40 - Orientação Objetos - Introdução classes pt 02
Summary
TLDRIn this Java tutorial, the instructor introduces object-oriented programming concepts by explaining how to create and work with classes and objects. The focus is on the `Student` class, which includes attributes like `name`, `age`, and `gender`. The lesson covers key concepts such as creating objects, handling default values, and managing object references. Emphasis is placed on the importance of initializing object attributes correctly to avoid unintended side effects when working with multiple instances. The video concludes with practical advice on how to handle shared default values and object reference variables safely in Java.
Takeaways
- 😀 Always use uppercase for package names in Java to maintain proper organization.
- 😀 A class in Java represents a blueprint for objects and can have attributes that define the properties of those objects.
- 😀 Objects created from a class in Java automatically inherit the attributes defined in the class.
- 😀 When a class attribute is not initialized, it will have a default value depending on its data type.
- 😀 Java variables referencing objects can be created without using the `new` keyword, but they will not have allocated memory until linked to an object.
- 😀 If you don’t assign a value to class attributes, Java will initialize them with default values like 0 for integers and null for objects.
- 😀 When creating multiple objects of the same class, each object has its own space in memory unless explicitly referenced to share data.
- 😀 Be cautious when initializing class-level variables, as changes to one object can affect others if they share the same reference.
- 😀 Avoid initializing shared variables with the same default values across all instances unless that’s the desired behavior.
- 😀 Pay attention to which reference variable you're working with to avoid unintended modifications to shared data.
Q & A
What is the primary topic discussed in this video tutorial?
-The primary topic is an introduction to object-oriented programming in Java, focusing on creating classes, objects, and understanding class attributes and object references.
What are the three attributes defined in the 'Estudante' class?
-The three attributes defined in the 'Estudante' class are 'nome' (name), 'idade' (age), and 'sexo' (gender).
What is the significance of the 'new' keyword in Java when creating objects?
-The 'new' keyword is used to allocate memory for a new object and create an instance of a class. It is essential for initializing objects and enabling access to their attributes.
What happens if an object is created but no reference variable is assigned?
-If an object is created without assigning a reference variable, memory space for the object is allocated, but it cannot be accessed since there is no reference to the object in memory.
What are default values assigned to class attributes in Java if they are not explicitly initialized?
-In Java, the default values for class attributes are 'null' for reference types (e.g., String) and '0' for primitive types (e.g., int).
Why is it important to initialize class-level variables properly?
-Proper initialization of class-level variables is crucial to avoid unintended sharing of values between different objects. Without proper initialization, multiple objects might end up having the same values, leading to unexpected behavior.
What could go wrong when all objects of a class share the same default value for an attribute?
-When all objects of a class share the same default value for an attribute, modifying this attribute in one object could unintentionally affect all objects, as they refer to the same value in memory.
What is the difference between a class variable and an object instance variable in terms of initialization?
-A class variable is shared by all instances of a class, and initializing it directly within the class will assign the same value to all objects. An object instance variable is unique to each object, and its value can be initialized individually.
How does using the 'new' keyword affect object memory and access to attributes?
-Using the 'new' keyword creates a new object and allocates memory for it. It allows access to the object's attributes, but until a reference variable is assigned, the object cannot be accessed or manipulated.
What caution should a programmer take when working with object references in Java?
-A programmer should be cautious not to mix up object references. If the same reference variable is reused or not properly differentiated, it can lead to unexpected behavior, as multiple objects might incorrectly point to the same memory space.
Outlines
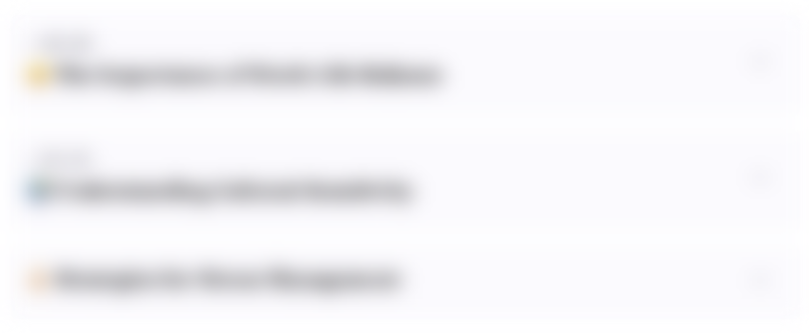
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
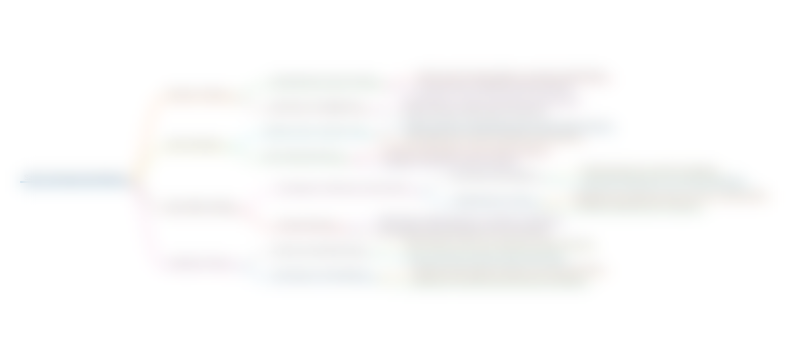
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
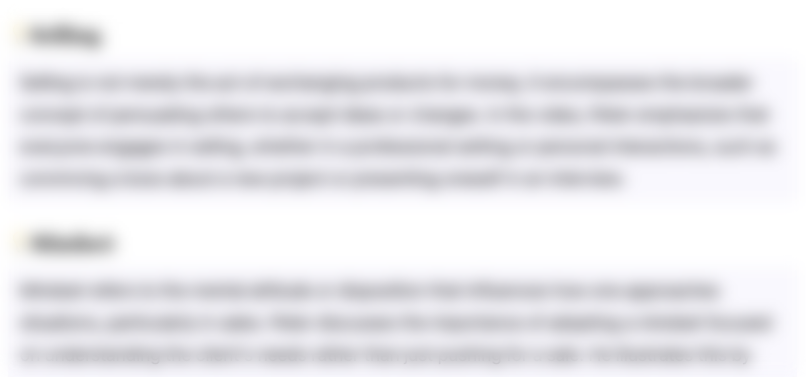
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
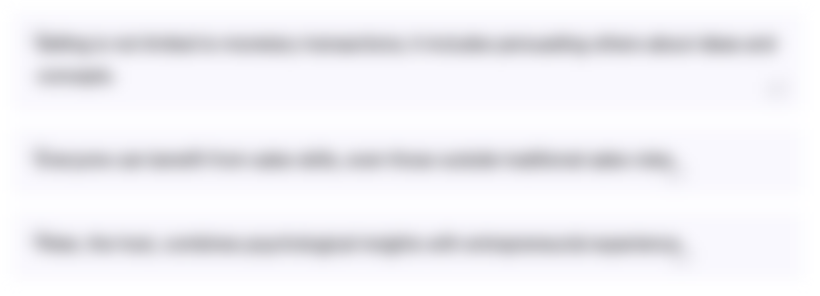
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
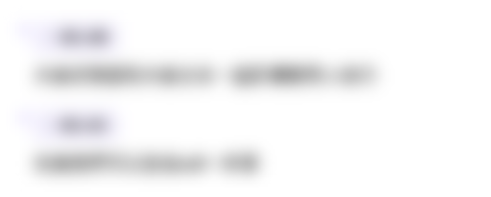
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)