Curso Java Intermedio #8 | Encapsulamiento en Java (POO)
Summary
TLDRIn this video, the instructor introduces the concept of encapsulation in Java, explaining its role in controlling access to class data and preventing unintended modifications. Through a practical exercise, learners create a class to simulate the logical operations of a washing machine. The class must receive inputs like weight and clothing type, perform actions such as water filling, washing, and drying, and ensure proper encapsulation of methods and attributes. The lesson emphasizes the importance of hiding unnecessary details, exposing only essential methods like `cicloFinalizado`, and applying access modifiers for data security.
Takeaways
- 😀 Encapsulation in Java controls access to the data within an object, specifying which methods and attributes are public and which are private.
- 😀 The purpose of encapsulation is to protect the internal state and behavior of a class from being modified in unexpected ways by other classes.
- 😀 In Java, access modifiers (public, default, protected, and private) are used to implement encapsulation and manage data visibility and security.
- 😀 The `Scanner` class is an example of encapsulation in Java, where users can interact with it without accessing or modifying its internal attributes or methods.
- 😀 Encapsulation in Java ensures that only the necessary methods and attributes are accessible to external users, enhancing security and reliability.
- 😀 The exercise in the video involves creating a class for the logical functions of a washing machine, which should be encapsulated and interact with data through public methods only.
- 😀 The class created in the exercise must receive the weight of clothes and the type of clothing as arguments through a constructor.
- 😀 Three essential methods need to be implemented for the washing machine class: water filling, washing, and drying, which correspond to the machine's operational functions.
- 😀 The class should be encapsulated correctly, restricting access to unnecessary variables and methods to ensure they cannot be modified externally.
- 😀 The only method that should be accessible for external use is `cycleComplete`, allowing other developers to interact with it while keeping the internal workings secure.
Q & A
What is encapsulation in Java?
-Encapsulation in Java refers to the concept of controlling access to the data within an object or class, specifically by defining which methods and attributes are public (accessible) and which are private (hidden from external access). This helps prevent unauthorized modification of the object's state.
Why is encapsulation important in Java programming?
-Encapsulation is important because it protects the internal state of an object, ensuring that external code cannot modify it unexpectedly. This leads to more maintainable and reliable code, reducing errors and unintended consequences.
What are the four access modifiers in Java?
-The four access modifiers in Java are `public`, `private`, `protected`, and `default` (no modifier). `public` allows full access, `private` restricts access to within the same class, `protected` allows access within the same package or subclasses, and `default` restricts access to within the same package.
How do you use the `this` keyword in Java?
-In Java, the `this` keyword refers to the current instance of the class. It is commonly used to distinguish between instance variables and method parameters when they have the same name. For example, `this.variable = variable;` assigns the method parameter to the instance variable.
What is the purpose of the constructor in the washing machine class?
-The constructor in the washing machine class is used to initialize the class with values for the weight of the laundry and the type of clothing. These values are received as arguments and assigned to private instance variables within the class.
What is the significance of private variables in the washing machine class?
-Private variables in the washing machine class ensure that certain data, such as the weight of the laundry or the washing status, cannot be accessed or modified directly by other classes. This is a key part of encapsulation, which protects the integrity of the class's internal state.
What methods are expected to be implemented in the washing machine class?
-The washing machine class should include methods for the following operations: filling water, washing clothes, and drying clothes. Additionally, the class should have a public method called `cicloFinalizado` (cycle completed), which can be accessed by external code to check if the washing cycle is complete.
How does the class handle the type of clothing in the washing machine example?
-The class handles the type of clothing by using an integer variable `tipoRopa`, which stores the value representing the type of clothing. The value is set to 1 for white clothes, 2 for colored clothes, and 0 when the type is not determined.
Why is encapsulation applied to variables like `kilos`, `llenadoCompleto`, and `tipoRopa`?
-Encapsulation is applied to these variables to restrict external access and modification. By making them private, the class ensures that these critical variables cannot be directly altered by other classes, which helps maintain control over the washing machine's operations and logic.
How does the `cicloFinalizado` method fit into the encapsulation design of the washing machine class?
-The `cicloFinalizado` method is the only public method in the class that provides external access. It is used to inform whether the washing cycle is complete, while all other internal methods and variables are kept private to protect the class's data integrity and avoid misuse.
Outlines
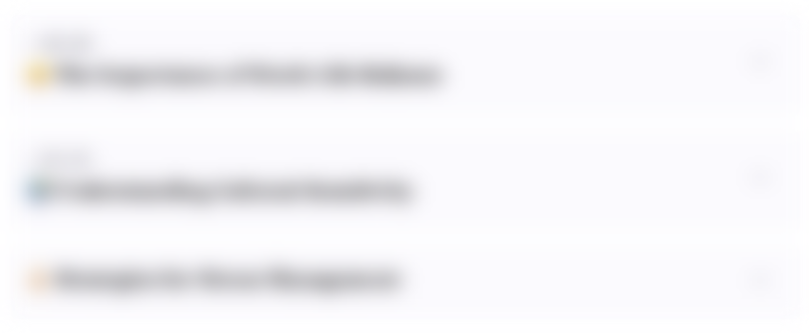
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
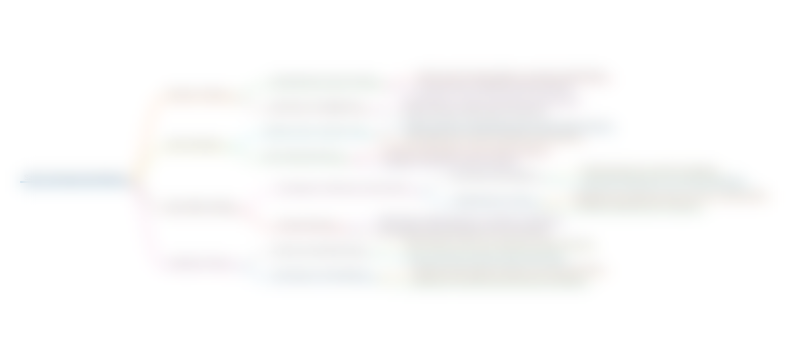
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
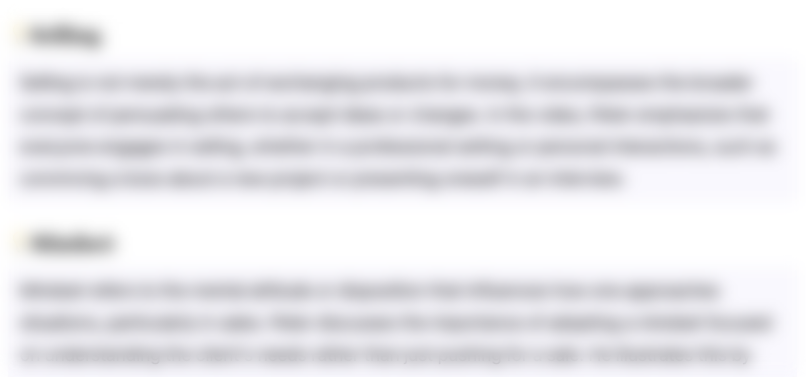
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
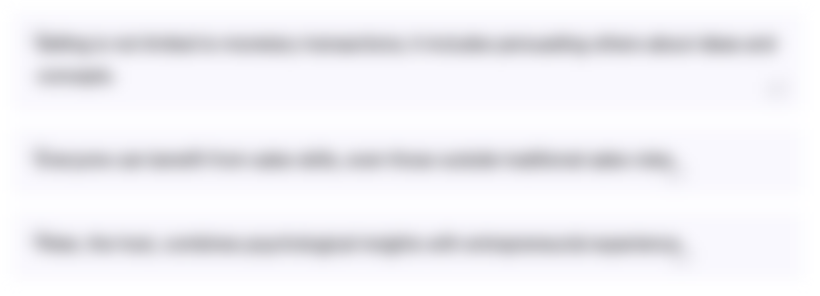
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
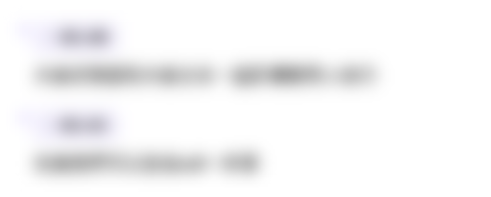
此内容仅限付费用户访问。 请升级后访问。
立即升级5.0 / 5 (0 votes)