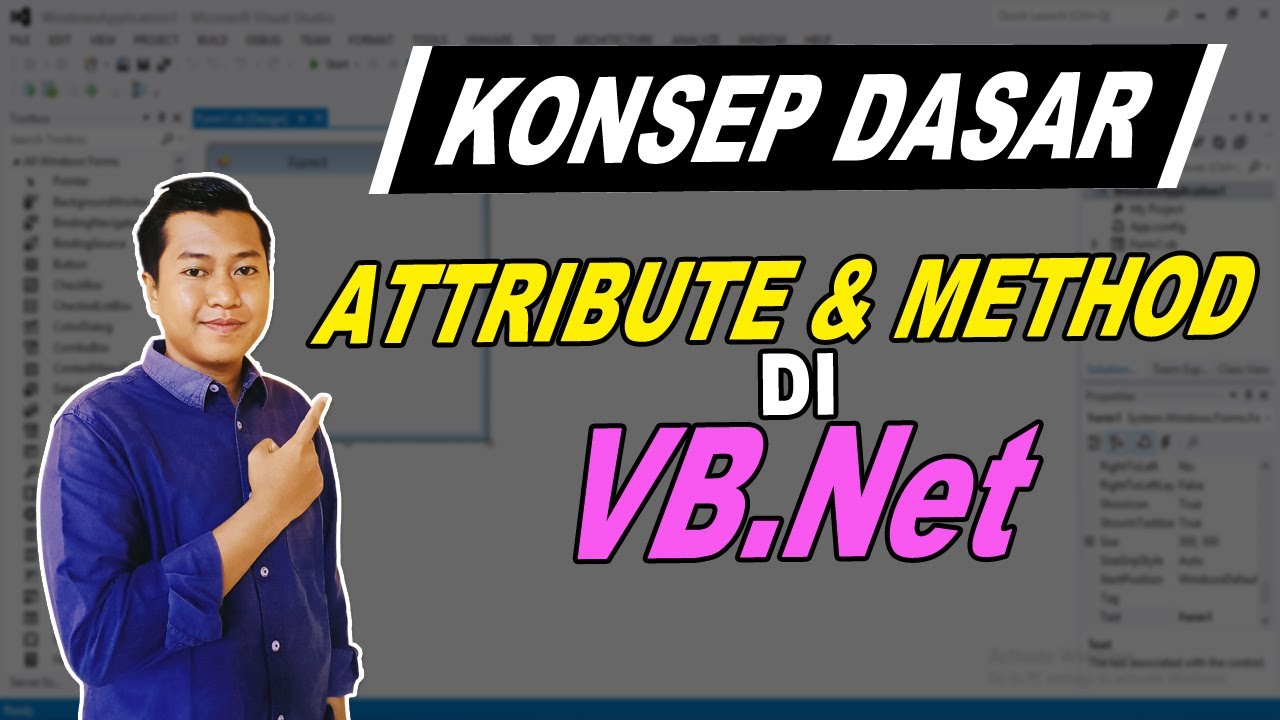
Memahami Konsep OOP di VB.Net: Penjelasan dan Contoh Attribute / Property & Method / Behavior
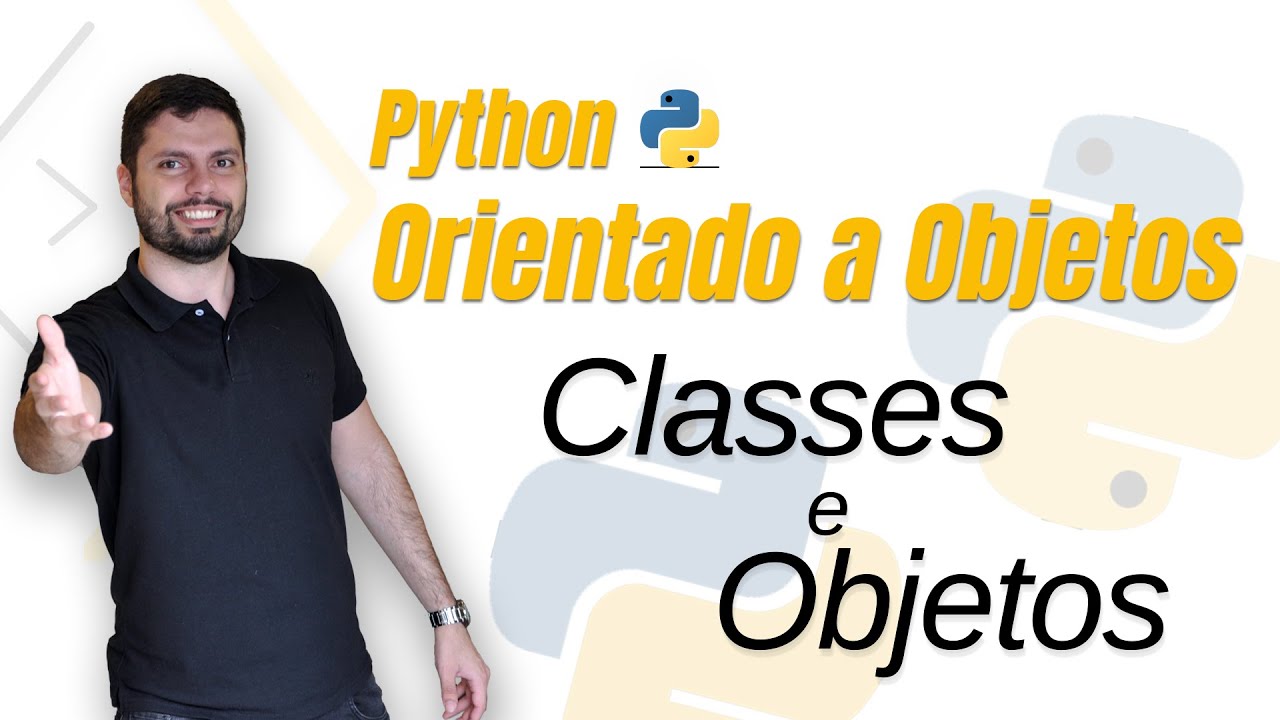
Como criar Classes e Objetos - Curso Python Orientado a Objetos [Passo a Passo]
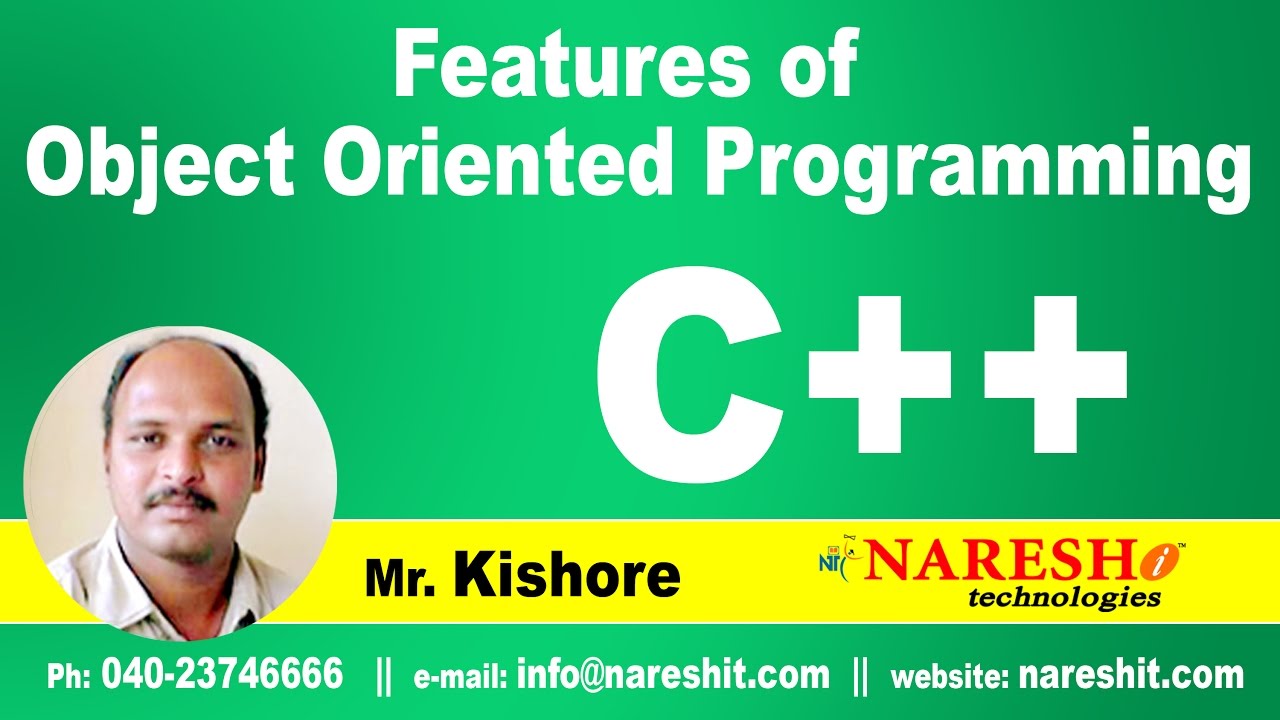
Object Oriented Programming Features Part 3 | C ++ Tutorial | Mr. Kishore
5.0 / 5 (0 votes)