#07 What is a Component | Angular Components & Directives| A Complete Angular Course
Summary
TLDRThis lecture provides an introduction to components in Angular, a key feature of the framework for building client-side applications. It explains that an Angular application is built by combining multiple independent components, starting with a root component (often called the app component). The lecture also covers the parent-child relationship between components and demonstrates how to create a component in Angular. Key steps include creating a TypeScript class, decorating it with the @Component decorator, and declaring it in the module file. A simple example of building a header component is shown.
Takeaways
- 📦 Angular is a component-based JavaScript framework used for building client-side applications.
- 🌳 Every Angular application has at least one root component, conventionally named 'App Component', which can contain child components.
- 🧩 A component is a small, reusable piece of a user interface, and Angular applications are built by combining multiple components.
- 📐 Components can be organized independently, such as a header, sidebar, footer, or content area, and then combined to form the overall application interface.
- 🔗 Components can also have nested child components, forming a parent-child relationship, like a navbar component inside a header component.
- 🏗️ To create a component in Angular, follow three steps: create a TypeScript class, decorate it with the @Component decorator, and declare it in the main module file.
- 📑 The @Component decorator is used to define a component, including its selector and template, which specify how the component will render in the DOM.
- 🗂️ Angular components must be registered in the 'declarations' array of the Angular module to be recognized and used by the framework.
- 🔀 Components can be inserted into the root component or other components using their selector (e.g., 'app-header' for a header component).
- 🛠️ The template for a component can be directly defined using the 'template' property or by specifying an external HTML file using 'templateUrl'.
Q & A
What is a component in Angular?
-A component in Angular is a small, reusable piece of a user interface. Components are the building blocks of Angular applications, and each Angular app has at least one component called the root component.
What is the purpose of the root component in an Angular application?
-The root component, often referred to as the 'app component', represents the entire Angular application. It is the parent component that contains all other components and forms the structure of the app.
How can different parts of the user interface be structured in Angular?
-In Angular, the user interface is split into separate components. For example, a web application could have components for the header, navbar, sidebar, main content, and footer. Each of these can be built independently and combined to create the full user interface.
What is the convention for naming a root component in Angular?
-By convention, the root component of an Angular application is named 'app component', although it can be named anything.
How do components form a parent-child relationship in Angular?
-In Angular, components can have a parent-child relationship where the root component (e.g., 'app component') contains child components like the header, navbar, and footer. Similarly, each child component can have its own child components.
What are the three main steps to creating and using a component in Angular?
-The three steps to create and use a component in Angular are: 1) Create a TypeScript class and export it, 2) Decorate the class with the '@Component' decorator, and 3) Declare the component in the main module file.
What is the purpose of the '@Component' decorator in Angular?
-The '@Component' decorator in Angular is used to define a class as a component. It provides metadata, such as the selector and template, that Angular uses to understand how the component should be rendered.
What is a selector in Angular, and how is it used?
-A selector in Angular is a property defined in the '@Component' decorator. It represents the custom HTML tag that will be used to display the component in the template. For example, the selector 'app-header' can be used in HTML as '<app-header></app-header>'.
How can the template of a component be defined?
-The template of a component in Angular can be defined using either the 'template' property (inline HTML within the component) or the 'templateUrl' property (linking to an external HTML file).
How does Angular become aware of a new component?
-Angular becomes aware of a new component when it is declared in the 'declarations' array of the module (typically 'AppModule'). The component also needs to be imported in the module file.
Outlines
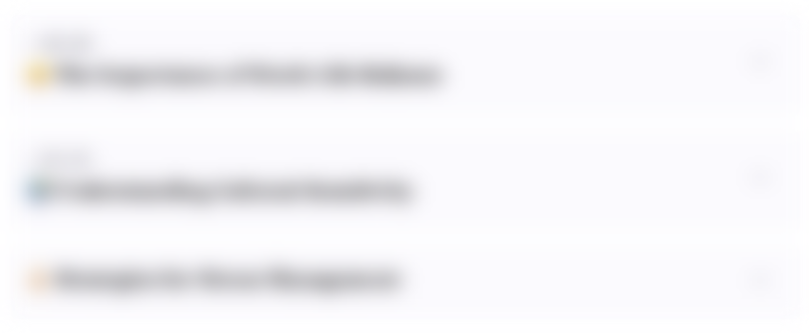
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
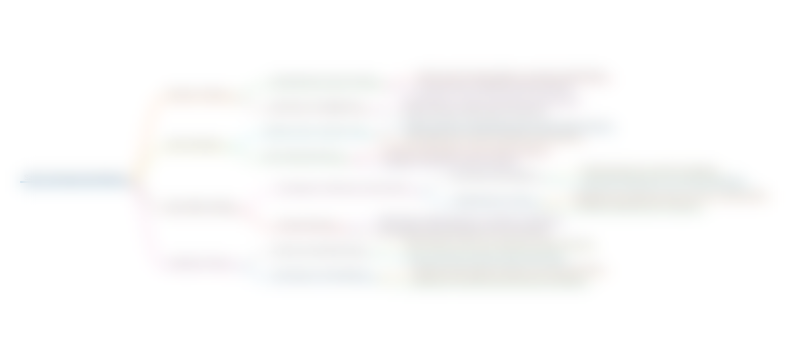
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
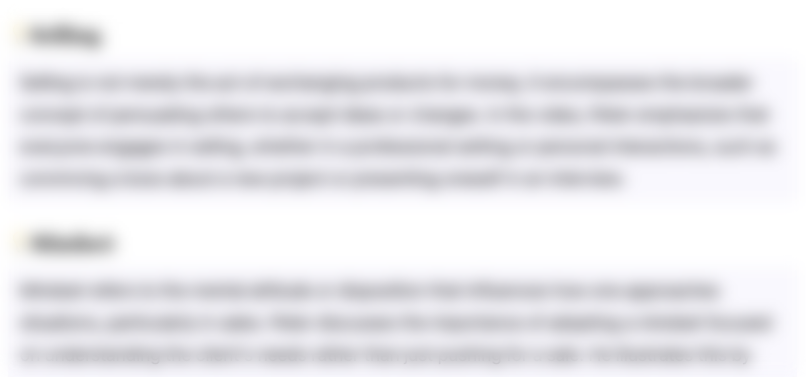
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
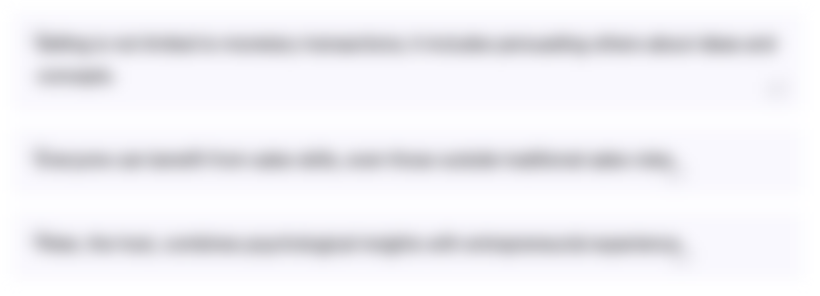
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
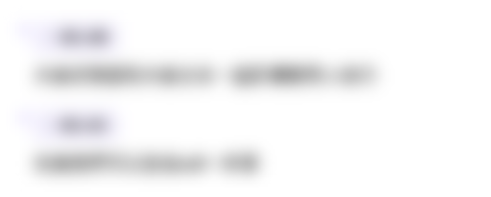
此内容仅限付费用户访问。 请升级后访问。
立即升级浏览更多相关视频
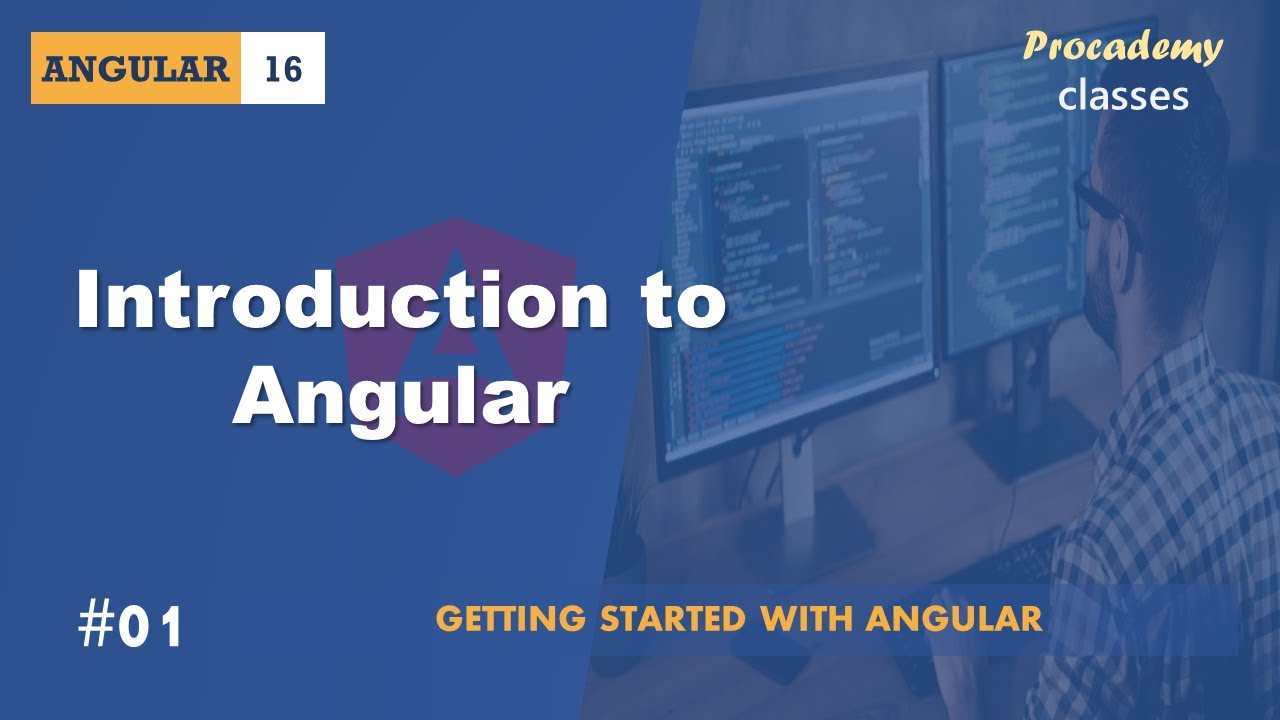
#01 Introduction to Angular | Getting Started with Angular | A Complete Angular Course
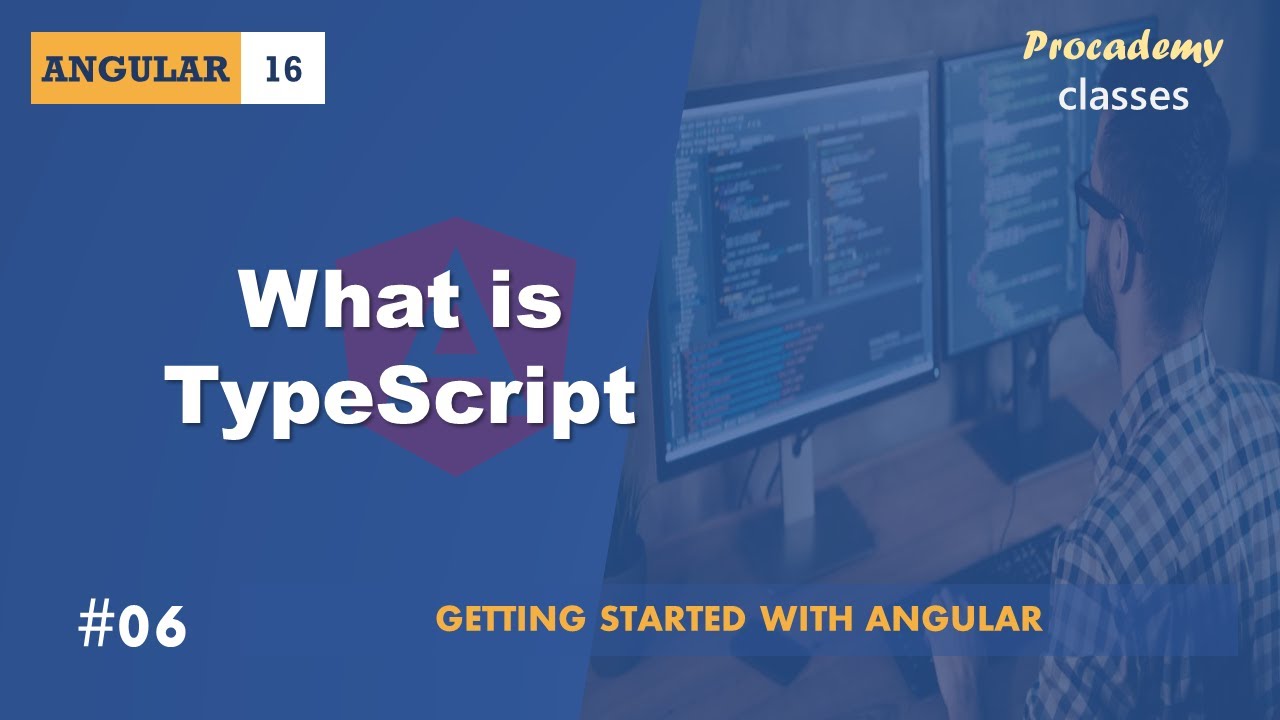
#06 What is TypeScript | Getting Started with Angular | A Complete Angular Course

Deferrable Views - New Feature in Angular 17
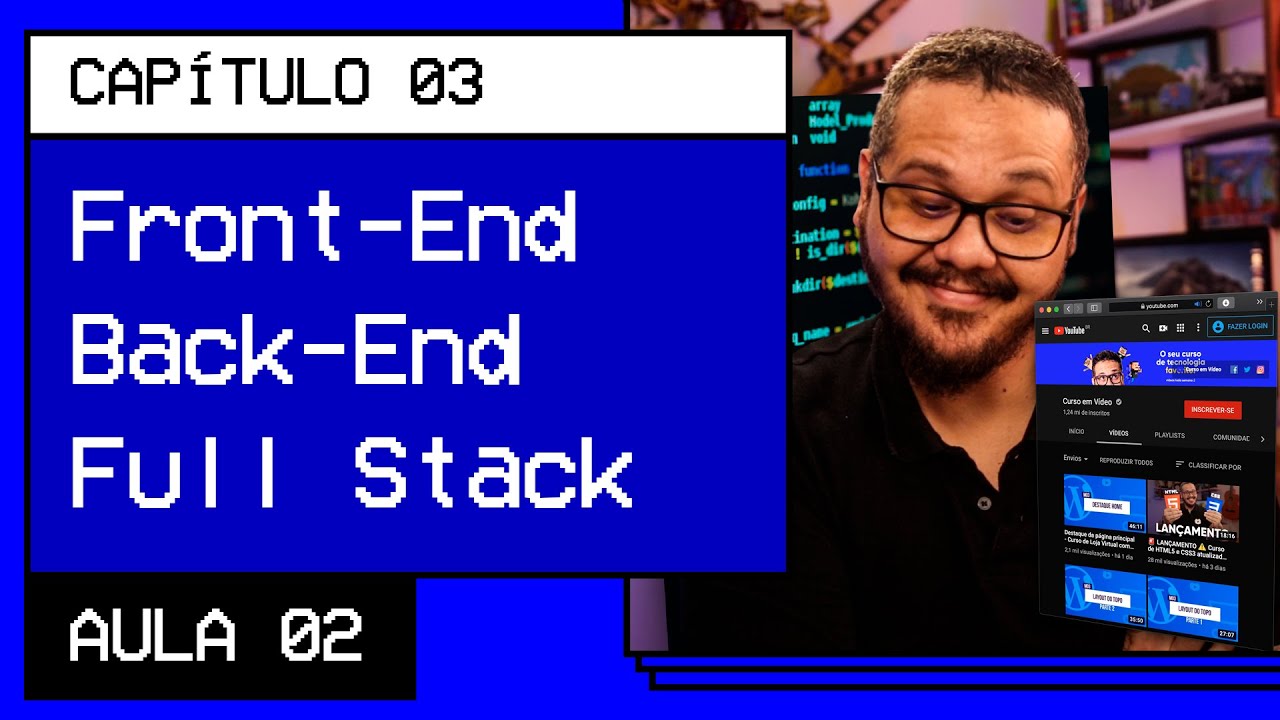
Front-end, Back-end e Full stack - @Curso em Vídeo HTML5 e CSS3
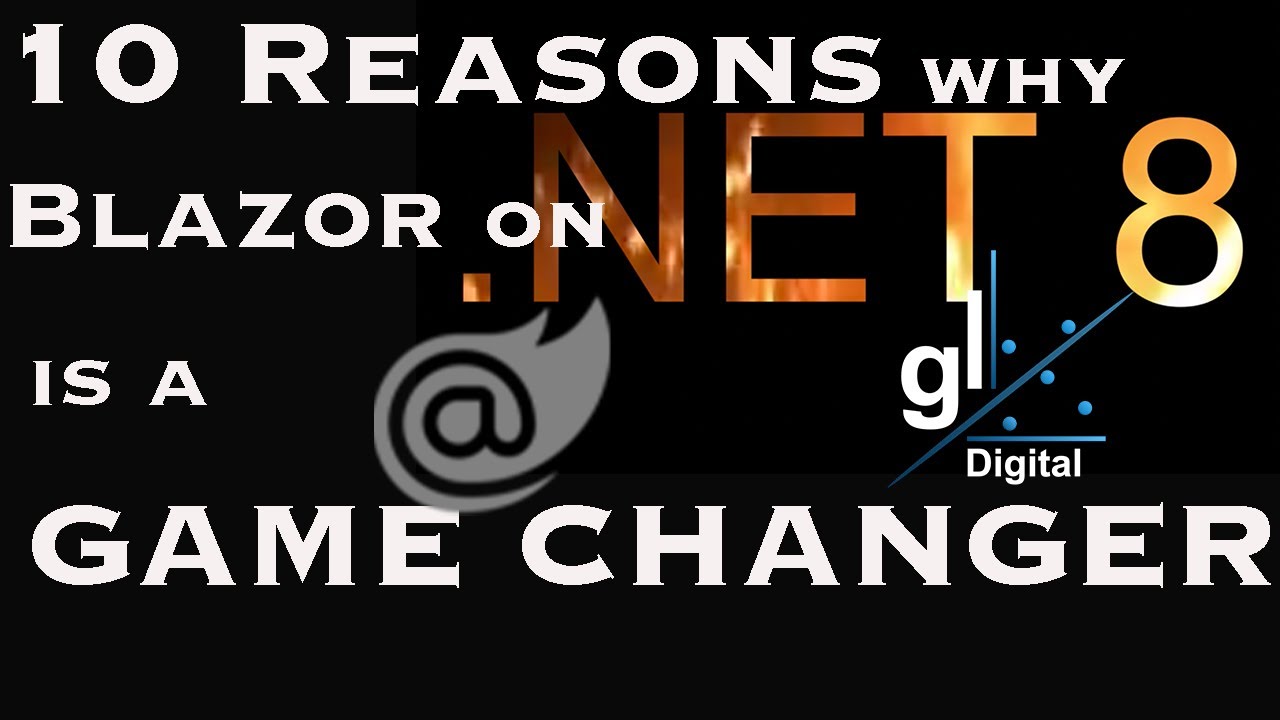
Blazor on .NET 8 - Ten Reasons why Blazor on .NET 8 is a Game Changer
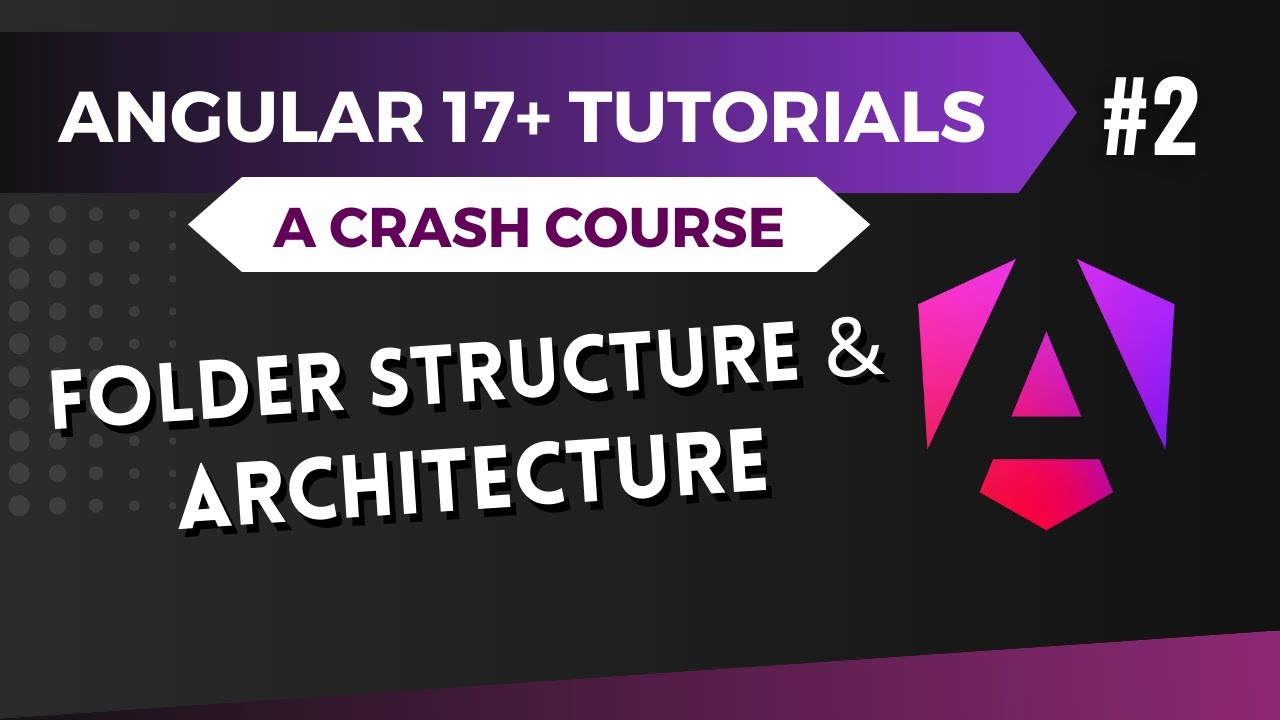
Angular 17 Tutorial - Folder Structure and Architecture #2
5.0 / 5 (0 votes)